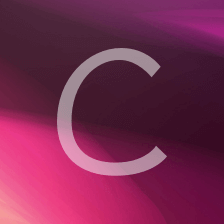
LeetCode (Google面试算法200题)
flyljg
正在成长中
展开
-
Leetcode: Sqrt(x)
Implement int sqrt(int x).Compute and return the square root of x.1. 二分法:用unsigned long long。最后返回值还要再检查一下。class Solution {public: int mySqrt(int x) { unsigned long long be原创 2015-08-09 18:04:34 · 605 阅读 · 0 评论 -
Single Number(找出只出现了一次的数,其它数都出现两次)
题目描述:找出只是出现一次的数字,其他的数字都出现两次。思路:根据x^x=0,x^y=y^x。只要将所有数异或就完成了。代码实现:class Solution{public: int singleNumber(vector &nums){ int x=0; for(int i=0;i<nums.size();i++){原创 2015-08-01 15:40:34 · 388 阅读 · 0 评论 -
Length of Last Word(返回最后一个字母的长度)
题目描述:Given a string s consists of upper/lower-case alphabets and empty space characters ' ', return the length of last word in the string.If the last word does not exist, return 0.Note原创 2015-08-01 18:23:45 · 542 阅读 · 0 评论 -
Valid Anagram
题目描述:Given two strings s and t, write a function to determine if t is an anagram of s.For example,s = "anagram", t = "nagaram", return true.s = "rat", t = "car", return false.Note:原创 2015-08-02 12:13:17 · 517 阅读 · 0 评论 -
Invert Binary Tree
题目描述:Invert a binary tree. 4 / \ 2 7 / \ / \1 3 6 9to 4 / \ 7 2 / \ / \9 6 3 1Trivia:This problem was inspired by this original tweet by原创 2015-08-02 13:21:55 · 301 阅读 · 0 评论 -
leetcode: Add Two Numbers
You are given two linked lists representing two non-negative numbers. The digits are stored in reverse order and each of their nodes contain a single digit. Add the two numbers and return it as a link原创 2015-08-14 10:59:38 · 451 阅读 · 0 评论 -
leetcode:Remove Nth Node From End of List
Given a linked list, remove the nth node from the end of list and return its head.For example, Given linked list: 1->2->3->4->5, and n = 2. After removing the second node from the end, the原创 2015-08-14 16:38:50 · 586 阅读 · 0 评论 -
leetcode : Balanced Binary Tree
Given a binary tree, determine if it is height-balanced.For this problem, a height-balanced binary tree is defined as a binary tree in which the depth of the two subtrees of every node never diffe原创 2015-09-01 20:41:22 · 384 阅读 · 0 评论 -
Remove Duplicates from Sorted List
Given a sorted linked list, delete all duplicates such that each element appear only once.For example,Given 1->1->2, return 1->2.Given 1->1->2->3->3, return 1->2->3.思路:算法没什么好说的,链表实现一个功能:原创 2015-08-07 12:35:04 · 358 阅读 · 0 评论 -
leetcode: Binary Tree Maximum Path Sum
Given a binary tree, find the maximum path sum.The path may start and end at any node in the tree.For example:Given the below binary tree, 1 / \ 2 3Return 6.原创 2015-09-02 17:24:40 · 348 阅读 · 0 评论 -
leetcode: Merge Sorted Array
Given two sorted integer arrays nums1 and nums2, merge nums2 into nums1 as one sorted array.Note:You may assume that nums1 has enough space (size that is greater or equal to m + n) to hold addit原创 2015-08-21 09:24:12 · 462 阅读 · 0 评论 -
