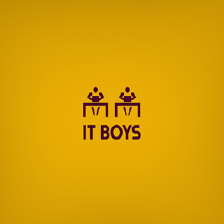
Leetcode面试题解
希望开一个解答leetcode.com上面试题的专栏,与众多的刷题爱好者交流和分享解题过程,探讨做题的方法和思路,望通过。
hjiam2
渴望成为一名码农,爱生活,爱运动,爱编程
展开
-
【Leetcode】Copy List with Random Pointer
A linked list is given such that each node contains an additional random pointer which could point to any node in the list or null.Return a deep copy of the list.题意:存在一个链表,里面有两个指针,一个指针指向下一节点,另原创 2014-04-14 15:38:21 · 1586 阅读 · 0 评论 -
【Leetcode】Flatten Binary Tree to Linked List
Given a binary tree, flatten it to a linked list in-place.For example,Given 1 / \ 2 5 / \ \ 3 4 6The flattened tree should look like: 1原创 2013-11-26 19:28:16 · 733 阅读 · 0 评论 -
【Leetcode】Pow(x, n)
Implement pow(x, n).题目的要求是计算x的n次方,主要需要注意的地方有以下几点:1.n应该分正数,0,负数三部分讨论,主要任何数的0次方为1,包括0的0次方。2.本题采用递归的方式计算,否则如果只是设计一个for循环的话,会出现Time Limit Exceeded的错误,本题的时间复杂度为class Solution {public: double p原创 2013-11-26 20:33:50 · 794 阅读 · 0 评论 -
【Leetcode】Add Binary
Given two binary strings, return their sum (also a binary string).For example,a = "11"b = "1"Return "100".给出两个二进制数表示的字符串,要求计算它们的和,并返回字符串,水题,注意一下字符串的范围是0至size-1即可。class Solution {pub原创 2013-11-26 21:05:30 · 588 阅读 · 0 评论 -
【Leetcode】Reverse Linked List II
Reverse a linked list from position m to n. Do it in-place and in one-pass.For example:Given 1->2->3->4->5->NULL, m = 2 and n = 4,return 1->4->3->2->5->NULL.Note:Given m, n satisfy t原创 2013-11-26 21:31:40 · 774 阅读 · 0 评论 -
【Leetcode】Edit Distance
Given two words word1 and word2, find the minimum number of steps required to convert word1 to word2. (each operation is counted as 1 step.)You have the following 3 operations permitted on a word:原创 2013-11-28 19:01:32 · 763 阅读 · 0 评论 -
【Leetcode】Construct Binary Tree from Preorder and Inorder Traversal
Given preorder and inorder traversal of a tree, construct the binary tree.Note:You may assume that duplicates do not exist in the tree.题意:给出二叉树的前序遍历和中序遍历序列,要求重构二叉树。这道题之前的博客有谈到,这里就不再仔细叙述了原创 2013-11-28 19:16:20 · 812 阅读 · 0 评论 -
【Leetcode】Remove Duplicates from Sorted List II
Given a sorted linked list, delete all nodes that have duplicate numbers, leaving only distinct numbers from the original list.For example,Given 1->2->3->3->4->4->5, return 1->2->5.Given 1->1-原创 2013-11-28 19:50:53 · 747 阅读 · 0 评论 -
【Leetcode】Letter Combinations of a Phone Number
Given a digit string, return all possible letter combinations that the number could represent.A mapping of digit to letters (just like on the telephone buttons) is given below.Input:Digit st原创 2013-11-28 20:25:07 · 715 阅读 · 0 评论 -
【Leetcode】Palindrome Partitioning
Given a string s, partition s such that every substring of the partition is a palindrome.Return all possible palindrome partitioning of s.For example, given s = "aab",Return [ ["aa","b"],原创 2013-11-28 22:07:35 · 705 阅读 · 0 评论 -
【Leetcode】Gas Station
There are N gas stations along a circular route, where the amount of gas at station i is gas[i].You have a car with an unlimited gas tank and it costs cost[i] of gas to travel from station i to原创 2013-11-29 13:44:35 · 972 阅读 · 0 评论 -
【Leetcode】Longest Substring Without Repeating Characters
Given a string, find the length of the longest substring without repeating characters. For example, the longest substring without repeating letters for "abcabcbb" is "abc", which the length is 3. Fo原创 2013-11-29 18:36:43 · 761 阅读 · 0 评论 -
【Leetcode】Distinct Subsequences
Given a string S and a string T, count the number of distinct subsequences of T in S.A subsequence of a string is a new string which is formed from the original string by deleting some (can be non原创 2013-12-04 01:28:54 · 653 阅读 · 0 评论 -
【Leetcode】Combination Sum II
Given a collection of candidate numbers (C) and a target number (T), find all unique combinations in C where the candidate numbers sums to T.Each number in C may only be used once in the combina原创 2013-12-05 12:01:25 · 724 阅读 · 0 评论 -
【Leetcode】Reverse Nodes in k-Group
题意:给你一个链表和正整数k,让链表每隔k个结点进行反转,最后若剩下不足k个结点,则不反转。个人感觉这题难度是有一定的,想了很久,也没有能得到一个比较清晰的解法。于是网上搜索了下,得到了以下这一种方案,我觉得关键点有这么几点:1)新建一个守护结点safeG,令其指向结点为head,然后定义三个ListNode指针pre,cur和post,分别指向safeG,head,head->n原创 2013-12-05 14:47:48 · 1072 阅读 · 0 评论 -
