import random
import heapq
from copy import deepcopy
# 生成迷宫
def generate_maze(width, height):
maze = [[1] * (2 * width + 1) for _ in range(2 * height + 1)]
stack = [(1, 1)]
maze[1][1] = 0
while stack:
x, y = stack[-1]
directions = [(2, 0), (-2, 0), (0, 2), (0, -2)]
random.shuffle(directions)
found = False
for dx, dy in directions:
nx, ny = x + dx, y + dy
if 0 < nx < 2 * width + 1 and 0 < ny < 2 * height + 1 and maze[ny][nx] == 1:
maze[y + dy // 2][x + dx // 2] = 0
maze[ny][nx] = 0
stack.append((nx, ny))
found = True
break
if not found:
stack.pop()
return maze
# 计算曼哈顿距离
def heuristic(a, b):
return abs(a[0] - b[0]) + abs(a[1] - b[1])
# A* 算法寻路
def a_star(maze, start, end):
open_list = []
heapq.heappush(open_list, (0, start))
came_from = {}
g_score = {start: 0}
f_score = {start: heuristic(start, end)}
while open_list:
_, current = heapq.heappop(open_list)
if current == end:
path = []
while current in came_from:
path.append(current)
current = came_from[current]
path.append(start)
path.reverse()
return path
x, y = current
neighbors = [(x + 1, y), (x - 1, y), (x, y + 1), (x, y - 1)]
for neighbor in neighbors:
nx, ny = neighbor
if 0 <= nx < len(maze[0]) and 0 <= ny < len(maze) and maze[ny][nx] == 0:
tentative_g_score = g_score[current] + 1
if neighbor not in g_score or tentative_g_score < g_score[neighbor]:
came_from[neighbor] = current
g_score[neighbor] = tentative_g_score
f_score[neighbor] = tentative_g_score + heuristic(neighbor, end)
heapq.heappush(open_list, (f_score[neighbor], neighbor))
return None
# 打印迷宫
def print_maze(maze, path=None):
maze_copy = deepcopy(maze)
if path:
for x, y in path:
maze_copy[y][x] = 2
for row in maze_copy:
for cell in row:
if cell == 0:
print(' ', end=' ')
elif cell == 1:
print('█', end=' ')
elif cell == 2:
print('*', end=' ')
print()
# 主函数
def main():
width = 10
height = 10
maze = generate_maze(width, height)
start = (1, 1)
end = (2 * width - 1, 2 * height - 1)
path = a_star(maze, start, end)
print("生成的迷宫:")
print_maze(maze)
if path:
print("\nAI 找到的路径:")
print_maze(maze, path)
print("\n记录的路径:", path)
else:
print("\n未找到路径。")
if __name__ == "__main__":
main()
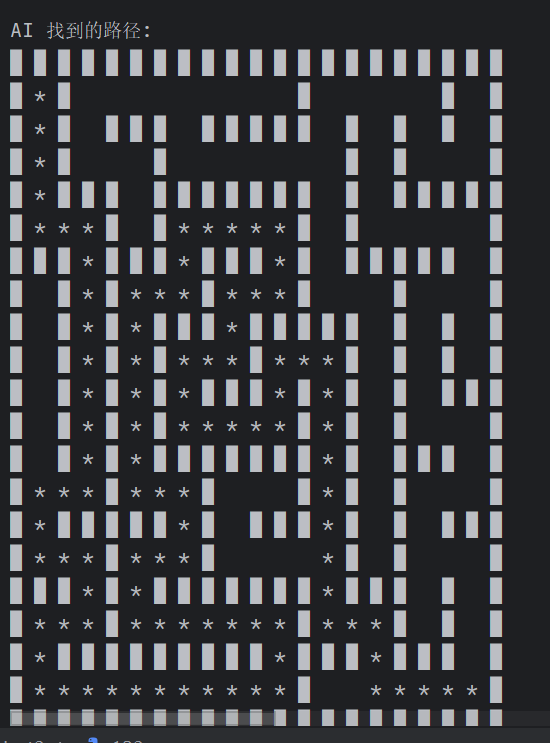