提示:文章写完后,目录可以自动生成,如何生成可参考右边的帮助文档
前言
实现划线功能并保存成图片:
使用UGUI拓展中的UILineRender,该资源UnityUIExtensions-2019-6.unitypackage可以在其他人的分享中找到
。
效果: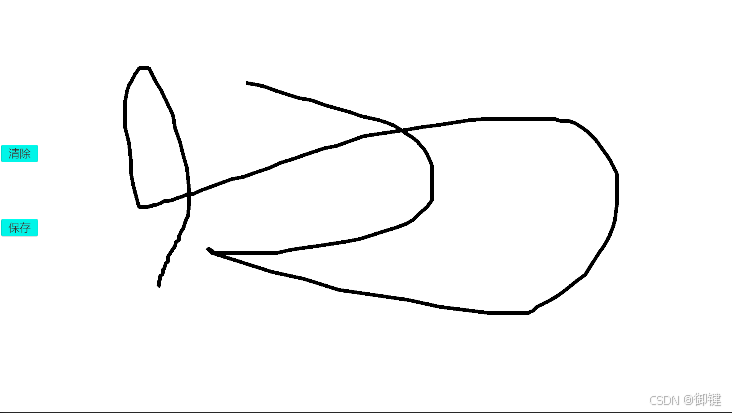
一、实现思路
制作UILineRender预制体:
设置transform和UILineRender组件的颜色和线条粗细。
二、核心代码
1.划线
代码如下(示例):
public List<Vector2> points = new List<Vector2>();
Vector3 point;
UILineRenderer currentLine;//当前line
public bool isSave;//是否正在保存
void Update()
{
if (isSave) return;
//window 或编辑器
if (Application.platform == RuntimePlatform.WindowsPlayer || Application.platform == RuntimePlatform.WindowsEditor)
{
WindowDrawLine();
}//安卓
else if (Application.platform == RuntimePlatform.Android)
{
}
}
/// <summary>
/// window平台划线
/// </summary>
private void WindowDrawLine()
{
if (Input.GetMouseButton(0))
{
if (this.points.Count == 0)
{
currentLine = CreatLine();
}
if (currentLine!=null&&point != Input.mousePosition)
{
point = Input.mousePosition;
points.Add(Input.mousePosition);
currentLine.Points = this.points.ToArray();
}
}
if (Input.GetMouseButtonUp(0))
{
if (this.currentLine != null)
{
this.point = Vector3.zero;
this.points.Clear();
this.currentLine = null;
}
}
}
2.完整代码
代码如下(示例):
using Sirenix.OdinInspector; using System; using System.Collections; using System.Collections.Generic; using System.IO; using System.Linq; using System.Threading.Tasks; using UnityEngine; using UnityEngine.UI.Extensions; /// <summary> /// 划线image /// </summary> public class DrawLineImage : MonoBehaviour { public GameObject linePre;//线的预制体 public Transform lineRoot;//线的根节点 public List<UILineRenderer> lines= new List<UILineRenderer>();//所有线条 public GameObject btns;//功能按钮 public GameObject tip;//提示消息 void Start() { } public List<Vector2> points = new List<Vector2>(); Vector3 point; UILineRenderer currentLine;//当前line public bool isSave;//是否正在保存 void Update() { if (isSave) return; //window 或编辑器 if (Application.platform == RuntimePlatform.WindowsPlayer || Application.platform == RuntimePlatform.WindowsEditor) { WindowDrawLine(); }//安卓 else if (Application.platform == RuntimePlatform.Android) { } } /// <summary> /// window平台划线 /// </summary> private void WindowDrawLine() { if (Input.GetMouseButton(0)) { if (this.points.Count == 0) { currentLine = CreatLine(); } if (currentLine!=null&&point != Input.mousePosition) { point = Input.mousePosition; points.Add(Input.mousePosition); currentLine.Points = this.points.ToArray(); } } if (Input.GetMouseButtonUp(0)) { if (this.currentLine != null) { this.point = Vector3.zero; this.points.Clear(); this.currentLine = null; } } } /// <summary> /// 创建line /// </summary> /// <returns></returns> private UILineRenderer CreatLine() { GameObject go=Instantiate(linePre,this.lineRoot); go.SetActive(true); UILineRenderer ui=go.GetComponent<UILineRenderer>(); lines.Add(ui); return ui; } /// <summary> /// 将Texture2D转png /// </summary> public void SavePng() { StartCoroutine(StartSavePng()); } IEnumerator StartSavePng() { this.isSave = true; this.btns.SetActive(false); yield return new WaitForEndOfFrame(); Texture2D tex = ScreenCapture.CaptureScreenshotAsTexture(); byte[] bs= tex.EncodeToPNG(); //保存 this.tip.SetActive(true); using (FileStream fs=new FileStream(Application.streamingAssetsPath+"/签名"+DateTime.Now.Ticks+".png",FileMode.CreateNew,FileAccess.Write,FileShare.Read)) { fs.Write(bs); } this.btns.SetActive(true); this.tip.SetActive(false); this.isSave = false; #if UNITY_EDITOR AssetDatabase.Refresh(); #endif } /// <summary> /// 清除 /// </summary> public void OnClearLine() { this.currentLine=null; this.points.Clear(); this.point = Vector3.zero; foreach (var item in lines) { DestroyImmediate(item.gameObject); } lines.Clear(); } }
总结
以上划线功能实现简单,需要用到UI拓展,功能也比较简单。