【Leetcode】Jump Game II
题意:给你一个数组,由数组起点0开始,每次可走的长度为0至所在位置的元素大小,问最少需要多少步可以走到终点?一开始想到了递归的方法,但是很显然,这会超时,下面是超时的方法。class Solution {public: int jump(int A[], int n) { return Jump(A,n,0); } int Jump(in原创 2013-12-05 16:41:03 · 737 阅读 · 0 评论 -
【Leetcode】Add Two Numbers
You are given two linked lists representing two non-negative numbers. The digits are stored in reverse order and each of their nodes contain a single digit. Add the two numbers and return it as a link原创 2013-12-05 17:22:42 · 786 阅读 · 0 评论 -
【Leetcode】Anagrams
Given an array of strings, return all groups of strings that are anagrams.Note: All inputs will be in lower-case.题意:给你一个字符串数组,返回所有变位字符串的集合(变位字符串的意思是两个字符串包含的字符类型和各个字符出现的次数均相等)。解题思路:1)对数组的每个原创 2013-12-05 19:37:45 · 681 阅读 · 0 评论 -
【Leetcode】Merge k Sorted Lists
Merge k sorted linked lists and return it as one sorted list. Analyze and describe its complexity.题意:将k个排序链表合并为一个新的排序链表。要注意的地方:1)memset的用法,这里花了我很长时间,关键在于char buffer1[20]和char *buffer2=new int[原创 2013-12-05 21:25:11 · 808 阅读 · 0 评论 -
【Leetcode】Two Sum
Given an array of integers, find two numbers such that they add up to a specific target number.The function twoSum should return indices of the two numbers such that they add up to the target, whe原创 2013-12-05 21:58:46 · 897 阅读 · 0 评论 -
【Leetcode】4Sum
Given an array S of n integers, are there elements a, b, c, and d in S such that a + b + c + d = target? Find all unique quadruplets in the array which gives the sum of target.Note:Elements原创 2013-12-05 23:02:53 · 773 阅读 · 0 评论 -
【Leetcode】ZigZag Conversion
The string "PAYPALISHIRING" is written in a zigzag pattern on a given number of rows like this: (you may want to display this pattern in a fixed font for better legibility)P A H NA P L S I原创 2013-12-15 12:52:01 · 1976 阅读 · 0 评论 -
【Leetcode】Longest Palindromic Substring
Given a string S, find the longest palindromic substring in S. You may assume that the maximum length of S is 1000, and there exists one unique longest palindromic substring.题意:给定一个字符串,返回字符串中最原创 2013-12-15 13:50:56 · 984 阅读 · 0 评论 -
【Leetcode】Sqrt(x)
Implement int sqrt(int x).Compute and return the square root of x.题意:计算整数x的平方根。这里我采用的方法是二分法,因为int能表示的最大整数为2^31-1=2147483647,开根号为46340。所以从1-46340区间进行二分查找,这里需要注意的是查找时,如果判断medium*mediumx是否成立,原创 2013-12-15 14:49:19 · 1213 阅读 · 0 评论 -
【Leetcode】Best Time to Buy and Sell Stock III
Say you have an array for which the ith element is the price of a given stock on day i.Design an algorithm to find the maximum profit. You may complete at most two transactions.Note:You may原创 2013-12-15 17:46:18 · 2202 阅读 · 2 评论 -
【Leetcode】Rotate List
Given a list, rotate the list to the right by k places, where k is non-negative.For example:Given 1->2->3->4->5->NULL and k = 2,return 4->5->1->2->3->NULL.题意:将链表右移k个位置。这题不难,但是有几个细节要注意:原创 2013-12-17 18:02:00 · 948 阅读 · 0 评论 -
【Leetcode】Valid Palindrome
Given a string, determine if it is a palindrome, considering only alphanumeric characters and ignoring cases.For example,"A man, a plan, a canal: Panama" is a palindrome."race a car" is not a原创 2013-12-17 19:03:15 · 853 阅读 · 0 评论 -
【Leetcode】First Missing Positive
Given an unsorted integer array, find the first missing positive integer.For example,Given [1,2,0] return 3,and [3,4,-1,1] return 2.Your algorithm should run in O(n) time and uses constant原创 2013-12-17 20:27:41 · 933 阅读 · 0 评论 -
【Leetcode】Permutation Sequence
The set [1,2,3,…,n] contains a total of n! unique permutations.By listing and labeling all of the permutations in order,We get the following sequence (ie, for n = 3):"123""132""213""231""3原创 2013-12-17 21:20:39 · 1000 阅读 · 0 评论 -
【Leetcode】Recover Binary Search Tree
Two elements of a binary search tree (BST) are swapped by mistake.Recover the tree without changing its structure.Note:A solution using O(n) space is pretty straight forward. Could you devis原创 2013-12-17 22:32:14 · 1041 阅读 · 0 评论 -
【Leetcode】Insert Interval
Given a set of non-overlapping intervals, insert a new interval into the intervals (merge if necessary).You may assume that the intervals were initially sorted according to their start times.E原创 2013-12-18 12:42:01 · 953 阅读 · 0 评论