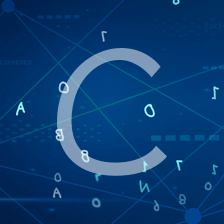
LeetCode
文章平均质量分 74
LarryNLPIR
专注NLP/IR/Machine Learning/Data Mining
展开
-
LeetCode Longest Common Prefix
Write a function to find the longest common prefix string amongst an array of strings.思路分析:这题很简单,基本就是以第一个字符串为标准,同时扫描后面的字符串对应index 字符是否相同,找到最大的相同前缀。但是实现的时候还是要注意一些corner case,比如输入数组为空或者只包含一个字符串的情况。AC Co原创 2015-02-23 11:09:36 · 1772 阅读 · 0 评论 -
LeetCode Maximum Depth of Binary Tree
Given a binary tree, find its maximum depth.The maximum depth is the number of nodes along the longest path from the root node down to the farthest leaf node.思路分析:这题比较简单,关于树的题目通常都可以用递归解决,这题也不例外,递归解法的思原创 2015-03-19 03:50:24 · 1668 阅读 · 0 评论 -
LeetCode 题目总结/分类
注:此分类仅供大概参考,没有精雕细琢。有不同意见欢迎评论~利用堆栈:http://oj.leetcode.com/problems/evaluate-reverse-polish-notation/http://oj.leetcode.com/problems/longest-valid-parentheses/ (也可以用一维数组,贪心)http://oj.leetcode.com/proble转载 2015-03-21 11:29:13 · 9165 阅读 · 0 评论 -
LeetCode Best Time to Buy and Sell Stock II
Say you have an array for which the ith element is the price of a given stock on day i.Design an algorithm to find the maximum profit. You may complete as many transactions as you like (ie, buy one an原创 2015-03-29 12:01:45 · 1507 阅读 · 0 评论 -
LeetCode Best Time to Buy and Sell Stock
Say you have an array for which the ith element is the price of a given stock on day i.If you were only permitted to complete at most one transaction (ie, buy one and sell one share of the stock), des原创 2015-03-29 12:15:13 · 1542 阅读 · 0 评论 -
LeetCode Surrounded Regions
Given a 2D board containing 'X' and 'O', capture all regions surrounded by 'X'.A region is captured by flipping all 'O's into 'X's in that surrounded region.For example,X X X XX O O XX X O XX O X X原创 2015-03-29 12:26:28 · 1292 阅读 · 0 评论 -
LeetCode First Missing Positive
Given an unsorted integer array, find the first missing positive integer.For example,Given [1,2,0] return 3,and [3,4,-1,1] return 2.Your algorithm should run in O(n) time and uses constant space.思路分析:原创 2015-03-29 13:01:58 · 1727 阅读 · 0 评论 -
LeetCode Longest Valid Parentheses
Given a string containing just the characters '(' and ')', find the length of the longest valid (well-formed) parentheses substring.For "(()", the longest valid parentheses substring is "()", which ha原创 2015-03-29 12:47:21 · 1661 阅读 · 0 评论 -
LeetCode Clone Graph
Clone an undirected graph. Each node in the graph contains a label and a list of its neighbors.OJ's undirected graph serialization:Nodes are labeled uniquely.We use # as a separator for each node, and原创 2015-03-29 12:36:21 · 1784 阅读 · 0 评论 -
LeetCode Longest Consecutive Sequence
Given an unsorted array of integers, find the length of the longest consecutive elements sequence.For example,Given [100, 4, 200, 1, 3, 2],The longest consecutive elements sequence is [1, 2, 3, 4]. Re原创 2015-03-22 12:08:06 · 1684 阅读 · 0 评论 -
LeetCode Minimum Depth of Binary Tree
Given a binary tree, find its minimum depth.The minimum depth is the number of nodes along the shortest path from the root node down to the nearest leaf node.思路分析:这题和Maximum Depth of Binary Tree类似,但是现原创 2015-03-19 13:25:30 · 1473 阅读 · 0 评论 -
LeetCode Same Tree
Given two binary trees, write a function to check if they are equal or not.Two binary trees are considered equal if they are structurally identical and the nodes have the same value.思路分析:判断两个树是否相同,基本也原创 2015-03-19 04:04:40 · 1592 阅读 · 0 评论 -
LeetCode First Missing Positive
Given an unsorted integer array, find the first missing positive integer.For example,Given [1,2,0] return 3,and [3,4,-1,1] return 2.Your algorithm should run in O(n) time and uses constant space.思路分析:原创 2014-12-31 15:07:00 · 3564 阅读 · 0 评论 -
