1.字符分类函数
C语⾔中有⼀系列的函数是专⻔做字符分类的,也就是⼀个字符是属于什么类型的字符的。这些函数的使⽤都需要包含⼀个头⽂件是 ctype.h![]()
2.字符转换函数
C语言提供了两个字符转换函数
int tolower ( int c ); // 将参数传进去的⼤写字⺟转⼩写int toupper ( int c ); // 将参数传进去的⼩写字⺟转⼤写
练习1:将小写字母转化为大写字母,将大写字母转化为小写字母
#include<ctype.h>
int main()
{
char n = 0;
while (scanf("%c", &n) != EOF)
{
if (islower(n))
{
printf("%c",toupper(n));
}
else if (isupper)
{
printf("%c",tolower(n));
}
}
return 0;
}
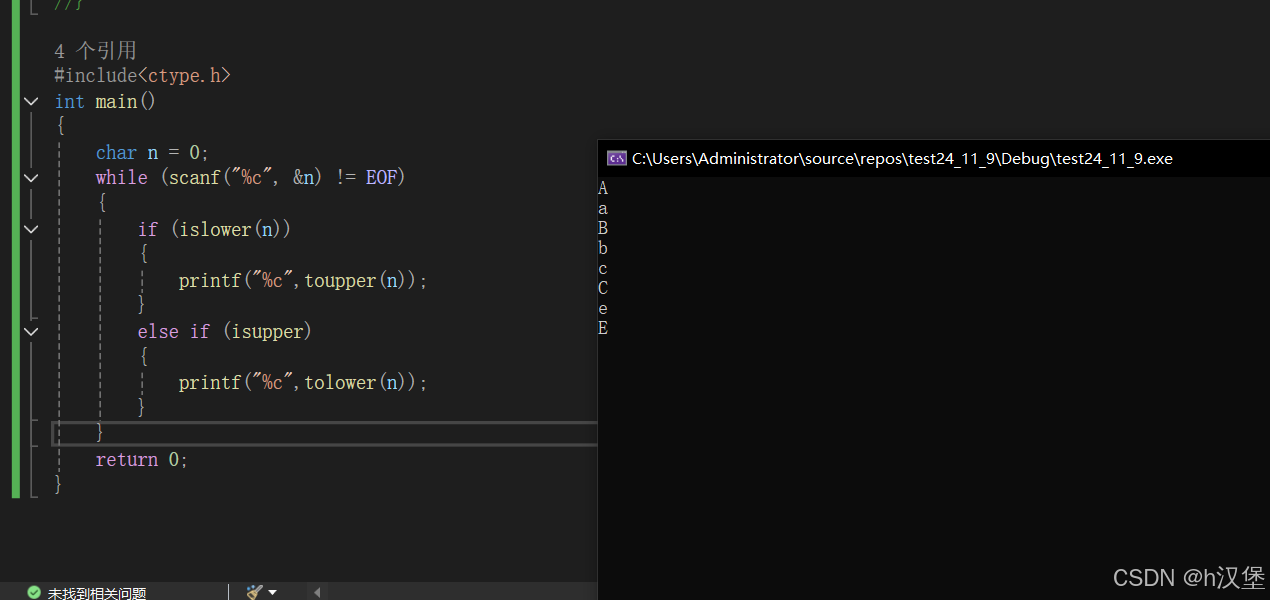
练习2:将文本中的小写字母转换成大写字母
#include<ctype.h>
int main()
{
int i = 0;
char arr[20] = "l like bite.\n";
while (arr[i])
{
if (islower(arr[i]))
{
arr[i]=toupper(arr[i]);
}
i++;
}
printf("%s\n", arr);
return 0;
}
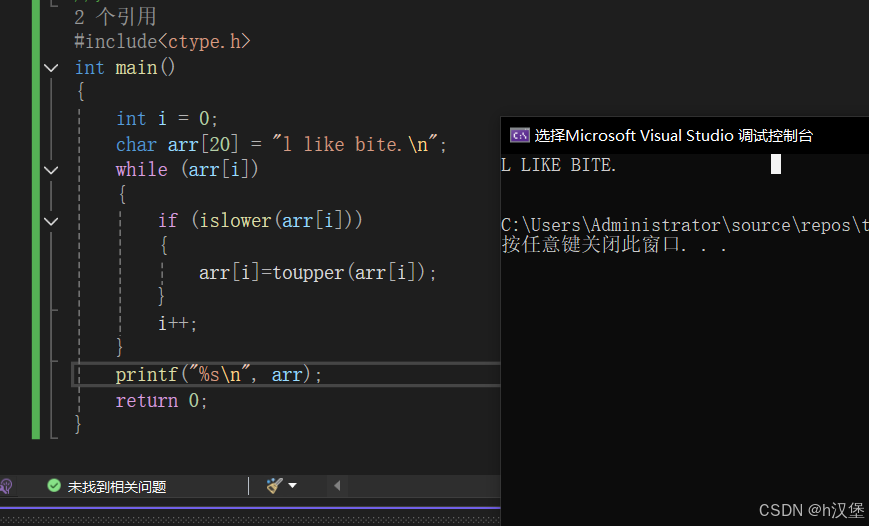
3.strlen的使用和模拟实现
size_t strlen ( const char * str );
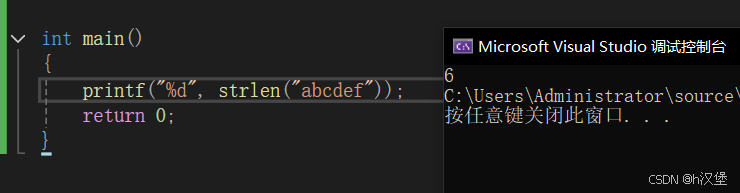
•
字符串以 '\0'
