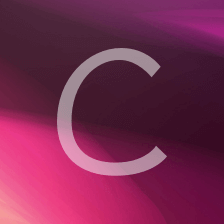
leetcode
iboxty
https://tyliupku.github.io/
展开
-
[leetcode]Maximum Product Subarray
Find the contiguous subarray within an array (containing at least one number) which has the largest product.For example, given the array [2,3,-2,4], the contiguous subarray [2,3] has the largest produ原创 2015-04-17 18:18:25 · 788 阅读 · 0 评论 -
[leetcode]Balanced Binary Tree
Given a binary tree, determine if it is height-balanced.For this problem, a height-balanced binary tree is defined as a binary tree in which the depth of the two subtrees of every node never differ by原创 2015-03-25 15:58:29 · 415 阅读 · 0 评论 -
[leetcode]Insertion Sort List
Sort a linked list using insertion sort.正好复习一下插入排序,它时间复杂度是O(n^2).最优复杂度:当输入数组就是排好序的时候,复杂度为O(n) (快速排序在这种情况下会产生O(n^2)的复杂度)。 最差复杂度:当输入数组为倒序时,复杂度为O(n^2)它是一种In-place sort,不需要额外的空间消耗。 实现的算法如下: (http://b原创 2015-03-25 13:56:59 · 420 阅读 · 0 评论 -
[leetcode]Unique Paths II
Follow up for “Unique Paths”:Now consider if some obstacles are added to the grids. How many unique paths would there be?An obstacle and empty space is marked as 1 and 0 respectively in the grid.DP 有障原创 2015-03-17 19:28:50 · 512 阅读 · 0 评论 -
[leetcode]Binary Tree Preorder Traversal
Given a binary tree, return the preorder traversal of its nodes’ values.For example: Given binary tree {1,#,2,3},1 \ 2 / 3树的前序遍历/** * Definition for binary tree * struct TreeNode原创 2015-03-22 23:25:18 · 535 阅读 · 0 评论 -
[leetcode]Factorial Trailing Zeroes
Given an integer n, return the number of trailing zeroes in n!.Note: Your solution should be in logarithmic time complexity.一开始就想1~n中统计被2整除和被5整除的因子有多少,取两个中较小的。但复杂度是O(nlgn),不符合题目要求。class Solution {publ原创 2015-03-21 12:48:45 · 308 阅读 · 0 评论 -
[leetcode]Sort List
Sort a linked list in O(n log n) time using constant space complexity.归并排序,找中点用快慢指针。/** * Definition for singly-linked list. * struct ListNode { * int val; * ListNode *next; * ListNode原创 2015-03-21 19:51:30 · 452 阅读 · 0 评论 -
[leetcode]Pascal's Triangle
Given numRows, generate the first numRows of Pascal’s triangle.For example, given numRows = 5, Return[ [1], [1,1], [1,2,1], [1,3,3,1], [1,4,6,4,1] ]class Solution {public: ve原创 2015-03-21 20:34:22 · 460 阅读 · 0 评论 -
[leetcode]Min Stack
Design a stack that supports push, pop, top, and retrieving the minimum element in constant time.push(x) – Push element x onto stack. pop() – Removes the element on top of the stack. top() – Get the原创 2015-03-21 20:19:25 · 426 阅读 · 0 评论 -
[leetcode]Repeated DNA Sequences
All DNA is composed of a series of nucleotides abbreviated as A, C, G, and T, for example: “ACGAATTCCG”. When studying DNA, it is sometimes useful to identify repeated sequences within the DNA.Write a原创 2015-03-17 20:03:18 · 479 阅读 · 0 评论 -
[leetcode]Dungeon Game
The demons had captured the princess (P) and imprisoned her in the bottom-right corner of a dungeon. The dungeon consists of M x N rooms laid out in a 2D grid. Our valiant knight (K) was initially posi原创 2015-03-21 12:10:46 · 435 阅读 · 0 评论 -
[leetcode]Remove Duplicates from Sorted List II
Given a sorted linked list, delete all nodes that have duplicate numbers, leaving only distinct numbers from the original list.For example, Given 1->2->3->3->4->4->5, return 1->2->5. Given 1->1->1->2原创 2015-04-04 17:11:11 · 812 阅读 · 0 评论 -
[leetcode]Remove Duplicates from Sorted List
Given a sorted linked list, delete all duplicates such that each element appear only once.For example, Given 1->1->2, return 1->2. Given 1->1->2->3->3, return 1->2->3.链表的简单应用,一开始没有加if(head==NULL) ret原创 2015-04-04 10:41:33 · 380 阅读 · 0 评论 -
