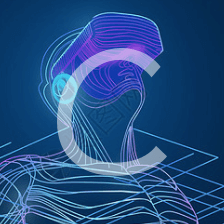
LeetCode
LarryInTokyo
这个作者很懒,什么都没留下…
展开
-
【LeetCode】Maximum Depth of Binary Tree
Given a binary tree, find its maximum depth.The maximum depth is the number of nodes along the longest path from the root node down to the farthest leaf node./** * Definition for binary tree原创 2014-03-12 15:40:05 · 567 阅读 · 0 评论 -
【LeetCode】Binary Tree Inorder Traversal
Given a binary tree, return the inorder traversal of its nodes' values.For example:Given binary tree {1,#,2,3}, 1 \ 2 / 3return [1,3,2].Note: Recursive solutio原创 2014-03-13 21:06:44 · 656 阅读 · 0 评论 -
【LeetCode】Pow(x, n)
Implement pow(x, n).// 二分法,x^n = x^{n/2} * x^{n/2} * x^{n\%2}// 时间复杂度 O(logn),空间复杂度 O(1)class Solution {public: double pow(double x, int n) { if (n < 0) return 1.0 / power(x, -转载 2014-03-14 13:08:57 · 665 阅读 · 0 评论 -
【LeetCode】Sqrt(x)
Implement int sqrt(int x).Compute and return the square root of x.思路:从[1,x/2]中利用二分法查找结果。可取小不可取大(如8只能返回2,不能返回3),故在增加left的时候,要保存当前的中间值。为了避免溢出,使用x/mid > mid,而不用x > mid*mid;class Solution {转载 2014-03-14 15:12:16 · 571 阅读 · 0 评论 -
【LeetCode】Container With Most Water
Given n non-negative integers a1, a2, ..., an, where each represents a point at coordinate (i, ai). n vertical lines are drawn such that the two endpoints of line i is at (i, ai) and (i, 0). Fin转载 2014-03-14 16:02:35 · 597 阅读 · 0 评论 -
【LeetCode】Triangle
Given a triangle, find the minimum path sum from top to bottom. Each step you may move to adjacent numbers on the row below.For example, given the following triangle[ [2], [3,4], [转载 2014-03-14 23:36:37 · 664 阅读 · 0 评论 -
【LeetCode】Search in Rotated Sorted Array
Suppose a sorted array is rotated at some pivot unknown to you beforehand.(i.e., 0 1 2 4 5 6 7 might become 4 5 6 7 0 1 2).You are given a target value to search. If found in the array retur原创 2014-03-15 15:42:02 · 547 阅读 · 0 评论 -
【LeetCode】Climbing Stairs
You are climbing a stair case. It takes n steps to reach to the top.Each time you can either climb 1 or 2 steps. In how many distinct ways can you climb to the top?class Solution {public: i原创 2014-03-12 22:20:56 · 527 阅读 · 0 评论 -
【LeetCode】Populating Next Right Pointers in Each Node
/** * Definition for binary tree with next pointer. * struct TreeLinkNode { * int val; * TreeLinkNode *left, *right, *next; * TreeLinkNode(int x) : val(x), left(NULL), right(NULL), next(NULL)原创 2014-04-13 23:45:36 · 749 阅读 · 0 评论 -
【LeetCode】Reverse Words in a String
Given an input string, reverse the string word by word.For example,Given s = "the sky is blue",return "blue is sky the".原创 2014-04-14 00:39:17 · 784 阅读 · 0 评论 -
【LeetCode】Merge Sorted Array
Given two sorted integer arrays A and B, merge B into A as one sorted array.Note:You may assume that A has enough space (size that is greater or equal to m + n) to hold additional elements from原创 2014-04-14 23:05:41 · 745 阅读 · 0 评论 -
【LeetCode】Balanced Binary Tree
/** * Definition for binary tree * struct TreeNode { * int val; * TreeNode *left; * TreeNode *right; * TreeNode(int x) : val(x), left(NULL), right(NULL) {} * }; */class Soluti原创 2014-04-14 22:46:44 · 685 阅读 · 0 评论 -
△【LeetCode】Remove Duplicates from Sorted Array
Given a sorted array, remove the duplicates in place such that each element appear only once and return the new length.Do not allocate extra space for another array, you must do this in place with原创 2014-03-14 12:46:44 · 627 阅读 · 0 评论 -