作为结束标志,strlen函数返回的是在字符串中
'\0'
前⾯出现的字符个数(不包
含
'\0'
)。
•
参数指向的字符串必须要以
'\0'
结束。
•
注意函数的返回值为 size_t,是无符号整形( 易错 )
•
strlen的使⽤需要包含头文件
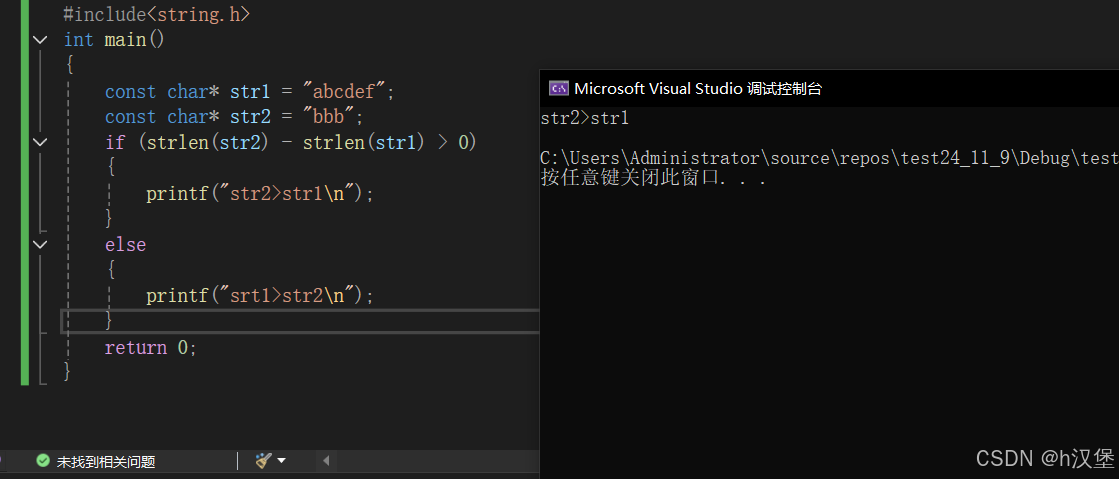
出现这样的结果就是因为他们返回值是无符号整形,运算结果转换成无符号数是一个很大的值。
strlen的模拟实现:
(1)计数器:
int my_strlen(const char* str)
{
int count = 0;
while (*str)
{
count++;
str++;
}
return count;
}
int main()
{
char arr[] = "abcedf";
printf("%d", my_strlen(arr));
return 0;
}
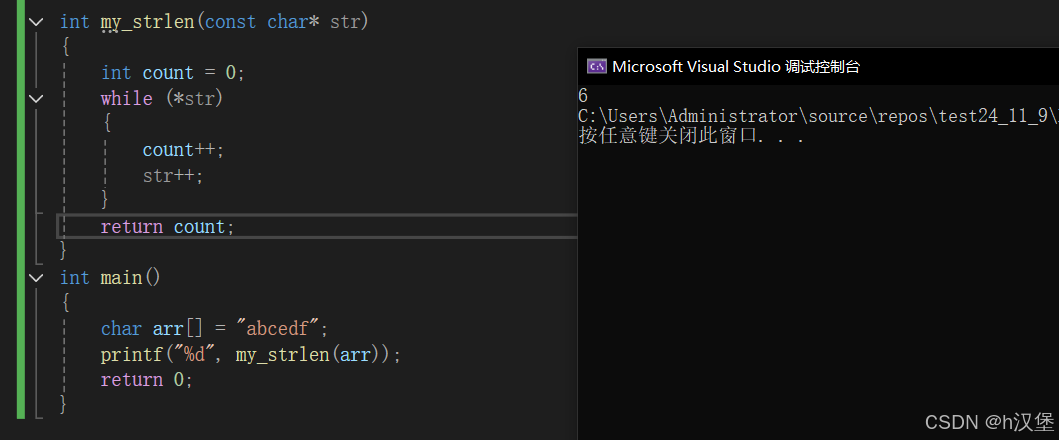
(2)指针运算:
int my_strlen(const char* str)
{
char* begin = (char*)str;
while (*str)
{
str++;
}
return str-begin;
}
int main()
{
char arr[] = "abcedf";
printf("%d", my_strlen(arr));
return 0;
}
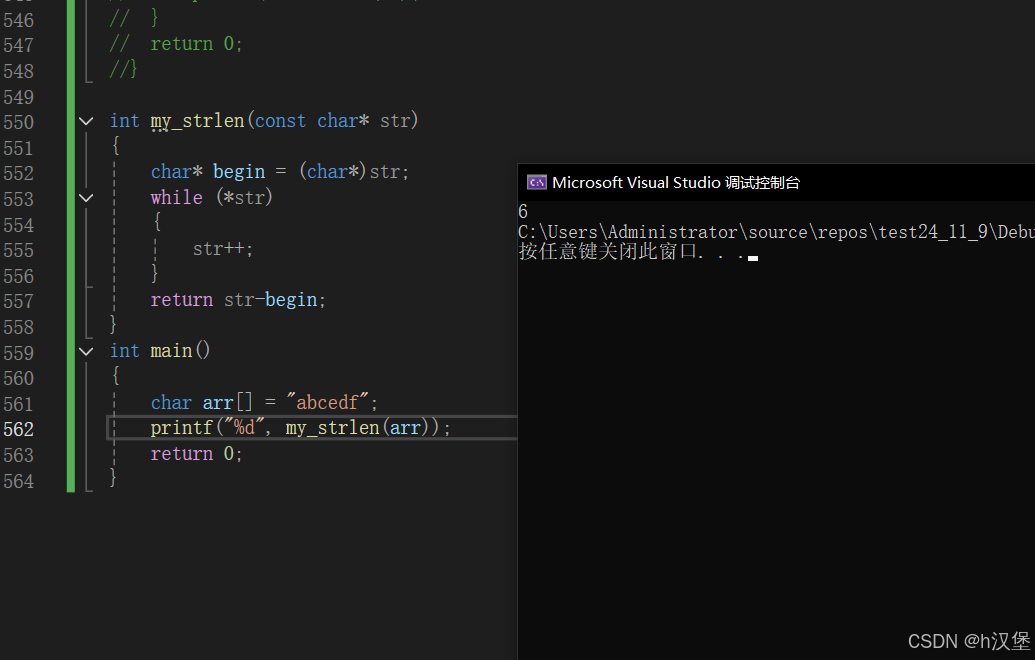
(3)递归法(无临时变量创建):
int my_strlen(const char* str)
{
if (*str!='\0')
{
return 1 + my_strlen(str + 1);
}
else
{
return 0;
