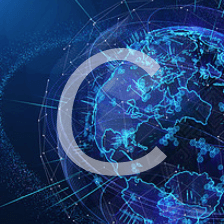
LeetCode
不学无术的小镇做题家
这个作者很懒,什么都没留下…
展开
-
1. 两数之和(简单)- LeetCode
题目描述解法使用哈希表,一次遍历即可。时间复杂度O(n)O(n)O(n),空间复杂度O(n)O(n)O(n)。class Solution: def twoSum(self, nums: List[int], target: int) -> List[int]: d = {} for i,num in enumerate(nums,0): if target-num in d.keys(): ret原创 2021-01-21 14:00:15 · 143 阅读 · 0 评论 -
7. 整数反转(简单)- LeetCode
题目描述自己解法很无脑的解法,先转成字符串再进行判断,Python代码:class Solution: def reverse(self, x: int) -> int: x = str(x) if not x[0].isnumeric(): #负数 x = x[0] + x[1:][::-1] pos = 0 for i in range(1,len(x)):原创 2020-09-12 14:40:47 · 157 阅读 · 0 评论 -
1552. 两球之间的磁力(中等)- LeetCode
题目描述解法自己没想出来,参考了大佬的思路解法,下面试着用自己的话讲一遍。这道题是属于求“最大最小”的题型,一般使用二分法,在本题中要求“最大的最小磁力”,我们先考虑最小磁力,最小磁力肯定出现在相邻两个数之间,比如数组:[1,2,3,4,6]的最小磁力就是1,要求取最小磁力,只需要遍历一遍即可。接下来我们来考虑最大磁力,在有序数组中要放置m个小球,也就是会产生m-1个距离,最大值为max,最小值为min,如果数组是连续的(在题意中就是假设所有位置都有篮子,但实际上可能不满足),那么小球均匀摆布原创 2020-09-02 09:12:29 · 323 阅读 · 0 评论 -
977. 有序数组的平方(简单)- LeetCode
题目描述解法双指针解法。设置一个变量index值为-1,先从左向右遍历数组,找到第一个大于等于零的元素,记录它的下标index。这一过程有三种可能结果:index = -1,表示没找到,说明数组全是负数index = 0,表示第一个数就是非负数,说明数组全是非负数0 < index < 数组长度,说明数组里既有负数,也有非负数第一、二种情况比较好办,直接按位赋值即可。第三种情况需要分别设置遍历负数的指针neg,遍历非负数的指针pos。neg向数组前端遍历,pos向数组末尾遍历,原创 2020-08-28 20:14:28 · 234 阅读 · 0 评论 -
1550. 存在连续三个奇数的数组(简单)- LeetCode
题目描述解法设置一个变量temp,当累计到3时跳出循环。时间复杂度O(n)O(n)O(n),空间复杂度O(1)O(1)O(1)。Python代码:class Solution: def threeConsecutiveOdds(self, arr: List[int]) -> bool: temp = 0 isFound = False for i in range(len(arr)): if arr[i] %原创 2020-08-26 14:02:15 · 305 阅读 · 0 评论 -
1333. 餐厅过滤器(中等)- LeetCode
题目描述解法Python代码参考了题解区大佬的解法,主要用了以下两个Python内置函数,比较方便:filter()函数sorted()函数Python代码:class Solution: def filterRestaurants(self, restaurants: List[List[int]], veganFriendly: int, maxPrice: int, maxDistance: int) -> List[int]: def isVeganF原创 2020-08-25 20:25:56 · 412 阅读 · 0 评论 -
189. 旋转数组(简单)- LeetCode
题目描述自己解法解法一 - 暴力求解直接原地移动数组,时间复杂度O(n2)O(n^2)O(n2),空间复杂度O(1)O(1)O(1):class Solution: def rotate(self, nums: List[int], k: int) -> None: """ Do not return anything, modify nums in-place instead. """ length = len(num原创 2020-08-23 17:24:47 · 217 阅读 · 0 评论 -
1534. 统计好三元组(中等)- LeetCode
题目描述解法题目的要求是求三元组个数,按照暴力解法的话算法复杂度会达到O(n3)O(n^3)O(n3),一个比较简便的做法是先求二元组,比如:∣arr[j]−arr[k]∣≤b|arr[j]-arr[k]| ≤ b∣arr[j]−arr[k]∣≤b对于每一个二元组,满足三元组要求的第三个数满足:∣arr[i]−arr[j]∣≤a |arr[i]−arr[j]|≤a ∣arr[i]−arr[j]∣≤a∣arr[i]−arr[k]∣≤c |arr[i]−arr[k]|≤c∣arr[i]−arr[原创 2020-08-19 23:23:11 · 232 阅读 · 0 评论 -
