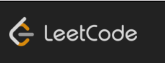
LeetCode
本专栏主要记录Leetcode上刷题过程中对于一道题的不同的解法和使用c++和python两种语言的实现。Github同时更新题解https://github.com/xuna123/LeetCode/tree/master
「已注销」
随缘。。。随缘。。。。。
展开
-
349. Intersection of Two Arrays(两数组的交集,vector中find()函数, 结果集去重)
题目Given two arrays, write a function to compute their intersection.Example: Given nums1 = [1, 2, 2, 1], nums2 = [2, 2], return [2].Note:Each element in the result must be unique.The result c...原创 2018-02-25 23:18:30 · 298 阅读 · 0 评论 -
350. Intersection of Two Arrays II(两数组交集)
题目Given two arrays, write a function to compute their intersection.Example: Given nums1 = [1, 2, 2, 1], nums2 = [2, 2], return [2, 2].Note:Each element in the result should appear as many tim...原创 2018-02-26 00:15:07 · 321 阅读 · 0 评论 -
292. Nim Game(尼姆游戏)
题目You are playing the following Nim Game with your friend: There is a heap of stones on the table, each time one of you take turns to remove 1 to 3 stones. The one who removes the last stone will be...原创 2018-02-26 16:30:29 · 3559 阅读 · 0 评论 -
387. First Unique Character in a String
题目Given a string, find the first non-repeating character in it and return it’s index. If it doesn’t exist, return -1.Examples:s = "leetcode"return 0.s = "loveleetcode",return 2.Note: You ...原创 2018-02-26 17:32:48 · 191 阅读 · 0 评论 -
70. Climbing Stairs(爬楼梯)
题目You are climbing a stair case. It takes n steps to reach to the top.Each time you can either climb 1 or 2 steps. In how many distinct ways can you climb to the top?Note: Given n will be a posi...原创 2018-02-24 11:13:29 · 284 阅读 · 0 评论 -
198. House Robber(动态规划--房屋强盗)
题目You are a professional robber planning to rob houses along a street. Each house has a certain amount of money stashed, the only constraint stopping you from robbing each of them is that adjacent h...原创 2018-02-24 11:47:23 · 1511 阅读 · 0 评论 -
303. Range Sum Query - Immutable(和累加法)
题目Given an integer array nums, find the sum of the elements between indices i and j (i ≤ j), inclusive.Example:Given nums = [-2, 0, 3, -5, 2, -1]sumRange(0, 2) -> 1sumRange(2, 5) -> -1...原创 2018-02-24 12:16:04 · 358 阅读 · 0 评论 -
455. Assign Cookies(分蛋糕--贪心)
题目Assume you are an awesome parent and want to give your children some cookies. But, you should give each child at most one cookie. Each child i has a greed factor gi, which is the minimum size of a...原创 2018-02-24 15:36:05 · 375 阅读 · 0 评论 -
202. Happy Number(unordered_map)
题目Write an algorithm to determine if a number is “happy”.A happy number is a number defined by the following process: Starting with any positive integer, replace the number by the sum of the squar...原创 2018-02-24 17:13:27 · 214 阅读 · 0 评论 -
204. Count Primes(厄拉多塞筛法--质数筛法)
题目Description:Count the number of prime numbers less than a non-negative number, n.题意小于n的素数的个数。题解 普通的筛法求素数超时。 提示中的一种解法:厄拉多塞筛法(https://leetcode.com/problems/count-primes/hints/)西元前2...原创 2018-02-25 22:57:44 · 690 阅读 · 0 评论 -
242. Valid Anagram(有颠倒顺序构成的字符串---字符串排序(c++和python)
题目Given two strings s and t, write a function to determine if t is an anagram of s. s = "anagram", t = "nagaram", return true. s = "rat", t = "car", return false. 题意判断 t 是否是由 s 颠倒顺序构成的...原创 2018-02-25 23:03:37 · 391 阅读 · 0 评论 -
Leetcode#697. Degree of an Array(数组的度)
题目Given a non-empty array of non-negative integers nums, the degree of this array is defined as the maximum frequency of any one of its elements.Your task is to find the smallest possible length o...原创 2018-02-18 23:48:12 · 661 阅读 · 0 评论 -
Leetcode#695. Max Area of Island(岛屿最大面积,深搜dfs)
题目Given a non-empty 2D array grid of 0’s and 1’s, an island is a group of 1’s (representing land) connected 4-directionally (horizontal or vertical.) You may assume all four edges of the grid are su...原创 2018-02-19 16:15:45 · 1161 阅读 · 0 评论 -
