第P2周:CIFAR10彩色图片识别
要求:
- 学习如何编写一个完整的深度学习程序
- 手动推导卷积层与池化层的计算过程
一、数据预处理
1. 导入库
import torch
import torch.nn as nn
import matplotlib.pyplot as plt
import torchvision
2. 导入数据
train_ds = torchvision.datasets.CIFAR10('data',
train=True,
transform=torchvision.transforms.ToTensor(),
download=True)
test_ds = torchvision.datasets.CIFAR10('data',
train=False,
transform=torchvision.transforms.ToTensor(),
download=True)
输出
Files already downloaded and verified
Files already downloaded and verified
使用加载器加载数据集
batch_size = 32
train_dl = torch.utils.data.DataLoader(train_ds,
batch_size=batch_size,
shuffle=True)
test_dl = torch.utils.data.DataLoader(test_ds,
batch_size=batch_size)
3. 可视化展示数据
imgs, labels = next(iter(train_dl))
plt.figure(figsize=(20, 5))
for i, imgs in enumerate(imgs[:20]):
npimg = imgs.numpy().transpose((1, 2, 0))
plt.subplot(2, 10, i + 1)
plt.imshow(npimg, cmap=plt.cm.binary)
plt.axis('off')
plt.show()
运行结果
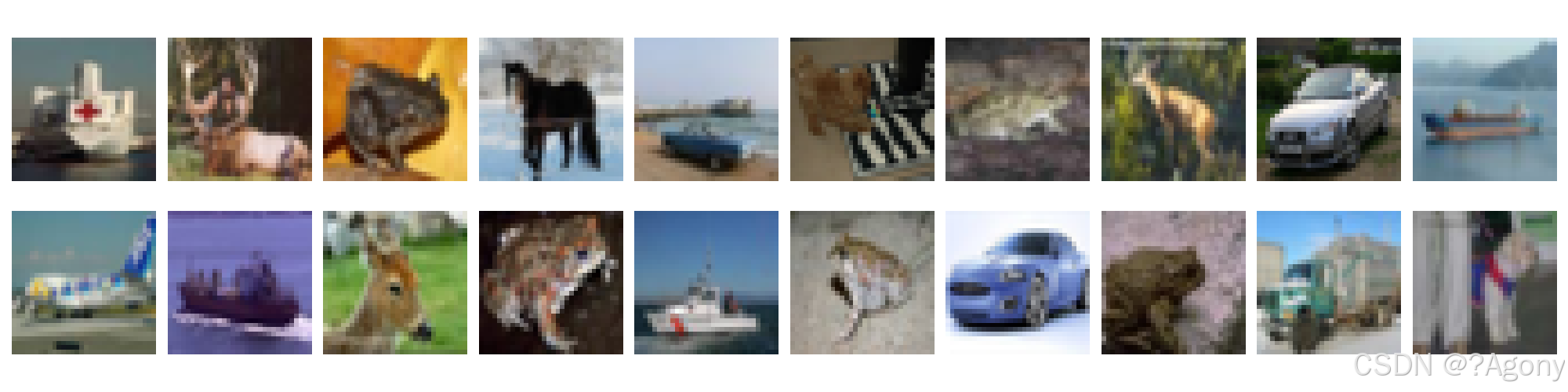
二、搭建CNN神经网络
- torch.nn.Conv2d()函数用法:torch.nn.Conv2d(in_channels, out_channels, kernel_size, stride=1, padding=0, dilation=1, groups=1, bias=True, padding_mode=‘zeros’, device=None, dtype=None)
1. 够建模型
num_classes = 10
class Model(nn.Module):
def __init__(self):
super().__init__()
self.conv1 = nn.Conv2d(3, 64, kernel_size=3)
self.pool1 = nn.MaxPool2d(kernel_size=2)
self.