}
}
int main()
{
char arr[] = "abcedf";
printf("%d", my_strlen(arr));
return 0;
}
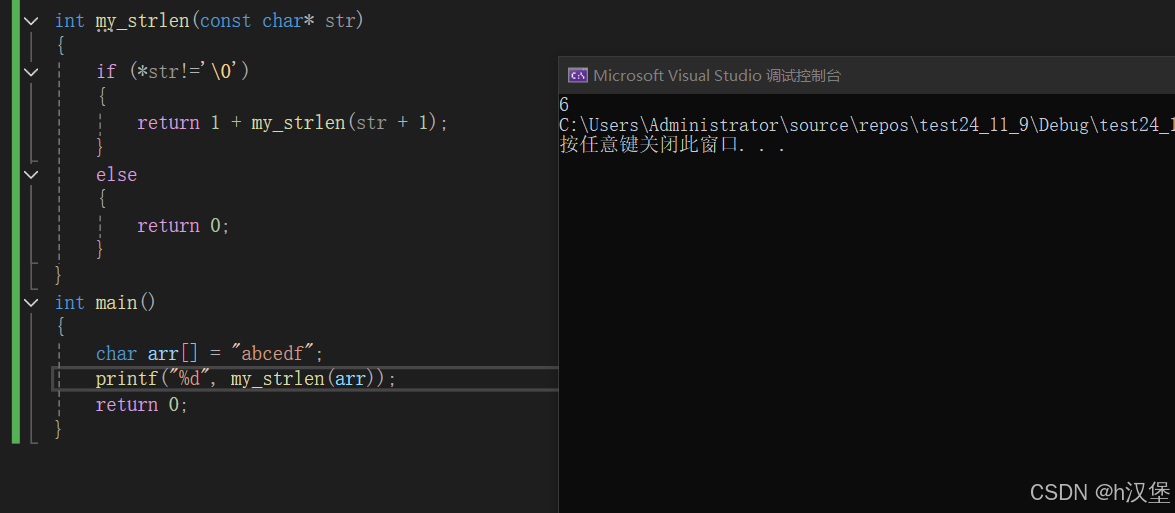
4.strcpy的使用和模拟实现
char * strcpy ( char * destination, const char * source );
•
Copies the C string pointed by source into the array pointed by destination, including the
terminating null character (and stopping at that point).
•
源字符串必须以
'\0'
结束。
•
会将源字符串中的
'\0'
拷贝到⽬标空间。
•
⽬标空间必须⾜够大,以确保能存放源字符串。
•
⽬标空间必须可修改。
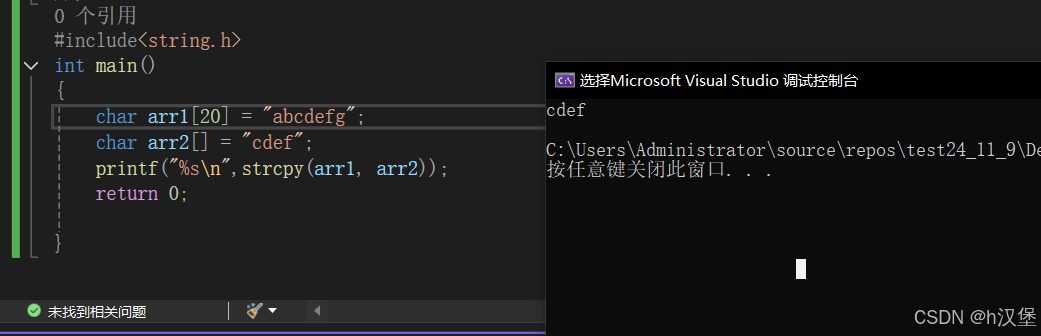
strcpy的模拟实现:
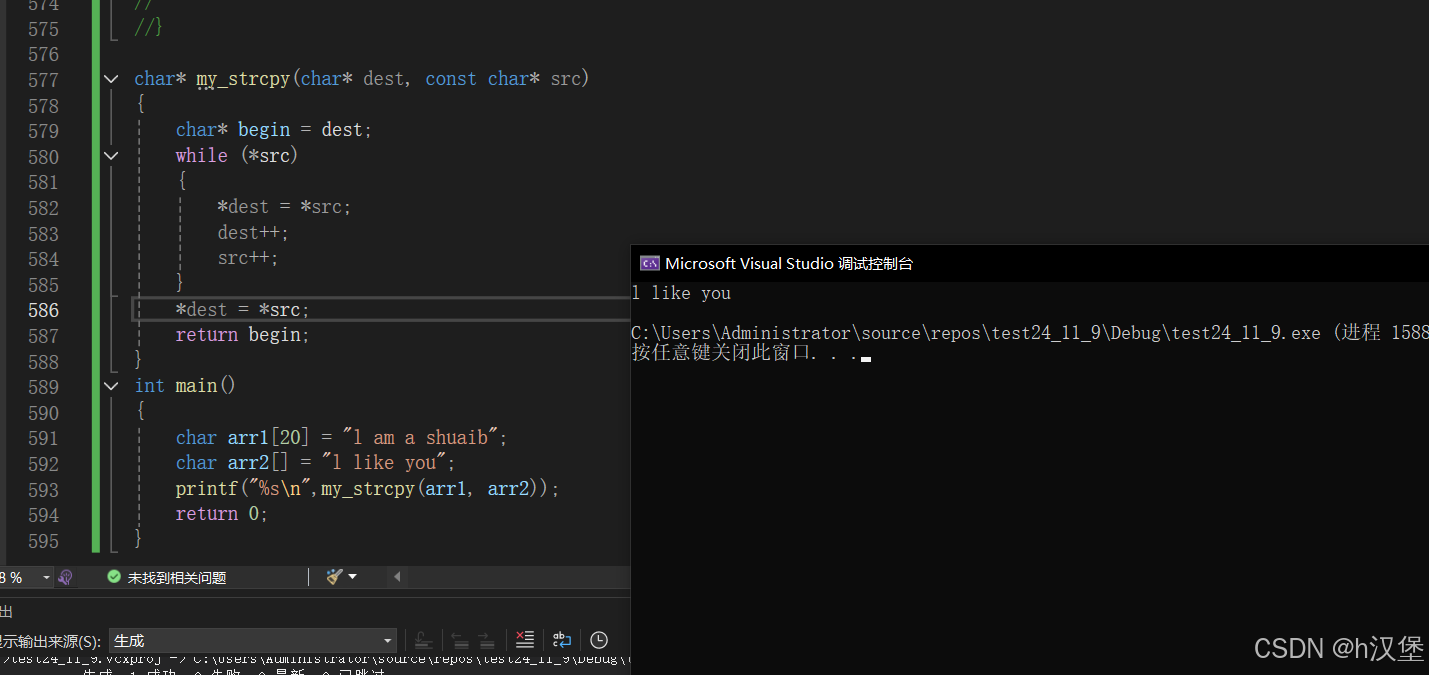
下面的那种更简洁
char* my_strcpy(char* dest, const char* src)
{
char* begin = dest;
while (*dest++=*src++)
{
;
}
return begin;
}
int main()
{
char arr1[20] = "l am a shuaib";
char arr2[] = "l like you";
printf("%s\n",my_strcpy(arr1, arr2));
return 0;
}
