- 首先,创建节点类,并在节点中定义前序、中序、后序的相关方法
public class Node {
private int id;
private String name;
private Node left;
private Node right;
public Node(int id, String name) {
this.id = id;
this.name = name;
}
public void preOrder(){
System.out.println(this);
if (this.left != null){
this.left.preOrder();
}
if (this.right != null){
this.right.preOrder();
}
}
public void midOrder(){
if (this.left != null){
this.left.midOrder();
}
System.out.println(this);
if (this.right != null){
this.right.midOrder();
}
}
public void postOrder(){
if (this.left != null){
this.left.postOrder();
}
if (this.right != null){
this.right.postOrder();
}
System.out.println(this);
}
public Node preSearch(int no){
if (this.id == no){
return this;
}
Node current = null;
if (this.left != null){
current = this.left.preSearch(no);
}
if (current != null){
return current;
}
if (this.right != null){
current = this.right.preSearch(no);
}
return current;
}
public Node midSearch(int no){
Node current = null;
if (this.left != null){
current = this.left.midSearch(no);
}
if (current != null){
return current;
}
if (this.id == no){
return this;
}
if (this.right != null){
current = this.right.midSearch(no);
}
return current;
}
public Node postSearch(int no){
Node current = null;
if (this.left != null){
current = this.left.postSearch(no);
}
if (current != null){
return current;
}
if (this.right != null){
current = this.right.postSearch(no);
}
if (current != null){
return current;
}
if (this.id == no){
return this;
}
return current;
}
}
- 然后,创建二叉树类,定义根节点属性,也需要前序、中序、后序的相关方法
public class BinaryTree {
private Node root;
public BinaryTree(Node root) {
this.root = root;
}
public void preOrder(){
if (this.root != null){
this.root.preOrder();
}
else {
System.out.println("binary tree is empty");
}
}
public void midOrder(){
if (this.root != null){
this.root.midOrder();
}
else {
System.out.println("binary tree is empty");
}
}
public void postOrder(){
if (this.root != null){
this.root.postOrder();
}
else {
System.out.println("binary tree is empty");
}
}
public Node preSearch(int no){
if (this.root != null){
return this.root.preSearch(no);
}else {
return null;
}
}
public Node midSearch(int no){
if (this.root != null){
return this.root.midSearch(no);
}else {
return null;
}
}
public Node postSearch(int no){
if (this.root != null){
return this.root.postSearch(no);
}else {
return null;
}
}
}
public class BinaryTreeTest {
public static void main(String[] args) {
Node node1 = new Node(1,"one");
Node node2 = new Node(2,"two");
Node node3 = new Node(3,"three");
Node node4 = new Node(4,"four");
Node node5 = new Node(5,"five");
node1.setLeft(node2);
node1.setRight(node3);
node3.setRight(node5);
node3.setLeft(node4);
BinaryTree binaryTree = new BinaryTree(node1);
binaryTree.preOrder();
System.out.println("------------------------");
binaryTree.midOrder();
System.out.println("------------------------");
binaryTree.postOrder();
System.out.println("------------------------");
Node node = binaryTree.preSearch(4);
System.out.println(node);
}
}
- 二叉树
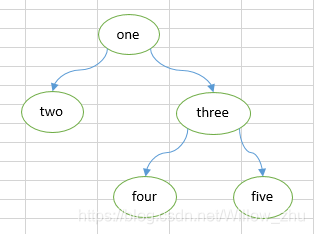
- 遍历结果
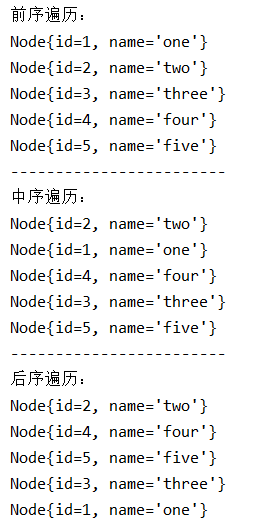