LeetCode Find Peak Element
A peak element is an element that is greater than its neighbors.Given an input array where num[i] ≠ num[i+1], find a peak element and return its index.The array may contain multiple peaks, in that cas原创 2015-01-05 06:55:59 · 3934 阅读 · 0 评论 -
LeetCode Intersection of Two Linked Lists
Write a program to find the node at which the intersection of two singly linked lists begins.For example, the following two linked lists:A: a1 → a2 ↘ c原创 2015-01-05 07:33:08 · 2983 阅读 · 0 评论 -
LeetCode Search in Rotated Sorted Array
Suppose a sorted array is rotated at some pivot unknown to you beforehand.(i.e., 0 1 2 4 5 6 7 might become 4 5 6 7 0 1 2).You are given a target value to search. If found in the array return its inde原创 2014-11-29 04:08:29 · 2288 阅读 · 0 评论 -
LeetCode Search Insert Position
Suppose a sorted array is rotated at some pivot unknown to you beforehand.(i.e., 0 1 2 4 5 6 7 might become 4 5 6 7 0 1 2).You are given a target value to search. If found in the array return its inde原创 2014-11-29 03:35:44 · 1830 阅读 · 0 评论 -
LeetCode Permutations
Given a collection of numbers, return all possible permutations.For example,[1,2,3] have the following permutations:[1,2,3], [1,3,2], [2,1,3], [2,3,1], [3,1,2], and [3,2,1].思路分析:这题是Permutations II问题的简原创 2014-11-23 13:01:17 · 2026 阅读 · 0 评论 -
LeetCode Unique Paths
A robot is located at the top-left corner of a m x n grid (marked 'Start' in the diagram below).The robot can only move either down or right at any point in time. The robot is trying to reach the bott原创 2015-01-05 07:13:37 · 1939 阅读 · 1 评论 -
LeetCode Jump Game
Given an array of non-negative integers, you are initially positioned at the first index of the array.Each element in the array represents your maximum jump length at that position.Determine if you ar原创 2015-01-05 07:24:32 · 1687 阅读 · 0 评论 -
LeetCode Word Search
Given a 2D board and a word, find if the word exists in the grid.The word can be constructed from letters of sequentially adjacent cell, where "adjacent" cells are those horizontally or vertically nei原创 2015-02-15 09:15:11 · 1931 阅读 · 0 评论 -
LeetCode Maximum Gap
Given an unsorted array, find the maximum difference between the successive elements in its sorted form.Try to solve it in linear time/space.Return 0 if the array contains less than 2 elements.You may原创 2015-03-18 08:40:39 · 2752 阅读 · 0 评论 -
LeetCode Insert Interval
Given a set of non-overlapping intervals, insert a new interval into the intervals (merge if necessary).You may assume that the intervals were initially sorted according to their start times.Example 1原创 2015-03-18 12:50:48 · 1248 阅读 · 0 评论 -
LeetCode Reverse Integer
Reverse digits of an integer.Example1: x = 123, return 321Example2: x = -123, return -321click to show spoilers.Have you thought about this?Here are some good questions to ask before coding. Bonus poi原创 2015-03-18 14:15:36 · 1254 阅读 · 0 评论 -
LeetCode Contains Duplicate III
Given an array of integers, find out whether there are two distinct indices i and j in the array such that the difference between nums[i] and nums[j] is at most t and the difference between i and j is原创 2015-06-15 14:02:42 · 3816 阅读 · 0 评论 -
LeetCode Invert Binary Tree
这是最近比较火的一个题目,因为一条推特的转播“Google HR:我们90%的工程师都用你写的软件,但是你竟然不会在白板上面反转一颗二叉树,所以滚吧”,各方看法不一,但看这个题目,的确是一个很简单的题目。考察最基本的递归和树操作。下面给出了递归实现和借助栈的迭代实现(非递归实现)。后一个版本的可扩展性更好,可以处理更大的树。实在是容易题,就是DFS遍历一遍树节点,把每个树节点的左右孩子互换就可以了。或许是那个ios开发牛人不屑于准备就去Google面试,结果被爆,与其说是能力问题,不如说是态度问题。原创 2015-06-15 10:46:20 · 3750 阅读 · 1 评论 -
LeetCode Missing Ranges [LeetCode Book Problem]
