Prime Ring Problem
Time Limit: 4000/2000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)Total Submission(s): 37378 Accepted Submission(s): 16494
Problem Description
A ring is compose of n circles as shown in diagram. Put natural number 1, 2, ..., n into each circle separately, and the sum of numbers in two adjacent circles should be a prime.
Note: the number of first circle should always be 1.
Note: the number of first circle should always be 1.
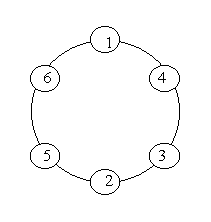
Input
n (0 < n < 20).
Output
The output format is shown as sample below. Each row represents a series of circle numbers in the ring beginning from 1 clockwisely and anticlockwisely. The order of numbers must satisfy the above requirements. Print solutions in lexicographical order.
You are to write a program that completes above process.
Print a blank line after each case.
You are to write a program that completes above process.
Print a blank line after each case.
Sample Input
6 8
Sample Output
Case 1: 1 4 3 2 5 6 1 6 5 2 3 4 Case 2: 1 2 3 8 5 6 7 4 1 2 5 8 3 4 7 6 1 4 7 6 5 8 3 2 1 6 7 4 3 8 5 2#include <cstdio> #include <cstring> #include <iostream> using namespace std; int n,h,prime[15] = {3,5,7,11,13,17,19,23,29,31,37}; //找出可能存在的最大的素数 int vis[25],s[25]={0,1}; bool check(int x,int y) //判断相邻的2个数之和是否为素数 { int i; for(i = 0; i < 11;i++) if(s[x] + s[y] == prime[i]) break; if(i == 11) return false; else return true; } void DFS(int k,int n) { if(k == n&&check(1,n)) //如果找到则输出 { for(int i = 1 ; i <= n; i++) { if(i != n) printf("%d ",s[i]); else printf("%d",s[i]); } cout << endl; return ; } for(int i = 2; i <= n; i++) //从2开始找到合适的书,因为1已经在s[]数组里面 { if(!vis[i]) //判断此数是否已经被标记过 { s[++h] = i; vis[i] = 1; if(check(h-1,h)) //这一步的判断相当于剪枝 DFS(k + 1,n); s[h--] = 0; //回溯 vis[i] = 0; } } } int main() { int flag = 0; while(scanf("%d",&n) != EOF) { printf("Case %d:\n",++flag); memset(vis,0,sizeof(vis)); h = 1; //对全局变量进行更新 DFS(1,n); cout << endl; //别忘了此处还有一个换行符 } return 0; }