#include<stdio.h>
#include<iostream>
#include<algorithm>
#include<cstring>
#include<string>
#include<vector>
#include<map>
#include<stack>
#include<queue>
#include<set>
#define endl '\n'
#define lson rt<<1,l,mid
#define rson rt<<1|1,mid+1,r
#define INF 0x3f3f3f3f
#define sync ios::sync_with_stdio(0);
const int N=100005;
using namespace std;
int L[N*4][12],R[N*4][12],sum[N*4],cnt=0;
int fa[N*10],a[12][N],n,m,q;
int rrs[12],ans;
bool flag;
int find(int x)
{
return x==fa[x]?x:fa[x]=find(fa[x]);
}
void update(int rt,int l,int r)
{
int mid=(l+r)>>1;
int ls=rt<<1,rs=rt<<1|1;
sum[rt]=sum[ls]+sum[rs];
for(int i=1;i<=n;i++)
{
fa[L[ls][i]]=L[ls][i];
fa[L[rs][i]]=L[rs][i];
fa[R[ls][i]]=R[ls][i];
fa[R[rs][i]]=R[rs][i];
}
for(int i=1;i<=n;i++)
{
if(a[i][mid]==a[i][mid+1])
{
int x=find(R[ls][i]),y=find(L[rs][i]);
if(x!=y)
{
sum[rt]--;
fa[y]=x;
}
}
}
for(int i=1;i<=n;i++)
{
L[rt][i]=find(L[ls][i]);
R[rt][i]=find(R[rs][i]);
}
}
void build(int rt,int l,int r)
{
if(l==r)
{
for(int i=1;i<=n;i++)
if(a[i][l]==a[i-1][l]) L[rt][i]=R[rt][i]=L[rt][i-1];
else L[rt][i]=R[rt][i]=++cnt,sum[rt]++;
return;
}
int mid=(l+r)>>1;
build(lson);
build(rson);
update(rt,l,r);
}
void query(int rt,int l,int r,int ll,int rr)
{
if(l>=ll&&r<=rr)
{
if(!flag)
{
flag=true;
ans=sum[rt];
for(int i=1;i<=n;i++) rrs[i]=R[rt][i];
}
else
{
ans+=sum[rt];
for(int i=1;i<=n;i++)
{
fa[rrs[i]]=rrs[i];
fa[L[rt][i]]=L[rt][i];
fa[R[rt][i]]=R[rt][i];
}
for(int i=1;i<=n;i++)
{
if(a[i][l]==a[i][l-1])
{
int x=find(rrs[i]),y=find(L[rt][i]);
if(x!=y)
{
ans--;
fa[x]=y;
}
}
}
for(int i=1;i<=n;i++)
rrs[i]=find(R[rt][i]);
}
return;
}
int mid=(l+r)>>1;
if(ll<=mid) query(lson,ll,rr);
if(rr>mid) query(rson,ll,rr);
}
int main()
{
scanf("%d%d%d",&n,&m,&q);
for(int i=1;i<=n;i++)
for(int j=1;j<=m;j++)
scanf("%d",&a[i][j]);
build(1,1,m);
while(q--)
{
int l,r;
scanf("%d%d",&l,&r);
flag=0;ans=0;
query(1,1,m,l,r);
printf("%d\n",ans);
}
return 0;
}
In his spare time Vladik estimates beauty of the flags.
Every flag could be represented as the matrix n × m which consists of positive integers.
Let's define the beauty of the flag as number of components in its matrix. We call component a set of cells with same numbers and between any pair of cells from that set there exists a path through adjacent cells from same component. Here is the example of the partitioning some flag matrix into components:
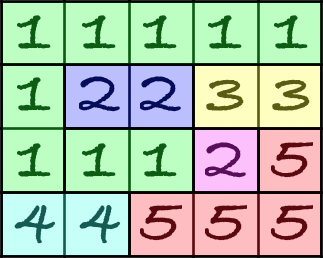
But this time he decided to change something in the process. Now he wants to estimate not the entire flag, but some segment. Segment of flag can be described as a submatrix of the flag matrix with opposite corners at (1, l) and (n, r), where conditions 1 ≤ l ≤ r ≤ m are satisfied.
Help Vladik to calculate the beauty for some segments of the given flag.
First line contains three space-separated integers n, m, q (1 ≤ n ≤ 10, 1 ≤ m, q ≤ 105) — dimensions of flag matrix and number of segments respectively.
Each of next n lines contains m space-separated integers — description of flag matrix. All elements of flag matrix is positive integers not exceeding 106.
Each of next q lines contains two space-separated integers l, r (1 ≤ l ≤ r ≤ m) — borders of segment which beauty Vladik wants to know.
For each segment print the result on the corresponding line.
4 5 4 1 1 1 1 1 1 2 2 3 3 1 1 1 2 5 4 4 5 5 5 1 5 2 5 1 2 4 5
6 7 3 4
Partitioning on components for every segment from first test case: