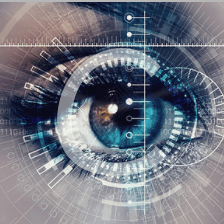
LeetCode
ziyue246
简单,安静
展开
-
Leetcode 873. 最长的斐波那契子序列的长度
LeetCode每日打卡 :动态规划+hash表原创 2022-07-09 23:35:51 · 268 阅读 · 0 评论 -
LeetCode 53. Maximum Subarray 时间复杂度(O(n))
class Solution: def maxSubArray(self, nums: [int]) -> int: res = nums[0] res_max = res for num in nums[1:]: res = num if res < 0 else res + num res_max = max(res_max, res) return res_max原创 2022-01-26 00:18:39 · 363 阅读 · 0 评论 -
LeetCode 42. Trapping Rain Water 时间复杂度(O(n))
class Solution: def trap(self, height: List[int]) -> int: def one_search(height): pre_v = 0 res = [] for index, h in enumerate(height): if index == 0: pre_v = h .原创 2022-01-25 22:32:44 · 4743 阅读 · 0 评论 -
139. Word Break 时间复杂度(O(n))
时间复杂度(O(n*m))思想:动态规划class Solution: def wordBreak(self, s: str, wordDict: [str]) -> bool: if len(s) == 0: return True words = set(wordDict) res = [False] * len(s) for i in range(len(s)): if i > 0 .原创 2020-09-24 22:53:22 · 359 阅读 · 0 评论 -
474. Ones and Zeroes 时间复杂度(O(k*m*n))
时间复杂度(O(k*m*n)),思想:动态规划,0,1背包问题class Solution: def findMaxForm(self, strs: [str], m: int, n: int) -> int: dp = [[0] * (n + 1) for _ in range((m + 1))] for item in strs: c0 = item.count('0') c1 = item.count.原创 2020-09-14 19:28:13 · 154 阅读 · 0 评论 -
142. Linked List Cycle II 时间复杂度(O(n))
时间复杂度(O(n)),思想:快慢指针,位置记录# Definition for singly-linked list.# class ListNode:# def __init__(self, x):# self.val = x# self.next = Noneclass Solution(object): def detectCycle(self, head: ListNode) -> ListNode: p,.原创 2020-09-05 18:34:30 · 143 阅读 · 0 评论 -
138. Copy List with Random Pointer 时间复杂度(O(n))
时间复杂度(O(n)) 思想:先插再去"""# Definition for a Node.class Node: def __init__(self, x: int, next: 'Node' = None, random: 'Node' = None): self.val = int(x) self.next = next self.random = random"""class Solution: def copyRa..原创 2020-09-05 17:57:52 · 136 阅读 · 0 评论 -
124. Binary Tree Maximum Path Sum 时间复杂度(O(n))
时间复杂度(O(n)),思想:后序遍历# Definition for a binary tree node.# class TreeNode:# def __init__(self, val=0, left=None, right=None):# self.val = val# self.left = left# self.right = rightclass Solution: def maxPathSum(self..原创 2020-09-05 15:26:22 · 157 阅读 · 0 评论 -
128. Longest Consecutive Sequence 时间复杂度(O(n))
时间复杂度(O(n)),思想:hash_setclass Solution: def longestConsecutive(self, nums: [int]) -> int: num_set, dup_num_set, max_len = set(nums), set(), 0 for num in nums: curr_len = 1 if num in dup_num_set: ..原创 2020-09-05 15:04:50 · 79 阅读 · 0 评论 -
114. Flatten Binary Tree to Linked List 时间复杂度(O(n))
时间复杂度(O(n)),思想:move# Definition for a binary tree node.# class TreeNode:# def __init__(self, val=0, left=None, right=None):# self.val = val# self.left = left# self.right = rightclass Solution: def flatten(self, roo.原创 2020-08-30 17:20:19 · 95 阅读 · 0 评论 -
98. Validate Binary Search Tree 时间复杂度(O(n))
时间复杂度(O(n)),思想:中序遍历# Definition for a binary tree node.# class TreeNode:# def __init__(self, val=0, left=None, right=None):# self.val = val# self.left = left# self.right = rightclass Solution: def isValidBST(self,原创 2020-08-30 13:09:43 · 138 阅读 · 0 评论 -
96. Unique Binary Search Trees 时间复杂度(O(n))
时间复杂度(O(n)),思想:动态规划class Solution: def numTrees(self, n: int) -> int: ts = [1, 1] for i in range(1, n): curr_num = 0 for j in range(i // 2 + 1): dup = 1 if j == i - j else 2 ..原创 2020-08-29 11:22:06 · 297 阅读 · 0 评论 -
85. Maximal Rectangle 时间复杂度(O(n))
时间复杂度(O(n)),思想:栈,同84. Largest Rectangle in Histogram 时间复杂度(O(n))https://blog.youkuaiyun.com/ziyue246/article/details/108251988class Solution: def maximalRectangle(self, matrix: [[str]]) -> int: if len(matrix) == 0: return 0 def lar.原创 2020-08-27 11:14:27 · 103 阅读 · 0 评论 -