Leetcode#746. Min Cost Climbing Stairs(最低花费登楼梯--动态规划)
题目On a staircase, the i-th step has some non-negative cost cost[i] assigned (0 indexed).Once you pay the cost, you can either climb one or two steps. You need to find minimum cost to reach the top...原创 2018-02-19 17:07:20 · 252 阅读 · 0 评论 -
Leetcode#561. Array Partition I
题目Given an array of 2n integers, your task is to group these integers into n pairs of integer, say (a1, b1), (a2, b2), …, (an, bn) which makes sum of min(ai, bi) for all i from 1 to n as large as po...原创 2018-02-19 21:27:12 · 216 阅读 · 0 评论 -
Leetcode#661. Image Smoother
题目Given a 2D integer matrix M representing the gray scale of an image, you need to design a smoother to make the gray scale of each cell becomes the average gray scale (rounding down) of all the 8 s...原创 2018-02-19 21:35:35 · 161 阅读 · 0 评论 -
155. Min Stack(带有获取当前最小值的栈)
题目Design a stack that supports push, pop, top, and retrieving the minimum element in constant time.push(x) -- Push element x onto stack.pop() -- Removes the element on top of the stack.top() -- ...原创 2018-02-21 13:48:20 · 316 阅读 · 0 评论 -
225. Implement Stack using Queues(用队列模拟栈)
题目Implement the following operations of a stack using queues.push(x) – Push element x onto stack.pop() – Removes the element on top of the stack.top() – Get the top element.empty() – Return wh...原创 2018-02-21 14:25:26 · 273 阅读 · 0 评论 -
232. Implement Queue using Stacks(用栈模拟队列)
题目Implement the following operations of a queue using stacks.push(x) -- Push element x to the back of queue.pop() -- Removes the element from in front of queue.peek() -- Get the front element.e...原创 2018-02-23 22:44:30 · 347 阅读 · 0 评论 -
496. Next Greater Element I(下一个更大的元素)
题目You are given two arrays (without duplicates) nums1 and nums2 where nums1’s elements are subset of nums2. Find all the next greater numbers for nums1’s elements in the corresponding places of nums...原创 2018-02-23 23:56:35 · 500 阅读 · 0 评论 -
682. Baseball Game(栈的应用)
题目You’re now a baseball game point recorder.Given a list of strings, each string can be one of the 4 following types:Integer (one round's score): Directly represents the number of points you get...原创 2018-02-24 00:53:17 · 378 阅读 · 0 评论 -
Leetcode#665. Non-decreasing Array(非递减数组)
题目Given an array with n integers, your task is to check if it could become non-decreasing by modifying at most 1 element.We define an array is non-decreasing if array[i] <= array[i + 1] holds f...原创 2018-02-18 21:52:06 · 298 阅读 · 0 评论 -
Leetcode#747. Largest Number At Least Twice of Others
题目In a given integer array nums, there is always exactly one largest element.Find whether the largest element in the array is at least twice as much as every other number in the array.If it is, ...原创 2018-02-18 22:02:55 · 280 阅读 · 0 评论 -
Leetcode#724. Find Pivot Index
题目Given an array of integers nums, write a method that returns the “pivot” index of this array.We define the pivot index as the index where the sum of the numbers to the left of the index is equal...原创 2018-02-18 22:07:56 · 352 阅读 · 0 评论 -
Leetcode#485. Max Consecutive Ones
题目Given a binary array, find the maximum number of consecutive 1s in this array.Example 1:Input: [1,1,0,1,1,1]Output: 3Explanation: The first two digits or the last three digits are consecut原创 2018-02-07 20:11:46 · 219 阅读 · 0 评论 -
Leetcode#605. Can Place Flowers
题目Suppose you have a long flowerbed in which some of the plots are planted and some are not. However, flowers cannot be planted in adjacent plots - they would compete for water and both would die.原创 2018-02-07 21:34:23 · 204 阅读 · 0 评论 -
Leetcode#189. Rotate Array(翻转数组)
题目Rotate an array of n elements to the right by k steps.For example, with n = 7 and k = 3, the array [1,2,3,4,5,6,7] is rotated to [5,6,7,1,2,3,4].Note: Try to come up as many solutions as you ...原创 2018-02-08 14:44:37 · 249 阅读 · 0 评论 -
