一、问题描述
基于角色的访问控制(Role-based access control, RBAC),是一种较新且广为使用的资源访问控制机制,本题是对该控制机制的简化。简化版的RBAC有用户和用户组两个核心概念,通过将用户添加到用户组中,并将所需的访问权限赋予用户组,从而实现快速灵活地对大量用户进行权限管理。
[基本要求]
(1)要求从文本文件中输入;
(2)用户信息包含以下内容:用户编号、姓名。用户组信息包含以下内容:用户组编号、用户组称呼、用户组职责。特别注意,用户组可以拥有不止一种权限;
(3)按照姓名查询,输出用户信息(包括其本人所属的所有用户组);
(4)显示某特定用户组中的所有用户;
(5)对某用户组添加用户;
(6)对某用户组删除用户;
(7)修改某用户或用户组的信息;
(8)两个用户组之间允许通过继承的方式共享用户和职责;
[继承举例]
系统中有“组长”和“组员”两个用户组。其中“组长”继承了“组员”的所有职责。将某用户加入到“组长”用户组中,在输出该用户所拥有的职责时,需要输出“组长”和“组员”的所有职责。同时输出“组员”用户组的成员时,也需要输出该用户。
二、解题思路:
1、这道题主要运用C++类的思想,虽然不难,但要实现的功能比较多,过程比较繁琐。首先,一个用户组设置一个类,这道题中,我们设置“组长”和“组员”两个类。我们在每个类中设置一个默认(缺省)的拷贝构造函数,在定义变量时就从文件中读取信息将其初始化。关于文件,我创建了MemberInformation.txt来保存“组员”组的信息;LeaderInformation.txt保存“组长”组的信息。
2、关于权限问题,相信不同的人会有不同的看法。这里,我认为“组长”和“组员”两个用户组的权限不同,相比于组员,组长拥有更多的权限。组长和组员均具有查询用户信息、显示组内所有用户信息、显示用户组信息(我自己增加的功能)的权限。但只有组长才具有添加、删除、修改用户(或用户组)信息的权限。
3、主要比较麻烦的地方在于对继承的考虑。虽然两个用户组的文件内容相对独立,但在初始化组员变量M时,其用户也包括组长(组长也是组员),所以也要读入“组长”组的用户信息。初始化组长变量L时,其职责也要包括组员的职责(组长除自己特殊的指责外,也有组员的职责),所以也要读入“组员”组的职责。以此类推,在添加、删除和修改时,都要注意可能需要同时对M和L两个变量进行调整。
附:文件内容
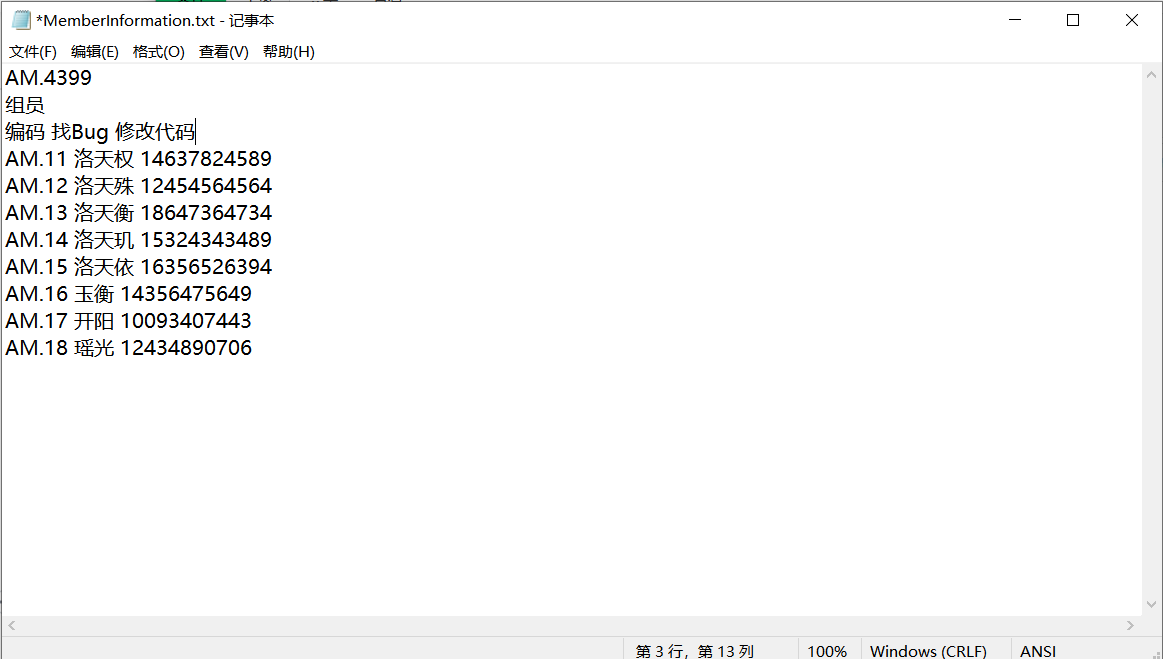
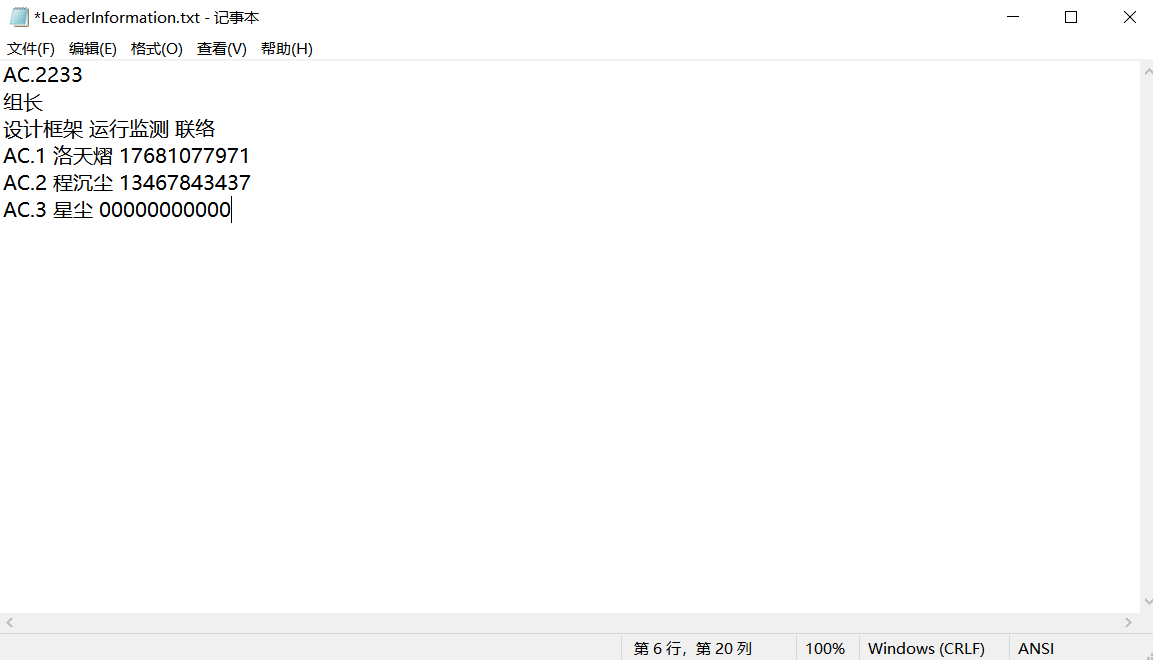
三、程序代码:
类的定义:
//保存人员信息的结构体
typedef struct PersonInformation {
char name[15]; //姓名
char phone[20]; //电话号码
char ID[20]; //用户编号
}PerIn;
//组员的类
class Member
{
private:
int Mnum; //用户数
PerIn user[maxp]; //用户信息
char num[20]; //用户组编号
char call[20]; //用户组称呼
char duty[maxn]; //用户组职责
public:
Member(); //缺省构造函数
int FindPerson(char* name1); //查询用户是否存在(若存在,返回用户序号)
void ShowPerson(int n); //显示某个用户的信息(n:用户序号)
void ShowPersons(); //显示组内所有用户的信息
void ShowGroup(); //显示“组员”组信息
void DeletePerson(int m, int n); //删除用户(m:是否从文件中删除 n:用户序号)
char* GetCall(); //返回用户组称呼
char* GetDuty(); //返回用户组职责
//添加用户(m:是否在文件中添加 n1:人员编号 n2:人员姓名 p:人员电话)
void InsertPerson(int m, char* n1, char* n2, char* p);
//修改用户信息(a:是否在文件中修改 m:修改的具体对象(编号、姓名或电话)n:修改人员的序号 s:修改后的结果)
void RevisePerson(int a, int m, int n, char* s);
//修改“组员”用户组信息(n:修改的具体对象(编号、称呼或职责) s:修改后的结果)
void ReviseGroup(int n, char* s);
};
//组长的类
class Leader
{
private:
int Lnum; //用户数
PerIn user[maxp]; //用户信息
char num[20]; //用户组编号
char call[20]; //用户组称呼
char duty[maxn]; //用户组职责
public:
Leader(); //缺省构造函数
int FindPerson(char* name1); //查询用户是否存在(若存在,返回用户序号)
void ShowPerson(int n); //显示某个用户的信息(n:用户序号)
void ShowPersons(); //显示组内所有用户的信息
void ShowGroup(); //显示“组长”组信息
void DeletePerson(int n); //删除用户(n:用户序号)
char* GetCall(); //返回用户组称呼
char* GetDuty(); //返回用户组职责
//添加用户(n1:人员编号 n2 : 人员姓名 p : 人员电话)
void InsertPerson(char* n1, char* n2, char* p);
//修改用户信息(m:修改的具体对象(编号、姓名或电话)n:修改人员的序号 s:修改后的结果)
void RevisePerson(int m, int n, char* s);
//修改“组长”用户组信息(m:是否修改文件 n:修改的具体对象(编号、姓名或电话) s:修改后的结果)
void ReviseGroup(int m, int n, char* s1, char* s2);
};
类中的函数:
//Member的缺省构造函数
Member::Member()
{
fstream file1, file2;
file1.open("MemberInformation.txt", ios::in);
if (file1.fail()) {
cout << "MemberInformation.txt打开失败" << endl;
exit(0);
}
//读取组长的人员信息(组长也是组员)
file2.open("LeaderInformation.txt", ios::in);
if (file2.fail()) {
cout << "LeaderInformation.txt打开失败" << endl;
exit(0);
}
char str[maxn];
int n = 0; //追踪MemberInformation.txt文件读取到了第几行
int t = 1;
//先读取“组员”组的全部信息
file1.getline(str, maxn);
while (t) {
if (file1.eof()) {
t = 0;
}
n++;
if (n == 1) {
//读取编号
strcpy(num, str);
}
else if (n == 2) {
//读取称呼
strcpy(call, str);
}
else if (n == 3) {
//读取职责
strcpy(duty, str);
}
else {
//读取成员(组员)
char mem[3][20]; //保存成员信息
int j1 = 0, k1 = 0;
for (int i = 0; i < strlen(str); i++) {
if (str[i] == ' ') {
mem[k1][j1] = '\0';
switch (k1)
{
case 0: strcpy(user[n - 3].ID, mem[k1]); break;
case 1: strcpy(user[n - 3].name, mem[k1]); break;
}
k1++;
j1 = 0;
}
else {
mem[k1][j1] = str[i];
j1++;
}
}
mem[k1][j1] = '\0';
strcpy(user[n - 3].phone, mem[k1]);
}
if (t) {
file1.getline(str, maxn);
}
}
//再读取“组长”组的成员信息
int m = 0; //追踪LeaderInformation.txt文件读取到了第几行
t = 1;
file2.getline(str, maxn);
while (t)
{
if (file2.eof()) {
t = 0;
}
m++;
if (m > 3) {
char lea[3][20];
int j2 = 0, k2 = 0;
for (int i = 0; i < strlen(str); i++) {
if (str[i] == ' ') {
lea[k2][j2] = '\0';
switch (k2)
{
case 0: strcpy(user[n + m - 6].ID, lea[k2]); break;
case 1: strcpy(user[n + m - 6].name, lea[k2]); break;
}
k2++;
j2 = 0;
}
else {
lea[k2][j2] = str[i];
j2++;
}
}
lea[k2][j2] = '\0';
strcpy(user[n + m - 6].phone, lea[k2]);
}
if (t) {
file2.getline(str, maxn);
}
}
Mnum = n + m - 6;
file1.close();
file2.close();
}
//Leader的缺省构造函数
Leader::Leader()
{
fstream file1, file2;
file1.open("LeaderInformation.txt", ios::in);
if (file1.fail()) {
cout << "LeaderInformation.txt打开失败" << endl;
exit(0);
}
//读取组员的职责信息(组员的职责也是组长的职责)
file2.open("MemberInformation.txt", ios::in);
if (file2.fail()) {
cout << "MemberInformation.txt打开失败" << endl;
exit(0);
}
//先读取“组长”组的全部信息
char str[maxn];
int n = 0; //追踪LeaderInformation.txt文件读取到了第几行
int t = 1;
file1.getline(str, maxn);
while (t) {
if (file1.eof()) {
t = 0;
}
n++;
if (n == 1) {
//读取编号
strcpy(num, str);
}
else if (n == 2) {
//读取称呼
strcpy(call, str);
}
else if (n == 3) {
//读取职责
strcpy(duty, str);
}
else {
//读取成员(组员)
char lea[3][20]; //保存成员信息
int j1 = 0, k1 = 0;
for (int i = 0; i < strlen(str); i++) {
if (str[i] == ' ')
{
lea[k1][j1] = '\0';
switch (k1)
{
case 0: strcpy(user[n - 3].ID, lea[k1]); break;
case 1: strcpy(user[n - 3].name, lea[k1]); break;
}
k1++;
j1 = 0;
}
else {
lea[k1][j1] = str[i];
j1++;
}
}
lea[k1][j1] = '\0';
strcpy(user[n - 3].phone, lea[k1]);
}
if (t) {
file1.getline(str, maxn);
}
}
//再读取“组员”组的职责信息
int m = 0; //追踪MemberInformation.txt文件读取到了第几行
t = 1;
file2.getline(str, maxn);
while (t) {
if (file2.eof()) {
t = 0;
}
m++;
if (m == 3) {
strcat(duty, " ");
strcat(duty, str);
break;
}
if (t) {
file2.getline(str, maxn);
}
}
Lnum = n - 3;
file1.close();
file2.close();
}
//Member查询用户是否存在(若存在,返回用户序号)
int Member::FindPerson(char* name1)
{
for (int i = 1; i <= Mnum; i++) {
if (strcmp(user[i].name, name1) == 0) {
return i;
}
}
return 0;
}
//Leader查询用户是否存在(若存在,返回用户序号)
int Leader::FindPerson(char* name1)
{
for (int i = 1; i <= Lnum; i++) {
if (strcmp(user[i].name, name1) == 0) {
return i;
}
}
return 0;
}
//Member显示某个用户的信息(n:用户序号)
void Member::ShowPerson(int n)
{
cout << "\n\t\t用户信息已导出\n";
cout << "\t\t编号:" << user[n].ID << endl;
cout << "\t\t姓名:" << user[n].name << endl;
cout << "\t\t联系电话:" << user[n].phone << endl;
}
//Leader显示某个用户的信息(n:用户序号)
void Leader::ShowPerson(int n)
{
cout << "\n\t\t用户信息已导出\n";
cout << "\t\t编号:" << user[n].ID << endl;
cout << "\t\t姓名:" << user[n].name << endl;
cout << "\t\t联系电话:" << user[n].phone << endl;
}
//Member返回用户组称呼
char* Member::GetCall()
{
return call;
}
//Leader返回用户组称呼
char* Leader::GetCall()
{
return call;
}
//Member返回用户组职责
char* Member::GetDuty()
{
return duty;
}
//Leader返回用户组职责
char* Leader::GetDuty()
{
return duty;
}
//Member显示组内所有用户的信息
void Member::ShowPersons()
{
cout << "\n\t\t组员信息已全部导出\n";
for (int i = 1; i <= Mnum; i++) {
cout << "\t\t编号:" << user[i].ID << "\t姓名:" << user[i].name << "\t电话:" << user[i].phone << endl;
}
cout << "\t\t信息导出完毕\n";
}
//Leader显示组内所有用户的信息
void Leader::ShowPersons()
{
cout << "\n\t\t组长信息已全部导出\n";
for (int i = 1; i <= Lnum; i++) {
cout << "\t\t编号:" << user[i].ID << "\t姓名:" << user[i].name << "\t电话:" << user[i].phone << endl;
}
cout << "\t\t信息导出完毕\n";
}
//Member显示“组员”组信息
void Member::ShowGroup()
{
cout << "\n\t\t用户组信息已导出\n";
cout << "\t\t编号:" << num << endl;
cout << "\t\t名称:" << call << endl;
cout << "\t\t职责:" << duty << endl;
cout << "\t\t信息导出完毕\n";
}
//Leader显示“组长”组信息
void Leader::ShowGroup()
{
cout << "\n\t\t用户组信息已导出\n";
cout << "\t\t编号:" << num << endl;
cout << "\t\t名称:" << call << endl;
cout << "\t\t职责:" << duty << endl;
cout << "\t\t信息导出完毕\n";
}
//Member删除用户(m:是否从文件中删除 n:用户序号)
void Member::DeletePerson(int m, int n)
{
//将用户在程序中删除
for (int i = n; i < Mnum; i++) {
strcpy(user[i].ID, user[i + 1].ID);
strcpy(user[i].name, user[i + 1].name);
strcpy(user[i].phone, user[i + 1].phone);
}
Mnum--;
if (m != 1) {
return;
}
//将用户从文件中删除
fstream file, file1;
file.open("MemberInformation.txt", ios::in);
if (file.fail()) {
cout << "MemberInformation.txt打开失败" << endl;
exit(0);
}
file1.open("EmptyFile.dat", ios::out);
if (file1.fail()) {
cout << "EmptyFile.dat打开失败" << endl;
exit(0);
}
int m1 = 1; //保存读到文件中的第几行
char str[maxn];
file.getline(str, maxn);
while (!file.eof()) {
if (m1 == n + 3) {
file.getline(str, maxn);
}
else {
if (m1 > 1) {
file1 << '\n';
}
file1 << str;
file.getline(str, maxn);
}
m1++;
}
if (m1 != n + 3) {
file1 << '\n' << str; //最后一行之后不能读入换行符
}
file.close();
file1.close();
file.open("MemberInformation.txt", ios::out);
if (file.fail()) {
cout << "MemberInformation.txt打开失败" << endl;
exit(0);
}
file1.open("EmptyFile.dat", ios::in);
if (file1.fail()) {
cout << "EmptyFile.dat打开失败" << endl;
exit(0);
}
file1.getline(str, maxn);
while (!file1.eof()) {
file << str << '\n';
file1.getline(str, maxn);
}
file << str; //最后一行之后不能读入换行符
file.close();
file1.close();
}
//Leader删除用户(n:用户序号)
void Leader::DeletePerson(int n)
{
//将用户在程序中删除
for (int i = n; i < Lnum; i++) {
strcpy(user[i].ID, user[i + 1].ID);
strcpy(user[i].name, user[i + 1].name);
strcpy(user[i].phone, user[i + 1].phone);
}
Lnum--;
//将用户从文件中删除
fstream file, file1;
file.open("LeaderInformation.txt", ios::in);
if (file.fail()) {
cout << "LeaderInformation.txt打开失败" << endl;
exit(0);
}
file1.open("EmptyFile.dat", ios::out);
if (file1.fail()) {
cout << "EmptyFile.dat打开失败" << endl;
exit(0);
}
int m1 = 1; //保存读到文件中的第几行
char str[maxn];
file.getline(str, maxn);
while (!file.eof()) {
if (m1 == n + 3) {
file.getline(str, maxn);
}
else {
if (m1 > 1) {
file1 << '\n';
}
file1 << str;
file.getline(str, maxn);
}
m1++;
}
if (m1 != n + 3) {
file1 << '\n' << str; //最后一行之后不能读入换行符
}
file.close();
file1.close();
file.open("LeaderInformation.txt", ios::out);
if (file.fail()) {
cout << "LeaderInformation.txt打开失败" << endl;
exit(0);
}
file1.open("EmptyFile.dat", ios::in);
if (file1.fail()) {
cout << "EmptyFile.dat打开失败" << endl;
exit(0);
}
file1.getline(str, maxn);
while (!file1.eof()) {
file << str << '\n';
file1.getline(str, maxn);
}
file << str; //最后一行之后不能读入换行符
file.close();
file1.close();
}
//Member添加用户(m:是否在文件中添加 n1:人员编号 n2:人员姓名 p:人员电话)
void Member::InsertPerson(int m, char* n1, char* n2, char* p)
{
//将用户在程序中添加
strcpy(user[Mnum + 1].ID, n1);
strcpy(user[Mnum + 1].name, n2);
strcpy(user[Mnum + 1].phone, p);
Mnum++; //人员加1
if (m != 1) {
return;
}
//将用户添加到文件中
string str1;
char str2[maxn];
str1.append(n1);
str1.append(1, ' ');
str1.append(n2);
str1.append(1, ' ');
str1.append(p);
int i;
for (i = 0; i < str1.length(); i++) {
str2[i] = str1[i];
}
str2[i] = '\0';
fstream file;
file.open("MemberInformation.txt", ios::out | ios::app);
if (file.fail()) {
cout << "MemberInformation.txt打开失败" << endl;
exit(0);
}
file.seekg(0L, ios::end); //添加到文件尾
file << '\n' << str2;
file.close();
}
//Leader添加用户(n1:人员编号 n2:人员姓名 p:人员电话)
void Leader::InsertPerson(char* n1, char* n2, char* p)
{
//将用户在程序中添加
strcpy(user[Lnum + 1].ID, n1);
strcpy(user[Lnum + 1].name, n2);
strcpy(user[Lnum + 1].phone, p);
Lnum++;
//将用户添加到文件中
string str1;
char str2[maxn];
str1.append(n1);
str1.append(1, ' ');
str1.append(n2);
str1.append(1, ' ');
str1.append(p);
int i;
for (i = 0; i < str1.length(); i++) {
str2[i] = str1[i];
}
str2[i] = '\0';
fstream file;
file.open("LeaderInformation.txt", ios::out | ios::app);
if (file.fail()) {
cout << "LeaderInformation.txt打开失败" << endl;
exit(0);
}
file.seekg(0L, ios::end); //添加到文件尾
file << '\n' << str2;
file.close();
}
//Member修改用户信息
//(a:是否在文件中修改 m:修改的具体对象(编号、姓名或电话)n:修改人员的序号 s:修改后的结果)
void Member::RevisePerson(int a, int m, int n, char* s)
{
//在程序中修改用户信息