[leetcode]Combination Sum
Given a set of candidate numbers (C) and a target number (T), find all unique combinations in C where the candidate numbers sums to T.The same repeated number may be chosen from C unlimited number of t原创 2015-04-15 16:23:02 · 619 阅读 · 0 评论 -
[leetcode]Subsets
Given a set of distinct integers, S, return all possible subsets.Note: Elements in a subset must be in non-descending order. The solution set must not contain duplicate subsets. For example, If S =原创 2015-04-14 10:06:48 · 374 阅读 · 0 评论 -
[leetcode]Combinations
Given two integers n and k, return all possible combinations of k numbers out of 1 … n.For example, If n = 4 and k = 2, a solution is:[ [2,4], [3,4], [2,3], [1,2], [1,3], [1,4], ]这道原创 2015-04-15 10:08:54 · 514 阅读 · 0 评论 -
[leetcode]Best Time to Buy and Sell Stock
Say you have an array for which the ith element is the price of a given stock on day i.If you were only permitted to complete at most one transaction (ie, buy one and sell one share of the stock), desi原创 2015-04-08 16:21:21 · 667 阅读 · 0 评论 -
[leetcode]Subsets II
Given a collection of integers that might contain duplicates, S, return all possible subsets.Note: Elements in a subset must be in non-descending order. The solution set must not contain duplicate su转载 2015-04-14 12:51:34 · 487 阅读 · 0 评论 -
[leetcode]Word Break II
Given a string s and a dictionary of words dict, add spaces in s to construct a sentence where each word is a valid dictionary word.Return all such possible sentences.For example, given s = “catsanddo原创 2015-04-08 15:51:16 · 692 阅读 · 0 评论 -
[leetcode]House Robber
You are a professional robber planning to rob houses along a street. Each house has a certain amount of money stashed, the only constraint stopping you from robbing each of them is that adjacent houses原创 2015-04-08 10:05:33 · 676 阅读 · 0 评论 -
[leetcode]Word Search
Given a 2D board and a word, find if the word exists in the grid.The word can be constructed from letters of sequentially adjacent cell, where “adjacent” cells are those horizontally or vertically neig原创 2015-04-13 12:34:41 · 701 阅读 · 0 评论 -
[leetcode]Single Number II
Given an array of integers, every element appears three times except for one. Find that single one.Note: Your algorithm should have a linear runtime complexity. Could you implement it without using ex原创 2015-04-05 11:19:13 · 538 阅读 · 0 评论 -
[leetcode]Single Number
Given an array of integers, every element appears twice except for one. Find that single one.Note:Your algorithm should have a linear runtime complexity. Could you implement it without using e转载 2015-04-05 10:41:19 · 408 阅读 · 0 评论 -
[leetcode]Reverse Bits
Reverse bits of a given 32 bits unsigned integer.For example, given input 43261596 (represented in binary as 00000010100101000001111010011100), return 964176192 (represented in binary as 00111001011110原创 2015-03-17 20:05:22 · 309 阅读 · 0 评论 -
[leetcode]Excel Sheet Column Number
Related to question Excel Sheet Column TitleGiven a column title as appear in an Excel sheet, return its corresponding column number.class Solution {public: int titleToNumber(string s) { i原创 2015-03-17 19:57:32 · 443 阅读 · 0 评论 -
[leetcode]Maximum Depth of Binary Tree
Given a binary tree, find its maximum depth.The maximum depth is the number of nodes along the longest path from the root node down to the farthest leaf node.DFS/** * Definition for binary tree * str原创 2015-03-18 12:57:21 · 471 阅读 · 0 评论 -
[leetcode]Binary Tree Postorder Traversal
