using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Threading;
namespace ProjectTest
{
class WorkFlow
{
protected string _name;
protected uint _id;
public bool Statue;//启用/禁用
public string Name
{
get
{
return _name;
}
}
public uint Id
{
get
{
return _id;
}
}
}
class Steps:WorkFlow
{
protected uint _index=1;
public uint Index
{
get
{
return _index;
}
}
public uint StepIndex;
public List<Point> PointList=new List<Point>();
public Steps(string name, uint id)
{
this._name = name;
this._id = id;
}
public int StepWork()
{
while (Index<= PointsMaxIndex && Index!=99)
{
int i = PointsWork(GetPointsByIndex(Index));
if (i == 99)
Console.WriteLine("异常错误");
else
if (i == 0)
_index++;
else
_index =(uint)i;
}
return 0;
}
public List<Point> GetPointsByIndex(uint index )
{
List<Point> mpoints = new List<Point>();
foreach(Point i in PointList)
{
if (i.Index == index)
mpoints.Add(i);
}
return mpoints;
}
public uint PointsMaxIndex
{
get
{
uint Mindex = 1;
foreach (Point i in PointList)
{
if (i.Index >= Mindex)
Mindex = i.Index;
}
return Mindex;
}
}
private int PointsWork(List<Point> points)
{
List<Task<int>> TaskList=new List<Task<int>>();
foreach(var i in points)
{
Task<int> m = OnePointWorAscnic(i);
TaskList.Add(m);
}
foreach (var task in TaskList)
{
int j = task.Result;
if (j != 0)
{
_index = (uint)j;
Console.WriteLine(this.Name + "步骤跳转到-->:" + _index);
return j;
}
}
Console.WriteLine("**************************************");
return 0;
}
//异步线程
private async Task<int> OnePointWorAscnic(Point point)
{
Console.WriteLine(point.Name+"开始工作");
int m= await Task.Run(()=> point.PointWork());
return m;
}
}
class Point : WorkFlow
{
protected uint _index;
public uint Index
{
get
{
return _index;
}
}
private string StrActMode;
List<string> parms;
public Point(string name,uint index,string StrActMode = "",List<string> parms=null)
{
this._name = name;
this._index = index;
this.StrActMode = StrActMode;
this.parms = parms;
}
public int PointWork()
{
int i = 20;
while (i > 0)
{
Thread.Sleep(100);
i--;
}
Action ab = (Action)Assembly.Load("ProjectTest").CreateInstance("ProjectTest."+StrActMode);
return ab.work(parms);
}
}
}
class Program
{
static void Main(string[] args)
{
Steps Mstep = new Steps("测试步骤",1);
Point point1 = new Point("步骤1--》",1, "ActPos",new List<string> { "位置1", "10"});
Point point2 = new Point("步骤2--》", 2, "ActPos", new List<string> { "位置1", "10" });
Point point3 = new Point("步骤3--》", 2, "ActPos", new List<string> { "位置2", "10" });
Point point4 = new Point("步骤4--》", 3, "ActPos", new List<string> { "位置3", "10" });
Point point5 = new Point("步骤8--》", 7, "ActPos", new List<string> { "位置4", "10" });
Point point6 = new Point("步骤6--》", 5, "ActIoSet", new List<string> { "气缸1", "SetON" });
Point point7 = new Point("步骤7--》", 6, "ActIoSet", new List<string> { "气缸2", "SetOFF" });
Point point8 = new Point("步骤5--》", 4, "ActIoCheck", new List<string> { "气缸2", "CheckON" ,"7","2"});
Mstep.PointList.Add(point1);
Mstep.PointList.Add(point2);
Mstep.PointList.Add(point3);
Mstep.PointList.Add(point4);
Mstep.PointList.Add(point5);
Mstep.PointList.Add(point6);