switch (m)
{
case 1: strcpy(user[n].ID, s); break;
case 2: strcpy(user[n].name, s); break;
case 3: strcpy(user[n].phone, s); break;
}
if (a != 1) {
return;
}
//将修改后的用户信息保存在文件中
string str1;
char str2[maxn];
str1.append(user[n].ID);
str1.append(1, ' ');
str1.append(user[n].name);
str1.append(1, ' ');
str1.append(user[n].phone);
int i;
for (i = 0; i < str1.length(); i++) {
str2[i] = str1[i];
}
str2[i] = '\0';
fstream file, file1;
file.open("MemberInformation.txt", ios::in);
if (file.fail()) {
cout << "MemberInformation.txt打开失败" << endl;
exit(0);
}
file1.open("EmptyFile.dat", ios::out);
if (file1.fail()) {
cout << "EmptyFile.dat打开失败" << endl;
exit(0);
}
int m1 = 1; //保存读到文件中的第几行
char str[maxn];
file.getline(str, maxn);
while (!file.eof())
{
if (m1 > 1) {
file1 << '\n';
}
if (m1 == n + 3) {
file1 << str2;
}
else {
file1 << str;
}
file.getline(str, maxn);
m1++;
}
if (m1 == n + 3) {
file1 << '\n' << str2; //最后一行之后不能读入换行符
}
else {
file1 << '\n' << str; //最后一行之后不能读入换行符
}
file.close();
file1.close();
file.open("MemberInformation.txt", ios::out);
if (file.fail()) {
cout << "MemberInformation.txt打开失败" << endl;
exit(0);
}
file1.open("EmptyFile.dat", ios::in);
if (file1.fail()) {
cout << "EmptyFile.dat打开失败" << endl;
exit(0);
}
file1.getline(str, maxn);
while (!file1.eof()) {
file << str << '\n';
file1.getline(str, maxn);
}
file << str;
file.close();
file1.close();
}
//Leader修改用户信息(m:修改的具体对象(编号、姓名或电话)n:修改人员的序号 s:修改后的结果)
void Leader::RevisePerson(int m, int n, char* s)
{
//在程序中修改用户信息
switch (m)
{
case 1: strcpy(user[n].ID, s); break;
case 2: strcpy(user[n].name, s); break;
case 3: strcpy(user[n].phone, s); break;
}
//将修改后的用户信息保存在文件中
string str1;
char str2[maxn];
str1.append(user[n].ID);
str1.append(1, ' ');
str1.append(user[n].name);
str1.append(1, ' ');
str1.append(user[n].phone);
int i;
for (i = 0; i < str1.length(); i++) {
str2[i] = str1[i];
}
str2[i] = '\0';
fstream file, file1;
file.open("LeaderInformation.txt", ios::in);
if (file.fail()) {
cout << "LeaderInformation.txt打开失败" << endl;
exit(0);
}
file1.open("EmptyFile.dat", ios::out);
if (file1.fail()) {
cout << "EmptyFile.dat打开失败" << endl;
exit(0);
}
int m1 = 1; //保存读到文件中的第几行
char str[maxn];
file.getline(str, maxn);
while (!file.eof()) {
if (m1 > 1) {
file1 << '\n';
}
if (m1 == n + 3) {
file1 << str2;
}
else {
file1 << str;
}
file.getline(str, maxn);
m1++;
}
if (m1 == n + 3) {
file1 << '\n' << str2; //最后一行之后不能读入换行符
}
else {
file1 << '\n' << str; //最后一行之后不能读入换行符
}
file.close();
file1.close();
file.open("LeaderInformation.txt", ios::out);
if (file.fail()) {
cout << "LeaderInformation.txt打开失败" << endl;
exit(0);
}
file1.open("EmptyFile.dat", ios::in);
if (file1.fail()) {
cout << "EmptyFile.dat打开失败" << endl;
exit(0);
}
file1.getline(str, maxn);
while (!file1.eof()) {
file << str << '\n';
file1.getline(str, maxn);
}
file << str;
file.close();
file1.close();
}
//Member修改“组员”用户组信息(n:修改的具体对象(编号、称呼或职责) s:修改后的结果)
void Member::ReviseGroup(int n, char* s)
{
//在程序中修改
switch (n)
{
case 1: strcpy(num, s); break;
case 2: strcpy(call, s); break;
case 3: strcpy(duty, s); break;
}
//将修改后的用户信息保存在文件中
fstream file, file1;
file.open("MemberInformation.txt", ios::in);
if (file.fail()) {
cout << "MemberInformation.txt打开失败" << endl;
exit(0);
}
file1.open("EmptyFile.dat", ios::out);
if (file1.fail()) {
cout << "EmptyFile.dat打开失败" << endl;
exit(0);
}
int m1 = 1; //保存读到文件中的第几行
char str[maxn];
file.getline(str, maxn);
while (!file.eof()) {
if (m1 == n) {
file1 << s << '\n';
}
else {
file1 << str << '\n';
}
file.getline(str, maxn);
m1++;
}
file1 << str; //最后一行之后不能读入换行符
file.close();
file1.close();
file.open("MemberInformation.txt", ios::out);
if (file.fail()) {
cout << "MemberInformation.txt打开失败" << endl;
exit(0);
}
file1.open("EmptyFile.dat", ios::in);
if (file1.fail()) {
cout << "EmptyFile.dat打开失败" << endl;
exit(0);
}
file1.getline(str, maxn);
while (!file1.eof()) {
file << str << '\n';
file1.getline(str, maxn);
}
file << str;
file.close();
file1.close();
}
//Leader修改“组长”用户组信息(m:是否修改文件 n:修改的具体对象(编号、称呼或专有职责)
//s1:组长的职责或其他修改后的结果 s2:组员的职责)
void Leader::ReviseGroup(int m, int n, char* s1, char* s2)
{
char s[maxn];
strcpy(s, s1);
//在程序中修改
if (n == 3) {
//如果修改的是职责
strcat(s1, " ");
strcat(s1, s2);
strcpy(duty, s1);
}
else {
switch (n)
{
case 1: strcpy(num, s1); break;
case 2: strcpy(call, s1); break;
}
}
if (m != 1) {
return;
}
//将修改后的用户信息保存在文件中
fstream file, file1;
file.open("LeaderInformation.txt", ios::in);
if (file.fail()) {
cout << "LeaderInformation.txt打开失败" << endl;
exit(0);
}
file1.open("EmptyFile.dat", ios::out);
if (file1.fail()) {
cout << "EmptyFile.dat打开失败" << endl;
exit(0);
}
int m1 = 1; //保存读到文件中的第几行
char str[maxn];
file.getline(str, maxn);
while (!file.eof()) {
if (m1 == n) {
file1 << s << '\n';
}
else {
file1 << str << '\n';
}
file.getline(str, maxn);
m1++;
}
file1 << str; //最后一行之后不能读入换行符
file.close();
file1.close();
file.open("LeaderInformation.txt", ios::out);
if (file.fail()) {
cout << "LeaderInformation.txt打开失败" << endl;
exit(0);
}
file1.open("EmptyFile.dat", ios::in);
if (file1.fail()) {
cout << "EmptyFile.dat打开失败" << endl;
exit(0);
}
file1.getline(str, maxn);
while (!file1.eof()) {
file << str << '\n';
file1.getline(str, maxn);
}
file << str;
file.close();
file1.close();
}
主函数:
# include <iostream>
# include <stdlib.h>
# include <fstream>
# include <string.h>
# include <string>
# include <stdlib.h>
# include <stdio.h>
# define maxp 15
# define maxn 100
void Frame1(); //大框架函数
void Frame2(); //小框架函数
void Menu_Member(); //组员专用菜单
void Menu_Leader(); //组长专用菜单
void Search(); //查询用户信息
void ShowPeople(); //显示组内用户信息
void ShowGroup(); //显示用户组信息
void Insert(); //添加用户信息
void Delete(); //删除用户信息
void Change(); //修改用户信息
Member M; //组员组
Leader L; //组长组
int main()
{
int select;
int identity; //用户身份变量