Given a binary tree, return the postorder traversal of its nodes’ values.For example: Given binary tree {1,#,2,3}, 1 \ 2 / 3 return [3,2,1].Note: Recursive solution is trivial原创 2015-03-25 12:35:50 · 401 阅读 · 0 评论 -
[leetcode]Same Tree
Given two binary trees, write a function to check if they are equal or not.Two binary trees are considered equal if they are structurally identical and the nodes have the same value./** * Definition f原创 2015-03-17 20:11:13 · 343 阅读 · 0 评论 -
[leetcode]Populating Next Right Pointers in Each Node
Given a binary tree struct TreeLinkNode { TreeLinkNode *left; TreeLinkNode *right; TreeLinkNode *next; }Populate each next pointer to point to its next right node. If there is原创 2015-03-18 11:38:44 · 361 阅读 · 0 评论 -
[leetcode]Symmetric Tree
Given a binary tree, check whether it is a mirror of itself (ie, symmetric around its center).DFS 对称的比较每个节点的值/** * Definition for binary tree * struct TreeNode { * int val; * TreeNode *lef原创 2015-03-17 19:22:06 · 443 阅读 · 0 评论 -
[leetcode]Unique Paths
A robot is located at the top-left corner of a m x n grid (marked ‘Start’ in the diagram below).The robot can only move either down or right at any point in time. The robot is trying to reach the botto原创 2015-03-17 19:26:25 · 391 阅读 · 0 评论 -
[leetcode]Triangle
Given a triangle, find the minimum path sum from top to bottom. Each step you may move to adjacent numbers on the row below.貌似想的有点复杂了,第一行一定只有一个元素class Solution {public: int minimumTotal(vector<vec原创 2015-03-17 19:35:38 · 386 阅读 · 0 评论 -
[leetcode]Majority Element
Given an array of size n, find the majority element. The majority element is the element that appears more than ⌊ n/2 ⌋ times.You may assume that the array is non-empty and the majority element always原创 2015-03-17 19:57:36 · 336 阅读 · 0 评论 -
[leetcode]Number of 1 Bits
Write a function that takes an unsigned integer and returns the number of ’1’ bits it has (also known as the Hamming weight).For example, the 32-bit integer ’11’ has binary representation 0000000000000原创 2015-03-17 20:06:23 · 390 阅读 · 0 评论 -
[leetcode]Populating Next Right Pointers in Each Node II
Follow up for problem “Populating Next Right Pointers in Each Node”.What if the given tree could be any binary tree? Would your previous solution still work?Note:You may only use constant extra space原创 2015-03-18 12:33:49 · 310 阅读 · 0 评论 -
[leetcode]Minimum Depth of Binary Tree
Given a binary tree, find its minimum depth.The minimum depth is the number of nodes along the shortest path from the root node down to the nearest leaf node.DFS/** * Definition for binary tree * str原创 2015-03-18 12:53:49 · 334 阅读 · 0 评论 -
[leetcode]Recover Binary Search Tree
Two elements of a binary search tree (BST) are swapped by mistake.Recover the tree without changing its structure.Note: A solution using O(n) space is pretty straight forward. Could you devise a const原创 2015-03-19 00:57:53 · 374 阅读 · 0 评论 -
[leetcode]Excel Sheet Column Title
Given a positive integer, return its corresponding column title as appear in an Excel sheet.class Solution {public: string convertToTitle(int n) { if(n<1) return ""; string s,str;原创 2015-03-17 19:49:10 · 339 阅读 · 0 评论 -
[leetcode]Sqrt(x)
Implement int sqrt(int x).Compute and return the square root of x.二分搜索class Solution {public: int sqrt(int x) { if(x==1||x==2||x==3) return 1; return binary(0,x,x); } int bi原创 2015-03-17 19:46:02 · 447 阅读 · 0 评论 -
[leetcode]Climbing Stairs
You are climbing a stair case. It takes n steps to reach to the top.Each time you can either climb 1 or 2 steps. In how many distinct ways can you climb to the top?DPclass Solution {public: int cl原创 2015-03-17 19:44:39 · 373 阅读 · 0 评论