篇博文主要是分析了C++ new()和构造函数的执行,delete()与析构函数的调用方式,通过全局重载和局部重载看清楚调用的关系
- using namespace std;
- #include <iostream>
- #include <cstdlib>
- class Student{
- public:
- Student(){
- cout << "Constructer" << endl;
- }
- ~Student(){
- cout << "析构函数" << endl;
- }
- static void* operator new(size_t size){
- cout << "own new function" << endl;
- Student* stu = (Student*)::operator new(size);
- return stu;
- }
- static void operator delete(void* p){
- cout << "own delete function" << endl;
- ::delete p;
- }
- static void* operator new[](size_t size){
- cout << "own new[] function" <<endl;
- return ::operator new(size);
- }
- };
- void* operator new(size_t size){
- cout << "global new function" << endl;
- void* p = malloc(size);
- return p;
- }
- void operator delete(void * p){
- cout << "global delete function"<< endl;
- free(p);
- }
- void operator delete[](void* p){
- cout << "global delete[] function" << endl;
- free(p);
- }
- void main(){
- Student* student = new Student();
- delete student;
- //Student* student2 = new Student[5];
- //delete[] student2;
- cin.get();
- }
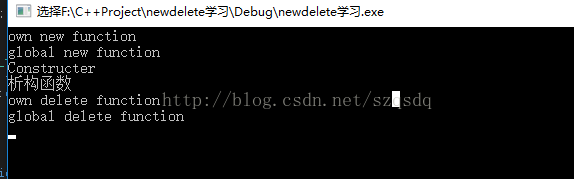
可以看出执行顺序为:内部重载的new-->全局重载的new()--->malloc()-->构造函数初始化-->内部的析构函数---》内部的delete()--》全局的delete()-->free()函数