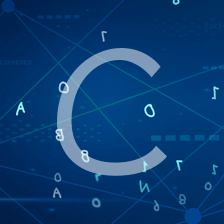
leetcode(java实现)
文章平均质量分 56
夜吟
这个作者很懒,什么都没留下…
展开
-
(java)Pascal's Triangle II
Given an index k, return the kth row of the Pascal's triangle.For example, given k = 3,Return [1,3,3,1].思路:我们可以把这个pascal三角用二维数组来解决。a[i][0]=1,a[i][j]=a[i-1][j-1]+a[i-1][j]根据此递推式就可以得出a[i原创 2015-12-10 14:27:19 · 329 阅读 · 0 评论 -
(java) Path Sum
Given a binary tree and a sum, determine if the tree has a root-to-leaf path such that adding up all the values along the path equals the given sum.For example:Given the below binary tree and sum原创 2015-12-10 14:33:23 · 222 阅读 · 0 评论 -
(java)Implement Stack using Queues
Implement the following operations of a stack using queues.push(x) -- Push element x onto stack.pop() -- Removes the element on top of the stack.top() -- Get the top element.empty() -- Return whet原创 2015-12-10 14:37:49 · 356 阅读 · 0 评论 -
(java)Remove Element
Given an array and a value, remove all instances of that value in place and return the new length.The order of elements can be changed. It doesn't matter what you leave beyond the new length.原创 2015-11-25 17:01:36 · 292 阅读 · 0 评论 -
(java)Balanced Binary Tree
Given a binary tree, determine if it is height-balanced.For this problem, a height-balanced binary tree is defined as a binary tree in which the depth of the two subtrees of every node never diffe原创 2015-11-18 18:39:56 · 353 阅读 · 0 评论 -
(java)Power of Two
Given an integer, write a function to determine if it is a power of two.思路:注意题意是判断一个数是不是2的幂。一直除以2,如果除不尽就不是2的幂,当等于1的时候,就返回true;代码如下(已通过leetcode)public class Solution { public boolean isPowerO原创 2015-11-18 18:38:30 · 383 阅读 · 0 评论 -
(java)Implement Queue using Stacks
Implement the following operations of a queue using stacks.push(x) -- Push element x to the back of queue.pop() -- Removes the element from in front of queue.peek() -- Get the front element.empty(原创 2015-11-16 09:19:00 · 279 阅读 · 0 评论 -
(java)happy number
Write an algorithm to determine if a number is "happy".A happy number is a number defined by the following process: Starting with any positive integer, replace the number by the sum of the squares原创 2015-11-16 09:14:48 · 297 阅读 · 0 评论 -
(java)Reverse Linked List
Reverse a singly linked list.思路:这个应该都会,只是简单的链表转置。就是设置三个指针一个p,一个pleft,一个pright.代码如下(已通过leetcode)public class Solution { public ListNode reverseList(ListNode head) { ListNode p=head;原创 2015-11-12 18:44:43 · 314 阅读 · 0 评论 -
(java)leetcode Number of 1 Bits
Write a function that takes an unsigned integer and returns the number of ’1' bits it has (also known as theHamming weight).For example, the 32-bit integer ’11' has binary representation 0000000原创 2015-11-12 18:34:41 · 346 阅读 · 0 评论 -
(java)leetcode 3sum cloest
Given an array S of n integers, find three integers in S such that the sum is closest to a given number, target. Return the sum of the three integers. You may assume that each input would have exact原创 2015-11-04 13:49:38 · 423 阅读 · 0 评论 -
(java)3sum
Given an array S of n integers, are there elements a, b, c in S such that a + b + c = 0? Find all unique triplets in the array which gives the sum of zero.Note:Elements in a triplet (a,b,c原创 2015-11-04 13:43:02 · 350 阅读 · 0 评论 -
(java)leetcode Longest Common Prefix
Write a function to find the longest common prefix string amongst an array of strings.思路1:本题是要找一个字符串数组的最长公共前缀。首先求出最短字符串的长度,然后从0到最短字符串长度遍历每个字符串,找到最长的公共前缀代码如下(已通过leetcode)public class Solution {原创 2015-10-30 18:47:02 · 350 阅读 · 0 评论 -
(java)leetcode Excel Sheet Column Number
