在读取前需要知道写入 write 这个API的返回值,如果写入失败会返回 -1;
接下来是我们的读取操作了,把写入的内容读取出来
先看一下头文件和API的原型

#include <stdio.h>
#include <unistd.h>
#include <string.h>
#include <stdlib.h>
int main()
{
int fd;
char *buf = "hello dzz hei shuai";
fd = open("./file1",O_RDWR);
if(fd == -1){
printf("open file1 failed\n");
fd = open("./file1", O_RDWR | O_CREAT, 0600);
if(fd > 0){
printf("create file1 sucess\n");
}
}
printf("open susccess : fd =%d\n",fd);
// ssize_t write(int fd, const void *buf, size_t count);
int n_write = write(fd, buf, strlen(buf));
if(n_write != -1){
printf("write %d byte to file\n",n_write);
}
// ssize_t read(int fd, void *buf, size_t count);
// 需要开辟一个空间用来存放你读取来的内容
char *readBuf = (char *)malloc(sizeof(char) * n_write + 1);
int n_read = read(fd, readBuf, n_write);
printf("read=%d,content=%s\n",n_read,readBuf);
close(fd);
return 0;
}

哈,现在看,结果并没有读取到写入的内容。
这是光标的问题,因为读的时候,光标已经在内容的最后了,所有读的一直是内容后面的内容
解决方法一:读的时候重新把关闭移到内容的最前面,重新打开最可以实现
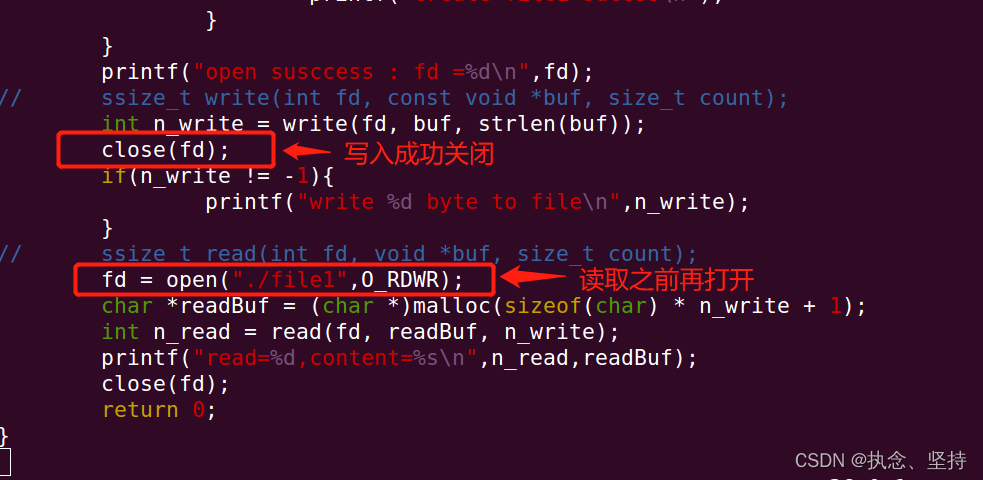
结果

这时候就能成功读取到file1文件里面的内容了
解决方法二:利用文件“”光标“”位置的API
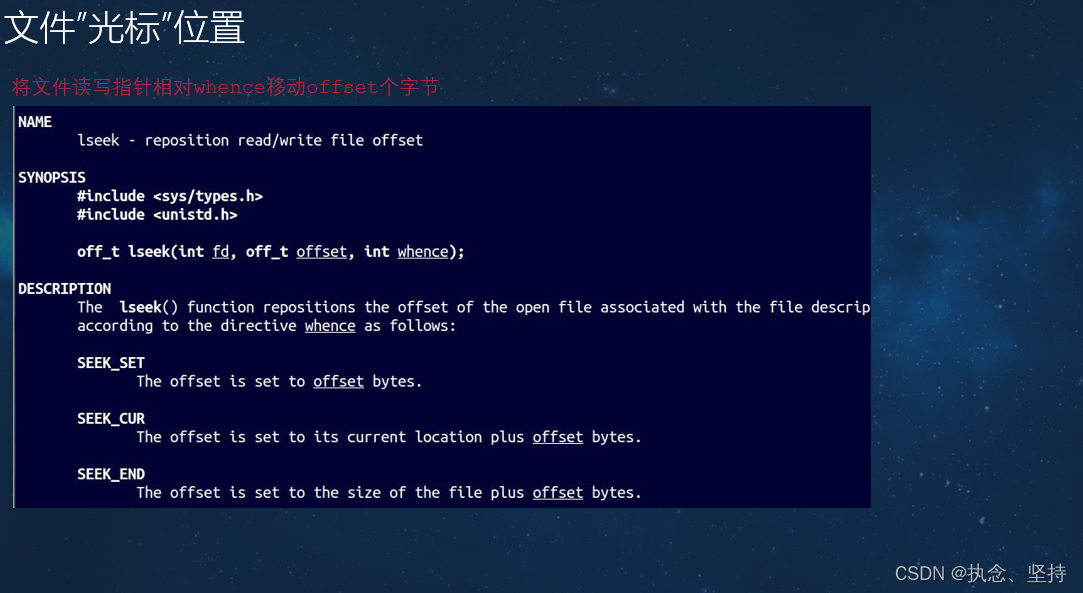
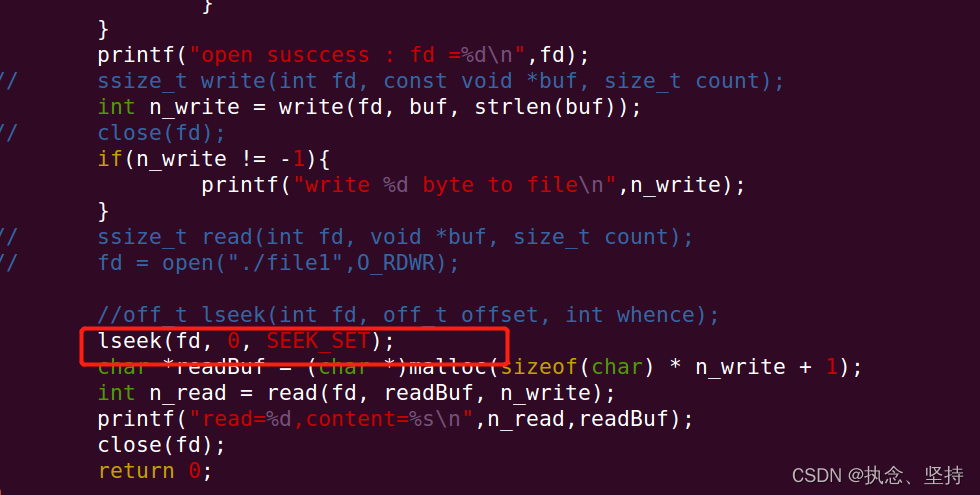
也可以利用其API来计算读出来的内容的大小
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <stdio.h>
#include <unistd.h>
#include <string.h>
#include <stdlib.h>
int main()
{
int fd;
char *buf = "hello dzz hei shuai";
fd = open("./file1",O_RDWR);
int filesize = lseek(fd, 0, SEEK_END);
printf("file is size is:%d\n",filesize);
close(fd);
return 0;
}
