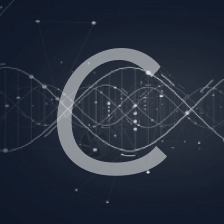
Data Structure and Algorithm
文章平均质量分 67
yangjin_unique
这个作者很懒,什么都没留下…
展开
-
队列(链表实现)
/* 链表队列 * front 为队头指针(链表头节点) * rear 为队尾指针 */ #include #include typedef struct list_t{ int _element; struct list_t *_next; }list_t; /* 要改变一个变量的值,需要传入变量的地址作参数; * 要改变一个指针的值,需要传入该指针的地址作参数(即指针的指针);原创 2012-08-03 09:45:11 · 376 阅读 · 0 评论 -
图深度优先遍历(递归和栈实现)
/* depth-first traversal * two methods: recursive and non-recursive * Graph is stored in an adjacency matrix */ #include #include #define MAX 10 int sp = 0;//stack pointer int stack[MAX]; /***原创 2012-08-07 11:09:49 · 10814 阅读 · 2 评论 -
找出图中的所有连通子图(创建图的邻接表,深度优先遍历查找子图)
/* 利用深度优先遍历,找出图中的所有连通图(子图) * 图用邻接表表示 *graph[], 利用边的信息来创建adjacency lists */ #include #include #define MAXVERTICES 20 #define MAXEDGES 20 typedef enum { FALSE, TRUE, TRISTATE } bool; typede原创 2012-08-08 14:44:06 · 8833 阅读 · 1 评论 -
删除C/C++文件中的注释(c实现)
/**@author: yangjin * * @date: 2012-7-27 * @funciton: delete all comments in a c file */ #include #include main(void) { FILE *fp1, *fp2; char c; if((fp1 = fopen("1.c", "r")) == NULL) { per原创 2012-07-27 20:50:11 · 1560 阅读 · 0 评论 -
Reverse 字符串
#include #include #include void reverseString(char str[]); void exchange(char *a, char *b); int main(int argc, char *argv[]) { //char x = 'a'; //char y = 'b'; char string[100]; if(ar原创 2012-07-27 20:51:49 · 401 阅读 · 0 评论 -
折半查找
#include #include #define NUM 8 int a[NUM] = {1, 2, 4, 5 ,8, 9, 10, 15 }; int binary_search(int number) { int mid, start = 0, end = NUM - 1; while(start <= end) { mid = (end + start) / 2;原创 2012-07-27 21:28:40 · 302 阅读 · 0 评论 -
希尔排序
#include #include void shell_sort(int v[], int n); void printarray(int a[], int n); main(void) { int a[5] = {5,4,3,2,1}; shell_sort(a, 5); } /*shell sort: increment*/ //add some printf to a原创 2012-07-28 15:33:21 · 329 阅读 · 0 评论 -
整形转换成字符串输出
#include #include void y_atoi(int n, char s[]); main(int argc, char *argv[]) { int a = -23456; char str[20]; /* if(argc < 2) { fprintf(stderr, "No arguments!\n"); exit(-1); } */ y_atoi原创 2012-07-28 20:43:09 · 751 阅读 · 0 评论 -
gerp(1):查找文件中指定的字符串,并打印该行
/** @autho: yj * @function: like gerp command in linux: find lines with a specified string in a file, and print these lines. * @usage: ./grep [string] [filename] **/ #include #include #include原创 2012-07-29 09:40:48 · 10322 阅读 · 0 评论 -
内存分配算法-(首次分配法和最佳分配法)
/* implement a memory allocation scheme by using algorithms first-fit, next-fit, and best-fit * freelist为空闲区链表的头,它的下一个节点才指向空闲缓冲区 * freelist是按首地址来排序的,而不是按size来排序。按首地址排序便于相邻空闲内存合并;按size大小来排序,便于分配内存 *原创 2012-08-06 20:49:55 · 7069 阅读 · 0 评论 -
统计输入中所有单词出现的次数(使用二叉查找树实现:递归和非递归)
/*@function:统计输入中的所有单词出现的次数 * @method: 使用二叉查找树。对输入的单词建立一颗二叉查找树,新输入的单词若存在于树中,则增加该节点的计数值;否则,新增一个节点 */ #include #include #include #include #define MAXWORD 100 typedef struct tnode { char word[MAXW原创 2012-08-05 16:22:38 · 1397 阅读 · 0 评论 -
