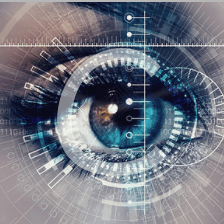
LeetCode
yang20141109
这个作者很懒,什么都没留下…
展开
-
Reverse Integer
Reverse digits of an integer.Example1: x = 123, return 321Example2: x = -123, return -321 解析:此问题难点溢出,比如,输入1234567899,反转后的数字为9987654321,该数字大于最大的整数,我们应该输出0。 方法一:首先我们把整数转换为字符串,然后对该字符串进行反转,最原创 2016-04-25 22:26:17 · 241 阅读 · 0 评论 -
C++标准库中next_permutation和pre_permutation实现原理
标准库中next_permutation函数:找当前序列中元素排列的下一个排列,按照字典顺序进行排列。比如说数组排列"123",那么它的下一个排列为"132",并且返回true。如果当前序列没有下一个排列,我们返回false,且把当前排列置为最小的排列,比如说:排列"321",因为该排列已经是最大的排列,所以它没有下一个排列。我们把该排列置为"123",并且返回false。 标准库实现两原创 2016-05-06 17:47:30 · 3591 阅读 · 3 评论 -
矩阵中从左上角到右下角的路径条数
题目:给定一个n*m矩阵,求从左上角到右下角总共存在多少条路径,每次只能向右走或者向下走。解法一:和上一篇文章的思想相似,在此不再重复叙述。int uniquePaths(int m, int n) { if (m <= 0 || n <= 0) return 0; vector data(n,1); int cur = 0; for(int i = 1; i < m; ++i)原创 2016-05-31 11:53:38 · 11079 阅读 · 0 评论 -
ReOrder List
Given a singly linked list L: L0→L1→…→Ln-1→Ln,reorder it to: L0→Ln→L1→Ln-1→L2→Ln-2→…You must do this in-place without altering the nodes' values.For example,Given{1,2,3,4}, reorder it to{1,4,2,3}.原创 2016-04-23 12:02:21 · 325 阅读 · 0 评论 -
Add Two Numbers
You are given two linked lists representing two non-negative numbers. The digits are stored in reverse order and each of their nodes contain a single digit. Add the two numbers and return it as a link原创 2016-04-23 20:23:35 · 230 阅读 · 0 评论 -
动态规划之最长递增子序列
题目一:给定一个长度为N的数组,找出一个最长的单调递增子序列(不一定连续,但是顺序不能乱)。并返回单调递增子序列的长度。 例如:给定一个长度为8的数组A{1,3,5,2,4,6,7,8},则其最长的单调递增子序列为{1,2,4,6,7,8},我们返回其长度6。 题目二:在题目一的基础上,我们要返回该子序列中的元素。例如,给定一个长度为8的数组A{1,3,5,2,4,6,7,8}原创 2016-05-08 17:12:24 · 900 阅读 · 0 评论 -
Longest Substring Without Repeating Characters
Given a string, find the length of the longest substring without repeating characters.Examples:Given "abcabcbb", the answer is "abc", which the length is 3.Given "bbbbb", the answer is "b"原创 2016-04-23 22:03:47 · 222 阅读 · 0 评论 -
连续子数组的最大乘积
题目:给定一个数组,求出这个数组中连续子数组的最大乘积,例如{2,3,-4,5},最大乘积为2*3=6。 解析:动态规划思想,保存两个临时变量maxProduct和minProduct,这两个临时变量分别存储,以当前位置结尾的连续子数组的最大乘积和最小乘积。int maxProduct(vector& nums) { int maxRes = nums[0]; int maxPro原创 2016-06-29 12:09:11 · 873 阅读 · 0 评论 -
leetcode 12 Integer to Roman(整型数转换为罗马数字)
罗马数字采用七个罗马字母作数字、即Ⅰ(1)、X(10)、C(100)、M(1000)、V(5)、L(50)、D(500),比如说IX(4),CCCXLV(345)。我们把1到3999数字转换为罗马数字。代码如下:string intToRoman(int num){ string M[] = { "", "M", "MM", "MMM" }; string C[] = { "", "C",原创 2016-07-23 20:44:34 · 403 阅读 · 0 评论 -
leetcode 13 Roman to Integer(罗马数字转换为整数)
把给定罗马数字转换为整型数字。int romanToInt(string s) { int res = 0; for (int i = 0; i < s.size(); ++i) { if (s[i] == 'I') { if (s[i + 1] == 'V') { res += 4; i++; } else if (s[i + 1] ==原创 2016-07-23 20:56:15 · 387 阅读 · 0 评论 -
集合中的子集(三种方法)
给定一个集合,求出这个集合的所有子集,比如:集合{1,2,3},子集为{},{1},{2},{3},{1,2},{1,3},{2,3},{1,2,3}。 解法一:递归void subsets(vector> &res, vector &path,vector &nums, int start){ res.push_back(path); for (int i = start; i原创 2016-07-01 11:46:46 · 3282 阅读 · 0 评论 -
leetcode 43 Multiply Strings (字符串相乘)
Given two numbers represented as strings, return multiplication of the numbers as a string.Note: The numbers can be arbitrarily large and are non-negative. Converting the input string to i原创 2016-05-28 16:31:31 · 454 阅读 · 0 评论 -
