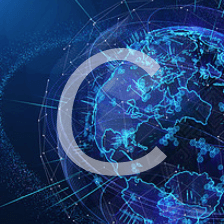
Leetcode
Roaring Kitty
供应链算法专家,量化交易爱好者,You Only Live Once!,技术交流:AllenYZXL
展开
-
66. Plus One - LeetCode
题目:Given a non-negative integer represented as a non-empty array of digits, plus one to the integer.You may assume the integer do not contain any leading zero, except the number 0 itself.T原创 2017-07-14 09:02:57 · 683 阅读 · 1 评论 -
Add Binary - LeetCode
题目:Given two binary strings, return their sum (also a binary string).For example,a = "11"b = "1"Return "100".思路:这道题目,主要思想就是从两个字符串尾部,逐位相加,并设置标志位,对一些特殊条件一定要注意,本身算法并不难,主要是if-else逻辑判断比较多原创 2017-07-14 12:22:41 · 269 阅读 · 0 评论 -
69. Sqrt(x)
题目:Implement int sqrt(int x).Compute and return the square root of x.思路:牛顿迭代法,公式:,最终公式:xi+1=xi - (xi2 - n) / (2xi) = xi - xi / 2 + n / (2xi) = xi / 2 + n / 2xi = (xi + n/xi) / 2,参考原创 2017-07-15 09:22:35 · 355 阅读 · 0 评论 -
70. Climbing Stairs
题目:You are climbing a stair case. It takes n steps to reach to the top.Each time you can either climb 1 or 2 steps. In how many distinct ways can you climb to the top?Note: Given n will原创 2017-07-15 10:26:26 · 314 阅读 · 0 评论 -
83. Remove Duplicates from Sorted List
题目:Given a sorted linked list, delete all duplicates such that each element appear only once.For example,Given 1->1->2, return 1->2.Given 1->1->2->3->3, return 1->2->3.思路:这道题目很简单的,就是原创 2017-07-15 11:43:12 · 217 阅读 · 0 评论 -
88. Merge Sorted Array
题目:Given two sorted integer arrays nums1 and nums2, merge nums2 into nums1 as one sorted array.Note:You may assume that nums1 has enough space (size that is greater or equal to m + n) to h原创 2017-07-16 11:09:11 · 296 阅读 · 0 评论 -
108. Convert Sorted Array to Binary Search Tree
题目:Given an array where elements are sorted in ascending order, convert it to a height balanced BST.思路:做这道题目前得理解高度平衡二叉树的定义:点击打开链接 ,本题解题思路还是从递归出发,但是要结合二叉查找树的定义,每次将数组二分中间值作为根节点,依次递归二分并将中间值原创 2017-07-20 10:59:01 · 867 阅读 · 0 评论 -
202. Happy Number
题目:Write an algorithm to determine if a number is "happy".A happy number is a number defined by the following process: Starting with any positive integer, replace the number by the sum of th原创 2017-07-30 14:52:05 · 451 阅读 · 0 评论 -
203. Remove Linked List Elements
题目:Remove all elements from a linked list of integers that have value val.ExampleGiven: 1 --> 2 --> 6 --> 3 --> 4 --> 5 --> 6, val = 6Return: 1 --> 2 --> 3 --> 4 --> 5思路:本题比较简单,主要考原创 2017-07-30 15:14:23 · 373 阅读 · 0 评论 -
204. Count Primes
题目:Description:Count the number of prime numbers less than a non-negative number, n.思路:本题主要采用埃拉托斯特尼筛法(详情请戳sieve of Eratosthenes)代码:class Solution {public: int countPrimes(int n)原创 2017-07-30 15:40:41 · 307 阅读 · 0 评论 -
206. Reverse Linked List
题目:Reverse a singly linked list.思路:递归的方法代码:/** * Definition for singly-linked list. * struct ListNode { * int val; * ListNode *next; * ListNode(int x) : val(x), next(NULL)原创 2017-07-30 16:49:00 · 302 阅读 · 0 评论 -
205. Isomorphic Strings
题目:Given two strings s and t, determine if they are isomorphic.Two strings are isomorphic if the characters in s can be replaced to get t.All occurrences of a character must be replaced原创 2017-07-30 17:13:57 · 368 阅读 · 0 评论 -
100. Same Tree
题目:Given two binary trees, write a function to check if they are equal or not.Two binary trees are considered equal if they are structurally identical and the nodes have the same value.思路:原创 2017-07-18 10:50:52 · 245 阅读 · 0 评论 -
217. Contains Duplicate