Mstep.PointList.Add(point7);
Mstep.PointList.Add(point8);
Mstep.StepWork();
Console.Read();
}
}
abstract class Action
{
protected List<string> parms;
public abstract int work(List<string> parms);
}
class ActPos: Action
{
public Pos mpos;
public ActPos()
{
}
public override int work(List<string> parms)
{
this.parms = parms;
string PosName = parms[0];
this.mpos = Motion.GetPosByName(PosName);
return mpos.MoveTo(parms[1]);
}
}
/// <summary>
/// IO运动
/// </summary>
class ActIoSet: Action
{
public SetIO MyIO;
public ActIoSet()
{
}
public override int work(List<string> parms)
{
this.parms = parms;
string PosName = parms[0];
this.MyIO = Motion.GetIOByName(PosName);
return MyIO.Set(parms[1]);
}
}
class ActIoCheck : Action
{
public SetIO MyIO;
public override int work(List<string> parms)
{
this.parms = parms;
string PosName = parms[0];
this.MyIO = Motion.GetIOByName(PosName);
int ret= MyIO.Check(parms[1]);
if (ret == 0)//检测成功跳转步骤到参数2
return Convert.ToInt32(parms[2]);
else//检测失败跳转步骤到参数3
return Convert.ToInt32(parms[3]);
}
}
class Pos
{
private string name;
public string Name
{
get { return name; }
}
private uint _id;
public uint Id
{
get
{
return _id;
}
}
private uint _index;
public uint Index
{
get
{
return _index;
}
}
public Pos(string name,uint id,uint index)
{
this.name = name;
this._id = id;
}
public int MoveTo(String WaitTime)
{
Console.WriteLine(Name + "执行定位运动");
Console.WriteLine("等待时间:" + WaitTime + "S");
return 0;
}
}
class SetIO
{
private string name;
public string Name
{
get { return name; }
}
private uint _id;
public uint Id
{
get
{
return _id;
}
}
private uint _index;
public uint Index
{
get
{
return _index;
}
}
public SetIO(string name, uint id, uint index)
{
this.name = name;
this._id = id;
this._index = index;
}
public int Set(String WorkType)
{
if (WorkType == "SetON")
Console.WriteLine(Name + "打开");
else if (WorkType == "SetOFF")
Console.WriteLine(Name + "关闭");
else
{
Console.WriteLine(Name + "命令错误");
return 1;
}
return 0;
}
public int Check(String WorkType)
{
if (WorkType == "CheckON")
Console.WriteLine(Name + "检测打开");
else if (WorkType == "CheckOFF")
Console.WriteLine(Name + "检测关闭");
else
{
Console.WriteLine(Name + "命令错误");
return 1;
}
return 0;
}
}
class Motion
{
private static Pos pos1 = new Pos("位置1", 1, 1);
private static Pos pos2 = new Pos("位置2", 2, 2);
private static Pos pos3 = new Pos("位置3", 3, 3);
private static Pos pos4 = new Pos("位置4", 4, 4);
private static List<Pos> PosList = new List<Pos> { pos1, pos2, pos3, pos4 };
public static Pos GetPosByName(string name)
{
foreach (var pos in PosList)
{
if (pos.Name == name)
return pos;
}
return null;
}
private static SetIO IO1 = new SetIO("气缸1", 1, 1);
private static SetIO IO2 = new SetIO("气缸2", 2, 2);
private static SetIO IO3 = new SetIO("气缸3", 3, 3);
private static SetIO IO4 = new SetIO("气缸4", 4, 4);
private static List<SetIO> IOList = new List<SetIO> { IO1, IO2, IO3, IO4 };
public static SetIO GetIOByName(string name)
{
foreach (var io in IOList)
{
if (io.Name == name)
return io;
}
return null;
}
}
```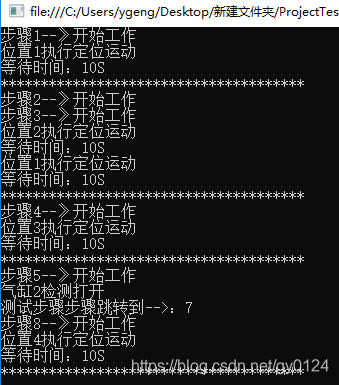
界面实现自动化编程
最新推荐文章于 2024-06-06 09:31:39 发布