- Color Random.ColorHSV() 颜色随机
- Vector3 Random.insideUnitCircle 圈内位置随机
- Vector3 Random.insideUnitSphere 球体内位置随机
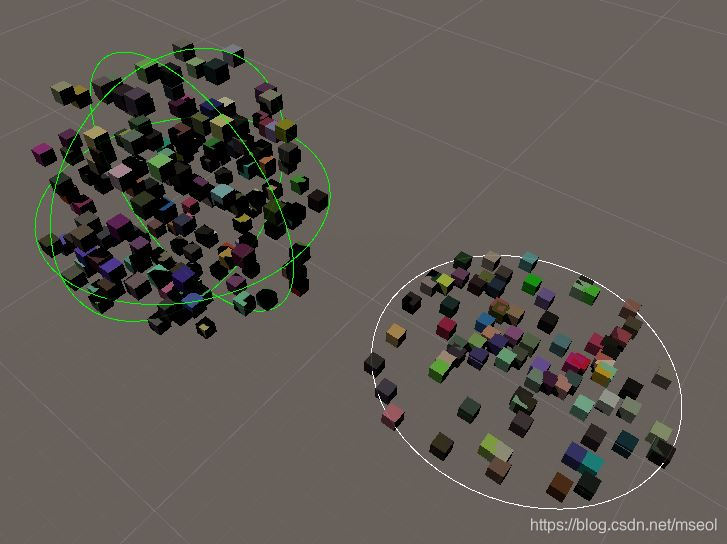
public class CircleRd : MonoBehaviour
{
[SerializeField] GameObject prefab;
[Range(0, 10), SerializeField] float radius = 5.0f;
void Update()
{
if (Input.GetKey(KeyCode.C))
InsideCircle();
}
void InsideCircle()
{
GameObject newCube = Instantiate(prefab);
newCube.transform.SetParent(transform);
Vector2 randomPos = Random.insideUnitCircle;
Vector3 newRandomPos = new Vector3(randomPos.x, 0, randomPos.y);
newCube.transform.localPosition = newRandomPos * radius;
newCube.GetComponent<MeshRenderer>().material.color = Random.ColorHSV();
}
void DrawCircle()
{
Gizmos.color = Color.white;
float theta = 0;
float x = radius * Mathf.Cos(theta);
float y = radius * Mathf.Sin(theta);
Vector3 pos = transform.position + new Vector3(x, 0, y);
Vector3 newPos = pos;
Vector3 lastPos = pos;
for (theta = 0.1f; theta < Mathf.PI * 2; theta += 0.1f)
{
x = radius * Mathf.Cos(theta);
y = radius * Mathf.Sin(theta);
newPos = transform.position + new Vector3(x, 0, y);
Gizmos.DrawLine(pos, newPos);
pos = newPos;
}
Gizmos.DrawLine(pos, lastPos);
}
void OnDrawGizmos()
{
DrawCircle();
}
}
public class SphereRd : MonoBehaviour
{
[SerializeField] GameObject prefab;
[Range(0, 10), SerializeField] float radius = 5.0f;
void Update()
{
if (Input.GetKey(KeyCode.S))
InsideSphere();
}
void InsideSphere()
{
GameObject newCube = Instantiate(prefab);
newCube.transform.SetParent(transform);
newCube.transform.localPosition = Random.insideUnitSphere * radius;
newCube.GetComponent<MeshRenderer>().material.color = Random.ColorHSV();
}
void DrawSphere()
{
Gizmos.color = Color.green;
Gizmos.DrawWireSphere(transform.position, radius);
}
void OnDrawGizmos()
{
DrawSphere();
}
}