提交代码
class Solution {
public double[] calcEquation(List<List<String>> equations, double[] values, List<List<String>> queries) {
String start, end;
Map<String, Map<String, Double>> graph=new HashMap<>();
for(int i=0;i<equations.size();i++) {
start = equations.get(i).get(0);
end = equations.get(i).get(1);
if(graph.containsKey(start)) {
graph.get(start).put(end, values[i]);
}else {
Map<String, Double> path=new HashMap<>();
path.put(end, values[i]);
graph.put(start, path);
}
if(graph.containsKey(end)) {
graph.get(end).put(start, 1/values[i]);
}else {
Map<String, Double> path=new HashMap<>();
path.put(start, 1/values[i]);
graph.put(end, path);
}
}
Map<String, Boolean> visited = new HashMap<>();
for(String node: graph.keySet())
visited.put(node, false);
double[] res=new double[queries.size()];
for(int i=0;i<queries.size();i++) {
start = queries.get(i).get(0);
end = queries.get(i).get(1);
visited.put(start, true);
visited.put(end, true);
res[i] = findPath(start, end, graph, visited);
visited.put(start, false);
visited.put(end, false);
}
return res;
}
private double findPath(String start, String end, Map<String, Map<String, Double>> graph, Map<String, Boolean> visited) {
if(!graph.containsKey(start)||!graph.containsKey(end)) return -1;
if(graph.containsKey(start)&&graph.get(start).containsKey(end))
return graph.get(start).get(end);
double path = -1;
for(String curNode : graph.keySet()) {
if(visited.get(curNode)) continue;
if(!graph.get(start).containsKey(curNode)) continue;
visited.put(curNode, true);
path = findPath(curNode, end, graph, visited);
visited.put(curNode, false);
if(path != -1) {
path = graph.get(start).get(curNode)*path;
break;
}
}
return path;
}
}
运行结果
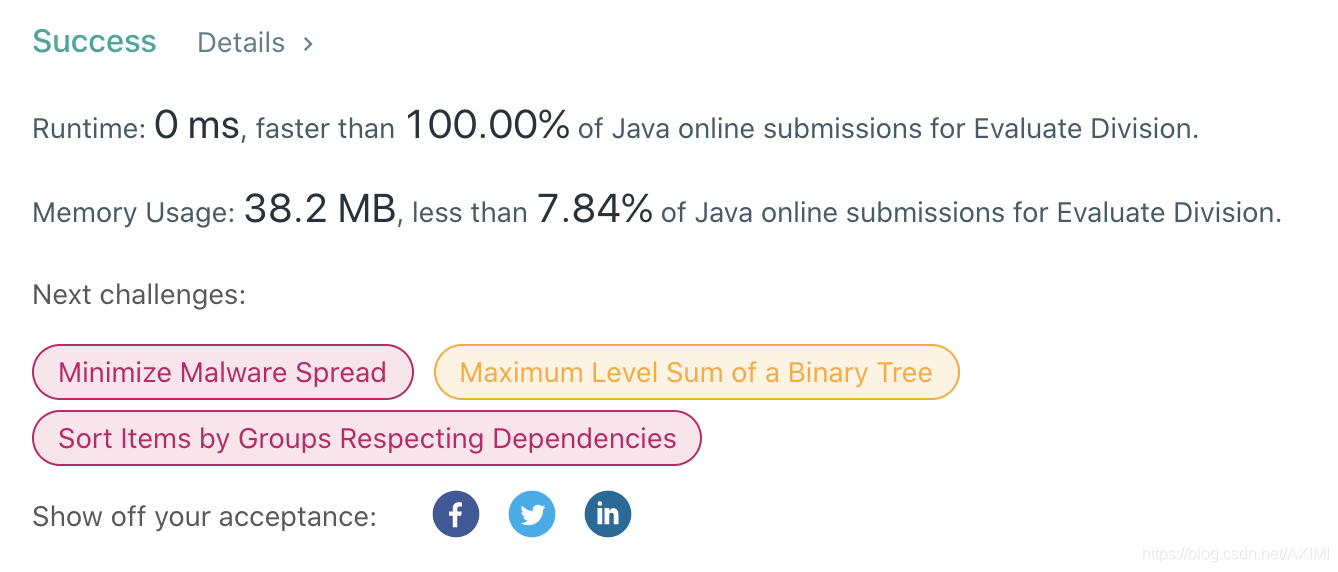