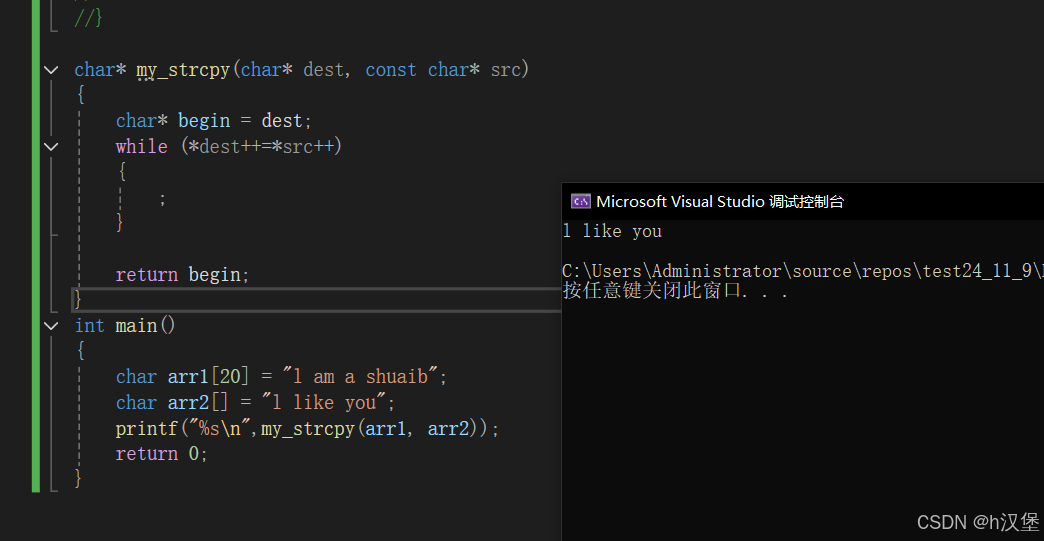
5.strcat的使用与模拟实现
char * strcat ( char * destination, const char * source );
•
Appends a copy of the source string to the destination string. The terminating null character
in destination is overwritten by the first character of source, and a null-character is included
at the end of the new string formed by the concatenation of both in destination.
•
源字符串必须以
'\0'
结束。
•
⽬标字符串中也得有
\0
,否则没办法知道追加从哪⾥开始。
•
⽬标空间必须有⾜够的⼤,能容纳下源字符串的内容。
•
⽬标空间必须可修改。
strcat的模拟实现:
char* my_strcay(char* str1, const char* str2)
{
char* begin = str1;
while (*str1)
{
str1++;
}
while (*str1++ = *str2++)
{
;
}
return begin;
}
int main()
{
char arr1[20] = "abcde";
char arr2[] = "fgjkh";
printf("%s\n", my_strcay(arr1, arr2));
return 0;
}
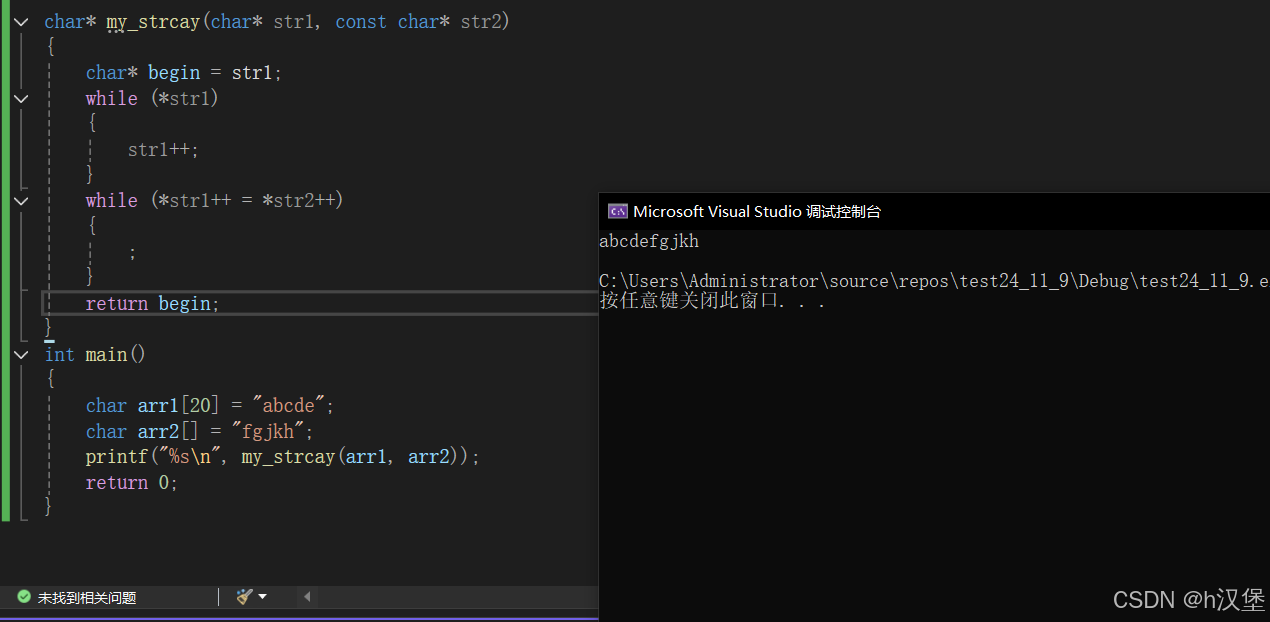
•
字符串⾃⼰给⾃⼰追加,如何?
