Alex教程每一P的教程原代码加上我自己的理解初步理解写的注释,可供学习Alex教程的人参考
此代码仅为较上一P有所改变的代码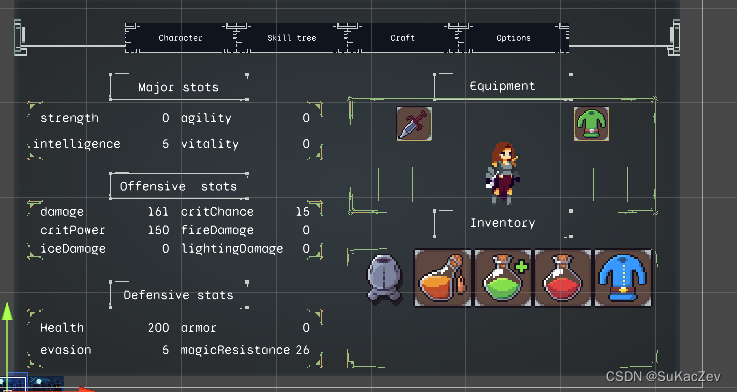
【Unity教程】从0编程制作类银河恶魔城游戏_哔哩哔哩_bilibili
UI_Statslot.cs
using System.Collections;
using System.Collections.Generic;
using TMPro;
using UnityEngine;
public class UI_Statslot : MonoBehaviour
{
[SerializeField] private StatType statType;
[SerializeField] private TextMeshProUGUI statValueText;
[SerializeField] private TextMeshProUGUI statNameText;
private void OnValidate()
{
gameObject.name = "Stat - " + statType.ToString();
if(statNameText != null)
{
statNameText.text = statType.ToString();
}
}
private void Start()
{
UpdateStatValueUI();
}
public void UpdateStatValueUI()
{
PlayerStats playerStats = PlayerManager.instance.player.GetComponent<PlayerStats>();
if(playerStats != null)
{
statValueText.text = playerStats.GetStats(statType).GetValue().ToString();
}
}
}
UI_equipementSlots.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.EventSystems;
public class UI_equipementSlots : UI_itemSlot
{
public EquipmentType slotType;//这怎么拿到的
private void OnValidate()
{
gameObject.name = "Equipment slot -" + slotType.ToString();
}
public override void OnPointerDown(PointerEventData eventData)
{
if (item == null || item.data == null)//修复点击空白处会报错的bug
return;
//点击装备槽后卸下装备
Inventory.instance.AddItem(item.data as ItemData_Equipment);
Inventory.instance.Unequipment(item.data as ItemData_Equipment);
CleanUpSlot();
}
}
UI_itemSlot.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using TMPro;
using UnityEngine.EventSystems;
public class UI_itemSlot : MonoBehaviour ,IPointerDownHandler
{
[SerializeField] private Image itemImage;
[SerializeField] private TextMeshProUGUI itemText;
public InventoryItem item;
public void UpdateSlots(InventoryItem _newItem)
{
item = _newItem;
itemImage.color = Color.white;
if (item != null)
{
itemImage.sprite = item.data.icon;
if (item.stackSize > 1)
{
itemText.text = item.stackSize.ToString();
}
else
{
itemText.text = "";
}
}
}
public void CleanUpSlot()//解决出现UI没有跟着Inventory变化的bug
{
item = null;
itemImage.sprite = null;
itemImage.color = Color.clear;
itemText.text = "";
}
public virtual void OnPointerDown(PointerEventData eventData)
{
if(item == null)//修复点击空白处会报错的bug
{
return;
}
if(Input.GetKey(KeyCode.LeftControl))
{
Inventory.instance.RemoveItem(item.data);
return;
}
if (item.data.itemType == ItemType.Equipment)
Inventory.instance.EquipItem(item.data);
}
}
Buff_Effcet.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
[CreateAssetMenu(fileName = "BUff effect", menuName = "Data/Item effect/Buff effect")]
public class Buff_Effect :ItemEffect
{
private PlayerStats stats;
[SerializeField] private StatType buffType;
[SerializeField] private float buffDuration;
[SerializeField] private int buffAmount;
public override void ExecuteEffect(Transform _respawnPosition)
{
stats = PlayerManager.instance.player.GetComponent<PlayerStats>();
stats.IncreaseStatBy(buffAmount, buffDuration, stats.GetStats(buffType));
}
}
Inventory.cs
using Newtonsoft.Json.Linq;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
using UnityEngine;
public class Inventory : MonoBehaviour
{
public static Inventory instance;
public List<ItemData> startingItem;
public List<InventoryItem> equipment;//inventoryItems类型的列表
public Dictionary<ItemData_Equipment, InventoryItem> equipmentDictionary;//以ItemData为Key寻找InventoryItem的字典
public List<InventoryItem> inventory;//inventoryItems类型的列表
public Dictionary<ItemData, InventoryItem> inventoryDictionary;//以ItemData为Key寻找InventoryItem的字典
public List<InventoryItem> stash;
public Dictionary<ItemData, InventoryItem> stashDictionary;
[Header("Inventory UI")]
[SerializeField] private Transform inventorySlotParent;
[SerializeField] private Transform stashSlotParent;
[SerializeField] private Transform equipmentSlotParent;
[SerializeField]