84. Largest Rectangle in Histogram 时间复杂度(O(n))
时间复杂度(O(n)),思想:栈class Solution: def largestRectangleArea(self, heights: [int]) -> int: area, max_area = [], 0 for i, h in enumerate(heights): start = i while len(area) > 0 and area[-1][1] > h: .原创 2020-08-27 08:12:50 · 115 阅读 · 0 评论 -
LeetCode 79. Word Search 时间复杂度(O(n))
时间复杂度(O(n)),思想:深度优先遍历class Solution: def exist(self, board: [[str]], word: str) -> bool: board_pos = {} for i in range(len(board)): for j in range(len(board[i])): ch = board[i][j] if ch.原创 2020-08-26 19:47:18 · 191 阅读 · 0 评论 -
494. Target Sum 时间复杂度(O(2^n))
思想:动态规划,提示一点,虽然时间复杂度是(O(2^n)),但是实际上在动态规划中,有些步数会得到优化import collectionsclass Solution: def findTargetSumWays(self, nums: [int], S: int) -> int: dp = {0: 1} for num in nums: ...原创 2019-11-14 20:50:10 · 544 阅读 · 0 评论 -
LeetCode 406. Queue Reconstruction by Height 时间复杂度(O(n))
时间复杂度(O(n)),思想:排序,规律from collections import dequeclass Solution: def reconstructQueue(self, people: [[]]) -> [[]]: people.sort(key=lambda k: k[0] + 1 / (k[1] + 1), reverse=True)...原创 2019-11-09 08:01:09 · 118 阅读 · 0 评论 -
LeetCode 15. 3Sum 时间复杂度(O( n2))
时间复杂度O( ) class Solution {public: vector<vector<int>> threeSum(vector<int>& nums) { for(int i=0;i<nums.size();++i){ for(int j=0;j<nums.size()...原创 2018-07-26 12:56:52 · 396 阅读 · 0 评论 -
LeetCode 16. 3Sum Closest 时间复杂度(O( n2))
时间复杂度O( ) class Solution {public: int threeSumClosest(vector<int>& nums, int target) { if(nums.size()<3)return -1; for(int i=0;i<nums.size();++i){ ...原创 2018-07-26 13:01:59 · 196 阅读 · 0 评论 -
LeetCode 17. Letter Combinations of a Phone Number 时间复杂度(O( k*n))
时间复杂度(O( k*n)) class Solution {public: vector<string> letterCombinations(string digits) { vector<string> result ; if(digits=="")return result; letter(digits,...原创 2018-07-26 13:04:21 · 297 阅读 · 0 评论 -
LeetCode 11. Container With Most Water 时间复杂度(O( n2))
时间复杂度O( ) ,空间复杂度O(n)class Solution {public: int maxArea(vector<int>& height) { vector<int> currCon(height.size(),0); for(int i=0;i<height.size();++i){ ...原创 2018-07-26 13:06:51 · 99 阅读 · 0 评论 -
LeetCode 10. Regular Expression Matching 时间复杂度(O( n))
时间复杂度(O( n)),思想:动态规划,递归不好意思,代码修修补补,写的比较乱class Solution {public: bool isMatch(string s, string p) { string pp = ""; for(int i=0;i<p.length();++i){ if(p.at(i)=='...原创 2018-07-26 13:20:37 · 290 阅读 · 0 评论 -
LeetCode 19. Remove Nth Node From End of List 时间复杂度(O( n))
时间复杂度(O( n))/** * Definition for singly-linked list. * struct ListNode { * int val; * ListNode *next; * ListNode(int x) : val(x), next(NULL) {} * }; */class Solution {public: ...原创 2018-07-26 15:33:31 · 94 阅读 · 0 评论 -
LeetCode 20. Valid Parentheses 时间复杂度(O( n))
时间复杂度(O( n))class Solution {public: bool isValid(string s) { if(s.length()%2==1)return false; vector<char> vec_ch ; for(int i=0;i<s.length();++i){ ...原创 2018-07-26 16:31:08 · 224 阅读 · 0 评论 -
LeetCode 23. Merge k Sorted Lists 时间复杂度(O( n*logk))
时间复杂度(O( )) 思想:归并排序class Solution {public: ListNode* mergeKLists(vector<ListNode*>& lists) { vector<ListNode*>::iterator itr = lists.begin(); while (i...原创 2018-07-26 18:29:47 · 291 阅读 · 0 评论 -
LeetCode 21. Merge Two Sorted Lists 时间复杂度(O( n))
时间复杂度(O( n)) 先写了23又回来刷了一下21,^_^class Solution {public: ListNode* mergeTwoLists(ListNode* l1, ListNode* l2) { if(l1==NULL) return l2; if(l2==NULL) return l1; ...原创 2018-07-26 18:39:30 · 302 阅读 · 0 评论 -
LeetCode 25. Reverse Nodes in k-Group 时间复杂度(O( n))
