O_EXCL 如果同时指定OCREAT,而文件已经存在,则出错,也就是返回-1
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <stdio.h>
int main()
{
int fd;
fd = open("./file1",O_RDWR|O_CREAT|O_EXCL,0600);
if(fd == -1){
printf("file cunzai\n");
}
return 0;
}

O_APPEND:每次写时都会加到文件的尾端
例如我的file1文件现有如下内容
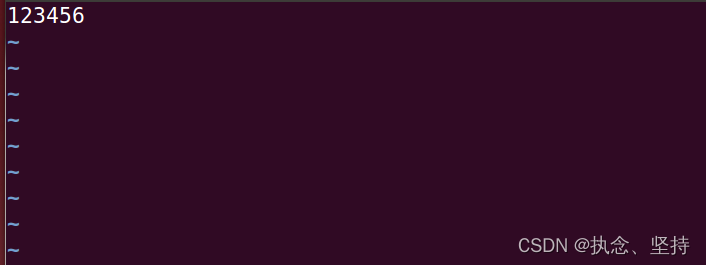
当使用代码往file1写入内容时,会出现:
include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <stdio.h>
#include <unistd.h>
#include <string.h>
#include <stdlib.h>
int main()
{
int fd;
char *buf = "hello dzz hei shuai";
fd = open("./file1",O_RDWR|O_APPEND);
printf("open susccess : fd =%d\n",fd);
int n_write = write(fd, buf, strlen(buf));
if(n_write != -1){
printf("write %d byte to file \n", n_write);
}
close(fd);
return 0;
}
再次查看可以看到
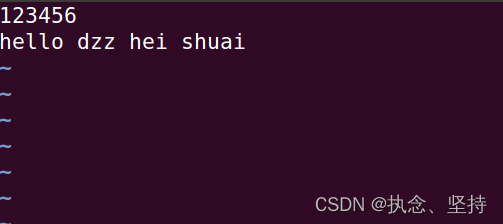
会把内容加到末尾,如果不使用O_APPEND则会出现覆盖现象
O_TRUNC:用该属性去打开文件是,如果这个文件中本来就有内容,而且为只读或只写成功打开,则将其长度截短为0。(也就是把原有的内容删掉,再添加新的)
例如,现在我的file1内容如下
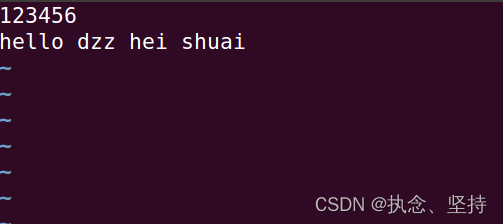
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <stdio.h>
#include <unistd.h>
#include <string.h>
#include <stdlib.h>
int main()
{
int fd;
char *buf = "test";
fd = open("./file1",O_RDWR|O_TRUNC); // 使用了O_TRUNC
printf("open susccess : fd =%d\n",fd);
int n_write = write(fd, buf, strlen(buf));
if(n_write != -1){
printf("write %d byte to file \n", n_write);
}
close(fd);
return 0;
}
现在再看file1
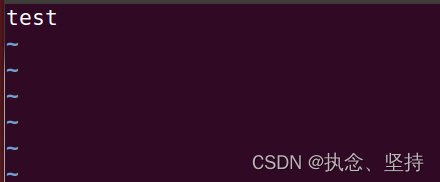
结果明显就是把原有的内容删除掉,再写入新的内容。