//MergeSort.h
#ifndef _MERGESORT_H_
#define _MERGESORT_H_
#include <stdlib.h>
#include <stdio.h>
#include <memory.h>
#include <time.h>
#define SIZE 15
typedef int Element;
typedef Element ListType[SIZE];
void CreateRandom(ListType List, int n);
void print(ListType List, int n);
//归并排序
void MergeSort(ListType List, int n);
#endif //_MERGESORT_H_
//MergeSort.c
#include "MergeSort.h"
void CreateRandom(ListType List, int n)
{
int i = 0;
srand((unsigned)time(NULL));
for (i = 0; i < n; ++i)
{
List[i] = rand() % 0x7F;
}
}
void print(ListType List, int n)
{
int i = 0;
for (i = 0; i < n; ++i)
{
printf("%d\t", List[i]);
}
printf("\n");
}
/***********************************************************
归并排序是利用分治思想,对一个无序序列进行不停的分割,直至分割成
n个子序列,再对每个子序列进行排序,排序完成后将所有有序子序列归并
成一个有序序列,排序完成
***********************************************************/
static void Merge(ListType List, ListType Tp, int Left, int Right)
{
int i = 0, Mid = 0, s1 = 0, s2 = 0;
for(i = Left; i <= Right; ++i) //将子数组的值赋值到辅助空间
{
Tp[i] = List[i];
}
Mid = (Left + Right) / 2; //找到辅助空间中元素的中间位置,将元素分为两份
s1 = Left;
s2 = Mid + 1;
while (s1 <= Mid && s2 <= Right) //比较辅助空间中的两份子数组,将较小的值放回
{
if( Tp[s1] >= Tp[s2] )
{
List[Left++] = Tp[s2++];
}
else
List[Left++] = Tp[s1++];
}
//放回剩余元素
while (s1 <= Mid)
List[Left++] = Tp[s1++];
while (s2 <= Right)
List[Left++] = Tp[s2++];
}
//分解无序数组
static void Resolve(ListType List, ListType Tp, int Left, int Right)
{
int Mid = 0;
if( Left >= Right )
return ;
Mid = (Left + Right) / 2;
Resolve(List, Tp, Left, Mid);
Resolve(List, Tp, Mid + 1, Right);
Merge(List, Tp, Left, Right);
}
void MergeSort(ListType List, int n)
{
ListType Tp; //辅助空间
memset(Tp, 0, sizeof(Tp));
Resolve(List, Tp, 0, n-1);
}
//main.c
#include "MergeSort.h"
int main(int argc, char **argv)
{
ListType List ={ 0 };
int n = sizeof(List) / sizeof(Element);
CreateRandom(List, n);
printf("排序前\n");
print(List, n);
MergeSort(List, n);
printf("排序后\n");
print(List, n);
system("pause");
return 0;
}
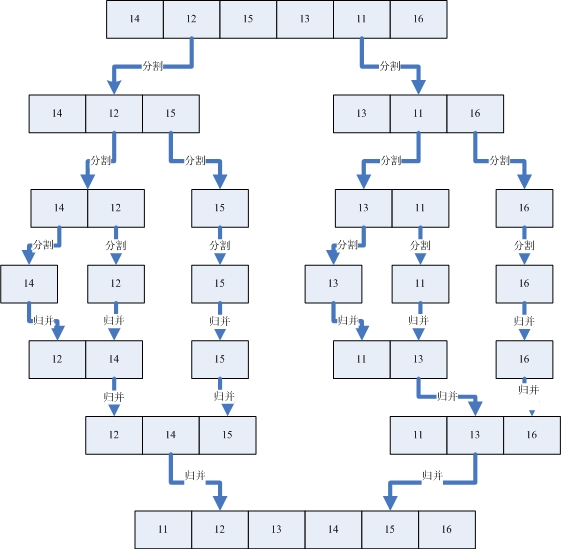