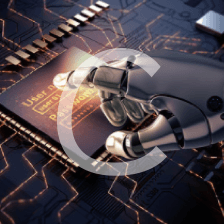
LeetCode
AI记忆
这个作者很懒,什么都没留下…
展开
-
[LeetCode]Best Time to Buy and Sell Stock
#define INF 0x6FFFFFFFclass Solution {public: int maxProfit(vector &prices) { // Start typing your C/C++ solution below // DO NOT write int main() function int maxDiff = 0; int curMinPrice原创 2013-05-27 19:14:16 · 835 阅读 · 0 评论 -
[LeetCode]Best Time to Buy and Sell Stock II
class Solution {public: int maxProfit(vector &prices) { // Start typing your C/C++ solution below // DO NOT write int main() function //sum all the consecutive positive diff int sum = 0; f原创 2013-05-27 19:14:21 · 913 阅读 · 0 评论 -
[LeetCode]Best Time to Buy and Sell Stock III
class Solution {//Let f[i][j] to be the maximum sum of j segments from the first i numbers, where the last element we choose is a[i]. //We have two strategies to achieve it:////1.Choosing the opti原创 2013-05-27 19:14:26 · 1378 阅读 · 0 评论 -
[LeetCode]Integer to Roman
class Solution {//refer to http://discuss.leetcode.com/questions/194/integer-to-roman @Ark//roman number//I II III IV V VI VII VIII IX//I 1//V 5//X 10//L 50//C 100//D 500//M 1000public: st原创 2013-05-29 19:42:49 · 1658 阅读 · 0 评论 -
[LeetCode]Letter Combinations of a Phone Number
class Solution {//static declare and definition with local scopepublic: void letterCombinations_aux(int step, string& path, vector& ans, const string& digits) { //pay attention to this kind of s原创 2013-05-29 19:43:12 · 3000 阅读 · 0 评论 -
[LeetCode]Pascal Triangle II
class Solution {public: vector getRow(int rowIndex) { // Start typing your C/C++ solution below // DO NOT write int main() function vector ans(rowIndex+2, 0); vector tmp(ro原创 2013-05-30 10:27:40 · 913 阅读 · 0 评论 -
[LeetCode]Pascal's Triangle
class Solution {public: vector > generate(int numRows) { // Start typing your C/C++ solution below // DO NOT write int main() function vector > ans; ans.resize(numRows);原创 2013-05-30 10:27:34 · 1620 阅读 · 0 评论 -
[LeetCode]Reverse Integer
class Solution {public: int reverse(int x) { // Start typing your C/C++ solution below // DO NOT write int main() function int sign = 1; if(x < 0) sign = -1; unsigned int num = x < 0 ? -x原创 2013-05-31 10:39:53 · 848 阅读 · 0 评论 -
[LeetCode]Remove Nth Node From End of List
struct ListNode { int val; ListNode *next; ListNode(int x) : val(x), next(NULL) {}};class Solution {public: ListNode *removeNthFromEnd(ListNode *head, int n) { // Start typing your原创 2013-05-31 10:39:34 · 1394 阅读 · 0 评论 -
[LeetCode]Roman to Integer
class Solution {//I V X L C D M//find out the regular patternpublic: int romanToInt(string s) { // Start typing your C/C++ solution below // DO NOT write int main() function if (s.size() ==原创 2013-06-01 09:44:15 · 924 阅读 · 0 评论 -
[LeetCode]Scramble String
class Solution {//recursive solution is straight forward, just think about a binary tree, we should check//if those sub trees are same(1. left==right, right==left or 2. left==left, right==right)//原创 2013-06-01 09:44:48 · 1903 阅读 · 0 评论 -
[LeetCode]Search Insert Position
class Solution {public: int searchInsert(int A[], int n, int target) { // Start typing your C/C++ solution below // DO NOT write int main() function int l = 0; int r = n-1; while (l <= r)原创 2013-06-01 09:45:26 · 1106 阅读 · 0 评论 -
[LeetCode]Valid Sudoku
class Solution {public: bool isValidSudoku(vector > &board) { // Start typing your C/C++ solution below // DO NOT write int main() function return CheckBlock(board) && CheckStrip(board); } b原创 2013-06-03 09:08:55 · 2456 阅读 · 0 评论 -
[LeetCode]Word Ladder II
