开发环境:
- 开发工具:IntelliJ IDEA
- Java版本:1.8
- Spring Boot版本:2.1.6.RELEASE
- Spring Cloud版本:Greenwich.SR1
1、创建server-eureka微服务:
(1)server-eureka组件选择
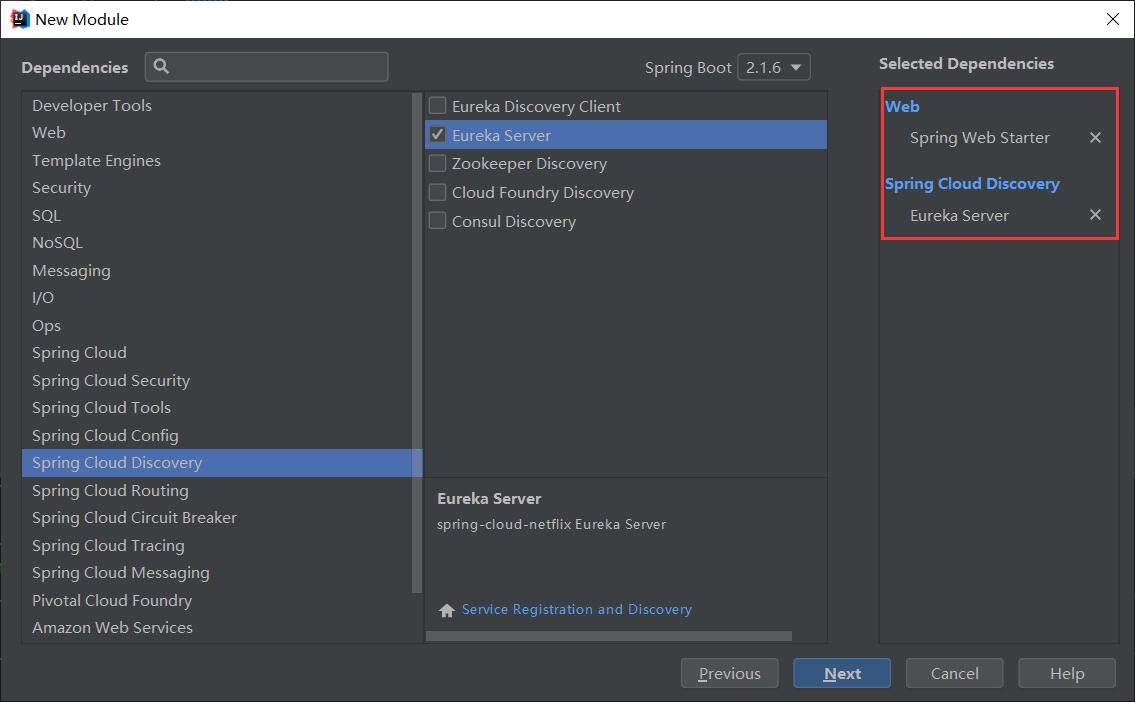
(2)server-eureka目录
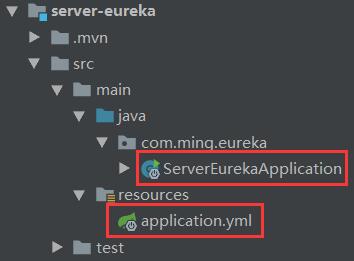
(3)application.yml
server:
#服务端口
port: 9000
spring:
application:
#服务名称
name: server
eureka:
#配置环境
environment: dev
#配置数据中心
datacenter: nanjing
instance:
#注册服务器名称
hostname: localhost
client:
#是否注册到服务中心
register-with-eureka: false
#是否检索服务
fetch-registry: false
service-url:
#客户端服务域
defaultZone: http://localhost:9000/eureka/
(4)ServerEurekaApplication
package com.ming.eureka;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.netflix.eureka.server.EnableEurekaServer;
@SpringBootApplication
@EnableEurekaServer //开启Eureka服务端
public class ServerEurekaApplication {
public static void main(String[] args) {
