栈
由于是链式结构的栈,所以栈的容量基本上可是等于无限。
#include<stdio.h>
#include<stdlib.h>
typedef int DataType;
typedef struct Node
{
DataType data;
int lengh;
struct Node * next;
}Stack;
void init_stack(Stack *stack);
//压栈
void stack_push(Stack *stack, DataType data);
//出栈
bool stack_pop(Stack *stack);
//显示栈顶元素
DataType get_top(Stack *stack);
//显示栈内的所有元素
bool show_stack(Stack *stack);
int main()
{
Stack stack;
init_stack(&stack);
printf("把元素0 1 2 3 4 5依次压进栈里\n");
stack_push(&stack,0);
stack_push(&stack,1);
stack_push(&stack,2);
stack_push(&stack,3);
stack_push(&stack,4);
stack_push(&stack,5);
printf("显示目前栈内元素\n");
show_stack(&stack);
printf("出栈1次");
stack_pop(&stack);
printf("显示目前栈内元素\n");
show_stack(&stack);
printf("出栈2次");
stack_pop(&stack);
stack_pop(&stack);
printf("显示目前栈内元素\n");
show_stack(&stack);
printf("目前栈顶元素为%d\n",get_top(&stack));
return 0;
}
//初始化栈
void init_stack(Stack *stack)
{
stack = (Stack *)malloc(sizeof(Stack));
stack->next = NULL;
stack->lengh = 0;
}
//压栈
void stack_push(Stack *stack, DataType data)
{
Stack *p = (Stack *)malloc(sizeof(Stack));
p->data = data;
p->next = stack->next;
stack->next = p;
stack->lengh ++;
}
//出栈
bool stack_pop(Stack *stack)
{
Stack *p = stack->next;
if(stack->next == NULL)
{
printf("栈空\n");
return false;
}
stack->next = p->next;
free(p);
stack->lengh --;
return true;
}
//显示栈顶元素
DataType get_top(Stack *stack)
{
if(stack->lengh == 0)
{
printf("栈空\n");
return false;
}
else
return stack->next->data;
}
//显示栈内的所有元素
bool show_stack(Stack *stack)
{
if(stack->lengh == 0)
{
printf("栈空\n");
return false;
}
Stack *p = stack;
printf("[");
for(int i = 0; i < stack->lengh; i++)
{
printf("%d, ",p->next->data);
p = p->next;
}
printf("]\n");
}
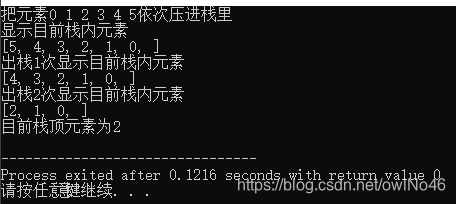