【LeetCode】Longest Substring Without Repeating Characters
Given a string, find the length of the longest substring without repeating characters. For example, the longest substring without repeating letters for "abcabcbb" is "abc", which the length is 3. Fo原创 2014-03-13 22:42:16 · 648 阅读 · 0 评论 -
【LeetCode】Search Insert Position
Given a sorted array and a target value, return the index if the target is found. If not, return the index where it would be if it were inserted in order.You may assume no duplicates in the array.原创 2014-03-12 20:36:09 · 557 阅读 · 0 评论 -
【LeetCode】Roman to Integer
Given a roman numeral, convert it to an integer.Input is guaranteed to be within the range from 1 to 3999.class Solution {public: int romanToInt(string s) { int ret = 0; f原创 2014-03-12 22:59:22 · 638 阅读 · 0 评论 -
【LeetCode】Remove Element
Given an array and a value, remove all instances of that value in place and return the new length.The order of elements can be changed. It doesn't matter what you leave beyond the new length.cla原创 2014-03-12 23:42:22 · 623 阅读 · 0 评论 -
【LeetCode】Reverse digits of an integer
Reverse digits of an integer.Example1: x = 123, return 321Example2: x = -123, return -321class Solution {public: int reverse(int x) { int ret = 0; bool positive = t原创 2014-03-12 15:09:14 · 638 阅读 · 0 评论 -
【LeetCode】Remove Duplicates from Sorted List
Given a sorted linked list, delete all duplicates such that each element appear only once.For example,Given 1->1->2, return 1->2.Given 1->1->2->3->3, return 1->2->3./** * Definition for s原创 2014-03-12 19:49:26 · 600 阅读 · 0 评论 -
【LeetCode】Linked List Cycle
Given a linked list, determine if it has a cycle in it.Follow up:Can you solve it without using extra space?/** * Definition for singly-linked list. * struct ListNode { * int val; *原创 2014-03-12 19:31:42 · 656 阅读 · 0 评论 -
【LeetCode】Same Tree
Given two binary trees, write a function to check if they are equal or not.Two binary trees are considered equal if they are structurally identical and the nodes have the same value./** * Def原创 2014-03-12 15:56:04 · 523 阅读 · 0 评论 -
【LeetCode】Best Time to Buy and Sell Stock II
Say you have an array for which the ith element is the price of a given stock on day i.Design an algorithm to find the maximum profit. You may complete as many transactions as you like (ie, buy on原创 2014-03-12 16:03:55 · 566 阅读 · 0 评论 -
【LeetCode】Maximum Subarray
Find the contiguous subarray within an array (containing at least one number) which has the largest sum.For example, given the array [−2,1,−3,4,−1,2,1,−5,4],the contiguous subarray [4,−1,2,1] ha原创 2014-03-12 21:00:40 · 511 阅读 · 0 评论 -
【LeetCode】Merge Two Sorted Lists
Merge two sorted linked lists and return it as a new list. The new list should be made by splicing together the nodes of the first two lists思路:不需要开辟新的链表首元素小的链表的头作为新链表的头。当一个链表的元素已经比较完时,另一原创 2014-03-13 12:40:49 · 610 阅读 · 0 评论 -
△【LeetCode】Binary Tree Preorder Traversal
Given a binary tree, return the preorder traversal of its nodes' values.For example:Given binary tree {1,#,2,3}, 1 \ 2 / 3return [1,2,3]./** * Definition for bi原创 2014-03-13 20:47:39 · 631 阅读 · 0 评论 -
【LeetCode】Convert Sorted Array to Binary Search Tree
/** * Definition for binary tree * struct TreeNode { * int val; * TreeNode *left; * TreeNode *right; * TreeNode(int x) : val(x), left(NULL), right(NULL) {} * }; */class Soluti原创 2014-04-14 00:29:23 · 639 阅读 · 0 评论