Frame1();
cout << "\n--------------------------------------------------- ";
cout << "欢迎使用RBAC系统";
cout << " -------------------------------------------------- \n";
Frame1();
cout << '\n';
//总的程序下,可以多次进行不同人员的操作
while (1) {
cout << "\n\t\t您的用户身份: (1)组员 (2)组长\n";
cout << "\t\t请选择:";
cin >> identity;
cout << endl;
Frame2();
cout << "\t\t** 欢迎来到用户界面 **\n";
Frame2();
if (identity == 1) {
//组员部分
int quit1 = 0;
while (1) {
int select1;
Menu_Member(); //组员专用菜单
cout << "\t\t请选择:";
cin >> select1;
switch (select1)
{
case 1: Search(); break; //查询用户信息
case 2: ShowPeople(); break; //显示用户组所有用户
case 3: ShowGroup(); break; //显示用户组信息
case 4: quit1 = 1; break; //退出
}
if (quit1) {
break;
}
}
}
else if (identity == 2) {
//组长部分
int quit2 = 0;
while (1) {
int select2;
Menu_Leader(); //组长专用菜单
cout << "\t\t请选择:";
cin >> select2;
switch (select2)
{
case 1: Search(); break; //查询用户信息
case 2: ShowPeople(); break; //显示用户组所有用户
case 3: ShowGroup(); break; //显示用户组信息
case 4: Insert(); break; //添加用户信息
case 5: Delete(); break; //删除用户信息
case 6: Change(); break; //修改用户信息
case 7: quit2 = 1; break; //退出
}
if (quit2) {
break;
}
}
}
cout << "\n\t\t是否退出程序: (1)继续运行\t(其他任意键)退出\n";
cout << "\t\t请选择:";
cin >> select;
if (select != 1) {
cout << "\n\t\t程序运行结束\n";
break;
}
}
return 0;
}
主体函数:
void Frame1()
{
for (int i = 0; i < 120; i++) {
cout << "*";
}
cout << "\n";
}
void Frame2()
{
cout << "\t\t";
for (int i = 0; i < 23; i++) {
cout << "*";
}
cout << "\n";
}
//组员专用菜单
void Menu_Member()
{
cout << "\n\t\t*******************************\n";
cout << "\t\t**(1)、查询用户 *************\n";
cout << "\t\t**(2)、显示组内用户信息 *****\n";
cout << "\t\t**(3)、显示用户组信息 *******\n";
cout << "\t\t**(4)、退出 *****************\n";
cout << "\t\t*******************************\n";
}
//组长专用菜单
void Menu_Leader()
{
cout << "\n\t\t*******************************\n";
cout << "\t\t**(1)、查询用户 *************\n";
cout << "\t\t**(2)、显示组内用户信息 *****\n";
cout << "\t\t**(3)、显示用户组信息 *******\n";
cout << "\t\t**(4)、添加用户 *************\n";
cout << "\t\t**(5)、删除用户 *************\n";
cout << "\t\t**(6)、修改信息 *************\n";
cout << "\t\t**(7)、退出 *****************\n";
cout << "\t\t*******************************\n";
}
//查询用户信息
void Search()
{
char name1[15];
int t = 1;
while (t) {
cout << "\n\t\t请输入要查询的用户姓名:";
cin >> name1;
int a, b;
a = L.FindPerson(name1);
b = M.FindPerson(name1);
//先判断是否为组长
if (a) {
//是组长
L.ShowPerson(a);
cout << "\t\t所属用户组:" << L.GetCall() << " " << M.GetCall() << endl;
cout << "\t\t信息导出完毕\n";
}
else if (b) {
//是组员
M.ShowPerson(b);
cout << "\t\t所属用户组:" << M.GetCall() << endl;
cout << "\t\t信息导出完毕\n";
}
else {
cout << "\t\t查无此人" << endl;
}
cout << "\n\t\t是否继续查询:(1)是 (2)否\n";
cout << "\t\t请选择:";
cin >> t;
if (t != 1) {
break;
}
}
}
//显示组内用户信息
void ShowPeople()
{
int select;
cout << "\n\t\t用户组:(1)组员 (2)组长" << endl;
cout << "\t\t请选择:";
cin >> select;
if (select == 1) {
//组员组
M.ShowPersons();
}
else {
//组长组
L.ShowPersons();
}
}
//显示用户组信息
void ShowGroup()
{
int select;
cout << "\n\t\t用户组:(1)组员 (2)组长" << endl;
cout << "\t\t请选择:";
cin >> select;
if (select == 1) {
//组员组
M.ShowGroup();
}
else {
//组长组
L.ShowGroup();
}
}
//添加用户信息
void Insert()
{
char name1[15], num1[20], phone1[20];
int t = 1;
while (t) {
int select;
cout << "\n\t\t用户所属的组:(1)组员(2)组长" << endl;
cout << "\t\t请选择:";
cin >> select;
cout << "\t\t请输入要添加的用户编号:";
cin >> num1;
cout << "\t\t请输入要添加的用户姓名:";
cin >> name1;
cout << "\t\t请输入要添加的用户联系电话:";
cin >> phone1;
if (select == 1) {
//添加组员
M.InsertPerson(1, num1, name1, phone1);
}
else {
//添加组长
L.InsertPerson(num1, name1, phone1);
M.InsertPerson(2, num1, name1, phone1); //无需添加到“组员”组文件中
}
cout << "\n\t\t是否继续添加:(1)是 (2)否\n";
cout << "\t\t请选择:";
cin >> t;
if (t != 1) {
break;
}
}
}
//删除用户信息
void Delete()
{
char name1[15];
int t = 1;
while (t) {
int select;
cout << "\n\t\t用户所属的组:(1)组员(2)组长" << endl;
cout << "\t\t请选择:";
cin >> select;
cout << "\n\t\t请输入要删除的用户姓名:";
cin >> name1;
int a, b;
if (select == 1) {
//删除组员
a = M.FindPerson(name1);
if (!a) {
cout << "\t\t查无此人" << endl; //该人不存在
}
else {
M.DeletePerson(1, a);
}
}
else
{
//删除组长
b = L.FindPerson(name1);
if (!b) {
cout << "\t\t查无此人" << endl; //该人不存在
}
else {
a = M.FindPerson(name1);
M.DeletePerson(2, a); //无需从文件中删除
L.DeletePerson(b);
}
}
cout << "\n\t\t是否继续删除:(1)是 (2)否\n";
cout << "\t\t请选择:";
cin >> t;
if (t != 1) {
break;
}
}
}
//修改用户或用户组信息
void Change()
{
int t = 1;
while (t) {
int select1, select2, select3, select4;
char str[maxn], name1[15];
int a, b;
cout << "\n\t\t所属的组:(1)组员(2)组长" << endl;
cout << "\t\t请选择:";
cin >> select1;
cout << "\n\t\t要修改的对象:(1)用户(2)用户组" << endl;
cout << "\t\t请选择:";
cin >> select2;
if (select1 == 1) {
//组员
if (select2 == 1) {
cout << "\n\t\t请输入要修改信息的人员姓名:";
cin >> name1;
a = M.FindPerson(name1);
if (!a) {
cout << "\t\t查无此人" << endl;
}
else {
cout << "\n\t\t要修改的项目:(1)编号(2)姓名(3)电话" << endl;
cout << "\t\t请选择:";
cin >> select3;
cout << "\n\t\t请输入修改后的结果:";
cin >> str;
M.RevisePerson(1, select3, a, str);
}
}
else {
cout << "\n\t\t要修改的项目:(1)编号(2)称呼(3)职责" << endl;
cout << "\t\t请选择:";
cin >> select4;
cin.ignore(); //过滤换行符
cout << "\n\t\t请输入修改后的结果:";
cin.getline(str, maxn);
if (select4 == 3) {
int m = 1;
char str1[maxn];
fstream file;
file.open("LeaderInformation.txt", ios::in);
if (file.fail()) {
cout << "LeaderInformation.txt打开失败" << endl;
exit(0);
}
file.getline(str1, maxn);
while (!file.eof()) {
if (m == 3)
{
break;
}
file.getline(str1, maxn);
m++;
}
file.close();
//组员的职责发生改变,组长的职责也会随之变化
L.ReviseGroup(2, select4, str1, str);
}
M.ReviseGroup(select4, str);
}
}
else {
if (select2 == 1) {
//组长
cout << "\n\t\t请输入要修改信息的人员姓名:";
cin >> name1;
b = L.FindPerson(name1);
if (!b) {
cout << "\t\t查无此人" << endl;
}
else {
a = M.FindPerson(name1);
cout << "\n\t\t要修改的项目:(1)编号(2)姓名(3)电话" << endl;
cout << "\t\t请选择:";
cin >> select3;
