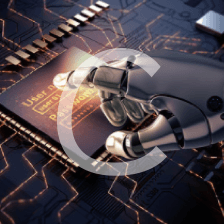
C++数据结构
Michael.Scofield
C++程序猿
展开
-
链表与数组的区别
二者都属于一种数据结构 从逻辑结构来看 数组必须事先定义固定的长度(元素个数),不能适应数据动态地增减的情况。当数据增加时,可能超出原先定义的元素个数;当数据减少时,造成内存浪费;数组可以根据下标直接存取。 链表动态地进行存储分配,可以适应数据动态地增减的情况,且可以方便地插入、删除数据项。(数组中插入、删除数据项时,需要移动其它数据项,非常繁琐)链表必须根据next指针找到下一个元素 从内存存储来看 (静态)数组从栈中分配空间, 对于程序员方便快速,但是自由度小 链表从堆中分配空间, 自由度大但是申请管原创 2021-05-07 11:13:20 · 152 阅读 · 0 评论 -
C++ 二分法
比较key与数组中间的值的大小,如果key大于中间值则在右半边继续以相同的方式查找,小于则在左半边继续查找。 int binary_search(vector<int>&arr,int key) { int n = arr.size(); int low = 0; int high = n-1; int mid = 0; while(low<high) { mid =floor((high+low)/2); if原创 2021-05-07 10:44:34 · 257 阅读 · 0 评论 -
map的find函数和count函数
1.find函数 find()函数返回被查找元素的迭代器,通过该迭代器访问该元素。 如果找不到则返回尾指针,即map.end() unordered_map<int,int>map = {{1,2},{3,4}}; auto it = map.find(1); cout<<it->second<<endl; //返回值为2 auto IT = map.count(1); cout<<IT<<en原创 2021-04-25 20:32:51 · 2086 阅读 · 0 评论 -
set容器的基本操作
#include "pch.h" #include <iostream> #include <string> using namespace std; //set mutiset 头文件 #include <set> //set容器特点:所有元素插入时候自动被排序 //set容器不允许插入重复值 set容器常用函数 使用时注意包含头文件<set> std::set and std::multiset associative containers /原创 2021-04-20 13:35:08 · 640 阅读 · 0 评论 -
vector容器的基本操作
#include "pch.h" #include <iostream> #include <vector> using namespace std; void test01() { /* 所谓动态增加大小,并不是在原空间之后续接新空间(因为无法保证原空间之后尚有可配置的空间),而是一块更大的内存空间,然后将原数据拷贝新空间,并释放原空间。因此,对vector的任何操作,一旦引起空间的重新配置,指向原vector的所有迭代器就都失效了。 */ vector<int&原创 2021-04-20 13:34:25 · 321 阅读 · 0 评论 -
二叉树的链表储存结构及实现
typedef int ElemType; struct NodeType { ElemType data; NodeType *lch; NodeType *rch; }; class BiTree { public: BiTree() { root = NULL; } ~BiTree() { destory(root); root = NULL; } void creat(); void preorder() { preorder(root); } void ino原创 2021-04-19 13:54:55 · 569 阅读 · 0 评论 -
队列的链表储存结构及实现
//队列的链表有附加头指针,指向队列的第一个数据结点的前一位置 typedef int Elemtype; struct quenode { Elemtype data; quenode *next; }; class LsQueue { private: quenode *front; quenode *rear; public: LsQueue(); ~LsQueue(); int IsEmpty(); //判断是否为空 void Display();原创 2021-04-19 13:50:04 · 241 阅读 · 0 评论 -
队列的顺序存储结构及实现
typedef int ElemType; //循环队列类 队尾入队 队顶出队 先进先出 //进队尾指针+1 出队头指针+1 //头指针位置始终是空的,进队时头指针位置不变,出队时尾指针位置不变。 class SeQueue { private: ElemType *elem; int front, rear; public: int MAXSIZE; SeQueue(int LengthInput) //初始化空队列 { elem = new ElemT.原创 2021-04-19 13:46:27 · 185 阅读 · 0 评论 -
栈的链表储存结构及实现
typedef int ElemType; struct Lsnode { ElemType data; Lsnode *next; }; class LsStack { private: Lsnode *top; public: LsStack() // 构造 { top = NULL; } ~LsStack() //析构 { SetEmpty(); } void SetEmpty(); //置空 int IsEmpty(); //判断 v原创 2021-04-19 13:39:30 · 269 阅读 · 0 评论 -
栈的顺序存储结构及实现
//栈的顺序储存结构 //顺序栈类的定义 typedef int ElemType; const int MAXSIZE = 100; class SqStack { private: ElemType elem[MAXSIZE]; int top; public: SqStack() { top = 0; } ~SqStack() { }; void SetEmpty() { top = 0; //置栈为空 } int IsEmpty(); //判断栈原创 2021-04-19 13:35:22 · 356 阅读 · 0 评论 -
线性表的链式储存结构及实现
typedef int ElemType; struct NodeType { ElemType data; //数据域 NodeType *next; //指针域 存放本结构体类型结点的首地址 }; class LinkList { private: NodeType *Head; public: LinkList(); //构造函数 ~LinkList(); //析构函数 void create(); //创建一个非空链表 voi原创 2021-04-19 13:28:26 · 190 阅读 · 0 评论 -
线性表的顺序储存结构及实现
typedef int ElemType; const int MAXSIZE = 100; class SqList { private: ElemType elem[MAXSIZE]; int length; public: SqList(void); ~SqList() { delete[]elem; }; void Create(); //创建一个顺序链表 void PrintOut(); //输出线性表 void原创 2021-04-19 13:22:09 · 153 阅读 · 0 评论