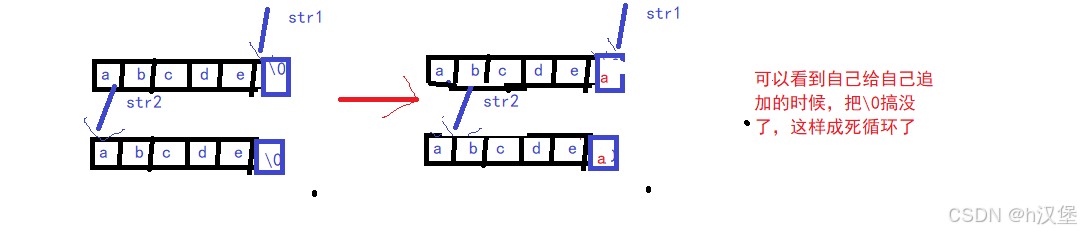
但是库函数的strcat是可以实现的,这个涉及到AMP算法
6.strcmp的使用和模拟实现
int strcmp ( const char * str1, const char * str2 );
•
This function starts comparing the first character of each string. If they are equal to each
other, it continues with the following pairs until the characters differ or until a terminating
null-character is reached.
•
标准规定:
◦
第⼀个字符串⼤于第⼆个字符串,则返回⼤于0的数字
◦
第⼀个字符串等于第⼆个字符串,则返回0
◦
第⼀个字符串⼩于第⼆个字符串,则返回⼩于0的数字
◦
那么如何判断两个字符串? 比较两个字符串中对应位置上字符ASCII码值的⼤小
strcmp的模拟实现:
int my_strcmp(const char* str1, const char* str2)
{
while (*str1 == *str2)
{
if (*str1 == '\0')
{
return 0;
}
str1++;
str2++;
}
return *str1 - *str2;
}
int main()
{
char arr1[] = "abg";
char arr2[] = "abg";
char arr3[] = "abcde";
printf("%d ", my_strcmp(arr1, arr2));
printf("%d ", my_strcmp(arr2, arr3));
return 0;
}
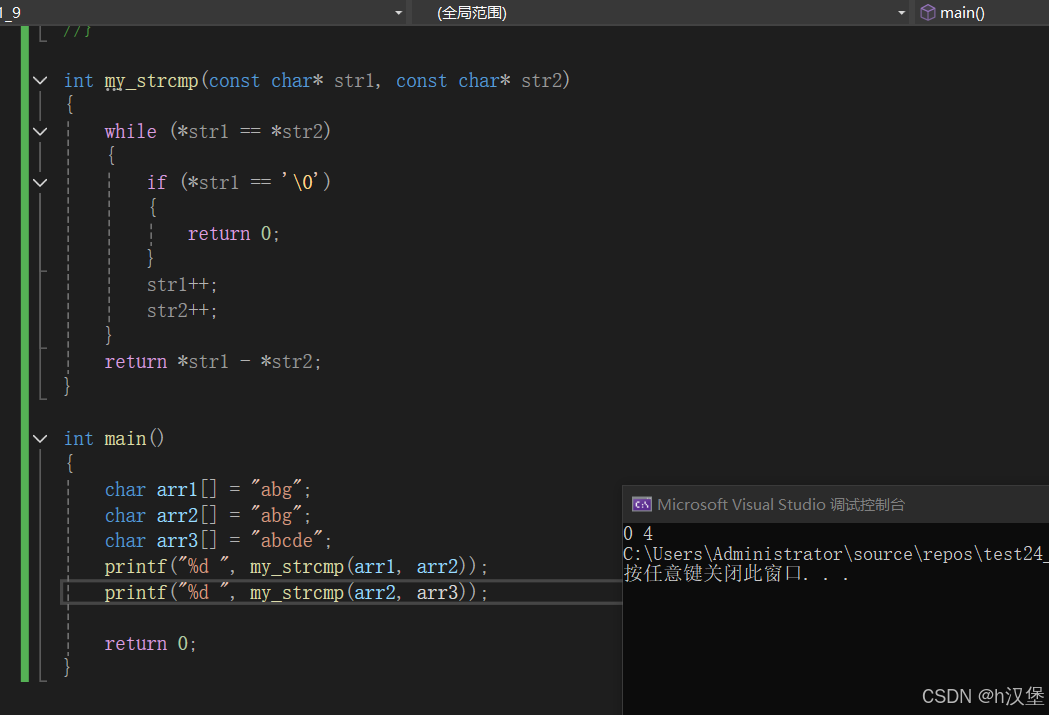
7.strncpy函数的使用
char * strncpy ( char * destination, const char * source, size_t num );
•
Copies the first num characters of source to destination. If the end of the source C string
(which is signaled by a null-character) is found before num characters have been copied,
destination is padded with zeros until a total of num characters have been written to it.