cout << "\n\t\t请输入修改后的结果:";
cin >> str;
M.RevisePerson(2, select3, a, str);
L.RevisePerson(select3, b, str);
}
}
else {
cout << "\n\t\t要修改的项目:(1)编号(2)称呼(3)专有职责" << endl;
cout << "\t\t请选择:";
cin >> select4;
cin.ignore(); //过滤换行符
cout << "\n\t\t请输入修改后的结果:";
cin.getline(str, maxn);
char str2[maxn];
strcpy(str2, M.GetDuty());
L.ReviseGroup(1, select4, str, str2);
}
}
cout << "\n\t\t是否继续修改:(1)是 (2)否\n";
cout << "\t\t请选择:";
cin >> t;
if (t != 1) {
break;
}
}
}
全部代码:
# include <iostream>
# include <stdlib.h>
# include <fstream>
# include <string.h>
# include <string>
# include <stdlib.h>
# include <stdio.h>
# define maxp 15
# define maxn 100
using namespace std;
//保存人员信息的结构体
typedef struct PersonInformation {
char name[15]; //姓名
char phone[20]; //电话号码
char ID[20]; //用户编号
}PerIn;
//组员的类
class Member
{
private:
int Mnum; //用户数
PerIn user[maxp]; //用户信息
char num[20]; //用户组编号
char call[20]; //用户组称呼
char duty[maxn]; //用户组职责
public:
Member(); //缺省构造函数
int FindPerson(char* name1); //查询用户是否存在(若存在,返回用户序号)
void ShowPerson(int n); //显示某个用户的信息(n:用户序号)
void ShowPersons(); //显示组内所有用户的信息
void ShowGroup(); //显示“组员”组信息
void DeletePerson(int m, int n); //删除用户(m:是否从文件中删除 n:用户序号)
char* GetCall(); //返回用户组称呼
char* GetDuty(); //返回用户组职责
//添加用户(m:是否在文件中添加 n1:人员编号 n2:人员姓名 p:人员电话)
void InsertPerson(int m, char* n1, char* n2, char* p);
//修改用户信息(a:是否在文件中修改 m:修改的具体对象(编号、姓名或电话)n:修改人员的序号 s:修改后的结果)
void RevisePerson(int a, int m, int n, char* s);
//修改“组员”用户组信息(n:修改的具体对象(编号、称呼或职责) s:修改后的结果)
void ReviseGroup(int n, char* s);
};
//组长的类
class Leader
{
private:
int Lnum; //用户数
PerIn user[maxp]; //用户信息
char num[20]; //用户组编号
char call[20]; //用户组称呼
char duty[maxn]; //用户组职责
public:
Leader(); //缺省构造函数
int FindPerson(char* name1); //查询用户是否存在(若存在,返回用户序号)
void ShowPerson(int n); //显示某个用户的信息(n:用户序号)
void ShowPersons(); //显示组内所有用户的信息
void ShowGroup(); //显示“组长”组信息
void DeletePerson(int n); //删除用户(n:用户序号)
char* GetCall(); //返回用户组称呼
char* GetDuty(); //返回用户组职责
//添加用户(n1:人员编号 n2 : 人员姓名 p : 人员电话)
void InsertPerson(char* n1, char* n2, char* p);
//修改用户信息(m:修改的具体对象(编号、姓名或电话)n:修改人员的序号 s:修改后的结果)
void RevisePerson(int m, int n, char* s);
//修改“组长”用户组信息(m:是否修改文件 n:修改的具体对象(编号、姓名或电话) s:修改后的结果)
void ReviseGroup(int m, int n, char* s1, char* s2);
};
//Member的缺省构造函数
Member::Member()
{
fstream file1, file2;
file1.open("MemberInformation.txt", ios::in);
if (file1.fail()) {
cout << "MemberInformation.txt打开失败" << endl;
exit(0);
}
//读取组长的人员信息(组长也是组员)
file2.open("LeaderInformation.txt", ios::in);
if (file2.fail()) {
cout << "LeaderInformation.txt打开失败" << endl;
exit(0);
}
char str[maxn];
int n = 0; //追踪MemberInformation.txt文件读取到了第几行
int t = 1;
//先读取“组员”组的全部信息
file1.getline(str, maxn);
while (t) {
if (file1.eof()) {
t = 0;
}
n++;
if (n == 1) {
//读取编号
strcpy(num, str);
}
else if (n == 2) {
//读取称呼
strcpy(call, str);
}
else if (n == 3) {
//读取职责
strcpy(duty, str);
}
else {
//读取成员(组员)
char mem[3][20]; //保存成员信息
int j1 = 0, k1 = 0;
for (int i = 0; i < strlen(str); i++) {
if (str[i] == ' ') {
mem[k1][j1] = '\0';
switch (k1)
{
case 0: strcpy(user[n - 3].ID, mem[k1]); break;
case 1: strcpy(user[n - 3].name, mem[k1]); break;
}
k1++;
j1 = 0;
}
else {
mem[k1][j1] = str[i];
j1++;
}
}
mem[k1][j1] = '\0';
strcpy(user[n - 3].phone, mem[k1]);
}
if (t) {
file1.getline(str, maxn);
}
}
//再读取“组长”组的成员信息
int m = 0; //追踪LeaderInformation.txt文件读取到了第几行
t = 1;
file2.getline(str, maxn);
while (t)
{
if (file2.eof()) {
t = 0;
}
m++;
if (m > 3) {
char lea[3][20];
int j2 = 0, k2 = 0;
for (int i = 0; i < strlen(str); i++) {
if (str[i] == ' ') {
lea[k2][j2] = '\0';
switch (k2)
{
case 0: strcpy(user[n + m - 6].ID, lea[k2]); break;
case 1: strcpy(user[n + m - 6].name, lea[k2]); break;
}
k2++;
j2 = 0;
}
else {
lea[k2][j2] = str[i];
j2++;
}
}
lea[k2][j2] = '\0';
strcpy(user[n + m - 6].phone, lea[k2]);
}
if (t) {
file2.getline(str, maxn);
}
}
Mnum = n + m - 6;
file1.close();
file2.close();
}
//Leader的缺省构造函数
Leader::Leader()
{
fstream file1, file2;
file1.open("LeaderInformation.txt", ios::in);
if (file1.fail()) {
cout << "LeaderInformation.txt打开失败" << endl;
exit(0);
}
//读取组员的职责信息(组员的职责也是组长的职责)
file2.open("MemberInformation.txt", ios::in);
if (file2.fail()) {
cout << "MemberInformation.txt打开失败" << endl;
exit(0);
}
//先读取“组长”组的全部信息
char str[maxn];
int n = 0; //追踪LeaderInformation.txt文件读取到了第几行
int t = 1;
file1.getline(str, maxn);
while (t) {
if (file1.eof()) {
t = 0;
}
n++;
if (n == 1) {
//读取编号
strcpy(num, str);
}
else if (n == 2) {
//读取称呼
strcpy(call, str);
}
else if (n == 3) {
//读取职责
strcpy(duty, str);
}
else {
//读取成员(组员)
char lea[3][20]; //保存成员信息
int j1 = 0, k1 = 0;
for (int i = 0; i < strlen(str); i++) {
if (str[i] == ' ')
{
lea[k1][j1] = '\0';
switch (k1)
{
case 0: strcpy(user[n - 3].ID, lea[k1]); break;
case 1: strcpy(user[n - 3].name, lea[k1]); break;
}
k1++;
j1 = 0;
}
else {
lea[k1][j1] = str[i];
j1++;
}
}
lea[k1][j1] = '\0';
strcpy(user[n - 3].phone, lea[k1]);
}
if (t) {
file1.getline(str, maxn);
}
}
//再读取“组员”组的职责信息
int m = 0; //追踪MemberInformation.txt文件读取到了第几行
t = 1;
file2.getline(str, maxn);
while (t) {
if (file2.eof()) {
t = 0;
}
m++;
if (m == 3) {
strcat(duty, " ");
strcat(duty, str);
break;
}
if (t) {
file2.getline(str, maxn);
}
}
Lnum = n - 3;
file1.close();
file2.close();
}
//Member查询用户是否存在(若存在,返回用户序号)
int Member::FindPerson(char* name1)
{
for (int i = 1; i <= Mnum; i++) {
if (strcmp(user[i].name, name1) == 0) {
return i;
}
}
return 0;
}
//Leader查询用户是否存在(若存在,返回用户序号)
int Leader::FindPerson(char* name1)
{
for (int i = 1; i <= Lnum; i++) {
if (strcmp(user[i].name, name1) == 0) {
return i;
}
}
return 0;
}
//Member显示某个用户的信息(n:用户序号)
void Member::ShowPerson(int n)
{
cout << "\n\t\t用户信息已导出\n";
cout << "\t\t编号:" << user[n].ID << endl;
cout << "\t\t姓名:" << user[n].name << endl;
cout << "\t\t联系电话:" << user[n].phone << endl;
