template<typename T>
class shared_ptr_my
{
public:
shared_ptr_my(T* p=nullptr);
shared_ptr_my(shared_ptr_my&);
shared_ptr_my& operator=(shared_ptr_my&);
shared_ptr_my(shared_ptr_my&&) noexcept;
shared_ptr_my& operator=(shared_ptr_my&&);
T* operator->();
T& operator*();
bool unique();
std::size_t use_count();
~shared_ptr_my();
private:
int* count;
T* p;
};
template<typename T>
shared_ptr_my<T>::shared_ptr_my(T* p):count(new int(1)),p(p)
{
cout << "构造函数 " << endl;
}
template<typename T>
shared_ptr_my<T>::~shared_ptr_my()
{
if (-- * count == 0) {
delete count;
delete p;
}
}
template<typename T>
shared_ptr_my<T>::shared_ptr_my(shared_ptr_my& other):p(other.p),count(&(++*other.count))
{
cout << "拷贝构造" << endl;
}
template<typename T>
T* shared_ptr_my<T>::operator->() {
return p;
}
template<typename T>
T& shared_ptr_my<T>::operator*() {
return *p;
}
template<typename T>
shared_ptr_my<T>& shared_ptr_my<T>::operator=(shared_ptr_my& other) {
++* other.count;
if (-- * count == 0) {
delete count;
delete p;
}
this->p = other.p;
this->count = other.count;
cout << "拷贝赋值 "<< endl;
return *this;
}
template<typename T>
shared_ptr_my<T>::shared_ptr_my(shared_ptr_my&& other)noexcept :p(std::move(other.p)),count(std::move(other.count)){
other.count = nullptr;
cout << "移动构造 " << endl;
}
template<typename T>
shared_ptr_my<T>& shared_ptr_my<T>::operator=(shared_ptr_my&& other) {
if (this == &other) {//防止自我赋值 判断this与other的地址
return *this;
}
p = other.p;
other.p = nullptr;
count = other.count;
other.count = nullptr;
cout << "移动赋值 " << endl;
return *this;
}
template<typename T>
std::size_t shared_ptr_my<T>::use_count() {
return *count;
}
template<typename T>
bool shared_ptr_my<T>::unique() {
return *count == 1;
}
int main()
{
shared_ptr_my<string> first(new string("first"));//构造
cout << first.use_count() << endl;
first = first;
cout << first.use_count() << endl;
first = std::move(first);
cout << first.use_count() << endl;
shared_ptr_my<string> second(first);//拷贝构造
cout << second.use_count() << endl;
shared_ptr_my<string> three(new string("three"));
cout << three.use_count() << endl;
three = second;//拷贝赋值
cout << three.use_count() << endl;
shared_ptr_my<string> four(std::move(three));//移动构造
cout << four.use_count() << endl;
shared_ptr_my<string> five(new string("five"));
cout << five.use_count() << endl;
five = std::move(four);//移动赋值
cout << five.use_count() << endl;
}
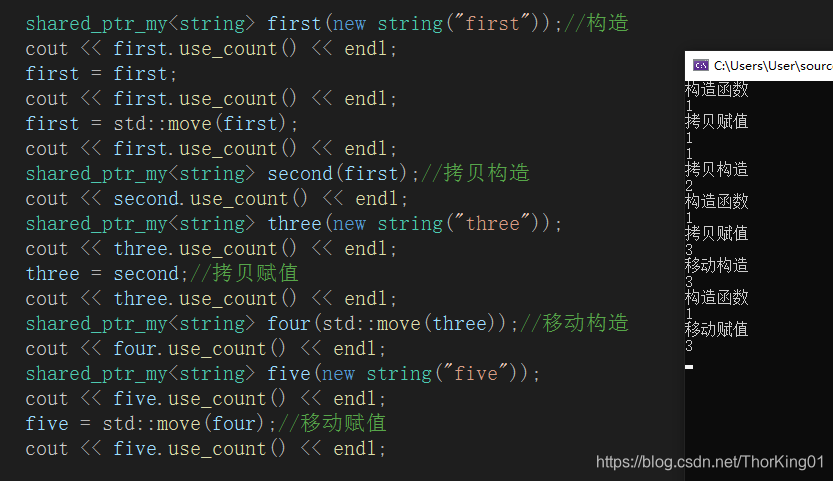