•
拷⻉num个字符从源字符串到⽬标空间。
•
如果源字符串的⻓度⼩于num,则拷⻉完源字符串之后,在⽬标的后边追加0,直到num个。
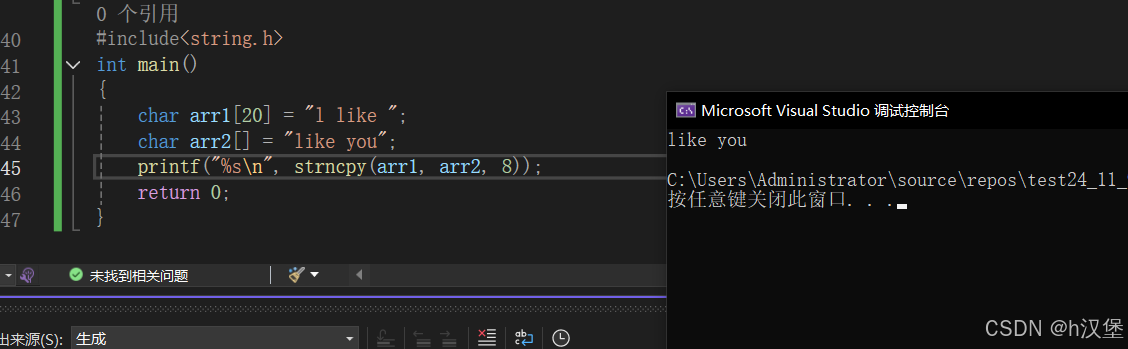
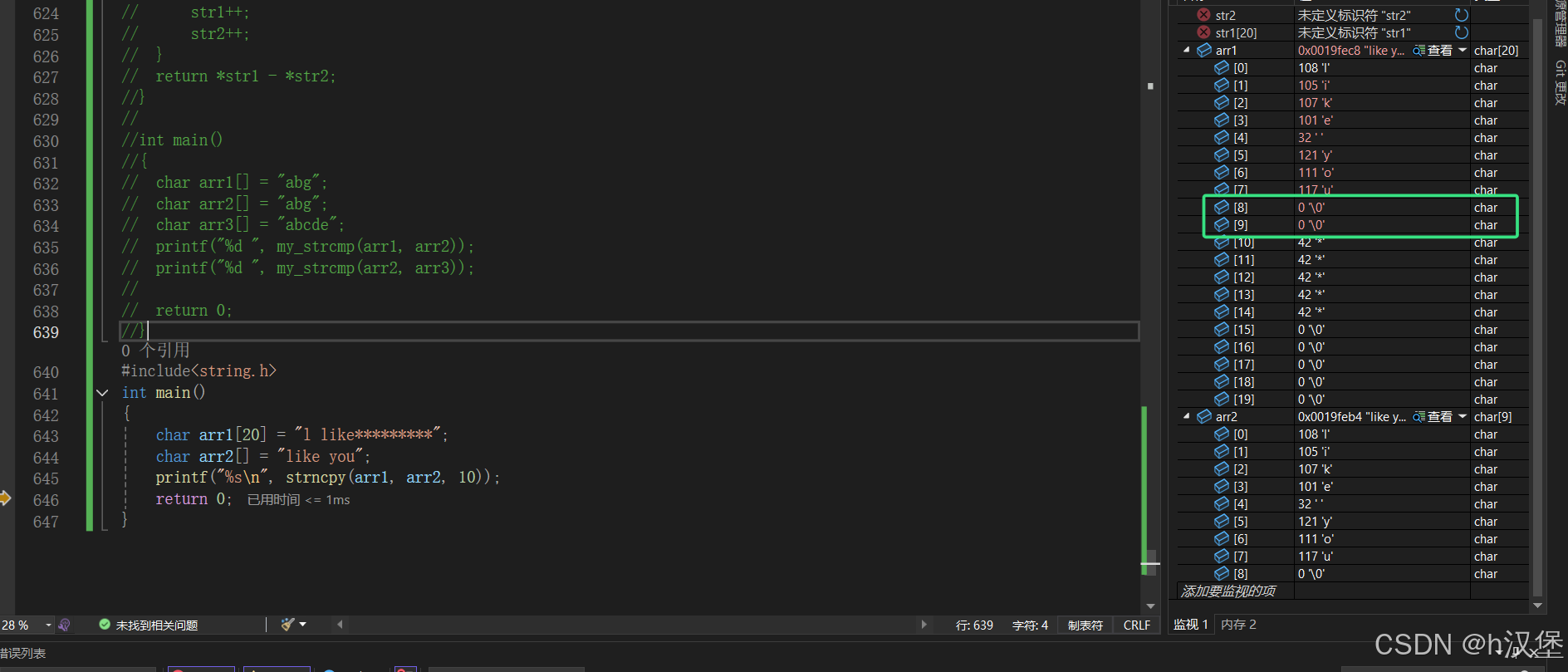
8.strncat
函数的使用
char * strncat ( char * destination, const char * source, size_t num );
•
Appends the first num characters of source to destination, plus a terminating null-character.