leetcode: Minimum Depth of Binary Tree
Given a binary tree, find its minimum depth.The minimum depth is the number of nodes along the shortest path from the root node down to the nearest leaf node.题目描述: 求二叉树的最小深度。和求原创 2015-08-21 09:44:24 · 477 阅读 · 0 评论 -
leetcode: Add Digits
Given a non-negative integer num, repeatedly add all its digits until the result has only one digit.For example:Given num = 38, the process is like: 3 + 8 = 11, 1 + 1 = 2. Since 2 has on原创 2015-08-21 15:18:56 · 639 阅读 · 0 评论 -
leetcode: Excel Sheet Column Number
Related to question Excel Sheet Column TitleGiven a column title as appear in an Excel sheet, return its corresponding column number.For example: A -> 1 B -> 2 C -> 3 ...原创 2015-08-21 15:56:41 · 640 阅读 · 0 评论 -
leetcode: Excel Sheet Column Title
Given a positive integer, return its corresponding column title as appear in an Excel sheet.For example: 1 -> A 2 -> B 3 -> C ... 26 -> Z 27 -> AA 28 -> AB Credits原创 2015-08-21 17:20:44 · 557 阅读 · 0 评论 -
一棵完全二叉树,找到每个节点在这一层右边的点(Populating Next Right Pointers in Each Node)
题目描述:给定一个完全二叉树,找到每个节点在这一层右边的点。思路:直接DFS。将每个点左儿子向右全部指向右儿子向左上对应的节点。代码实现:/** * Definition for binary tree with next pointer. * struct TreeLinkNode { * int val; * TreeLinkNode *left原创 2015-07-31 20:07:40 · 720 阅读 · 0 评论 -
判断一个字符串是否是另一个字符串的子串(Implement strStr() )
问题描述:这是算法中比较经典的问题,判断一个字符串是否是另一个字符串的字串。思路:这个题目最经典的算法应该是KMP算法,KMP算法是最优的线性算法,复杂度已经达到了这个问题的下线。但是KMP算法的过程比较复杂,在面试过程中很难在很短的时间内实现,所以在一般的面试中很少让实现KMP算法。代码实现:class Solution {public: int strSt原创 2015-08-01 17:23:12 · 4013 阅读 · 0 评论 -
逆波兰表达式求值(Evaluate Reverse Polish Notation)
问题描述:逆波兰表达式求值,经典问题。思路:遇到数字入栈,遇到符号取栈顶的两个元素出来,再将结果入栈,最后栈里剩下的元素就是结果了。实现代码:class Solution {public: int evalRPN(vector& tokens) { stack st; for(auto &s:tokens){原创 2015-08-01 15:28:24 · 475 阅读 · 0 评论 -
Leetcode: Max Points on a Line
Given n points on a 2D plane, find the maximum number of points that lie on the same straight line.分析:任意一条直线都可以表述为y = ax + b假设,有两个点(x1,y1), (x2,y2),如果他们都在这条直线上则有y1 = kx1 +b原创 2015-08-09 19:09:46 · 460 阅读 · 0 评论 -
leetcode:LRU Cache
Design and implement a data structure for Least Recently Used (LRU) cache. It should support the following operations: get and set.get(key) - Get the value (will always be positive) of the key if原创 2015-08-10 19:33:17 · 499 阅读 · 0 评论 -
leetcode: Binary Tree Preorder Traversal
Given a binary tree, return the preorder traversal of its nodes' values.For example:Given binary tree {1,#,2,3}, 1 \ 2 / 3return [1,2,3].Note: Recursive soluti原创 2015-08-11 21:22:56 · 469 阅读 · 0 评论 -
leetcode: Binary Tree Preorder Traversal
Given a binary tree, return the preorder traversal of its nodes' values.For example:Given binary tree {1,#,2,3}, 1 \ 2 / 3return [1,2,3].Note: Recursive soluti原创 2015-08-26 17:17:02 · 481 阅读 · 0 评论 -