堆排序
#include #include void swap(int *x, int *y) { int temp; temp = *x; *x = *y; *y = temp; } /* ajust position of i-th node */ /* 不断的将i与其子节点比较,将i放入正确的位置 */ void adjust(int list[], int i, int n) { i原创 2012-08-04 21:40:08 · 425 阅读 · 0 评论 -
队列-数组实现
队列元素用{ }圈起来,方便查看原创 2012-08-02 20:27:06 · 304 阅读 · 0 评论 -
循环队列—数组实现
/* circular queue: 牺牲一个存储节点,对头front指示的节点不用于存储数据,起标志性作用 * front: 队头元素的前一个位置 * rear: 队尾元素 */ #include #include #define MAX 10 //size of queue int insertCircularQueue(int queue[], int front,int *re原创 2012-08-02 20:27:53 · 397 阅读 · 0 评论 -
链表基本操作
main.c #include #include #include "list.h" main(void) { int min, max; char item_choice; list_t *head; list_t *new_head; int n; printf("------------------------原创 2012-08-02 20:31:25 · 349 阅读 · 0 评论 -
循环链表
/* a circular linked list is a list in which the link field of the last node is made to * point to the first node of the list. * In the case of circular lists, the empty list also should be circul原创 2012-08-02 20:32:37 · 384 阅读 · 0 评论 -
双向链表
/* keep a head and end node for doubly linked list * Note: here wu should the end node */ #include #include typedef struct _list { int _element; struct _list *_left, *_right; }list_t; lis原创 2012-08-02 20:33:19 · 347 阅读 · 0 评论 -
堆栈(数组实现)
/* array for stack * top: 栈顶指针,指向最后一个元素的下一个空位置 */ #include #include #define MAX 5 //size of queue int stack[MAX]; int top; void push(int stack[], int *top, int value) { if(*top < MAX) { stack原创 2012-08-02 20:54:00 · 591 阅读 · 0 评论 -
优先队列(链表实现)
插入时需要按照优先级从高到低排序,一般操作系统的任务队列调度会用到 /* 优先队列(链表实现) * front 为队头指针(链表头节点) * rear 为队尾指针 */ #include #include typedef struct list_t{ int _element; int _priority; struct list_t *_next; }list_t; /*原创 2012-08-03 10:44:25 · 1768 阅读 · 1 评论 -
binary tree(basic operation)
/** @author: yj * time: 2012/4 * usage: basic function for binary tree */ #include "tree.h" #include #include /** create binary tree parameters: none return: head原创 2012-08-03 11:09:02 · 463 阅读 · 0 评论 -
二叉查找树
/* binary search tree * 1. Any node in the left subtree is less than the root; * 2. Any node in the right subtree is greater than the root; * 3. if we traverse a binary search tree in inorder, we原创 2012-08-03 20:48:54 · 406 阅读 · 0 评论 -
统计输入中关键词出现的次数(二分查找实现)
/* 统计输入的c语言中关键词出现的次数 * @method: 使用二分查找实现,keytab[]关键词必须是按首字母排好序的关键词表 */ #include #include #include #include struct key { char *word; int count; } keytab[] = { "char", 0, "int", 0, "vo原创 2012-08-05 14:51:40 · 1146 阅读 · 0 评论 -
快速排序(K&R)
/**qsort.c: quick sort. divide-and-conquer */ #include void qsort(int v[], int left, int right); main(void) { int i=0; int a[5] = {5,4,3,2,1}; qsort(a, 0, 4); while(i原创 2012-07-30 21:53:27 · 2165 阅读 · 0 评论