题目:Given an array of integers, find if the array contains any duplicates. Your function should return true if any value appears at least twice in the array, and it should return false if every ele原创 2017-07-31 08:54:25 · 386 阅读 · 0 评论 -
219. Contains Duplicate II
题目:Given an array of integers and an integer k, find out whether there are two distinct indices i and j in the array such that nums[i] = nums[j] and the absolute difference between i and j i原创 2017-07-31 09:03:54 · 321 阅读 · 0 评论 -
225. Implement Stack using Queues
题目:Implement the following operations of a stack using queues.push(x) -- Push element x onto stack.pop() -- Removes the element on top of the stack.top() -- Get the top element.empty() -- Re原创 2017-07-31 09:45:02 · 381 阅读 · 0 评论 -
107.Binary Tree Level Order Traversal II
题目:Given a binary tree, return the bottom-up level order traversal of its nodes' values. (ie, from left to right, level by level from leaf to root).For example:Given binary tree [3,9,20,nu原创 2017-07-19 11:32:12 · 310 阅读 · 0 评论 -
101. Symmetric Tree
题目:Given a binary tree, check whether it is a mirror of itself (ie, symmetric around its center).For example, this binary tree [1,2,2,3,4,4,3] is symmetric: 1 / \ 2 2 / \ / \3原创 2017-07-19 09:45:04 · 280 阅读 · 0 评论 -
104. Maximum Depth of Binary Tree
题目:Given a binary tree, find its maximum depth.The maximum depth is the number of nodes along the longest path from the root node down to the farthest leaf node.思路:本题思路有两种思路:1.递归法 2.层次法原创 2017-07-19 11:02:01 · 339 阅读 · 0 评论 -
110. Balanced Binary Tree
题目:Given a binary tree, determine if it is height-balanced.For this problem, a height-balanced binary tree is defined as a binary tree in which the depth of the two subtrees of every node ne原创 2017-07-23 09:20:07 · 350 阅读 · 0 评论 -
111. Minimum Depth of Binary Tree
题目:Given a binary tree, find its minimum depth.The minimum depth is the number of nodes along the shortest path from the root node down to the nearest leaf node.思路:本题思路递归,为了求最小值,那么在递归返回时原创 2017-07-23 09:46:23 · 238 阅读 · 0 评论 -
112. Path Sum
题目:Given a binary tree and a sum, determine if the tree has a root-to-leaf path such that adding up all the values along the path equals the given sum.For example:Given the below binary tree原创 2017-07-23 10:30:13 · 289 阅读 · 0 评论 -
371. Sum of Two Integers
题目:Calculate the sum of two integers a and b, but you are not allowed to use the operator + and -.Example:Given a = 1 and b = 2, return 3.思路:本题求和,条件是不允许使用操作符‘+’,那么一般来说,倾向的思路是使用位操作,梳理位操原创 2017-08-08 08:58:01 · 330 阅读 · 0 评论 -
342. Power of Four
题目:iven an integer (signed 32 bits), write a function to check whether it is a power of 4.Example:Given num = 16, return true. Given num = 5, return false.Follow up: Could you solve it原创 2017-08-06 09:21:32 · 332 阅读 · 0 评论 -
226. Invert Binary Tree
题目:Invert a binary tree. 4 / \ 2 7 / \ / \1 3 6 9to 4 / \ 7 2 / \ / \9 6 3 1思路:1.层次遍历法,每层遍历就替换左右结点;2.递归法代码(层次遍历法):class Solution {p原创 2017-08-01 11:26:28 · 229 阅读 · 0 评论 -
374. Guess Number Higher or Lower
题目:We are playing the Guess Game. The game is as follows:I pick a number from 1 to n. You have to guess which number I picked.Every time you guess wrong, I'll tell you whether the nu原创 2017-08-08 09:55:33 · 229 阅读 · 0 评论 -
383. Ransom Note
题目:Given an arbitrary ransom note string and another string containing letters from all the magazines, write a function that will return true if the ransom note can be constructed from the magaz原创 2017-08-08 10:05:32 · 353 阅读 · 0 评论 -