(将source指向字符串的前num个字符追加到destination指向的字符串末尾,再追加⼀个
\0
字
符)。
•
If the length of the C string in source is less than num, only the content up to the terminating
null-character is copied.(如果source 指向的字符串的⻓度⼩于num的时候,只会将字符串中到
\0
的内容追加到destination指向的字符串末尾)。
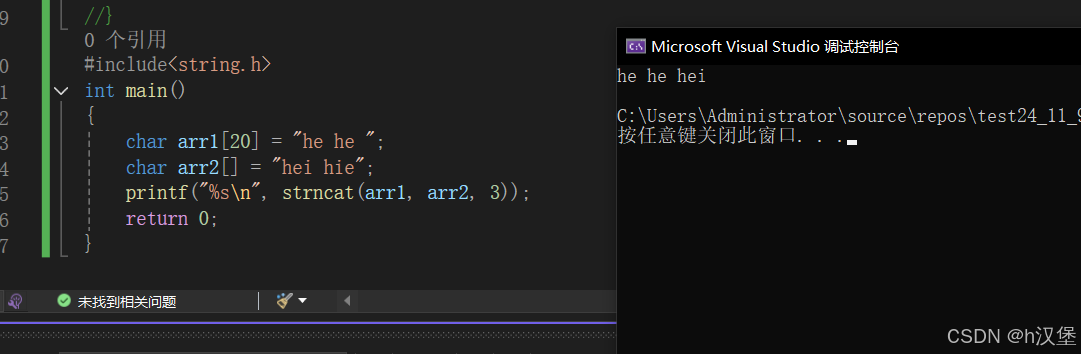
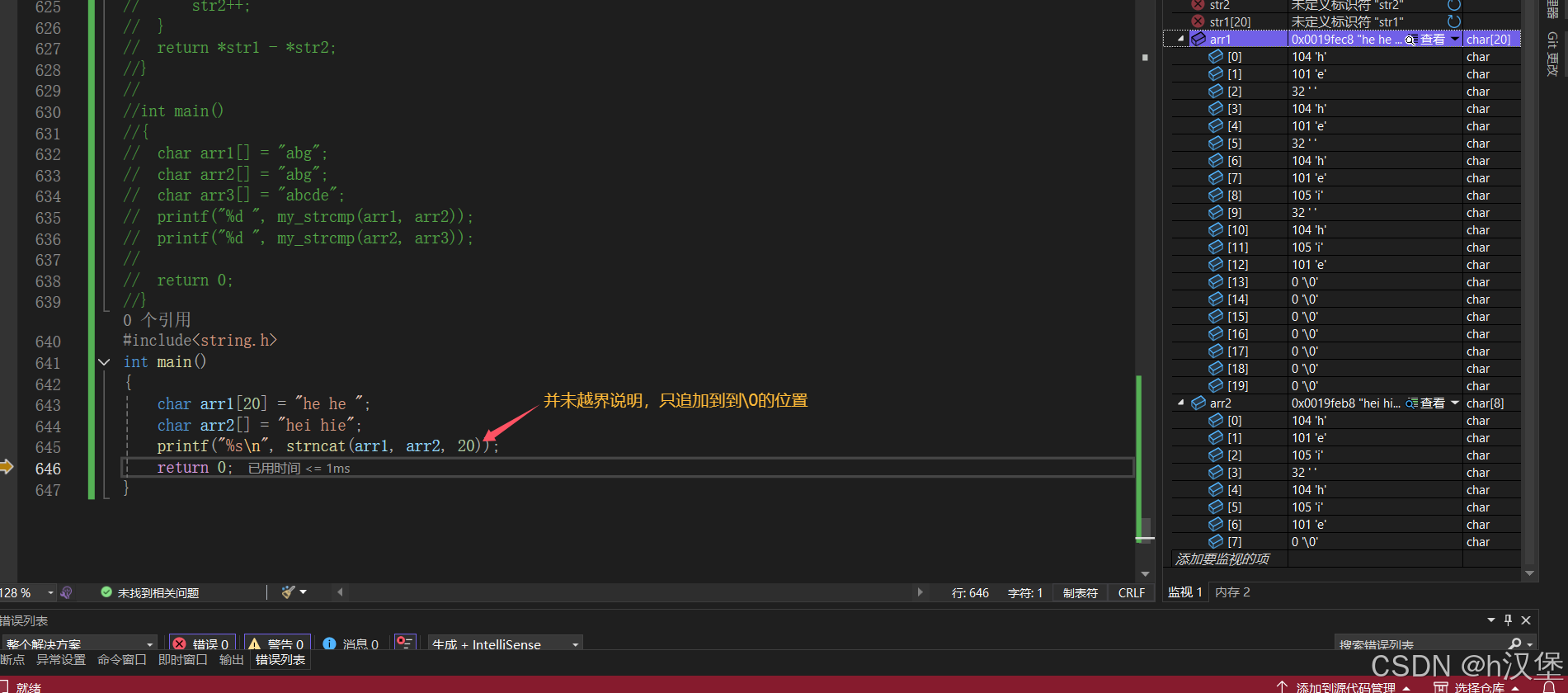
7.strncmp函数的使用
int strncmp ( const char * str1, const char * str2, size_t num );
⽐较str1和str2的前num个字符,如果相等就继续往后⽐较,最多⽐较num个字⺟,如果提前发现不⼀ 样,就提前结束,⼤的字符所在的字符串⼤于另外⼀个。如果num个字符都相等,就是相等返回0.
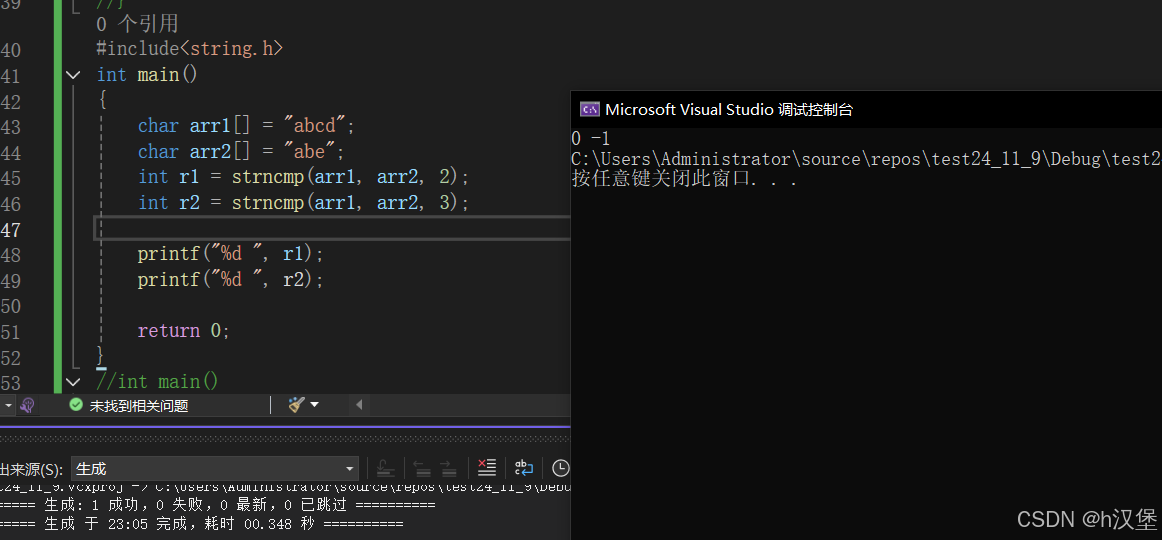
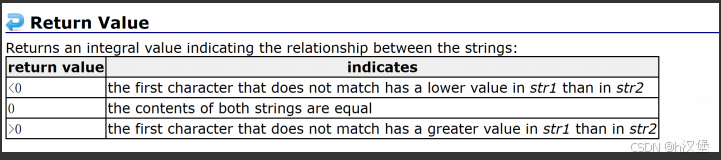
虽然标准是返回大于零小于零的数,但是很多编译器返回的就是0 1 -1
8.strstr的使用和模拟实现
const char * strstr ( const char * str1, const char * str2 );
Returns a pointer to the first occurrence of str2 in str1, or a null pointer if str2 is not part of str1.
