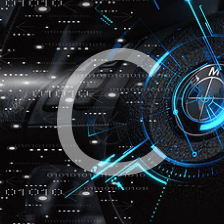
Linked List
文章平均质量分 74
zshouyi
这个作者很懒,什么都没留下…
展开
-
24. Swap Nodes in Pairs
Given a linked list, swap every two adjacent nodes and return its head.For example,Given 1->2->3->4, you should return the list as 2->1->4->3.Your algorithm should use only constant space. Y原创 2016-12-28 12:08:54 · 201 阅读 · 0 评论 -
109. Convert Sorted List to Binary Search Tree
Given a singly linked list where elements are sorted in ascending order, convert it to a height balanced BST.第一种方法,把linkedlist转化成arraylist,使用二分法构建BST。代码如下:/** * Definition for singly-linked lis原创 2017-06-05 02:52:22 · 265 阅读 · 0 评论 -
61. Rotate List
Given a list, rotate the list to the right by k places, where k is non-negative.For example:Given 1->2->3->4->5->NULL and k = 2,return 4->5->1->2->3->NULL.先遍历列表,得到列表长度。用k%len得到实际的step。如果step原创 2017-04-04 11:34:55 · 294 阅读 · 0 评论 -
86. Partition List
Given a linked list and a value x, partition it such that all nodes less than x come before nodes greater than or equal to x.You should preserve the original relative order of the nodes in each of原创 2017-04-04 10:53:02 · 279 阅读 · 0 评论 -
147. Insertion Sort List
Sort a linked list using insertion sort.linked list 的insertion sort,需要记住第一个node,以后的每一个curnode从第一个往后放到合适的位置。代码如下:/** * Definition for singly-linked list. * public class ListNode { * int va原创 2017-03-14 15:04:18 · 271 阅读 · 0 评论 -
328. Odd Even Linked List
Given a singly linked list, group all odd nodes together followed by the even nodes. Please note here we are talking about the node number and not the value in the nodes.You should try to do it in原创 2017-03-14 02:35:21 · 199 阅读 · 0 评论 -
148. Sort List
Sort a linked list in O(n log n) time using constant space complexity.这里采用merge sort。每次把linked list用slow和fast分成两段,再进行merge。代码如下:/** * Definition for singly-linked list. * public class ListNo原创 2017-03-13 09:52:08 · 202 阅读 · 0 评论 -
138. Copy List with Random Pointer
A linked list is given such that each node contains an additional random pointer which could point to any node in the list or null.Return a deep copy of the list.这道题的意思是让完全拷贝一个链表。方法一:第一次遍历原创 2017-03-06 08:46:08 · 267 阅读 · 0 评论 -
142. Linked List Cycle II
Given a linked list, return the node where the cycle begins. If there is no cycle, return null.Note: Do not modify the linked list.Follow up:Can you solve it without using extra space?这道题开原创 2017-01-19 15:33:33 · 269 阅读 · 0 评论 -
83. Remove Duplicates from Sorted List
Given a sorted linked list, delete all duplicates such that each element appear only once.For example,Given 1->1->2, return 1->2.Given 1->1->2->3->3, return 1->2->3.删除已经排序的list的重复val的node,保证原创 2017-01-12 08:18:52 · 273 阅读 · 0 评论 -
203. Remove Linked List Elements
Remove all elements from a linked list of integers that have value val.ExampleGiven: 1 --> 2 --> 6 --> 3 --> 4 --> 5 --> 6, val = 6Return: 1 --> 2 --> 3 --> 4 --> 5Credits:Special thank原创 2017-01-12 08:03:05 · 230 阅读 · 0 评论 -
234. Palindrome Linked List
Given a singly linked list, determine if it is a palindrome.Follow up:Could you do it in O(n) time and O(1) space?判断一个链表是不是构成回文。如果是数组时,我们会从中间开始向两边比较是不是相等。那么换作链表,只能单向访问,怎么办?可以从中间node开始,后半部分进行原创 2017-01-12 07:20:30 · 247 阅读 · 0 评论 -
160. Intersection of Two Linked Lists
Write a program to find the node at which the intersection of two singly linked lists begins.For example, the following two linked lists: A: a1 → a2 ↘原创 2017-01-11 16:46:07 · 217 阅读 · 0 评论 -
141. Linked List Cycle
Given a linked list, determine if it has a cycle in it.Follow up:Can you solve it without using extra space?双指针问题,一个runner 和一个walker,runner 每次向后移动两个,walker 每次向后移动一个。当walker 等于runner 时,说明存在闭环原创 2017-01-11 14:27:44 · 262 阅读 · 0 评论 -
206. Reverse Linked List
Reverse a singly linked list.click to show more hints.Hint:A linked list can be reversed either iteratively or recursively. Could you implement both?这道题可以看看这篇文章的指导:http://algorithms.tutori原创 2017-01-11 14:17:22 · 233 阅读 · 0 评论 -
237. Delete Node in a Linked List
Write a function to delete a node (except the tail) in a singly linked list, given only access to that node.Supposed the linked list is 1 -> 2 -> 3 -> 4 and you are given the third node with val原创 2017-01-10 05:52:09 · 241 阅读 · 0 评论 -
379. Design Phone Directory
Design a Phone Directory which supports the following operations:get: Provide a number which is not assigned to anyone.check: Check if a number is available or not.release: Recycle or release原创 2017-06-16 14:54:07 · 394 阅读 · 0 评论