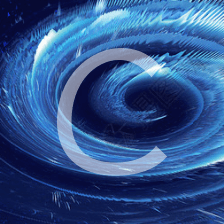
LeetCode
文章平均质量分 85
小鱼人会飞
本科就读于吉林大学现阶段在攻读北京大学智能科学技术硕士学位研究方向计算机视觉计算机图形学等领域
展开
-
Minimum(Maximum) Depth of Binary Tree
题目:Given a binary tree, find its minimum depth.The minimum depth is the number of nodes along the shortest path from the root node down to the nearest leaf node.此题与计算二叉树的高度或深度不同,他是到最高叶子节点的路径长度,是原创 2013-06-27 22:34:02 · 1336 阅读 · 0 评论 -
Flatten Binary Tree to Linked List
题目Given a binary tree, flatten it to a linked list in-place.For example,Given 1 / \ 2 5 / \ \ 3 4 6The flattened tree should look like:原创 2013-07-14 10:46:01 · 779 阅读 · 0 评论 -
sqrt(x)
题目Implement int sqrt(int x).Compute and return the square root of x.思路可以用牛顿迭代法求平方根,Xk+1 = 1/2 * ( Xk + n/Xk ) ;要注意,函数的输入和返回都是 int 型。代码class Solution {public: int sqrt(in原创 2013-07-15 10:52:49 · 774 阅读 · 0 评论 -
Set Matrix Zeroes
题目Given a m x n matrix, if an element is 0, set its entire row and column to 0. Do it in place.Follow up:Did you use extra space?A straight forward solution using O(mn) space is原创 2013-07-15 11:11:54 · 834 阅读 · 0 评论 -
Plus One
题目Given a number represented as an array of digits, plus one to the number.实现数组 vector 的地位存储number的高位。class Solution {public: vector plusOne(vector &digits) { // Start typing yo原创 2013-07-14 21:00:51 · 906 阅读 · 0 评论 -
Binary Tree Level Order Traversal
题目:Given a binary tree, return the level order traversal of its nodes' values. (ie, from left to right, level by level).For example:Given binary tree {3,9,20,#,#,15,7}, 3 / \ 9 20原创 2013-06-28 14:28:59 · 1351 阅读 · 0 评论 -
Best Time to Buy and Sell Stock III
题目:Say you have an array for which the ith element is the price of a given stock on dayi.Design an algorithm to find the maximum profit. You may complete at most two transactions.Note:You may原创 2013-06-27 18:31:35 · 948 阅读 · 0 评论 -
Best Time to Buy and Sell Stock
题目:数组中某数字减去其左边的某数字得到一个数对之差,求所有数对之差的最大值。例如:数组{2, 4, 1, 16, 7, 5, 11, 9}中,数对之差的最大值是15(16 - 1)分析:看到这个题目,很多人的第一反应是找到这个数组的最大值和最小值,然后觉得最大值减去最小值就是最终的结果。但由于我们无法保证最大值一定位于数组的右边。让每一个数字逐个减原创 2013-06-27 13:08:58 · 1039 阅读 · 0 评论 -
Best Time to Buy and Sell Stock II
题目Design an algorithm to find the maximum profit. You may complete as many transactions as you like (ie, buy one and sell one share of the stock multiple times). However, you may not engage in multi原创 2013-06-27 16:22:17 · 1134 阅读 · 0 评论 -
valid palindrome
题目:Given a string, determine if it is a palindrome, considering only alphanumeric characters and ignoring cases.For example,"A man, a plan, a canal: Panama" is a palindrome."race a car" is not原创 2013-06-29 15:40:39 · 1394 阅读 · 0 评论 -
Triangle
题目:Given a triangle, find the minimum path sum from top to bottom. Each step you may move to adjacent numbers on the row below.For example, given the following triangle[ [2], [3,4原创 2013-06-29 15:06:05 · 1074 阅读 · 0 评论 -
Add Two Numbers
题目:You are given two linked lists representing two non-negative numbers. The digits are stored in reverse order and each of their nodes contain a single digit. Add the two numbers and return it as a原创 2013-06-30 19:06:29 · 812 阅读 · 0 评论 -
Median of Two Sorted Arrays
题目:给定两个已经排序好的数组(可能为空),找到两者所有元素中第k大的元素。另外一种更加具体的形式是,找到所有元素的中位数。本篇文章我们只讨论更加一般性的问题:如何找到两个数组中第k大的元素?不过,测试是用的两个数组的中位数的题目,该题可以解决所有求有序数组A和B有序合并之后第k小的数!该题的重要结论:如果A[k/2-1] 思路:方案1:假设两个数组总共有n个元素,转载 2013-06-30 20:45:59 · 858 阅读 · 0 评论 -