Given a sorted integer array where the range of elements are [lower, upper] inclusive, return its missing ranges.For example, given [0, 1, 3, 50, 75], lower = 0 and upper = 99, return ["2", "4->49", "原创 2015-06-15 12:59:49 · 3232 阅读 · 0 评论 -
LeetCode Implement Stack using Queues
Implement the following operations of a stack using queues.push(x) -- Push element x onto stack.pop() -- Removes the element on top of the stack.top() -- Get the top element.empty() -- Return whether原创 2015-07-27 16:38:42 · 3067 阅读 · 0 评论 -
LeetCode Find Minimum in Rotated Sorted Array II
Follow up for "Find Minimum in Rotated Sorted Array":What if duplicates are allowed?Would this affect the run-time complexity? How and why?Suppose a sorted array is rotated at some pivot unknown to yo原创 2015-07-27 16:25:57 · 2817 阅读 · 0 评论 -
LeetCode Maximal Square
Given a 2D binary matrix filled with 0's and 1's, find the largest square containing all 1's and return its area.For example, given the following matrix:1 0 1 0 01 0 1 1 11 1 1 1 11 0 0 1 0Return原创 2015-07-27 16:09:17 · 3164 阅读 · 0 评论 -
LeetCode Product of Array Except Self
Given an array of n integers where n > 1, nums, return an array output such that output[i] is equal to the product of all the elements of nums except nums[i].Solve it without division and in O(n).For原创 2015-07-19 14:47:06 · 4011 阅读 · 0 评论 -
LeetCode Majority Element
Given an array of size n, find the majority element. The majority element is the element that appears more than ⌊ n/2 ⌋ times.You may assume that the array is non-empty and the majority element always原创 2015-07-20 14:58:41 · 3112 阅读 · 0 评论 -
LeetCode Implement Queue using Stacks
Implement the following operations of a queue using stacks.push(x) -- Push element x to the back of queue.pop() -- Removes the element from in front of queue.peek() -- Get the front element.empty() --原创 2015-07-21 14:48:29 · 3307 阅读 · 0 评论 -
LeetCode Number of 1 Bits
Write a function that takes an unsigned integer and returns the number of ’1' bits it has (also known as the Hamming weight).For example, the 32-bit integer ’11' has binary representation 000000000000原创 2015-06-15 12:45:05 · 2290 阅读 · 0 评论 -
LeetCode Binary Tree Right Side View
Given a binary tree, imagine yourself standing on the right side of it, return the values of the nodes you can see ordered from top to bottom.For example:Given the following binary tree, 1原创 2015-05-25 14:37:25 · 2255 阅读 · 0 评论 -
LeetCode Permutation Sequence
The set [1,2,3,…,n] contains a total of n! unique permutations.By listing and labeling all of the permutations in order,We get the following sequence (ie, for n = 3):"123""132""213""231""312""321"Give原创 2015-04-20 13:29:07 · 1503 阅读 · 0 评论 -
LeetCode Copy List with Random Pointer
A linked list is given such that each node contains an additional random pointer which could point to any node in the list or null.Return a deep copy of the list.思路分析:这题要求拷贝链表,包括内容,next指针和random指针。容易想原创 2015-04-20 14:08:09 · 1408 阅读 · 0 评论 -
LeetCode House Robber
You are a professional robber planning to rob houses along a street. Each house has a certain amount of money stashed, the only constraint stopping you from robbing each of them is that adjacent house原创 2015-04-20 12:33:11 · 3502 阅读 · 0 评论 -
LeetCode Contains Duplicate II
Given an array of integers and an integer k, find out whether there there are two distinct indices i and j in the array such that nums[i] = nums[j] and the difference between iand j is at most k.思路分析:原创 2015-05-31 11:54:39 · 7837 阅读 · 0 评论 -
LeetCode Contains Duplicate
Given an array of integers, find if the array contains any duplicates. Your function should return true if any value appears at least twice in the array, and it should return false if every element is原创 2015-05-31 12:00:03 · 5865 阅读 · 0 评论