SpringApplication.run(ServerEurekaApplication.class, args);
}
}
2、创建server-config微服务:
(1)server-config组件选择
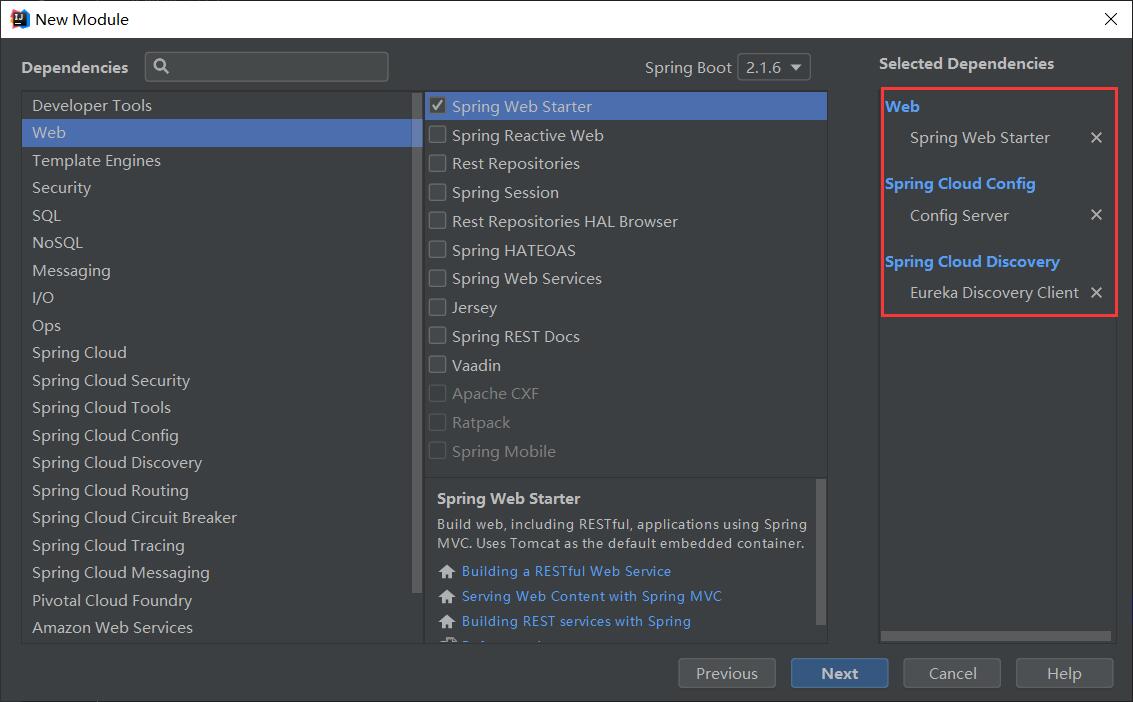
(2)server-config目录
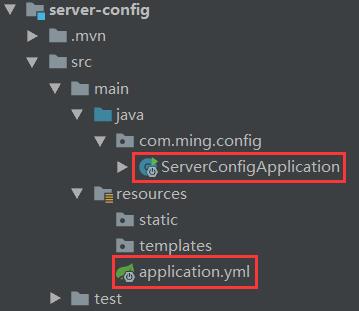
(3)ServerConfigApplication
package com.ming.config;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.config.server.EnableConfigServer;
import org.springframework.cloud.netflix.eureka.EnableEurekaClient;
@SpringBootApplication
@EnableEurekaClient //开启Eureka客户端
@EnableConfigServer //开启Config服务端
public class ServerConfigApplication {
public static void main(String[] args) {
SpringApplication.run(ServerConfigApplication.class, args);
}
}
(4)application.yml
server:
#服务端口
port: 9090
spring:
#服务名称
application:
name: config-server
cloud:
config:
server:
git:
#仓库地址
uri: https://github.com/SuperLonelyCat/config-repo.git
eureka:
client:
service-url:
#客户端服务域
defaultZone: http://localhost:9000/eureka/
3、创建client-config微服务:
(1)client-config组件选择
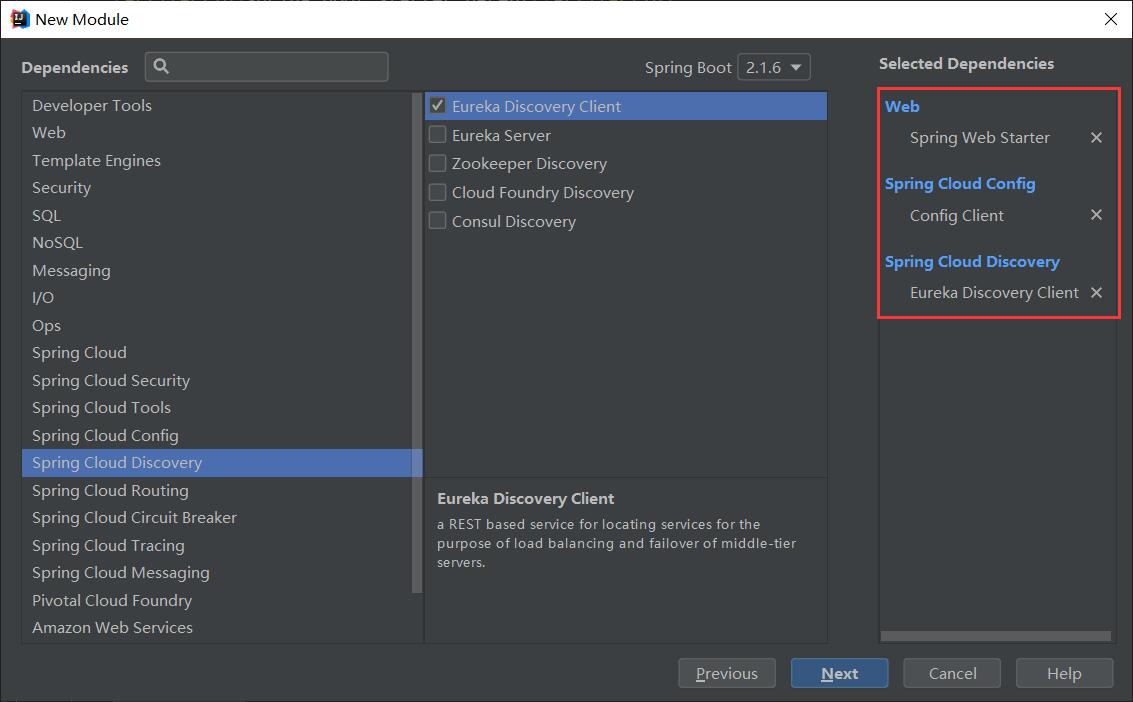
(2)server-config目录
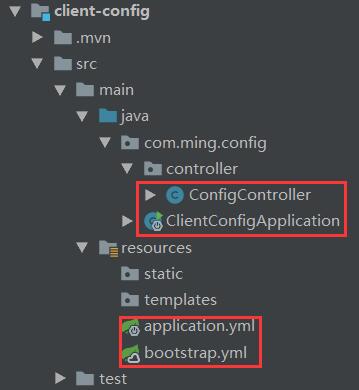
(3)ClientConfigApplication
package com.ming.config;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.netflix.eureka.EnableEurekaClient;
@SpringBootApplication
@EnableEurekaClient //开启Eureka客户端
public class ClientConfigApplication {
public static void main(String[] args) {
SpringApplication.run(ClientConfigApplication.class, args);
}
}
(4)application.yml
server:
#服务端口
port: 9091
(5)bootstrap.yml
#系统优先加载bootstrap.yml文件,一般用于配置系统级别的参数
spring:
#服务名称
application:
name: config-client
cloud:
config:
#配置开发环境
profile: dev
#config服务端serviceId
discovery:
#开启config服务发现
enabled: true
#config服务端serviceId,即client-config的服务名称