Longest Substring Without Repeating Characters
题目 实现 class Solution {public: int lengthOfLongestSubstring(string s) { // Start typing your C/C++ solution below // DO NOT write int main() function int len =原创 2013-07-15 15:32:53 · 849 阅读 · 0 评论 -
Minimum Path Sum
题目Given a m x n grid filled with non-negative numbers, find a path from top left to bottom right which minimizes the sum of all numbers along its path.Note: You can only move either do原创 2013-07-15 14:58:31 · 728 阅读 · 0 评论 -
Longest Palindromic Substring
题目Given a string S, find the longest palindromic substring in S. You may assume that the maximum length of S is 1000, and there exists one unique longest palindromic substring.思路一:最原始的 Bru原创 2013-07-15 21:16:27 · 1156 阅读 · 0 评论 -
Merge Sorted Array
题目Given two sorted integer arrays A and B, merge B into A as one sorted array.Note:You may assume that A has enough space to hold additional elements from B. The number of elements initialized i原创 2013-07-16 21:20:54 · 781 阅读 · 0 评论 -
Path Sum
题目:Given a binary tree and a sum, determine if the tree has a root-to-leaf path such that adding up all the values along the path equals the given sum.For example:Given the below binary tree and原创 2013-07-01 12:20:03 · 1022 阅读 · 0 评论 -
Sum Root to Leaf Numbers
题目:Given a binary tree containing digits from 0-9 only, each root-to-leaf path could represent a number.An example is the root-to-leaf path 1->2->3 which represents the number123.Find the total原创 2013-07-01 15:36:09 · 886 阅读 · 0 评论 -
Search in Rotated Sorted Array II
题目Follow up for "Search in Rotated Sorted Array":What if duplicates are allowed?Would this affect the run-time complexity? How and why?Write a function to determine if a given target is in t原创 2013-07-16 17:06:57 · 831 阅读 · 0 评论 -
Two Sum
题目:Given an array of integers, find two numbers such that they add up to a specific target number.The function twoSum should return indices of the two numbers such that they add up to the target,原创 2013-06-30 16:55:16 · 814 阅读 · 0 评论 -
Climbing Stairs
题目You are climbing a stair case. It takes n steps to reach to the top.Each time you can either climb 1 or 2 steps. In how many distinct ways can you climb to the top?思路一递归,大数据超时class Solut原创 2013-07-18 13:26:20 · 903 阅读 · 0 评论 -
Partition List
题目Given a linked list and a value x, partition it such that all nodes less than x come before nodes greater than or equal tox.You should preserve the original relative order of the nodes in ea原创 2013-07-17 19:53:02 · 719 阅读 · 0 评论 -
Reverse Linked List II
题目Reverse a linked list from position m to n. Do it in-place and in one-pass.For example:Given 1->2->3->4->5->NULL, m = 2 and n = 4,return 1->4->3->2->5->NULL.Note:Given m, n satisfy原创 2013-07-17 21:13:39 · 761 阅读 · 0 评论 -
Remove Element
题目Given an array and a value, remove all instances of that value in place and return the new length.The order of elements can be changed. It doesn't matter what you leave beyond the new length.思原创 2013-07-18 13:10:48 · 745 阅读 · 0 评论 -
Maximum Subarray
题目Find the contiguous subarray within an array (containing at least one number) which has the largest sum.For example, given the array [−2,1,−3,4,−1,2,1,−5,4],the contiguous subarray [4,−1,2,1]原创 2013-07-18 17:40:15 · 807 阅读 · 0 评论 -
Pow(x, n)