leetcode: Binary Tree Postorder Traversal
Given a binary tree, return the inorder traversal of its nodes' values.For example:Given binary tree {1,#,2,3}, 1 \ 2 / 3return [1,3,2].Note: Recursive solutio原创 2015-08-26 17:31:52 · 552 阅读 · 0 评论 -
leetcode: Valid Parentheses
题目描述:Given a string containing just the characters '(', ')', '{', '}', '[' and ']', determine if the input string is valid.The brackets must close in the correct order, "()" and "()[]{}" are原创 2015-08-11 19:41:29 · 740 阅读 · 0 评论 -
有序数组转换为平衡二叉搜索树(Convert Sorted Array to Binary Search Tree)
题目描述:给定一个有序数组,数组元素升序排列,将该有序数组转换为一个平衡二叉搜索树。思路:首先,这个问题很容易用递归方法来解决。所谓的平衡的定义:就是二叉树的子树高度之差不能超过1。如果要从一个有序数组中选择一个元素作为根节点,首先应该用二分查找算法,对数组进行均匀换分,然后将有序数组的中间元素作为根节点。选择中间元素作为根节点并创建之后,剩下的元素分为两部分,可以看做是两个数组,原创 2015-07-31 11:25:08 · 606 阅读 · 2 评论 -
从根到叶的每条路径构成一个数,求这些数的和(Sum Root to Leaf Numbers)
题目描述:从根到叶子的每条路径构成一个数,求这些数的和。思路:直接DFS,每次到叶子加就可以了。代码实现:/** * Definition for a binary tree node. * struct TreeNode { * int val; * TreeNode *left; * TreeNode *right; *原创 2015-07-31 12:31:03 · 678 阅读 · 2 评论 -
leetcode: Binary Tree Postorder Traversal
Given a binary tree, return the postorder traversal of its nodes' values.For example:Given binary tree {1,#,2,3}, 1 \ 2 / 3return [3,2,1].Note: Recursive solut原创 2015-08-26 17:48:25 · 590 阅读 · 0 评论 -
判断是否是回文串(Valid Palindrome)
题目描述:判断一个字符串是否是回文串。注意只有大小写字母和数字才算是串的内容,其他字符都跳过。思路:左右两个指针逐渐往中间逼近就可以了。isalnum()函数是判断是否是字母数字的,其他字符跳过;tolower()函数是将字母数字都转换成小写进行判断。算法实现:class Solution{public: bool isPalindrome(str原创 2015-07-31 12:08:37 · 720 阅读 · 2 评论 -
求树上有没有一条从根到叶子的路节点的值加起来为N(Path Sum)
题目描述:求树上有没有从根节点到叶子的路节点的值加起来为N。思路:DFS。到叶子判断累加值是否等于SUM。代码实现:/** * Definition for a binary tree node. * struct TreeNode { * int val; * TreeNode *left; * TreeNode *right原创 2015-07-31 22:57:00 · 628 阅读 · 0 评论 -
leetcode: Maximum Depth of Binary Tree
Given a binary tree, find its maximum depth.The maximum depth is the number of nodes along the longest path from the root node down to the farthest leaf node.思路:递归求解二叉树的深度,左右子树较深的+1(;解释主要原原创 2015-08-12 21:49:44 · 583 阅读 · 0 评论 -
leetcode: Same Tree
Given two binary trees, write a function to check if they are equal or not.Two binary trees are considered equal if they are structurally identical and the nodes have the same value.题目描述:原创 2015-08-27 16:43:41 · 568 阅读 · 0 评论 -
leetcode: Symmetric Tree
Given a binary tree, check whether it is a mirror of itself (ie, symmetric around its center).For example, this binary tree is symmetric: 1 / \ 2 2 / \ / \3 4 4 3But the f原创 2015-08-27 17:46:14 · 492 阅读 · 0 评论 -
leetcode——Move Zeroes
Given an array nums, write a function to move all 0's to the end of it while maintaining the relative order of the non-zero elements.For example, given nums = [0, 1, 0, 3, 12], after calling you原创 2015-09-22 16:07:01 · 410 阅读 · 0 评论