时间复杂度(O( n)) class Solution {public: ListNode* reverseKGroup(ListNode* head, int k) { if(k<=1||head==NULL)return head; ListNode* node = head; int len = 0; ...原创 2018-07-26 19:33:55 · 115 阅读 · 0 评论 -
LeetCode 29. Divide Two Integers 时间复杂度(O(1))
时间复杂度(O(1))该题要求不让用乘、除、取模运算,等有时间我再补上正确答案。class Solution {public: int divide(int dividend, int divisor) { if(dividend==-2147483648 && divisor==1)return -2147483648; if(...原创 2018-07-27 09:15:58 · 131 阅读 · 0 评论 -
LeetCode 123. Best Time to Buy and Sell Stock III 时间复杂度(O(n2))
时间复杂度(O( n2)),感觉效率有些低,等有时间再想想有木有其他方法优化。class Solution {public: int maxProfit(vector<int>& prices) { if(prices.size()<2)return 0; int max = onemaxProfit(prices,0,pr...原创 2018-08-03 16:54:03 · 105 阅读 · 0 评论 -
LeetCode 136. Single Number 时间复杂度(O( n))
时间复杂度(O( n))class Solution {public: int singleNumber(vector<int>& nums) { if(nums.size()==0) return 0; int result = 0; for(int i=0;i < nums.size();i++) ...原创 2018-08-03 16:59:40 · 153 阅读 · 0 评论 -
LeetCode 137. Single Number II 时间复杂度(O( n))
时间复杂度(O( n)),思想,位运算 class Solution {public: int singleNumber(vector<int>& nums) { vector<int> bits(64,0); for(int i=0;i<nums.size();++i) for(int j=0;j<bits...原创 2018-08-03 17:37:50 · 134 阅读 · 0 评论 -
LeetCode 32. Longest Valid Parentheses 时间复杂度(O(n))
1、时间复杂度(O(n^2)),思想,暴力查找class Solution {public: int longestValidParentheses(string s) { int maxLen=0; for(int index=0;index< s.size();++index){ vector<char>...原创 2018-07-30 13:47:31 · 108 阅读 · 0 评论 -
LeetCode 155. Min Stack 时间复杂度(O( n))
时间复杂度(O( n))class MinStack {public: vector<int> vec; vector<int> vecmin; MinStack() { } void push(int x) { vec.push_back(x); if(vecmin.empty()) ...原创 2018-08-03 18:07:05 · 213 阅读 · 0 评论 -
LeetCode 198. House Robber 时间复杂度(O( n))
时间复杂度(O( n)),思想,动态规划class Solution {public: int rob(vector<int>& nums) { if(nums.size()==0)return 0; if(nums.size()==1)return nums.back(); int sum1=nums[1];//...原创 2018-08-03 18:29:13 · 189 阅读 · 0 评论 -
LeetCode 206. Reverse Linked List 时间复杂度(O( n))
时间复杂度(O( n))class Solution {public: ListNode* reverseList(ListNode* head) { if(head==NULL||head->next==NULL)return head; ListNode* p = head; while(p->next!=NULL)...原创 2018-08-03 18:36:37 · 296 阅读 · 0 评论 -
LeetCode 228. Summary Ranges 时间复杂度(O( n))
时间复杂度(O( n)),我的vc++6.0 对string 支持效果不太好,所以这题用了javaclass Solution { public List<String> summaryRanges(int[] nums) { List<String> list = new ArrayList<>(); if(num...原创 2018-08-03 19:20:10 · 124 阅读 · 0 评论 -
LeetCode 235. Lowest Common Ancestor of a Binary Search Tree 时间复杂度(O( logn))
时间复杂度(O( logn))class Solution {public: TreeNode* lowestCommonAncestor(TreeNode* root, TreeNode* p, TreeNode* q) { if(root==NULL||root->val==p->val||root->val==q->val) retur...原创 2018-08-12 13:26:03 · 139 阅读 · 0 评论 -
LeetCode 236. Lowest Common Ancestor of a Binary Tree 时间复杂度(O( logn))
时间复杂度(O( logn))class Solution {public: TreeNode* lowestCommonAncestor(TreeNode* root, TreeNode* p, TreeNode* q) { if(root==NULL||root->val==p->val||root->val==q->val) retu...原创 2018-08-12 13:25:49 · 169 阅读 · 0 评论 -
LeetCode 801. Minimum Swaps To Make Sequences Increasing 时间复杂度(O(n))
时间复杂度(O(n)),思想:动态规划class Solution {public: int minSwap(vector<int>& A, vector<int>& B) { int step1 = -1 ; int step2 = -1 ; int moveTime=0; f...原创 2018-07-27 10:36:07 · 211 阅读 · 0 评论 -
LeetCode 35. Search Insert Position 时间复杂度(O(log(n)))
时间复杂度(O(log(n))),思想,二分查找class Solution {public: int searchInsert(vector<int>& nums, int target) { int start = 0; int end = nums.size()-1; while(start<=end)...原创 2018-08-09 08:58:44 · 109 阅读 · 0 评论