231. Power of Two
题目:Given an integer, write a function to determine if it is a power of two.思路:本题思路很简单就是递归的调用代码:class Solution {public: bool isPowerOfTwo(int n) { if(n==1) return原创 2017-08-01 13:38:22 · 349 阅读 · 0 评论 -
232. Implement Queue using Stacks
题目:Implement the following operations of a queue using stacks.push(x) -- Push element x to the back of queue.pop() -- Removes the element from in front of queue.peek() -- Get the front eleme原创 2017-08-01 13:46:44 · 240 阅读 · 0 评论 -
118. Pascal's Triangle
题目:Given numRows, generate the first numRows of Pascal's triangle.For example, given numRows = 5,Return[ [1], [1,1], [1,2,1], [1,3,3,1], [1,4,6,4,1]]思路:Pascal三角形,每一行除原创 2017-07-24 09:51:53 · 269 阅读 · 0 评论 -
119. Pascal's Triangle II
题目:Given an index k, return the kth row of the Pascal's triangle.For example, given k = 3,Return [1,3,3,1].Note:Could you optimize your algorithm to use only O(k) extra space?思路:本题原创 2017-07-24 10:06:40 · 244 阅读 · 0 评论 -
53. Maximum Subarray
题目:Find the contiguous subarray within an array (containing at least one number) which has the largest sum.For example, given the array [-2,1,-3,4,-1,2,1,-5,4],the contiguous subarray [4,-原创 2017-07-24 10:45:14 · 301 阅读 · 0 评论 -
121. Best Time to Buy and Sell Stock
题目:Say you have an array for which the ith element is the price of a given stock on day i.If you were only permitted to complete at most one transaction (ie, buy one and sell one share of th原创 2017-07-24 10:48:10 · 243 阅读 · 0 评论 -
234. Palindrome Linked List
题目:Given a singly linked list, determine if it is a palindrome.思路:遍历一次链表维护一个数组并存储对应value,再从头遍历链表的同时从尾部遍历数组,并进行比较代码:/** * Definition for singly-linked list. * struct ListNode { * int原创 2017-08-02 08:56:50 · 462 阅读 · 0 评论 -
235. Lowest Common Ancestor of a Binary Search Tree
题目:Given a binary search tree (BST), find the lowest common ancestor (LCA) of two given nodes in the BST.According to the definition of LCA on Wikipedia: “The lowest common ancestor is defin原创 2017-08-02 09:28:52 · 238 阅读 · 0 评论 -
242. Valid Anagram
题目:Given two strings s and t, write a function to determine if t is an anagram of s.For example,s = "anagram", t = "nagaram", return true.s = "rat", t = "car", return false.Note:Yo原创 2017-08-02 10:00:16 · 248 阅读 · 0 评论 -
387. First Unique Character in a String
题目:Given a string, find the first non-repeating character in it and return it's index. If it doesn't exist, return -1.Examples:s = "leetcode"return 0.s = "loveleetcode",return 2.原创 2017-08-09 09:14:36 · 217 阅读 · 0 评论 -
389. Find the Difference
题目:Given two strings s and t which consist of only lowercase letters.String t is generated by random shuffling string s and then add one more letter at a random position.Find the letter原创 2017-08-09 09:27:58 · 210 阅读 · 0 评论 -
400. Nth Digit
题目:Find the nth digit of the infinite integer sequence 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, ...Note:n is positive and will fit within the range of a 32-bit signed integer (n 31).Example原创 2017-08-09 09:49:38 · 266 阅读 · 0 评论 -
447. Number of Boomerangs
题目:Given n points in the plane that are all pairwise distinct, a "boomerang" is a tuple of points (i, j, k) such that the distance between iand j equals the distance between i and k (the o原创 2017-08-16 11:54:51 · 221 阅读 · 0 评论