栈
由于使用数组写的,所以初始化的时候需要给定栈的容量大小,需要考虑栈是否满的情况,其实数组也是可以写像链式栈那种不会满的特性的, 可以直接通过malloc()函数来进行扩容, 我上一篇博客用顺序表就实现了这个功能。
#include<stdio.h>
#include<stdlib.h>
typedef int DataType;
typedef struct{
DataType *data;
int MaxSize;
int top;
}Stack;
//初始化栈
void init_stack(Stack *stack, int Max);
//判断栈是否为空
bool is_empty(Stack *stack);
//判断栈是否满
bool is_full(Stack *stack);
//压栈
bool stack_push(Stack *stack, DataType data);
//出栈
bool stack_pop(Stack *stack);
//获取栈顶元素
DataType get_top(Stack *stack);
//显示栈的所有元素
bool show_stack(Stack *stack);
int main()
{
Stack stack;
init_stack(&stack,6);
stack_push(&stack,0);
stack_push(&stack,1);
stack_push(&stack,2);
stack_push(&stack,3);
stack_push(&stack,4);
stack_push(&stack,5);
stack_push(&stack,6);
show_stack(&stack);
stack_pop(&stack);
stack_pop(&stack);
show_stack(&stack);
stack_pop(&stack);
stack_pop(&stack);
stack_pop(&stack);
stack_pop(&stack);
stack_pop(&stack);
show_stack(&stack);
stack_push(&stack,6);
show_stack(&stack);
printf("%d", get_top(&stack)) ;
return 0;
}
//初始化栈
void init_stack(Stack *stack, int Max)
{
stack->MaxSize = Max;
stack->data = (DataType*)malloc(sizeof(DataType) * Max);
if(stack->data == NULL)
{
printf("栈内存分配失败\n");
exit(-1);
}
stack->top = -1;
return;
}
//判断栈是否为空
bool is_empty(Stack *stack)
{
if(stack->top == -1)
return true;
else
return false;
}
//判断栈是否满
bool is_full(Stack *stack)
{
if(stack->top >= stack->MaxSize - 1)
return true;
else
return false;
}
//压栈
bool stack_push(Stack *stack, DataType data)
{
if(is_full(stack))
{
printf("栈满,不能添加元素\n");
return false;
}
stack->top ++;
stack->data[stack->top] = data;
return true;
}
//出栈
bool stack_pop(Stack *stack)
{
if(is_empty(stack))
{
printf("栈空,不能出栈元素\n");
return false;
}
stack->top --;
return true;
}
//获取栈顶元素
DataType get_top(Stack *stack)
{
return stack->data[stack->top];
}
//显示栈的所有元素
bool show_stack(Stack *stack)
{
if(is_empty(stack))
{
printf("栈空,没有元素\n");
return false;
}
printf("[");
for(int i = 0; i <= stack->top; i++)
{
printf("%d, ",stack->data[i]);
}
printf("]\n");
return true;
}
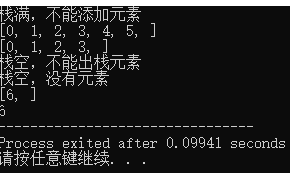