你应该随遇而安,尽可能地享受生活。
Program.cs代码:
internal class Program
{
private static void Main(string[] args)
{
MethodOne();
MethodTwo();
MethodThree();
MethodFour();
Console.WriteLine();
}
private static void MethodOne()
{
Expression<Func<int, bool>> lambda = num => num < 5;
Console.WriteLine(lambda.Compile()(5));
Console.ReadLine();
}
private static void MethodTwo()
{
ParameterExpression numParam = Expression.Parameter(typeof(int), "num");
ConstantExpression five = Expression.Constant(5, typeof(int));
BinaryExpression numLessThanFive = Expression.LessThan(numParam, five);
var exp = Expression.Lambda<Func<int, bool>>(numLessThanFive, new ParameterExpression[] { numParam });
Console.WriteLine(exp.Compile()(10));
Console.ReadLine();
}
private static void MethodThree()
{
ParameterExpression value = Expression.Parameter(typeof(int), "value");
ParameterExpression result = Expression.Parameter(typeof(int), "result");
LabelTarget label = Expression.Label(typeof(int));
BlockExpression block = Expression.Block(new[] { result },
Expression.Assign(result, Expression.Constant(1)),
Expression.Loop(
Expression.IfThenElse(
Expression.GreaterThan(value, Expression.Constant(1)),
Expression.MultiplyAssign(result, Expression.PostDecrementAssign(value)),
Expression.Break(label, result)
),
label
)
);
int factorial = Expression.Lambda<Func<int, int>>(block, value).Compile()(5);
Console.WriteLine("阶乘的结果:" + factorial);
Console.ReadLine();
}
private static void MethodFour()
{
Expression<Func<int, bool>> exprTree = num => num < 5;
var param = exprTree.Parameters[0];
var operation = (BinaryExpression)exprTree.Body;
var left = (ParameterExpression)operation.Left;
var right = (ConstantExpression)operation.Right;
Console.WriteLine("分解表达式: {0} => {1} {2} {3}", param.Name, left.Name, operation.NodeType, right.Value);
Console.ReadLine();
}
}
运行结果如图:
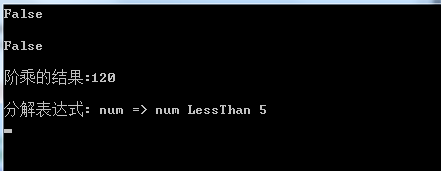