逻辑分析:
- 栈的基本操作有两个:入栈和出栈。我们依次从 1 到 n 入栈,每次可以选择是否出栈。栈的容量不能超过 m。
- 如果某个数字要出栈,它要么是当前栈顶的数字,要么需要先通过入栈来使其成为栈顶。
代码实现:
#include<stdio.h>
#include<stdbool.h>
#define MAX_N 1008
bool isValidOutput(int m, int n, int output[]) {
int Stack[MAX_N];
int top = -1;
int in = 1;
for (int i = 0; i < n; i++) {
int out = output[i];
while (in <= n && (top == -1 || Stack[top] != out)) {
Stack[++top] = in;
in++;
if (top >= m) {
return false;
}
}
if (Stack[top] == out) {
top--;
}
else {
return false;
}
}
return true;
}
int main() {
int m, n, k;
scanf("%d %d %d", &m, &n, &k);
for (int i = 0; i < k; i++) {
int output[MAX_N];
for (int j = 0; j < n; j++) {
scanf("%d", &output[j]);
}
if (isValidOutput(m, n, output)) {
printf("YES\n");
} else {
printf("NO\n");
}
}
return 0;
}
测试详情:
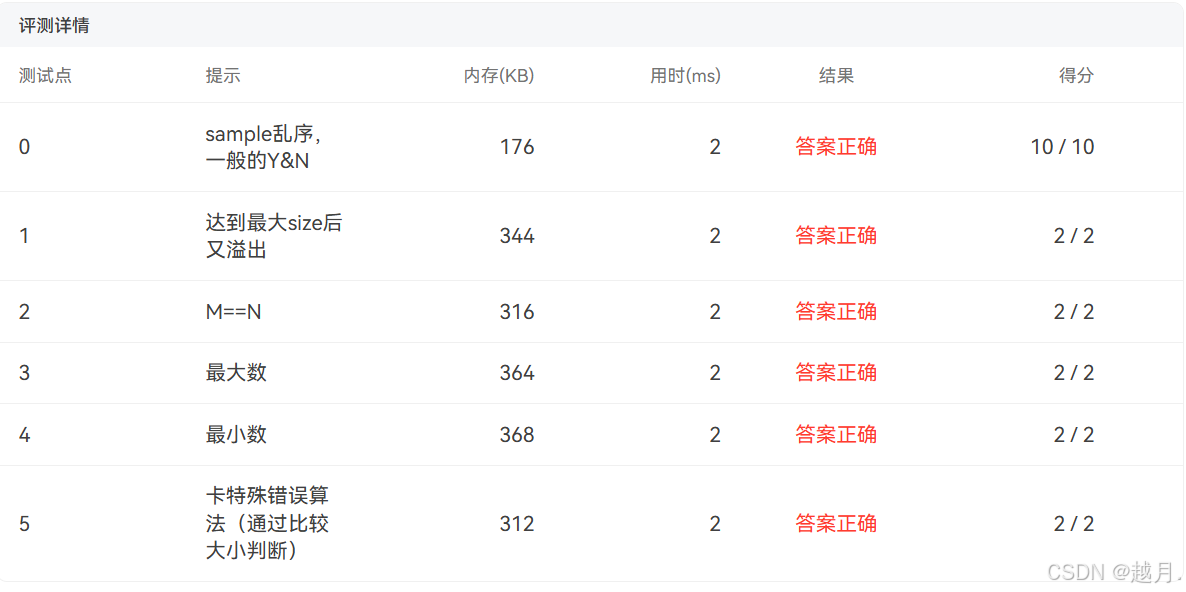