31. 下一个排列(中等)
题目描述解法自己有想到解题的思路,就是尽量使低位的大数与最接近它的高位的小数交换,再使后面的序列升序排列即可,但是代码实现的时候出问题,看了看大佬的题解,大佬的题解思路就是清晰,自己写了个Python版本的:时间复杂度O(n)O(n)O(n),空间复杂度O(1)O(1)O(1)class Solution: def nextPermutation(self, nums: List[int]) -> None: """ Do not return any原创 2020-08-19 15:10:48 · 159 阅读 · 0 评论 -
面试题 17.05. 字母与数字(中等)- LeetCode
题目描述自己解法利用前缀和,时间复杂度O(n)O(n)O(n),空间复杂度O(n)O(n)O(n):class Solution: def findLongestSubarray(self, array: List[str]) -> List[str]: ans = [] locDict = {0:-1} temp = 0 for i,str in enumerate(array): if '0'原创 2020-08-08 19:20:47 · 172 阅读 · 0 评论 -
面试题 16.20. T9键盘(中等)- LeetCode
题目描述自己解法class Solution: def getValidT9Words(self, num: str, words: List[str]) -> List[str]: keyboard = {2:['a','b','c'],3:['d','e','f'],4:['g','h','i'],5:['j','k','l'],6:['m','n','o'],7:['p','q','r','s'], \ 8:['t','u','v'],9:['w',原创 2020-08-05 23:22:59 · 348 阅读 · 0 评论 -
229. 求众数 II(中等)- LeetCode
题目描述自己解法时间复杂度O(n)O(n)O(n),空间复杂度O(1)O(1)O(1)。class Solution: def majorityElement(self, nums: List[int]) -> List[int]: ans = [] if not nums: return ans cand1,count1 = nums[0],0 cand2,count2 = nums[0],0原创 2020-08-03 15:19:25 · 138 阅读 · 0 评论 -
560. 和为K的子数组(中等)- LeetCode
题目描述自己解法解法一:暴力求解,时间复杂度O(n2)O(n^2)O(n2),空间复杂度O(1)O(1)O(1):class Solution: def subarraySum(self, nums: List[int], k: int) -> int: ans = 0 L = len(nums) for i,val in enumerate(nums): remain = k - val原创 2020-08-01 23:35:34 · 145 阅读 · 0 评论 -
1491. 去掉最低工资和最高工资后的工资平均值(简单)- LeetCode
题目描述自己解法直接按题目思路模拟,没有使用API。时间复杂度O(n)O(n)O(n),空间复杂度O(1)O(1)O(1)。class Solution: def average(self, salary: List[int]) -> float: minIndex,maxIndex = 0,0 L = len(salary) for i in range(1,L): if salary[i] < salar原创 2020-07-24 12:26:34 · 267 阅读 · 0 评论 -
面试题 05.08. 绘制直线(中等)- LeetCode
题目描述自己解法class Solution: def drawLine(self, length: int, w: int, x1: int, x2: int, y: int) -> List[int]: ans = [0 for i in range(length)] arr = [0 for i in range(32 * length)] ## 绘制直线 if x1 > x2: x1,x原创 2020-07-22 17:11:42 · 196 阅读 · 0 评论 -
670. 最大交换(中等)- LeetCode
题目描述自己解法先将输入数字num转换为从高位到低位排列的数组arr,再对arr从大到小排序得到sort_arr,从前往后遍历,找出arr与sort_arr不同的第一个元素的下标start,该下标的元素就是将要与低位的大数进行替换的元素,在从start开始遍历求取最大数,将其与start下标的元素交换。最后再将原数组还原为数字输出即可。时间复杂度O(nlogn)O(nlogn)O(nlogn),空间复杂度O(n)O(n)O(n)。class Solution: def maximumSwap原创 2020-07-20 16:27:07 · 156 阅读 · 0 评论 -