service-id: config-server
#设置分支
label: feature
eureka:
client:
service-url:
#客户端服务域
defaultZone: http://localhost:9000/eureka/
(5)ConfigController
package com.ming.config.controller;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class ConfigController {
@Value("${username}")
private String username;
@Value("${password}")
private String password;
@RequestMapping("/getMsg")
public String getMsg() {
return "username: " + username + " password: " + password;
}
}
4、GitHub仓库文件:
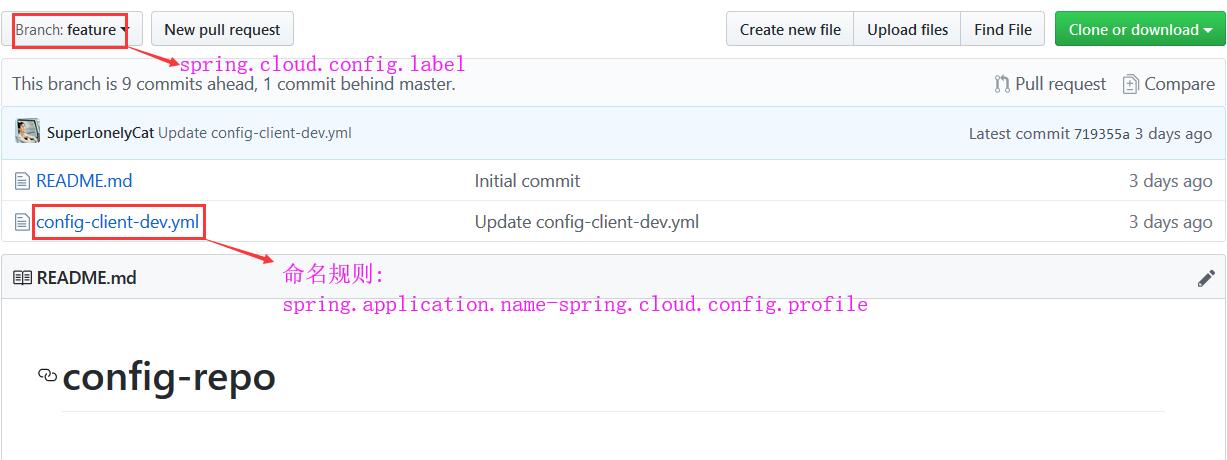
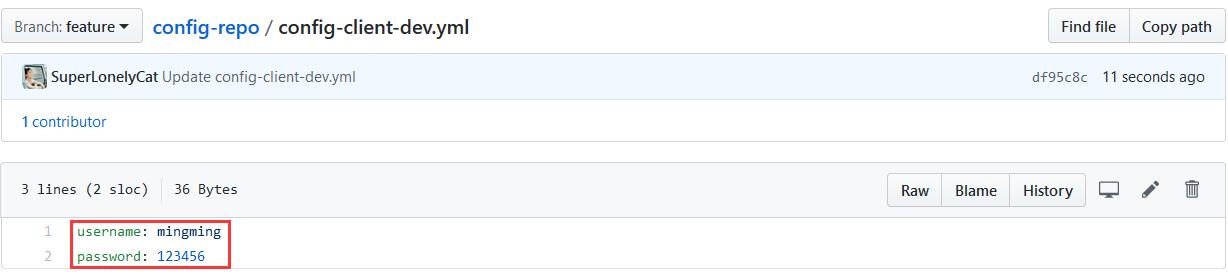
5、工程测试:
先依次点击ServerEurekaApplication、ServerConfigApplication,工程启动成功后。在浏览器地址栏访问 http://localhost:9000,其结果如下(两个微服务实例启动正常):
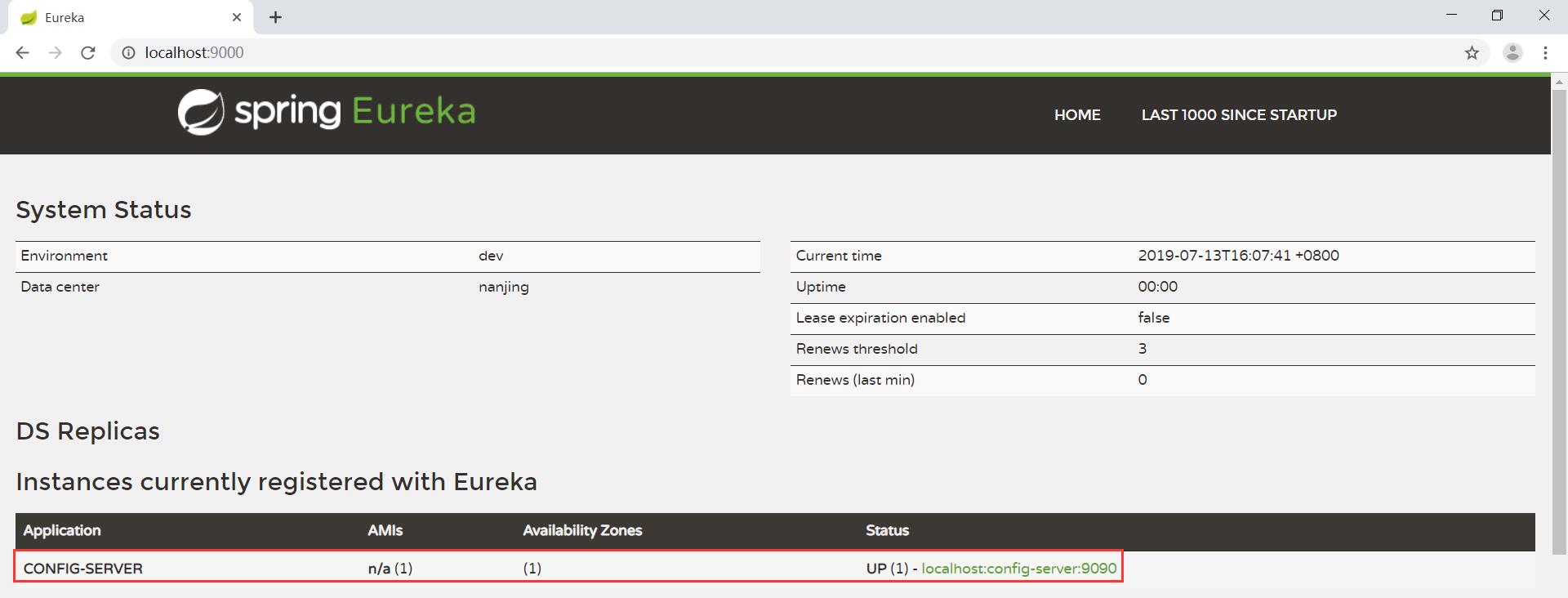
再点击ServerConfigApplication,工程启动完成后。在浏览器地址栏访问 http://localhost:9091/getMsg, 其结果如下:
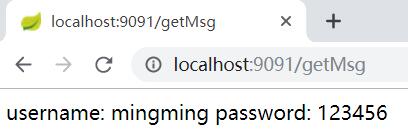