}
//Leader显示某个用户的信息(n:用户序号)
void Leader::ShowPerson(int n)
{
cout << "\n\t\t用户信息已导出\n";
cout << "\t\t编号:" << user[n].ID << endl;
cout << "\t\t姓名:" << user[n].name << endl;
cout << "\t\t联系电话:" << user[n].phone << endl;
}
//Member返回用户组称呼
char* Member::GetCall()
{
return call;
}
//Leader返回用户组称呼
char* Leader::GetCall()
{
return call;
}
//Member返回用户组职责
char* Member::GetDuty()
{
return duty;
}
//Leader返回用户组职责
char* Leader::GetDuty()
{
return duty;
}
//Member显示组内所有用户的信息
void Member::ShowPersons()
{
cout << "\n\t\t组员信息已全部导出\n";
for (int i = 1; i <= Mnum; i++) {
cout << "\t\t编号:" << user[i].ID << "\t姓名:" << user[i].name << "\t电话:" << user[i].phone << endl;
}
cout << "\t\t信息导出完毕\n";
}
//Leader显示组内所有用户的信息
void Leader::ShowPersons()
{
cout << "\n\t\t组长信息已全部导出\n";
for (int i = 1; i <= Lnum; i++) {
cout << "\t\t编号:" << user[i].ID << "\t姓名:" << user[i].name << "\t电话:" << user[i].phone << endl;
}
cout << "\t\t信息导出完毕\n";
}
//Member显示“组员”组信息
void Member::ShowGroup()
{
cout << "\n\t\t用户组信息已导出\n";
cout << "\t\t编号:" << num << endl;
cout << "\t\t名称:" << call << endl;
cout << "\t\t职责:" << duty << endl;
cout << "\t\t信息导出完毕\n";
}
//Leader显示“组长”组信息
void Leader::ShowGroup()
{
cout << "\n\t\t用户组信息已导出\n";
cout << "\t\t编号:" << num << endl;
cout << "\t\t名称:" << call << endl;
cout << "\t\t职责:" << duty << endl;
cout << "\t\t信息导出完毕\n";
}
//Member删除用户(m:是否从文件中删除 n:用户序号)
void Member::DeletePerson(int m, int n)
{
//将用户在程序中删除
for (int i = n; i < Mnum; i++) {
strcpy(user[i].ID, user[i + 1].ID);
strcpy(user[i].name, user[i + 1].name);
strcpy(user[i].phone, user[i + 1].phone);
}
Mnum--;
if (m != 1) {
return;
}
//将用户从文件中删除
fstream file, file1;
file.open("MemberInformation.txt", ios::in);
if (file.fail()) {
cout << "MemberInformation.txt打开失败" << endl;
exit(0);
}
file1.open("EmptyFile.dat", ios::out);
if (file1.fail()) {
cout << "EmptyFile.dat打开失败" << endl;
exit(0);
}
int m1 = 1; //保存读到文件中的第几行
char str[maxn];
file.getline(str, maxn);
while (!file.eof()) {
if (m1 == n + 3) {
file.getline(str, maxn);
}
else {
if (m1 > 1) {
file1 << '\n';
}
file1 << str;
file.getline(str, maxn);
}
m1++;
}
if (m1 != n + 3) {
file1 << '\n' << str; //最后一行之后不能读入换行符
}
file.close();
file1.close();
file.open("MemberInformation.txt", ios::out);
if (file.fail()) {
cout << "MemberInformation.txt打开失败" << endl;
exit(0);
}
file1.open("EmptyFile.dat", ios::in);
if (file1.fail()) {
cout << "EmptyFile.dat打开失败" << endl;
exit(0);
}
file1.getline(str, maxn);
while (!file1.eof()) {
file << str << '\n';
file1.getline(str, maxn);
}
file << str; //最后一行之后不能读入换行符
file.close();
file1.close();
}
//Leader删除用户(n:用户序号)
void Leader::DeletePerson(int n)
{
//将用户在程序中删除
for (int i = n; i < Lnum; i++) {
strcpy(user[i].ID, user[i + 1].ID);
strcpy(user[i].name, user[i + 1].name);
strcpy(user[i].phone, user[i + 1].phone);
}
Lnum--;
//将用户从文件中删除
fstream file, file1;
file.open("LeaderInformation.txt", ios::in);
if (file.fail()) {
cout << "LeaderInformation.txt打开失败" << endl;
exit(0);
}
file1.open("EmptyFile.dat", ios::out);
if (file1.fail()) {
cout << "EmptyFile.dat打开失败" << endl;
exit(0);
}
int m1 = 1; //保存读到文件中的第几行
char str[maxn];
file.getline(str, maxn);
while (!file.eof()) {
if (m1 == n + 3) {
file.getline(str, maxn);
}
else {
if (m1 > 1) {
file1 << '\n';
}
file1 << str;
file.getline(str, maxn);
}
m1++;
}
if (m1 != n + 3) {
file1 << '\n' << str; //最后一行之后不能读入换行符
}
file.close();
file1.close();
file.open("LeaderInformation.txt", ios::out);
if (file.fail()) {
cout << "LeaderInformation.txt打开失败" << endl;
exit(0);
}
file1.open("EmptyFile.dat", ios::in);
if (file1.fail()) {
cout << "EmptyFile.dat打开失败" << endl;
exit(0);
}
file1.getline(str, maxn);
while (!file1.eof()) {
file << str << '\n';
file1.getline(str, maxn);
}
file << str; //最后一行之后不能读入换行符
file.close();
file1.close();
}
//Member添加用户(m:是否在文件中添加 n1:人员编号 n2:人员姓名 p:人员电话)
void Member::InsertPerson(int m, char* n1, char* n2, char* p)
{
//将用户在程序中添加
strcpy(user[Mnum + 1].ID, n1);
strcpy(user[Mnum + 1].name, n2);
strcpy(user[Mnum + 1].phone, p);
Mnum++; //人员加1
if (m != 1) {
return;
}
//将用户添加到文件中
string str1;
char str2[maxn];
str1.append(n1);
str1.append(1, ' ');
str1.append(n2);
str1.append(1, ' ');
str1.append(p);
int i;
for (i = 0; i < str1.length(); i++) {
str2[i] = str1[i];
}
str2[i] = '\0';
fstream file;
file.open("MemberInformation.txt", ios::out | ios::app);
if (file.fail()) {
cout << "MemberInformation.txt打开失败" << endl;
exit(0);
}
file.seekg(0L, ios::end); //添加到文件尾
file << '\n' << str2;
file.close();
}
//Leader添加用户(n1:人员编号 n2:人员姓名 p:人员电话)
void Leader::InsertPerson(char* n1, char* n2, char* p)
{
//将用户在程序中添加
strcpy(user[Lnum + 1].ID, n1);
strcpy(user[Lnum + 1].name, n2);
strcpy(user[Lnum + 1].phone, p);
Lnum++;
//将用户添加到文件中
string str1;
char str2[maxn];
str1.append(n1);
str1.append(1, ' ');
str1.append(n2);
str1.append(1, ' ');
str1.append(p);
int i;
for (i = 0; i < str1.length(); i++) {
str2[i] = str1[i];
}
str2[i] = '\0';
fstream file;
file.open("LeaderInformation.txt", ios::out | ios::app);
if (file.fail()) {
cout << "LeaderInformation.txt打开失败" << endl;
exit(0);
}
file.seekg(0L, ios::end); //添加到文件尾
file << '\n' << str2;
file.close();
}
//Member修改用户信息
//(a:是否在文件中修改 m:修改的具体对象(编号、姓名或电话)n:修改人员的序号 s:修改后的结果)
void Member::RevisePerson(int a, int m, int n, char* s)
{
//在程序中修改用户信息
switch (m)
{
case 1: strcpy(user[n].ID, s); break;
case 2: strcpy(user[n].name, s); break;
case 3: strcpy(user[n].phone, s); break;
}
if (a != 1) {
return;
}
//将修改后的用户信息保存在文件中
string str1;
char str2[maxn];
str1.append(user[n].ID);
str1.append(1, ' ');
str1.append(user[n].name);
str1.append(1, ' ');
str1.append(user[n].phone);
int i;
for (i = 0; i < str1.length(); i++) {
str2[i] = str1[i];
}
str2[i] = '\0';
fstream file, file1;
file.open("MemberInformation.txt", ios::in);