695. 岛屿的最大面积(中等)- LeetCode
题目描述自己解法广度优先搜索(BFS),遍历过的点都置0,时间复杂度O(m∗n)O(m*n)O(m∗n),空间复杂度O(min[m,n])O(min[m,n])O(min[m,n])class Solution: def maxAreaOfIsland(self, grid: List[List[int]]) -> int: maxArea = 0 H,W = len(grid),len(grid[0]) queue = collecti原创 2020-07-18 12:53:32 · 250 阅读 · 0 评论 -
122. 买卖股票的最佳时机 II(中等)- LeetCode
题目描述自己解法采取的方法是计算折线图的每个上升区段,将峰值与低谷值相减得到每个上升区段的利润,最后进行累加,类似于官方题解中的第二种解法。时间复杂度O(n)O(n)O(n),空间复杂度O(1)O(1)O(1)。class Solution: def maxProfit(self, prices: List[int]) -> int: ans = 0 start,end = 0,1 length = len(prices)原创 2020-07-18 00:07:58 · 144 阅读 · 0 评论 -
200. 岛屿数量(中等)- LeetCode
题目描述自己解法广度优先遍历(BFS),使用列表queue模拟队列,isSelected矩阵存储该点是否被遍历过。时间复杂度O(m∗n)O(m*n)O(m∗n),空间复杂度O(m∗n)O(m*n)O(m∗n)class Solution: def numIslands(self, grid: List[List[str]]) -> int: isSelected = [[False for line in row]for row in grid] cou原创 2020-07-16 15:34:51 · 149 阅读 · 0 评论 -
1144. 递减元素使数组呈锯齿状(中等)- LeetCode
题目描述自己解法动态规划的思想,分别计算按奇、偶数索引形成锯齿需要的次数,在进行比较。时间复杂度O(n)O(n)O(n),空间复杂度O(1)O(1)O(1)。class Solution: def movesToMakeZigzag(self, nums: List[int]) -> int: count_odd,count_even = 0,0 temp_odd,temp_even = 0,0 for i in range(1,len(原创 2020-07-15 23:58:00 · 870 阅读 · 0 评论 -
121. 买卖股票的最佳时机(简单)- LeetCode
题目描述自己解法及改进维持一个数组,用动态规划的思想,记录在某一天卖出时的最大利润,最后遍历求取最大值,即买卖股票的最佳策略。缺点是空间复杂度过高。class Solution: def maxProfit(self, prices: List[int]) -> int: if not prices: return 0 profit = [0 for val in prices] minPrice = prices原创 2020-07-14 14:47:29 · 161 阅读 · 0 评论 -
566. 重塑矩阵(简单)- LeetCode
题目描述自己解法先把原矩阵转换为一个列表,再依次读入:时间复杂度O(m∗n)O(m*n)O(m∗n),空间复杂度O(m∗n)O(m*n)O(m∗n)class Solution: def matrixReshape(self, nums: List[List[int]], r: int, c: int) -> List[List[int]]: if r * c != len(nums) * len(nums[0]): return nums原创 2020-07-13 22:52:07 · 191 阅读 · 0 评论 -
面试题 16.04. 井字游戏(中等)- LeetCode
题目描述自己解法按照行、列、对角线三种情况分别讨论,时间复杂度O(n2)O(n^2)O(n2),空间复杂度O(1)O(1)O(1)class Solution: def tictactoe(self, board: List[str]) -> str: H = len(board[0]) ## 行满足 for i in range(H): last_char = board[i][0] ex原创 2020-07-12 14:54:28 · 256 阅读 · 0 评论 -
766. 托普利茨矩阵(简单)- LeetCode
题目描述自己解法对于一个M×NM×NM×N矩阵,遍历前M−1M-1M−1行的前N−1N-1N−1个元素,假设下标为(i,j)(i,j)(i,j),若该元素与下标为(i+1,j+1)(i+1,j+1)(i+1,j+1)的元素相等,则满足题目条件,若不相等,则不满足。时间复杂度O(M×N)O(M×N)O(M×N),空间复杂度O(1)O(1)O(1)。class Solution: def isToeplitzMatrix(self, matrix: List[List[int]]) ->原创 2020-07-12 00:19:51 · 193 阅读 · 0 评论 -