Leetcode#219. Contains Duplicate II(包含副本II)
题目Given an array of integers and an integer k, find out whether there are two distinct indices i and j in the array such that nums[i] = nums[j] and the absolute difference between i and j is at most...原创 2018-02-08 18:30:18 · 196 阅读 · 0 评论 -
Leetcode#532. K-diff Pairs in an Array(k差异对)
题目Given an array of integers and an integer k, you need to find the number of unique k-diff pairs in the array. Here a k-diff pair is defined as an integer pair (i, j), where i and j are both number...原创 2018-02-09 16:59:57 · 290 阅读 · 0 评论 -
Leetcode#566. Reshape the Matrix(重塑矩阵)
题目In MATLAB, there is a very useful function called ‘reshape’, which can reshape a matrix into a new one with different size but keep its original data.You’re given a matrix represented by a two-d...原创 2018-02-09 17:31:35 · 259 阅读 · 0 评论 -
Leetcode#581. Shortest Unsorted Continuous Subarray(最短未排连续子数组)
题目Given an integer array, you need to find one continuous subarray that if you only sort this subarray in ascending order, then the whole array will be sorted in ascending order, too.You need to f...原创 2018-02-09 18:13:24 · 240 阅读 · 0 评论 -
Leetcode#M628.aximum Product of Three Numbers(三个数最大积)
题目Given an integer array, find three numbers whose product is maximum and output the maximum product.Example 1:Input: [1,2,3]Output: 6Example 2:Input: [1,2,3,4]Output: 24Note:1.The l...原创 2018-02-09 22:22:00 · 232 阅读 · 0 评论 -
Leetcode#643. Maximum Average Subarray I(连续子数组的最大平均值)
题目Given an array consisting of n integers, find the contiguous subarray of given length k that has the maximum average value. And you need to output the maximum average value.Example 1:Input: [1...原创 2018-02-10 14:34:27 · 1292 阅读 · 0 评论 -
Leetcode#674. Longest Continuous Increasing Subsequence(最长连续递增子序列的长度))
题目Given an unsorted array of integers, find the length of longest continuous increasing subsequence (subarray).Example 1:Input: [1,3,5,4,7]Output: 3Explanation: The longest continuous increasi...原创 2018-02-16 21:53:16 · 306 阅读 · 0 评论 -
Leetcode#167. Two Sum II - Input array is sorted(双向查找))
题目Given an array of integers that is already sorted in ascending order, find two numbers such that they add up to a specific target number.The function twoSum should return indices of the two numbers s原创 2018-02-07 15:02:06 · 234 阅读 · 0 评论 -
Leetcode#217. Contains Duplicate(包含副本)
题目Given an array of integers, find if the array contains any duplicates. Your function should return true if any value appears at least twice in the array, and it should return false if every elemen原创 2018-02-07 15:35:27 · 206 阅读 · 0 评论 -
Leetcode#283. Move Zeroes
题目Given an array nums, write a function to move all 0’s to the end of it while maintaining the relative order of the non-zero elements.For example, given nums = [0, 1, 0, 3, 12], after calling you原创 2018-02-07 19:21:29 · 220 阅读 · 0 评论 -
Leetcode#448. Find All Numbers Disappeared in an Array
题目Given an array of integers where 1 ≤ a[i] ≤ n (n = size of array), some elements appear twice and others appear once.Find all the elements of [1, n] inclusive that do not appear in this array.原创 2018-02-07 19:29:41 · 206 阅读 · 0 评论 -
Leetcode#766. Toeplitz Matrix (托普利兹矩阵)
题目A matrix is Toeplitz if every diagonal from top-left to bottom-right has the same element.Now given an M x N matrix, return True if and only if the matrix is Toeplitz.Example 1:Input: matrix原创 2018-02-07 19:40:07 · 1174 阅读 · 0 评论 -
Leetcode#414. Third Maximum Number
题目Given a non-empty array of integers, return the third maximum number in this array. If it does not exist, return the maximum number. The time complexity must be in O(n).Example 1:Input: [3, 2,原创 2018-02-07 19:54:46 · 197 阅读 · 0 评论