?题目描述
给定一个未排序的整数数组,找出最长连续序列的长度。
要求算法的时间复杂度为 O(n)。
示例:
输入: [100, 4, 200, 1, 3, 2]
输出: 4
解释: 最长连续序列是 [1, 2, 3, 4]。它的长度为 4。
? Method(C++): hash map
class Solution {
public:
int longestConsecutive(vector<int>& nums) {
unordered_set<int> h(nums.begin(), nums.end());
int ans = 0;
for (int num : nums)
if (!h.count(num - 1)) {
int next = num + 1;
while (h.count(next)) next++;
ans = max(ans, next - num);
}
return ans;
}
};
? Method(C++): hash map
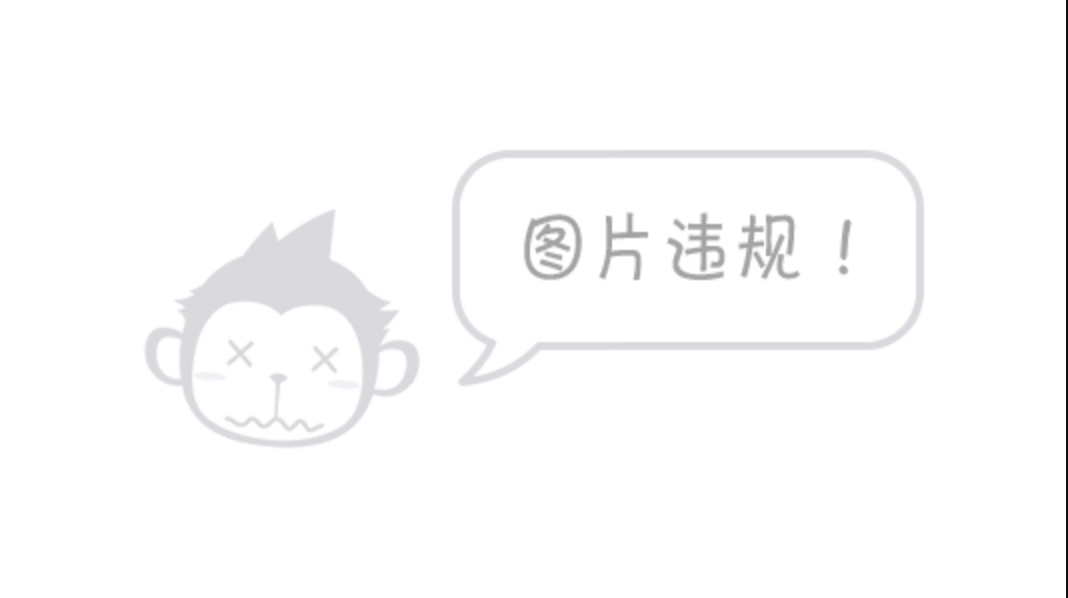
class Solution {
public:
int longestConsecutive(vector<int> &nums) {
unordered_map<int, int> h;
int ans = 0;
for (int num:nums) {
if (h.count(num)) continue;
auto it_l = h.find(num - 1); // 如果找到了比num小1的数,就返回它的指针
auto it_r = h.find(num + 1);
int l = (it_l == h.end()) ? 0 : it_l->second;
int r = (it_r == h.end()) ? 0 : it_r->second;
if (l > 0 && r > 0) {
h[num] = h[num - l] = h[num + r] = r + l + 1;
} else if (l > 0) {
h[num] = h[num - l] = l + 1;
} else if (r > 0) {
h[num] = h[num + r] = r + 1;
} else {
h[num] = 1;
}
}
for (const auto &kv:h) {
ans = max(ans, kv.second);
}
return ans;
}
};
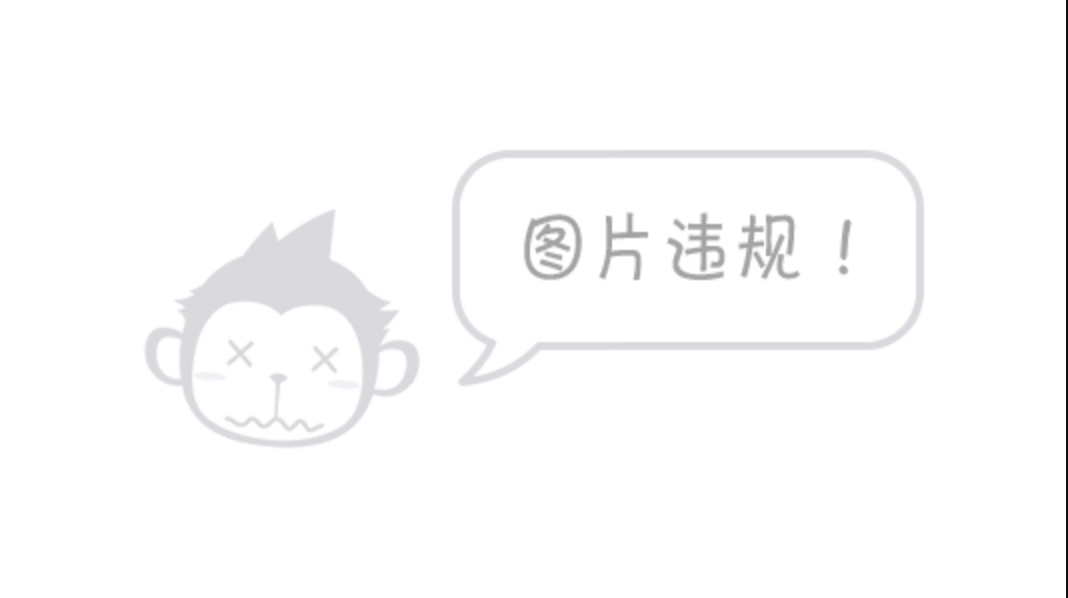