1385. 两个数组间的距离值(简单)- LeetCode
题目描述自己解法没想到除了暴力求解以外的解法,时间复杂度O(mn)O(mn)O(mn),空间复杂度O(1)O(1)O(1)。class Solution: def findTheDistanceValue(self, arr1: List[int], arr2: List[int], d: int) -> int: ans = 0 for val1 in arr1: exist_d = True for v原创 2020-07-10 17:14:32 · 229 阅读 · 0 评论 -
1414. 和为 K 的最少斐波那契数字数目(中等)- LeetCode
题目描述自己解法直觉上采用贪心策略,从大到小选择斐波那契数字,这样可以使得个数总和最少。时间复杂度O(45)O(45)O(45),空间复杂度O(45)O(45)O(45),因为在k≤109k≤10^9k≤109的范围内,本解法最多有45个斐波那契数。class Solution: def findMinFibonacciNumbers(self, k: int) -> int: Fibonacci = [1,1] ans = 0 whi原创 2020-07-06 11:57:57 · 284 阅读 · 0 评论 -
1343. 大小为 K 且平均值大于等于阈值的子数组数目(中等)- LeetCode
题目描述自己解法从数组的第k个元素开始遍历,每次减去开头元素,加上末尾元素,进行判断即可。时间复杂度O(n)O(n)O(n),空间复杂度O(1)O(1)O(1)。class Solution: def numOfSubarrays(self, arr: List[int], k: int, threshold: int) -> int: sub = sum(arr[:k]) ans = 1 if sub >= threshold * k else原创 2020-07-05 17:54:12 · 220 阅读 · 0 评论 -
169. 多数元素(简单)- LeetCode
题目描述自己解法第一种:哈希表统计class Solution: def majorityElement(self, nums: List[int]) -> int: ValCount = dict() ans = 0 for val in nums: if val in ValCount: ValCount[val] += 1 else:原创 2020-07-04 11:44:12 · 174 阅读 · 0 评论 -
867. 转置矩阵(简单)- LeetCode
题目描述自己解法按照转置矩阵的定义进行数据填充:class Solution: def transpose(self, A: List[List[int]]) -> List[List[int]]: H,W = len(A),len(A[0]) ans = [] for i in range(W): ans.append([]) for i in range(W): for原创 2020-07-03 11:34:47 · 189 阅读 · 0 评论 -
1304. 和为零的N个唯一整数(简单)- LeetCode
题目描述自己解法自己的想法是以0为中心正负对称构造数组,并且不想判断n是否为奇数或偶数,所以初始化全零数组,在range(0,n-1,2)范围内改变数组的值即可。时间复杂度O(n)O(n)O(n),空间复杂度O(1)O(1)O(1)(不包括返回的数组)。class Solution: def sumZero(self, n: int) -> List[int]: ans = [0] * n for i in range(0,n-1,2):原创 2020-07-02 23:12:07 · 188 阅读 · 0 评论 -
219. 存在重复元素 II(简单)- LeetCode
题目描述自己解法设置一个字典,遍历数组,记录元素下标,若碰到重复元素,与字典中存储的下标比较,若满足条件返回:class Solution: def containsNearbyDuplicate(self, nums: List[int], k: int) -> bool: num_pos = dict() for i,val in enumerate(nums): if val in num_pos:原创 2020-07-01 16:10:59 · 140 阅读 · 0 评论 -
914. 卡牌分组(简单)- LeetCode
题目描述自己解法遍历数组计数,求出最小牌数,根据最小牌数求出它的所有因数,再遍历检查即可class Solution: def hasGroupsSizeX(self, deck: List[int]) -> bool: card_count = dict() factor = [] for card in deck: if card in card_count: card_coun原创 2020-06-30 10:45:35 · 213 阅读 · 0 评论 -