Related to question Excel Sheet Column TitleGiven a column title as appear in an Excel sheet, return its corresponding column number.For example: A -> 1 B -> 2 C -> 3 ...原创 2015-10-30 18:42:10 · 350 阅读 · 0 评论 -
(java)Climbing Stairs
You are climbing a stair case. It takes n steps to reach to the top.Each time you can either climb 1 or 2 steps. In how many distinct ways can you climb to the top?思路:先计算出几个特殊情况的楼梯数目所需的步数的情况,原创 2015-11-15 12:45:53 · 553 阅读 · 0 评论 -
(java)leetcode Contains Duplicate
Given an array of integers, find if the array contains any duplicates. Your function should return true if any value appears at least twice in the array, and it should return false if every element原创 2015-10-30 18:31:11 · 364 阅读 · 0 评论 -
(java)leetcode Move Zeros
Given an array nums, write a function to move all 0's to the end of it while maintaining the relative order of the non-zero elements.For example, given nums = [0, 1, 0, 3, 12], after calling you原创 2015-10-29 19:11:29 · 633 阅读 · 0 评论 -
(java)leetcode Same Tree
Given two binary trees, write a function to check if they are equal or not.Two binary trees are considered equal if they are structurally identical and the nodes have the same value.思路:本题是比较两棵原创 2015-10-29 19:06:19 · 286 阅读 · 0 评论 -
(java)leetcode Delete Node in a Linked List
Write a function to delete a node (except the tail) in a singly linked list, given only access to that node.Supposed the linked list is 1 -> 2 -> 3 -> 4 and you are given the third node with val原创 2015-10-29 18:59:30 · 292 阅读 · 0 评论 -
(java) leetcode Add Digits
Given a non-negative integer num, repeatedly add all its digits until the result has only one digit.For example:Given num = 38, the process is like: 3 + 8 = 11, 1 + 1 = 2. Since 2 has on原创 2015-10-29 17:04:28 · 492 阅读 · 0 评论 -
(java)leetcode problem192 Nim Game
You are playing the following Nim Game with your friend: There is a heap of stones on the table, each time one of you take turns to remove 1 to 3 stones. The one who removes the last stone will be the原创 2015-10-29 16:57:53 · 456 阅读 · 0 评论 -
leetcode problem13 Rome to Integer
Given a roman numeral, convert it to an integer.Input is guaranteed to be within the range from 1 to 3999.思路:从前向后遍历这个字符串如果当前值等于前一个值 则用一个临时变量存储当前的值 如III=3;如果当前值比前一个值大 则这个临时变量存储的值应该为当前值减原创 2015-10-28 17:12:00 · 331 阅读 · 0 评论 -
leetcode Problem12 Integer to Roman
Given an integer, convert it to a roman numeral.Input is guaranteed to be within the range from 1 to 3999.思路:首先先把num按照个位十位百位千位拆分,然后对每一位上的数字按照roman to integer的对应表格,转化为对应的roman,然后就所有转化的再拼起来就是所求的原创 2015-10-28 17:06:40 · 331 阅读 · 0 评论 -
LeetCode Problem10 Regular Expression Matching
'.' Matches any single character.'*' Matches zero or more of the preceding element.The matching should cover the entire input string (not partial).The function prototype should be:bool isMatch(c原创 2015-10-26 17:30:04 · 546 阅读 · 0 评论 -
leetcode Problem8_StringtoInt
Implement atoi to convert a string to an integer.思路:本题的题目本身比较简单,但是case非常的多比如:1 "+"2 " +-12"3 " 0012a45"4 溢出等等,本题的重点就在于处理这些case。下面代码(已经通过lootcode)今天就刷到这,保持基本每天4题的速度public class原创 2015-10-24 17:56:20 · 395 阅读 · 0 评论 -
leetcode problem7 Reverse Integer
Reverse digits of an integer.Example1: x = 123, return 321Example2: x = -123, return -321思路:题目本身简单的不能再简单了,但是其中有一个边界问题,也是这个题目存在的道理,就是当输出的整数没有越界,而转置之后的整数可能会越界。比如 1534236469本身并没有越界,而转置之后就96463243原创 2015-10-24 16:45:54 · 456 阅读 · 0 评论 -
Leetcood Problem3 longest substring
Given a string, find the length of the longest substring without repeating characters. For example, the longest substring without repeating letters for "abcabcbb" is "abc", which the length is 3. Fo原创 2015-10-23 15:51:08 · 558 阅读 · 0 评论 -