(函数返回字符串str2在字符串str1中第⼀次出现的位置)。
The matching process does not include the terminating null-characters, but it stops there.(字符
串的⽐较匹配不包含
\0
字符,以
\0
作为结束标志)。
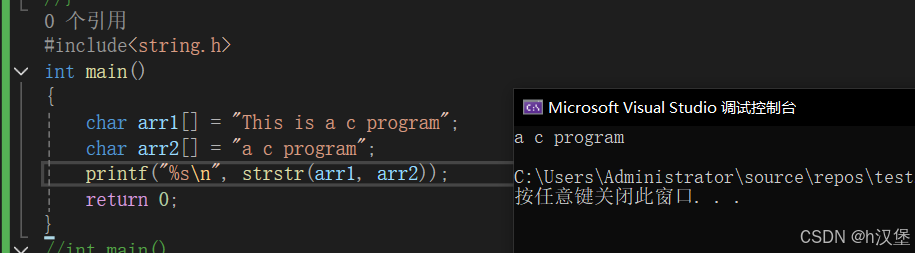
strstr的模拟实现:
#include<string.h>
char* my_strstr(const char* str1, const char* str2)
{
char* cur = (char*)str1;
char *s1, *s2;
if (!*str2 )
{
return cur;
}
while (*cur)
{
s1 = cur;
s2 = (char*)str2;
while (*s1 && *s2 && *s1 == *s2)
{
s1++;
s2++;
}
cur++;
if (!*s2)
{
return cur;
}
}
return NULL;
}
int main()
{
char arr1[] = "This is a c program";
char arr2[] = "a c program";
printf("%s\n", my_strstr(arr1, arr2));
return 0;
}
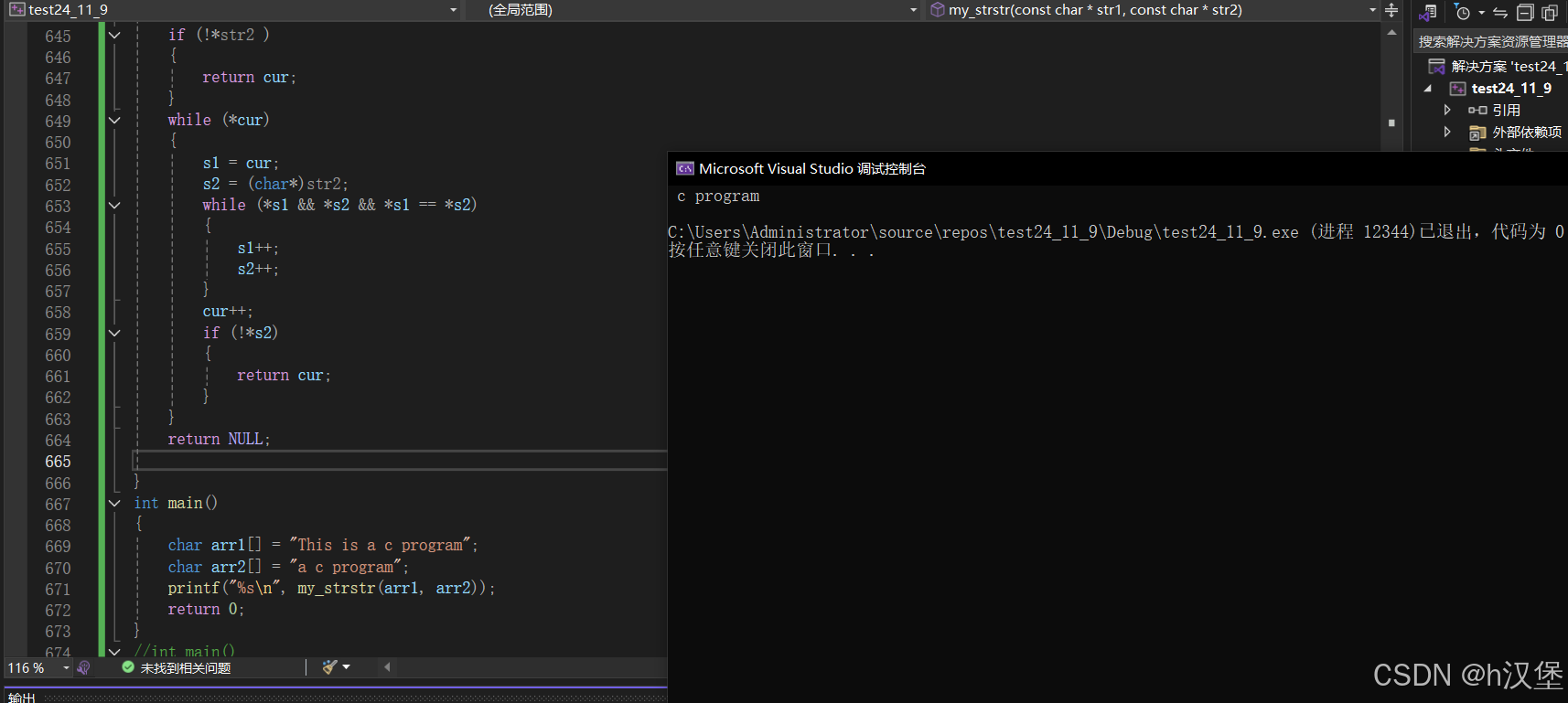