#include "main.h"
#include <time.h>
#include <iostream>
#include <iomanip>
using namespace std;
int main()
{
time_t time_get = 0,time_get1 = 0;
TIME_S Time { };
while (1)
{
reset(time_get, Time);
time_get = time(0);
if (time_get != time_get1)
{
time_get1 = time_get;
get_year(time_get, Time);
get_month(time_get, Time);
get_day(time_get, Time);
get_hour(time_get, Time);
get_minute(time_get, Time);
get_second(time_get, Time);
show(Time);
#if _SHOW_TIME_ONE_LINE
cout << '\r';
#endif
}
}
return 0;
}
#include "main.h"
#include <cstdbool>
#include <ctime>
#include <iostream>
#include <iomanip>
using namespace std;
bool is_leap(unsigned int para_time)
{
if (((para_time % 400) == 0) || (((para_time % 4) == 0) && ((para_time % 100) != 0)))
return true;
else
return false;
}
void get_year(time_t& para_time1,TIME_S & para_time2)
{
unsigned int year = 1970;
while (1)
{
para_time2.year = year;
if (is_leap(year))
{
if (para_time1 < TIME_SEC_LEAP)
break;
para_time1 -= TIME_SEC_LEAP;
}
else
{
if (para_time1 < TIME_SEC_ORDN)
break;
para_time1 -= TIME_SEC_ORDN;
}
year++;
}
}
void get_month(time_t& time_para1, TIME_S& time_para2)
{
unsigned int month = 1;
while (1)
{
switch (month)
{
case 1:
case 3:
case 5:
case 7:
case 8:
case 10:
case 12:time_para2.month++; if (time_para1 < TIME_31DAY)return; time_para1 -= TIME_31DAY; break;
case 4:
case 6:
case 9:
case 11:time_para2.month++; if (time_para1 < TIME_30DAY)return; time_para1 -= TIME_30DAY; break;
case 2:
time_para2.month++;
if (is_leap(time_para2.year))
{
if (time_para1 < TIME_29DAY)
return;
time_para1 -= TIME_29DAY;
}
else
{
if (time_para1 < TIME_28DAY)
return;
time_para1 -= TIME_28DAY;
}
break;
default:
break;
}
month++;
}
}
void get_day(time_t& time_para1, TIME_S& time_para2)
{
time_para2.day = ((unsigned int)time_para1 / TIME_1DAY) + 1;
time_para1 %= TIME_1DAY;
}
void get_hour(time_t& time_para1, TIME_S& time_para2)
{
time_para2.hour = ((unsigned int)time_para1 / TIME_1HOUR) + 8;
if (time_para2.hour > 23)
time_para2.hour -= 24;
time_para1 %= TIME_1HOUR;
}
void get_minute(time_t& time_para1, TIME_S& time_para2)
{
time_para2.minute = (unsigned int)time_para1 / TIME_1MINUTE;
time_para1 %= TIME_1MINUTE;
}
void get_second(time_t& time_para1, TIME_S& time_para2)
{
time_para2.second = (unsigned int)time_para1;
}
void reset(time_t& para1, TIME_S& para2)
{
para1 = 0;
para2.day = 0;
para2.hour = 0;
para2.minute = 0;
para2.month = 0;
para2.second = 0;
para2.year = 0;
}
void show(TIME_S& para)
{
cout << para.year << "年" << para.month << fixed << setw(2)
<< setfill('0') << "月" << fixed << setw(2) << setfill('0')
<< para.day << "日 " << fixed << setw(2) << setfill('0')
<< para.hour << ":" << fixed << setw(2) << setfill('0')
<< para.minute << ":" << fixed << setw(2) << setfill('0')
<< para.second;
#if _SHOW_TIME_ONE_LINE == 0
cout << endl;
#endif
}
#pragma once
#ifndef __MAIN_H__
#define __MAIN_H__
#include <ctime>
#define _SHOW_TIME_ONE_LINE 0
#define TIME_1MINUTE 60
#define TIME_1HOUR (60 * TIME_1MINUTE)
#define TIME_1DAY (24 * TIME_1HOUR)
#define TIME_28DAY (28 * TIME_1DAY)
#define TIME_29DAY (29 * TIME_1DAY)
#define TIME_30DAY (30 * TIME_1DAY)
#define TIME_31DAY (31 * TIME_1DAY)
#define TIME_SEC_LEAP ((31 + 29 + 31 + 30 + 31 + 30 + 31 + 31 + 30 + 31 + 30 + 31) * TIME_1DAY)
#define TIME_SEC_ORDN (TIME_SEC_LEAP - (TIME_1DAY))
struct TIME_S
{
unsigned int year;
unsigned int month;
unsigned int day;
unsigned int hour;
unsigned int minute;
unsigned int second;
};
bool is_leap(unsigned int para_time);
void get_year(time_t& para_time,TIME_S & para_time2);
void get_month(time_t& time_para1, TIME_S& time_para2);
void get_day(time_t& time_para1, TIME_S& time_para2);
void get_hour(time_t& time_para1, TIME_S& time_para2);
void get_minute(time_t& time_para1, TIME_S& time_para2);
void get_second(time_t& time_para1, TIME_S& time_para2);
void reset(time_t& para1, TIME_S& para2);
void show(TIME_S& para);
#endif
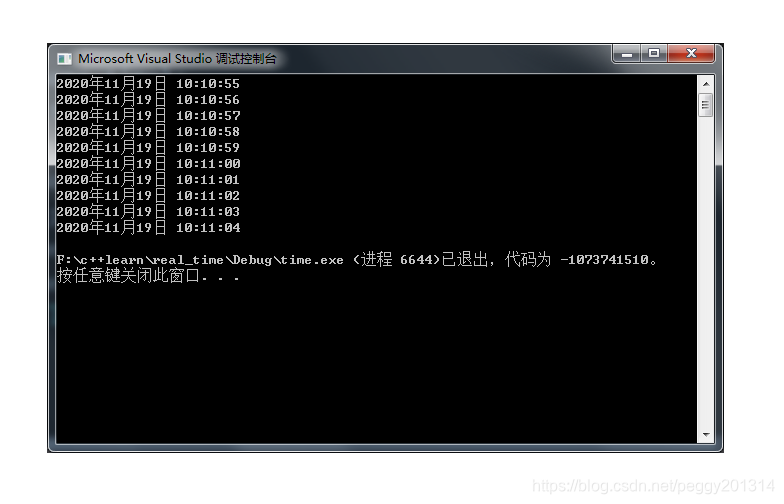