Leetcode Problem4 Median of Two Sorted Arrays
There are two sorted arrays nums1 and nums2 of size m and n respectively. Find the median of the two sorted arrays. The overall run time complexity should be O(log (m+n)).思路1:本题毫无疑问第一反应肯定是使用递归求解。此原创 2015-10-23 17:10:32 · 361 阅读 · 0 评论 -
LeetCode Problem6 ZigZag Conversion
The string "PAYPALISHIRING" is written in a zigzag pattern on a given number of rows like this: (you may want to display this pattern in a fixed font for better legibility)P A H NA P L S I原创 2015-10-24 16:16:59 · 369 阅读 · 0 评论 -
(java)Remove Duplicates from Sorted List
Given a sorted linked list, delete all duplicates such that each element appear only once.For example,Given 1->1->2, return 1->2.Given 1->1->2->3->3, return 1->2->3.思路:就是一个普通的链表操作,设置两个指针,p原创 2015-11-15 12:51:40 · 306 阅读 · 0 评论 -
(java)leetcode Valid Anagram
Given two strings s and t, write a function to determine if t is an anagram of s.For example,s = "anagram", t = "nagaram", return true.s = "rat", t = "car", return false.Note:You may ass原创 2015-11-10 14:07:43 · 287 阅读 · 0 评论 -
LeetCode Palindrome Number
Determine whether an integer is a palindrome. Do this without extra space.思路:就是简单的判断是不是回文数,在头尾设置两个指针i和j,判断是不是一直相等就行。本题的关键是不要有额外的空间,所以创建一个数组就是不可行的了,我是将其转换成了字符串。代码如下,已通过(leetcode)扯淡几句:由于在机房没带耳机,没法学别原创 2015-10-24 19:49:38 · 343 阅读 · 0 评论 -
Leetcode Problem2 two numbers
Add Two NumbersYou are given two linked lists representing two non-negative numbers. The digits are stored in reverse order and each of their nodes contain a single digit. Add the two num原创 2015-10-23 14:02:37 · 410 阅读 · 0 评论 -
leetcode Problem1 two sum
Given an array of integers, find two numbers such that they add up to a specific target number.The function twoSum should return indices of the two numbers such that they add up to the target, whe原创 2015-10-22 23:28:30 · 492 阅读 · 0 评论 -
(java)Remove Duplicates from Sorted Array
Given a sorted array, remove the duplicates in place such that each element appear only once and return the new length.Do not allocate extra space for another array, you must do this in place with原创 2015-11-25 17:13:56 · 275 阅读 · 0 评论 -
(java)Binary Tree Level Order Traversal II
Given a binary tree, return the bottom-up level order traversal of its nodes' values. (ie, from left to right, level by level from leaf to root).For example:Given binary tree {3,9,20,#,#,15,7},原创 2015-11-25 17:10:31 · 232 阅读 · 0 评论 -
(java)Symmetric Tree
Given a binary tree, check whether it is a mirror of itself (ie, symmetric around its center).For example, this binary tree is symmetric: 1 / \ 2 2 / \ / \3 4 4 3But the f原创 2015-11-25 16:54:42 · 264 阅读 · 0 评论 -
(java)leetcode Lowest Common Ancestor of a Binary Search Tree
Given a binary search tree (BST), find the lowest common ancestor (LCA) of two given nodes in the BST.According to the definition of LCA on Wikipedia: “The lowest common ancestor is defined betw原创 2015-11-10 14:12:28 · 339 阅读 · 0 评论 -
(java)leetcode Invert Binary Tree
Invert a binary tree. 4 / \ 2 7 / \ / \1 3 6 9to 4 / \ 7 2 / \ / \9 6 3 1Trivia:This problem was inspired by this original tweet by Max Howe原创 2015-10-30 18:24:38 · 385 阅读 · 0 评论 -
LeetCode Problem5 LongestPalindromicSubstring
Given a string S, find the longest palindromic substring in S. You may assume that the maximum length of S is 1000, and there exists one unique longest palindromic substring.思路:1 本题有两种想法,第一种是找原创 2015-10-24 15:42:40 · 382 阅读 · 0 评论