leetcode 1 Two Sum(在无序数组中找两个数之和与目标值相等,两种方法)
Given an array of integers, return indices of the two numbers such that they add up to a specific target. You may assume that each input would have exactly one solution.Example: Given nums原创 2016-05-28 09:26:51 · 3036 阅读 · 0 评论 -
Palindrome Number(回文整数)
Determine whether an integer is a palindrome. Do this without extra space.解析:把该整数倒转过来,如果和原来的数字相等,则说明是回文整数。注意:任何负数都不是回文整数。bool isPalindrome(int x){ if (x < 0) return false; int res = 0; int val原创 2016-04-26 17:06:50 · 386 阅读 · 0 评论 -
String to Integer (atoi字符串转换为整数)
Implement atoi to convert a string to an integer.解析:(1)字符串必须以数字、空格、正负号开头。如果以空格开头,第一个非空格字符必须是数字或者正负号。正负号不能连续出现在字符串开始位置。(2)字符串中含有字母,那么我们只考虑字符之前的数字。比如:"123e123",转换以后为123。(3)如果字符转换以后的数字大于最大整数的值,则返回最原创 2016-04-26 17:16:47 · 408 阅读 · 0 评论 -
Container With Most Water (容器中盛最多的水)
Given n non-negative integers a1, a2, ..., an, where each represents a point at coordinate (i, ai). n vertical lines are drawn such that the two endpoints of line i is at (i, ai) and (i, 0). Find two原创 2016-04-26 20:10:18 · 471 阅读 · 0 评论 -
3Sum Closest(离目标值最近的三数之和)
Given an array S of n integers, find three integers in S such that the sum is closest to a given number, target. Return the sum of the three integers. You may assume that each input would have exactly原创 2016-04-27 10:28:26 · 516 阅读 · 0 评论 -
3Sum(数组中三个数之和为零)
Given an array S of n integers, are there elements a, b, c in S such that a + b + c = 0? Find all unique triplets in the array which gives the sum of zero.For example, given array S = {-1 0 1 2 -1 -原创 2016-04-27 09:09:10 · 834 阅读 · 0 评论 -
leetcode(198,213)House Robber(I,II)
题目一: You are a professional robber planning to rob houses along a street. Each house has a certain amount of money stashed, the only constraint stopping you from robbing each of them is that adja原创 2016-05-26 20:27:20 · 286 阅读 · 0 评论 -
leetcode 66(plus one)
Given a non-negative number represented as an array of digits, plus one to the number. The digits are stored such that the most significant digit is at the head of the list. 例如:数组{1,2,3},加一以原创 2016-05-26 22:10:23 · 314 阅读 · 0 评论 -
leetcode 328 Odd Even Linked List(调整链表使得奇数位置的元素位于偶数位置元素之前)
Given a singly linked list, group all odd nodes together followed by the even nodes. Please note here we are talking about the node number and not the value in the nodes. You should try to do it原创 2016-05-27 15:31:17 · 1781 阅读 · 0 评论 -
leetcode 299 Bulls and Cows
You are playing the following Bulls and Cows game with your friend: You write down a number and ask your friend to guess what the number is. Each time your friend makes a guess, you provide a hint t原创 2016-05-27 19:56:42 · 304 阅读 · 0 评论 -
顺时针九十度旋转矩阵(两种方法)
题目:给定一个n*n的矩阵,顺时针把矩阵旋转90度。比如说{{1,2,3}{4,5,6}{7,8,9}}。顺时针旋转90度为{{7,4,1}{8,5,2}{9,6,3}}。 解法一:时间复杂度为o(n*n),空间复杂度为o(n)。步骤:(1)把矩阵的行当做一个整体,逆序矩阵中的行,也就是说把matrix[i]行和matrix[len - i]进行交换。(2)在交换后的矩阵中,按照对角原创 2016-05-27 21:37:27 · 24981 阅读 · 0 评论 -
集合中的子集2(含有重复元素)
给定一个集合,含有重复元素,求这个集合的子集,例如:集合{1,2,2},子集为{},{1},{2},{1,2},{2,2},{1,2,2}。 解法一:递归void subsetsWithDup(vector> &res, vector &path,vector &nums, int start){ res.push_back(path); for (int i = start; i原创 2016-07-01 17:33:38 · 824 阅读 · 0 评论