if (file.fail()) {
cout << "MemberInformation.txt打开失败" << endl;
exit(0);
}
file1.open("EmptyFile.dat", ios::out);
if (file1.fail()) {
cout << "EmptyFile.dat打开失败" << endl;
exit(0);
}
int m1 = 1; //保存读到文件中的第几行
char str[maxn];
file.getline(str, maxn);
while (!file.eof())
{
if (m1 > 1) {
file1 << '\n';
}
if (m1 == n + 3) {
file1 << str2;
}
else {
file1 << str;
}
file.getline(str, maxn);
m1++;
}
if (m1 == n + 3) {
file1 << '\n' << str2; //最后一行之后不能读入换行符
}
else {
file1 << '\n' << str; //最后一行之后不能读入换行符
}
file.close();
file1.close();
file.open("MemberInformation.txt", ios::out);
if (file.fail()) {
cout << "MemberInformation.txt打开失败" << endl;
exit(0);
}
file1.open("EmptyFile.dat", ios::in);
if (file1.fail()) {
cout << "EmptyFile.dat打开失败" << endl;
exit(0);
}
file1.getline(str, maxn);
while (!file1.eof()) {
file << str << '\n';
file1.getline(str, maxn);
}
file << str;
file.close();
file1.close();
}
//Leader修改用户信息(m:修改的具体对象(编号、姓名或电话)n:修改人员的序号 s:修改后的结果)
void Leader::RevisePerson(int m, int n, char* s)
{
//在程序中修改用户信息
switch (m)
{
case 1: strcpy(user[n].ID, s); break;
case 2: strcpy(user[n].name, s); break;
case 3: strcpy(user[n].phone, s); break;
}
//将修改后的用户信息保存在文件中
string str1;
char str2[maxn];
str1.append(user[n].ID);
str1.append(1, ' ');
str1.append(user[n].name);
str1.append(1, ' ');
str1.append(user[n].phone);
int i;
for (i = 0; i < str1.length(); i++) {
str2[i] = str1[i];
}
str2[i] = '\0';
fstream file, file1;
file.open("LeaderInformation.txt", ios::in);
if (file.fail()) {
cout << "LeaderInformation.txt打开失败" << endl;
exit(0);
}
file1.open("EmptyFile.dat", ios::out);
if (file1.fail()) {
cout << "EmptyFile.dat打开失败" << endl;
exit(0);
}
int m1 = 1; //保存读到文件中的第几行
char str[maxn];
file.getline(str, maxn);
while (!file.eof()) {
if (m1 > 1) {
file1 << '\n';
}
if (m1 == n + 3) {
file1 << str2;
}
else {
file1 << str;
}
file.getline(str, maxn);
m1++;
}
if (m1 == n + 3) {
file1 << '\n' << str2; //最后一行之后不能读入换行符
}
else {
file1 << '\n' << str; //最后一行之后不能读入换行符
}
file.close();
file1.close();
file.open("LeaderInformation.txt", ios::out);
if (file.fail()) {
cout << "LeaderInformation.txt打开失败" << endl;
exit(0);
}
file1.open("EmptyFile.dat", ios::in);
if (file1.fail()) {
cout << "EmptyFile.dat打开失败" << endl;
exit(0);
}
file1.getline(str, maxn);
while (!file1.eof()) {
file << str << '\n';
file1.getline(str, maxn);
}
file << str;
file.close();
file1.close();
}
//Member修改“组员”用户组信息(n:修改的具体对象(编号、称呼或职责) s:修改后的结果)
void Member::ReviseGroup(int n, char* s)
{
//在程序中修改
switch (n)
{
case 1: strcpy(num, s); break;
case 2: strcpy(call, s); break;
case 3: strcpy(duty, s); break;
}
//将修改后的用户信息保存在文件中
fstream file, file1;
file.open("MemberInformation.txt", ios::in);
if (file.fail()) {
cout << "MemberInformation.txt打开失败" << endl;
exit(0);
}
file1.open("EmptyFile.dat", ios::out);
if (file1.fail()) {
cout << "EmptyFile.dat打开失败" << endl;
exit(0);
}
int m1 = 1; //保存读到文件中的第几行
char str[maxn];
file.getline(str, maxn);
while (!file.eof()) {
if (m1 == n) {
file1 << s << '\n';
}
else {
file1 << str << '\n';
}
file.getline(str, maxn);
m1++;
}
file1 << str; //最后一行之后不能读入换行符
file.close();
file1.close();
file.open("MemberInformation.txt", ios::out);
if (file.fail()) {
cout << "MemberInformation.txt打开失败" << endl;
exit(0);
}
file1.open("EmptyFile.dat", ios::in);
if (file1.fail()) {
cout << "EmptyFile.dat打开失败" << endl;
exit(0);
}
file1.getline(str, maxn);
while (!file1.eof()) {
file << str << '\n';
file1.getline(str, maxn);
}
file << str;
file.close();
file1.close();
}
//Leader修改“组长”用户组信息(m:是否修改文件 n:修改的具体对象(编号、称呼或专有职责)
//s1:组长的职责或其他修改后的结果 s2:组员的职责)
void Leader::ReviseGroup(int m, int n, char* s1, char* s2)
{
char s[maxn];
strcpy(s, s1);
//在程序中修改
if (n == 3) {
//如果修改的是职责
strcat(s1, " ");
strcat(s1, s2);
strcpy(duty, s1);
}
else {
switch (n)
{
case 1: strcpy(num, s1); break;
case 2: strcpy(call, s1); break;
}
}
if (m != 1) {
return;
}
//将修改后的用户信息保存在文件中
fstream file, file1;
file.open("LeaderInformation.txt", ios::in);
if (file.fail()) {
cout << "LeaderInformation.txt打开失败" << endl;
exit(0);
}
file1.open("EmptyFile.dat", ios::out);
if (file1.fail()) {
cout << "EmptyFile.dat打开失败" << endl;
exit(0);
}
int m1 = 1; //保存读到文件中的第几行
char str[maxn];
file.getline(str, maxn);
while (!file.eof()) {
if (m1 == n) {
file1 << s << '\n';
}
else {
file1 << str << '\n';
}
file.getline(str, maxn);
m1++;
}
file1 << str; //最后一行之后不能读入换行符
file.close();
file1.close();
file.open("LeaderInformation.txt", ios::out);
if (file.fail()) {
cout << "LeaderInformation.txt打开失败" << endl;
exit(0);
}
file1.open("EmptyFile.dat", ios::in);
if (file1.fail()) {
cout << "EmptyFile.dat打开失败" << endl;
exit(0);
}
file1.getline(str, maxn);
while (!file1.eof()) {
file << str << '\n';
file1.getline(str, maxn);
}
file << str;
file.close();
file1.close();
}
void Frame1(); //大框架函数
void Frame2(); //小框架函数
void Menu_Member(); //组员专用菜单
void Menu_Leader(); //组长专用菜单
void Search(); //查询用户信息
void ShowPeople(); //显示组内用户信息
void ShowGroup(); //显示用户组信息
void Insert(); //添加用户信息
void Delete(); //删除用户信息
void Change(); //修改用户信息
Member M; //组长组
Leader L; //组员组
int main()
{
int select;
int identity; //用户身份变量
Frame1();
cout << "\n--------------------------------------------------- ";
cout << "欢迎使用RBAC系统";
cout << " -------------------------------------------------- \n";
Frame1();
cout << '\n';
//总的程序下,可以多次进行不同人员的操作
while (1) {
cout << "\n\t\t您的用户身份: (1)组员 (2)组长\n";
cout << "\t\t请选择:";
cin >> identity;
cout << endl;
Frame2();
cout << "\t\t** 欢迎来到用户界面 **\n";
Frame2();
if (identity == 1) {
//组员部分
int quit1 = 0;
while (1) {
int select1;
Menu_Member(); //组员专用菜单
cout << "\t\t请选择:";
cin >> select1;
switch (select1)
{
case 1: Search(); break; //查询用户信息
case 2: ShowPeople(); break; //显示用户组所有用户
case 3: ShowGroup(); break; //显示用户组信息