class Solution { //Split the problem in three steps: // //1.build the adjacency list //2.do a BFS to get a vector> prev array. For example, prev[1] = [2, 3, 0] means we can go from (2 to 1) or (3原创 2013-06-03 09:09:29 · 2345 阅读 · 0 评论 -
[LeetCode]Two Sum
class Solution {public: struct Node { int num, idx; Node(int _num = 0, int _idx = 0):num(_num),idx(_idx){} bool operator < (const Node& orh) const { if(num == orh.num) return idx原创 2013-05-28 12:08:11 · 2898 阅读 · 1 评论 -
[LeetCode]Median of Two Sorted Arrays
class Solution {//more detail refer to: http://fisherlei.blogspot.com/2012/12/leetcode-median-of-two-sorted-arrays.html//using the method of getting the kth number in the two sorted array to solve t原创 2013-05-30 10:24:31 · 1678 阅读 · 0 评论 -
[LeetCode]Add Two Numbers
struct ListNode { int val; ListNode *next; ListNode(int x) : val(x), next(NULL) {}};class Solution {//dummy node in the head of the result, avoid judging NULL of headpublic: ListNode *addTwoN原创 2013-05-27 19:13:52 · 1040 阅读 · 0 评论 -
[LeetCode]Longest Substring Without Repeating Characters
class Solution {//put last char in the end of the string //to avoid extra judge, when go to the end without repeating charpublic: int lengthOfLongestSubstring(string s) { // Start typing your C/原创 2013-05-29 19:43:52 · 1774 阅读 · 0 评论 -
[LeetCode]String to Integer (atoi)
class Solution {//note://1. NULL//2. sign//3. prefix ' '//4. out of range, to avoid this we use long longpublic: int atoi(const char *str) { // Start typing your C/C++ solution below // DO原创 2013-06-01 09:46:08 · 4720 阅读 · 3 评论 -
[LeetCode]Longest Palindromic Substring
class Solution {//insert special character, then enumerate every character in workStr and record the max substring O(n^2)public: string longestPalindrome(string s) { // Start typing your C/C++ so原创 2013-05-29 19:43:48 · 1499 阅读 · 0 评论 -
[LeetCode]ZigZag Conversion
class Solution {//be careful with the special case//when done with coding, figure out some cases (including illegal, normal, edge cases)//to run with this solution, this will help to get a bug-free原创 2013-06-03 09:09:50 · 1131 阅读 · 0 评论 -
[LeetCode]Palindrome Number
class Solution {//compare both the left most and the right most digit, //if not equal return falsepublic: bool isPalindrome(int x) { // Start typing your C/C++ solution below // DO NOT write i原创 2013-05-30 10:26:01 · 3667 阅读 · 4 评论 -
[LeetCode]Regular Expression Matching
class Solution {//1. If the next character of p is NOT '*', then it must match the current character of s. //Continue pattern matching with the next character of both s and p.//2. If the next chara原创 2013-05-31 10:39:13 · 2499 阅读 · 0 评论 -
[LeetCode]Trapping Rain Water
class Solution {//O(n)//find the highest bar as one end of the wall//then check left side from left to right, //record the left most high height "h" along the way, //if A[i] < h && A[i] < A[mid],原创 2013-05-28 12:08:04 · 1539 阅读 · 0 评论 -
[LeetCode]Container With Most Water
class Solution {//Find two lines, which together with x-axis forms a container, such that the container contains the most water.//if do not together with x-axis forms a container, //and together wi原创 2013-05-28 12:06:56 · 1540 阅读 · 0 评论 -
[LeetCode]3Sum Closest
class Solution {//1. sort the sequence//2. fix i, then increase j and decrease j accordinglypublic: int threeSumClosest(vector &num, int target) { // Start typing your C/C++ solution below //原创 2013-05-27 19:13:22 · 919 阅读 · 0 评论 -
[LeetCode]Longest Common Prefix