999. 可以被一步捕获的棋子数(简单)- LeetCode
题目描述自己解法先找到车的位置,再遍历四个方向即可。class Solution: def numRookCaptures(self, board: List[List[str]]) -> int: ans = 0 x,y = 0,0 ## 找到车的位置 for i in range(8): for j in range(8): if board[i][j] ==原创 2020-06-29 16:18:45 · 177 阅读 · 0 评论 -
1232. 缀点成线(简单)- LeetCode
题目描述自己解答以第一点为参考,计算斜率,考虑斜率不存在的特殊情况即可class Solution: def checkStraightLine(self, coordinates: List[List[int]]) -> bool: if coordinates[1][0] != coordinates[0][0]: k_start =(coordinates[1][1] - coordinates[0][1]) / (coordinates原创 2020-06-28 16:54:49 · 174 阅读 · 0 评论 -
697. 数组的度(简单)- LeetCode
题目描述解法首先要确定数组中最大频数的元素,如果有多个最大频数的元素,要比较其最短连续数组的长度,取其最小值def findShortSubArray(nums): left,right,count = {},{},{} for i,val in enumerate(nums): if val not in left: left[val] = i right[val] = i count[val] = count原创 2020-06-28 14:41:19 · 127 阅读 · 0 评论 -
面试题 17.10. 主要元素(简单)- LeetCode
题目描述自己解法解法一:先给列表排序,在排序完的数组中,下标为数组长度/2的元素有可能是主元素,再统计数组中该元素的个数,若超过一半则证明是主元素。class Solution: def majorityElement(self, nums: List[int]) -> int: nums = sorted(nums) element = nums[len(nums) // 2] count = 0 for val in原创 2020-06-27 01:07:07 · 271 阅读 · 0 评论 -
661. 图片平滑器(简单)- LeetCode
题目描述自己解法挨个遍历矩阵下标,判定矩阵范围,如果范围合理,则统计个数:class Solution: def imageSmoother(self, M: List[List[int]]) -> List[List[int]]: H,W = len(M),len(M[0]) A = [[0] * W for _ in M] for i in range(H): for j in range(W):原创 2020-06-25 22:17:35 · 425 阅读 · 0 评论 -
643. 子数组最大平均数 I(简单)- LeetCode
题目描述自己解法暴力求解的话空间复杂度为O(n2)O(n^2)O(n2),故采用滑动窗口法,只遍历一次数组,依次减去开头和加上末尾元素进行比较。class Solution: def findMaxAverage(self, nums: List[int], k: int) -> float: sum_val = sum(nums[:k]) res = sum_val for i in range(k,len(nums)):原创 2020-06-20 00:45:01 · 131 阅读 · 0 评论 -
1346. 检查整数及其两倍数是否存在(简单)- LeetCode
题目描述官方解答暴力求解排序+双指针class Solution: def checkIfExist(self, arr: List[int]) -> bool: arr.sort() q = 0 for p in range(len(arr)): while q < len(arr) and arr[p] * 2 > arr[q]: q += 1原创 2020-06-18 23:35:34 · 142 阅读 · 0 评论 -
1184. 公交站间的距离(简单)- LeetCode
题目描述自己解答由于题目中是无向图,所以a->b和b->a的距离一致,为简化算法,当start>destination时,将二者的值调换。遍历累加数组下标范围[start,destination)的值,另外一条路径的值就是数组总和减去该值,比较二者取其较小者即可。class Solution: def distanceBetweenBusStops(self, distance: List[int], start: int, destination: int) -> int原创 2020-06-17 15:04:24 · 255 阅读 · 0 评论