6-7 Isomorphic(20 分)
Two trees, T1
and T2
, are isomorphic if T1
can be transformed into T2
by swapping left and right children of (some of the) nodes in T1
. For instance, the two trees in Figure 1 are isomorphic because they are the same if the children of A, B, and G, but not the other nodes, are swapped. Give a polynomial time algorithm to decide if two trees are isomorphic.
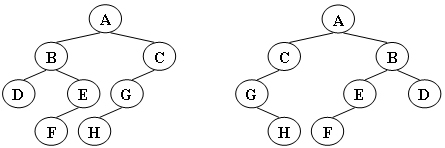
Figure 1
Format of functions:
int Isomorphic( Tree T1, Tree T2 );
where Tree
is defined as the following:
typedef struct TreeNode *Tree;
struct TreeNode {
ElementType Element;
Tree Left;
Tree Right;
};
The function is supposed to return 1 if T1
and T2
are indeed isomorphic, or 0 if not.
Sample program of judge:
#include <stdio.h>
#include <stdlib.h>
typedef char ElementType;
typedef struct TreeNode *Tree;
struct TreeNode {
ElementType Element;
Tree Left;
Tree Right;
};
Tree BuildTree(); /* details omitted */
int Isomorphic( Tree T1, Tree T2 );
int main()
{
Tree T1, T2;
T1 = BuildTree();
T2 = BuildTree();
printf(“%d\n”, Isomorphic(T1, T2));
return 0;
}
/* Your function will be put here */
Sample Output 1 (for the trees shown in Figure 1):
1
Sample Output 2 (for the trees shown in Figure 2):
0
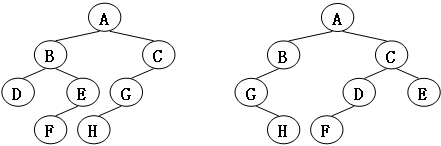
Figure2
作者: 陈越
单位: 浙江大学
时间限制: 400ms
内存限制: 64MB
代码长度限制: 16KB
int Isomorphic(Tree T1, Tree T2)
{int flag = 1, flag1 = 1, flag2 = 1, flag3 = 1, flag4 = 1;
if (T1 != NULL && T2 != NULL) {
if (T1->Element != T2->Element)
flag = 0;
else {
flag1 = Isomorphic(T1->Left, T2->Left);
flag2 = Isomorphic(T1->Left, T2->Right);
flag3 = Isomorphic(T1->Right, T2->Left);
flag4 = Isomorphic(T1->Right, T2->Right);
flag = (flag1&flag4) | (flag2&flag3);
}
}
if (T1 == NULL && T2 != NULL)
flag = 0;
if (T1 != NULL && T2 == NULL)
flag = 0;
return flag;
}