class Solution {//1. find out the minimum size string as the pivot string//2. check every other string and update the minSizepublic: string longestCommonPrefix(vector &strs) { // Start原创 2013-05-29 19:43:16 · 1988 阅读 · 0 评论 -
[LeetCode]3Sum
class Solution {public: void threeSum(int step, int sum, vector& path, vector>& ans, const vector& num, vector& used) { if(path.size() == 3) { ans.push_back(path); return;原创 2013-05-27 19:12:49 · 1046 阅读 · 0 评论 -
[LeetCode]4Sum
class Solution {//fix i, j, then let p q walk to find out the fourSum target O(n^3)//there exists O(n^2logn) solutionpublic: vector > fourSum(vector &num, int target) { // Start typing your C/C+原创 2013-05-27 19:13:06 · 1875 阅读 · 0 评论 -
[LeetCode]Swap Nodes in Pairs
struct ListNode { int val; ListNode *next; ListNode(int x) : val(x), next(NULL) {}};class Solution {//when finished the code, find come cases to test it firstpublic: ListNode *swapPairs(ListN原创 2013-05-28 12:07:51 · 1166 阅读 · 0 评论 -
[LeetCode]Reverse Nodes in k-Group
struct ListNode { int val; ListNode *next; ListNode(int x) : val(x), next(NULL) {}};class Solution {//once the link order of the list is changed, //we must keep the previous pointer(p in the c原创 2013-05-31 10:40:02 · 1650 阅读 · 0 评论 -
[LeetCode]Remove Duplicates from Sorted Array
class Solution {public: int removeDuplicates(int A[], int n) { // Start typing your C/C++ solution below // DO NOT write int main() function if(0 == n) return 0; int len = 1; for (int i =原创 2013-05-31 10:39:17 · 900 阅读 · 0 评论 -
[LeetCode]Remove Element
class Solution {public: int removeElement(int A[], int n, int elem) { // Start typing your C/C++ solution below // DO NOT write int main() function int len = 0; for (int i = 0; i < n; ++i)原创 2013-05-31 10:39:31 · 1554 阅读 · 2 评论 -
[LeetCode]Divide Two Integers
class Solution {//note: //1. min negative int number->unsigned int//2. construct divisor tablepublic: int divide(int dividend, int divisor) { // Start typing your C/C++ solution below // DO N原创 2013-05-28 12:07:19 · 1703 阅读 · 0 评论 -
[LeetCode]Next Permutation
class Solution {//from right to left find the minimum larger number to replace the current number//once find, swap(current, minimum larger number), then sort(current+1, end)//find out the regular p原创 2013-05-30 10:25:57 · 1653 阅读 · 0 评论 -
[LeetCode]Substring with Concatenation of All Words
class Solution {//because all the word have same length, if they do not have the same length then they can not be solve by //the solution below//so it can be solve by iterating finding the substrin原创 2013-06-01 09:46:20 · 2175 阅读 · 0 评论 -
[LeetCode]Longest Valid Parentheses
class Solution {//once meet Parentheses, we should think of stack//then it is some thing trivialpublic: int longestValidParentheses(string s) { // Start typing your C/C++ solution below // DO原创 2013-05-29 19:43:55 · 1559 阅读 · 0 评论 -
[LeetCode]Search in Rotated Sorted Array
class Solution {//observation is the key, try to solve it by modifying binary searchpublic: int search(int A[], int n, int target) { // Start typing your C/C++ solution below // DO NOT write in原创 2013-06-01 09:45:14 · 1748 阅读 · 0 评论 -
[LeetCode]Valid Parentheses
class Solution {//stackpublic: bool isValid(string s) { // Start typing your C/C++ solution below // DO NOT write int main() function stack charStack; for (int i = 0; i < s.size(); ++i) {原创 2013-06-03 09:08:48 · 987 阅读 · 0 评论 -
[LeetCode]Generate Parentheses
class Solution {public: void generateParenthesis_aux(vector& remainNum, stack& stackPath, string& path, vector& ans) { if(remainNum[0] == 0 && remainNum[1] == 0) { ans.push_back(path);原创 2013-05-29 19:42:28 · 2088 阅读 · 0 评论