case 4: quit1 = 1; break; //退出
}
if (quit1) {
break;
}
}
}
else if (identity == 2) {
//组长部分
int quit2 = 0;
while (1) {
int select2;
Menu_Leader(); //组长专用菜单
cout << "\t\t请选择:";
cin >> select2;
switch (select2)
{
case 1: Search(); break; //查询用户信息
case 2: ShowPeople(); break; //显示用户组所有用户
case 3: ShowGroup(); break; //显示用户组信息
case 4: Insert(); break; //添加用户信息
case 5: Delete(); break; //删除用户信息
case 6: Change(); break; //修改用户信息
case 7: quit2 = 1; break; //退出
}
if (quit2) {
break;
}
}
}
cout << "\n\t\t是否退出程序: (1)继续运行\t(其他任意键)退出\n";
cout << "\t\t请选择:";
cin >> select;
if (select != 1) {
cout << "\n\t\t程序运行结束\n";
break;
}
}
return 0;
}
void Frame1()
{
for (int i = 0; i < 120; i++) {
cout << "*";
}
cout << "\n";
}
void Frame2()
{
cout << "\t\t";
for (int i = 0; i < 23; i++) {
cout << "*";
}
cout << "\n";
}
//组员专用菜单
void Menu_Member()
{
cout << "\n\t\t*******************************\n";
cout << "\t\t**(1)、查询用户 *************\n";
cout << "\t\t**(2)、显示组内用户信息 *****\n";
cout << "\t\t**(3)、显示用户组信息 *******\n";
cout << "\t\t**(4)、退出 *****************\n";
cout << "\t\t*******************************\n";
}
//组长专用菜单
void Menu_Leader()
{
cout << "\n\t\t*******************************\n";
cout << "\t\t**(1)、查询用户 *************\n";
cout << "\t\t**(2)、显示组内用户信息 *****\n";
cout << "\t\t**(3)、显示用户组信息 *******\n";
cout << "\t\t**(4)、添加用户 *************\n";
cout << "\t\t**(5)、删除用户 *************\n";
cout << "\t\t**(6)、修改信息 *************\n";
cout << "\t\t**(7)、退出 *****************\n";
cout << "\t\t*******************************\n";
}
//查询用户信息
void Search()
{
char name1[15];
int t = 1;
while (t) {
cout << "\n\t\t请输入要查询的用户姓名:";
cin >> name1;
int a, b;
a = L.FindPerson(name1);
b = M.FindPerson(name1);
//先判断是否为组长
if (a) {
//是组长
L.ShowPerson(a);
cout << "\t\t所属用户组:" << L.GetCall() << " " << M.GetCall() << endl;
cout << "\t\t信息导出完毕\n";
}
else if (b) {
//是组员
M.ShowPerson(b);
cout << "\t\t所属用户组:" << M.GetCall() << endl;
cout << "\t\t信息导出完毕\n";
}
else {
cout << "\t\t查无此人" << endl;
}
cout << "\n\t\t是否继续查询:(1)是 (2)否\n";
cout << "\t\t请选择:";
cin >> t;
if (t != 1) {
break;
}
}
}
//显示组内用户信息
void ShowPeople()
{
int select;
cout << "\n\t\t用户组:(1)组员 (2)组长" << endl;
cout << "\t\t请选择:";
cin >> select;
if (select == 1) {
//组员组
M.ShowPersons();
}
else {
//组长组
L.ShowPersons();
}
}
//显示用户组信息
void ShowGroup()
{
int select;
cout << "\n\t\t用户组:(1)组员 (2)组长" << endl;
cout << "\t\t请选择:";
cin >> select;
if (select == 1) {
//组员组
M.ShowGroup();
}
else {
//组长组
L.ShowGroup();
}
}
//添加用户信息
void Insert()
{
char name1[15], num1[20], phone1[20];
int t = 1;
while (t) {
int select;
cout << "\n\t\t用户所属的组:(1)组员(2)组长" << endl;
cout << "\t\t请选择:";
cin >> select;
cout << "\t\t请输入要添加的用户编号:";
cin >> num1;
cout << "\t\t请输入要添加的用户姓名:";
cin >> name1;
cout << "\t\t请输入要添加的用户联系电话:";
cin >> phone1;
if (select == 1) {
//添加组员
M.InsertPerson(1, num1, name1, phone1);
}
else {
//添加组长
L.InsertPerson(num1, name1, phone1);
M.InsertPerson(2, num1, name1, phone1); //无需添加到“组员”组文件中
}
cout << "\n\t\t是否继续添加:(1)是 (2)否\n";
cout << "\t\t请选择:";
cin >> t;
if (t != 1) {
break;
}
}
}
//删除用户信息
void Delete()
{
char name1[15];
int t = 1;
while (t) {
int select;
cout << "\n\t\t用户所属的组:(1)组员(2)组长" << endl;
cout << "\t\t请选择:";
cin >> select;
cout << "\n\t\t请输入要删除的用户姓名:";
cin >> name1;
int a, b;
if (select == 1) {
//删除组员
a = M.FindPerson(name1);
if (!a) {
cout << "\t\t查无此人" << endl; //该人不存在
}
else {
M.DeletePerson(1, a);
}
}
else
{
//删除组长
b = L.FindPerson(name1);
if (!b) {
cout << "\t\t查无此人" << endl; //该人不存在
}
else {
a = M.FindPerson(name1);
M.DeletePerson(2, a); //无需从文件中删除
L.DeletePerson(b);
}
}
cout << "\n\t\t是否继续删除:(1)是 (2)否\n";
cout << "\t\t请选择:";
cin >> t;
if (t != 1) {
break;
}
}
}
//修改用户信息
void Change()
{
int t = 1;
while (t) {
int select1, select2, select3, select4;
char str[maxn], name1[15];
int a, b;
cout << "\n\t\t所属的组:(1)组员(2)组长" << endl;
cout << "\t\t请选择:";
cin >> select1;
cout << "\n\t\t要修改的对象:(1)用户(2)用户组" << endl;
cout << "\t\t请选择:";
cin >> select2;
if (select1 == 1) {
//组员
if (select2 == 1) {
cout << "\n\t\t请输入要修改信息的人员姓名:";
cin >> name1;
a = M.FindPerson(name1);
if (!a) {
cout << "\t\t查无此人" << endl;
}
else {
cout << "\n\t\t要修改的项目:(1)编号(2)姓名(3)电话" << endl;
cout << "\t\t请选择:";
cin >> select3;
cout << "\n\t\t请输入修改后的结果:";
cin >> str;
M.RevisePerson(1, select3, a, str);
}
}
else {
cout << "\n\t\t要修改的项目:(1)编号(2)称呼(3)职责" << endl;
cout << "\t\t请选择:";
cin >> select4;
cin.ignore(); //过滤换行符
cout << "\n\t\t请输入修改后的结果:";
cin.getline(str, maxn);
if (select4 == 3) {
int m = 1;
char str1[maxn];
fstream file;
file.open("LeaderInformation.txt", ios::in);
if (file.fail()) {
cout << "LeaderInformation.txt打开失败" << endl;
exit(0);
}
file.getline(str1, maxn);
while (!file.eof()) {
if (m == 3)
{
break;
}
file.getline(str1, maxn);
m++;
}
file.close();
//组员的职责发生改变,组长的职责也会随之变化
L.ReviseGroup(2, select4, str1, str);
}
M.ReviseGroup(select4, str);
}
}
else {
if (select2 == 1) {
//组长
cout << "\n\t\t请输入要修改信息的人员姓名:";
cin >> name1;
b = L.FindPerson(name1);
if (!b) {
cout << "\t\t查无此人" << endl;
}
else {
a = M.FindPerson(name1);
cout << "\n\t\t要修改的项目:(1)编号(2)姓名(3)电话" << endl;
cout << "\t\t请选择:";
cin >> select3;
cout << "\n\t\t请输入修改后的结果:";
cin >> str;
M.RevisePerson(2, select3, a, str);
L.RevisePerson(select3, b, str);
}
}
else {
cout << "\n\t\t要修改的项目:(1)编号(2)称呼(3)专有职责" << endl;
cout << "\t\t请选择:";
cin >> select4;
cin.ignore(); //过滤换行符
cout << "\n\t\t请输入修改后的结果:";
cin.getline(str, maxn);
char str2[maxn];
strcpy(str2, M.GetDuty());
L.ReviseGroup(1, select4, str, str2);
}
}
cout << "\n\t\t是否继续修改:(1)是 (2)否\n";
cout << "\t\t请选择:";
cin >> t;
if (t != 1) {
break;
}
}
}
四、总结
至于运行示例,这里就不展示了,想试试的可以自行复制粘贴。但务必按照系统提示运行。
在许多操作上,两个组都是大同小异,但我还是分开来写,当然是为了水行数啦!(划掉)。我之前写过的课设里有用过函数模板,如果构建模板的话,应该可以合在一起,但需要从很多细节上去协调,所以就懒得试了,复制粘贴它不香吗?(划掉)感兴趣的小伙伴可以试试。
以上是我对这题的看法,很高兴与大家分享。