题目Implement pow(x, n).思路:二分法class Solution {public: double pow(double x, int n) { // Start typing your C/C++ solution below // DO NOT write int main() function原创 2013-07-19 12:59:26 · 914 阅读 · 0 评论 -
Jump Game II
题目Given an array of non-negative integers, you are initially positioned at the first index of the array.Each element in the array represents your maximum jump length at that position.Your goal i原创 2013-07-20 15:07:29 · 734 阅读 · 0 评论 -
4Sum
题目Given an array S of n integers, are there elements a, b, c, and d in S such that a + b + c + d = target? Find all unique quadruplets in the array which gives the sum of target.Note:原创 2013-07-21 17:04:19 · 993 阅读 · 0 评论 -
3Sum
题目Given an array S of n integers, are there elements a, b, c in S such that a + b + c = 0? Find all unique triplets in the array which gives the sum of zero.Note:Elements in a triple原创 2013-07-21 13:55:12 · 1199 阅读 · 0 评论 -
Container With Most Water
题目Given n non-negative integers a1, a2, ..., an, where each represents a point at coordinate (i, ai). n vertical lines are drawn such that the two endpoints of line i is at (i, ai) and (原创 2013-07-21 19:55:03 · 1198 阅读 · 2 评论 -
Simplify Path
题目Given an absolute path for a file (Unix-style), simplify it.For example,path = "/home/", => "/home"path = "/a/./b/../../c/", => "/c"Corner Cases:Did you consider the case原创 2013-07-21 22:10:46 · 799 阅读 · 0 评论 -
N-Queens
题目The n-queens puzzle is the problem of placing n queens on an n�n chessboard such that no two queens attack each other.Given an integer n, return all distinct solutions to the n原创 2013-07-22 12:41:09 · 1306 阅读 · 1 评论 -
N-Queens II
题目Follow up for N-Queens problem.Now, instead outputting board configurations, return the total number of distinct solutions.思路一用一个NXN的 flag 矩阵记录可能每一个皇后可能的位置和不能的位置,分别是0和1 。class So原创 2013-07-22 11:01:48 · 1157 阅读 · 0 评论 -
Count and Say
题目The count-and-say sequence is the sequence of integers beginning as follows:1, 11, 21, 1211, 111221, ...1 is read off as "one 1" or 11.11 is read off as "two 1s" or 21.21 i原创 2013-07-22 23:35:40 · 1343 阅读 · 1 评论 -
Generate Parentheses
题目Given n pairs of parentheses, write a function to generate all combinations of well-formed parentheses.For example, given n = 3, a solution set is:"((()))", "(()())", "(())()", "()(())",原创 2013-07-22 17:08:48 · 781 阅读 · 0 评论 -
Interleaving String
题目判断两个字符串 s1 和 s2 是否可以拼接出 s3Given s1, s2, s3, find whether s3 is formed by the interleaving of s1 and s2.For example,Given:s1 = "aabcc",s2 = "dbbca",When s3 = "aadbbcbcac", return原创 2013-07-22 16:31:51 · 835 阅读 · 0 评论 -
Length of Last Word
题目Given a string s consists of upper/lower-case alphabets and empty space characters ' ', return the length of last word in the string.If the last word does not exist, return 0.Note:原创 2013-07-22 18:56:21 · 820 阅读 · 0 评论 -
Spiral Matrix I(II)
题目:螺旋序矩阵Given a matrix of m x n elements (m rows, n columns), return all elements of the matrix in spiral order.For example,Given the following matrix:[ [ 1, 2, 3 ], [ 4, 5, 6 ], [ 7原创 2013-07-21 21:19:37 · 849 阅读 · 0 评论 -
Reverse Nodes in k-Group
题目Given a linked list, reverse the nodes of a linked list k at a time and return its modified list.If the number of nodes is not a multiple of k then left-out nodes in the end should remain as原创 2013-07-29 00:27:38 · 903 阅读 · 0 评论