2121SC@SDUSC
一、storm实时计算系统中的tuple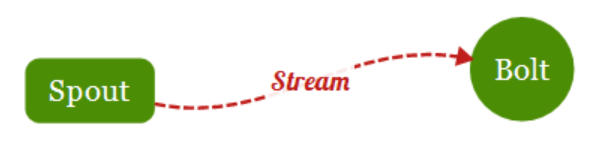
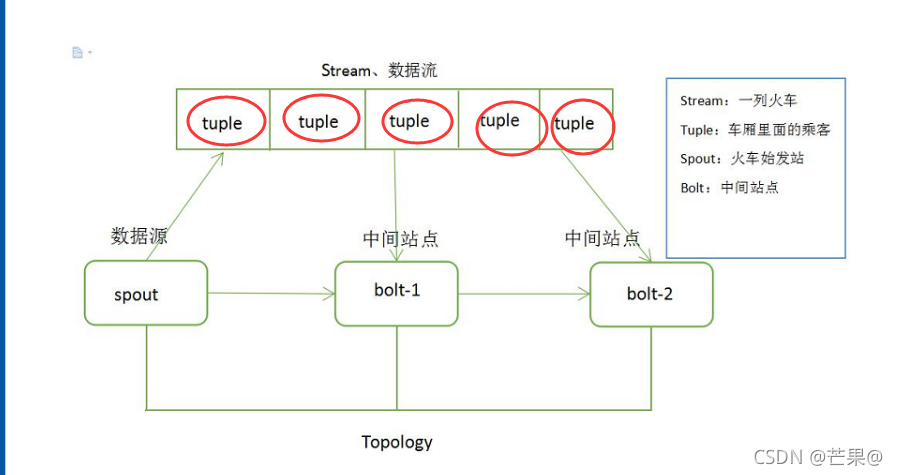
storm中每一个Topology(任务)要从数据源中获取数据,然后进行后续处理。在Topology中从外部数据源获取数据的组件,称之为Spout,处理数据的组件,称之为bolt。
如上图,在这个Topology中,我们看到一个Spout和一个Bolt,两者之间的这个数据通道我们称之为Stream(流)。tuple是Stream的最小组成单元,也可以看成topology的每一个“消息”。tuple是storm的主要数据结构,并且是storm中使用的最基本单元、数据模型和元组,下面对tuple源码进行简要分析。
二、ITuple接口
ITuple是最顶层接口,定义了元组应该支持的最基本的方法
public interface ITuple {
// 返回tuple中的数据
int size();
/**
* 如果元组包括这个具体的field就返回true
*/
boolean contains(String field);
/**
*获取这个元组中的文件名
*/
Fields getFields();
/**
* 返回文件位置
*
* @throws IllegalArgumentException - if field does not exist
*/
int fieldIndex(String field);
/**
* 列出一个元组序列基于 fields selector.
*/
List<Object> select(Fields selector);
/**
* 获取第i个数据
* @throws IndexOutOfBoundsException - if the index is out of range `(index < 0 || index >= size())`
*/
Object getValue(int i);
/**
* 返回元组第i位的field
* @throws ClassCastException If that field is not a String
* @throws IndexOutOfBoundsException - if the index is out of range `(index < 0 || index >= size())`
*/
String getString(int i);
/**
* 获取元组第i位的数据,且数据类型是int
* @throws ClassCastException If that field is not a Integer
* @throws IndexOutOfBoundsException - if the index is out of range `(index < 0 || index >= size())`
*/
Integer getInteger(int i);
/**
*获取元组第i位的数据,且数据类型是long
*
* @throws ClassCastException If that field is not a Long
* @throws IndexOutOfBoundsException - if the index is out of range `(index < 0 || index >= size())`
*/
Long getLong(int i);
/**
*获取元组第i位的数据,且数据类型是Boolean
*
* @throws ClassCastException If that field is not a Boolean
* @throws IndexOutOfBoundsException - if the index is out of range `(index < 0 || index >= size())`
*/
Boolean getBoolean(int i);
/**
* 获取元组第i位的数据,且数据类型是short
* @throws ClassCastException If that field is not a Short
* @throws IndexOutOfBoundsException - if the index is out of range `(index < 0 || index >= size())`
*/
Short getShort(int i);
/**
* 获取元组第i位的数据,且数据类型是byte
*
* @throws ClassCastException If that field is not a Byte
* @throws IndexOutOfBoundsException - if the index is out of range `(index < 0 || index >= size())`
*/
Byte getByte(int i);
/**
* 获取元组第i位的数据,且数据类型是double
*
* @throws ClassCastException If that field is not a Double
* @throws IndexOutOfBoundsException - if the index is out of range `(index < 0 || index >= size())`
*/
Double getDouble(int i);
/**
* 获取元组第i位的数据,且数据类型是float
*
* @throws ClassCastException If that field is not a Float
* @throws IndexOutOfBoundsException - if the index is out of range `(index < 0 || index >= size())`
*/
Float getFloat(int i);
/**
* 获取元组中的数组
* @throws ClassCastException If that field is not a byte array
* @throws IndexOutOfBoundsException - if the index is out of range `(index < 0 || index >= size())`
*/
byte[] getBinary(int i);
/**
* 获取具有特定名称的字段。返回对象,因为元组是动态类型的
*
* @throws IllegalArgumentException - if field does not exist
*/
Object getValueByField(String field);
/**
* Gets the String field with a specific name.
*
* @throws ClassCastException If that field is not a String
* @throws IllegalArgumentException - if field does not exist
*/
String getStringByField(String field);
/**
* 获取具有特定名称的Integer字段。
*
* @throws ClassCastException If that field is not an Integer
* @throws IllegalArgumentException - if field does not exist
*/
Integer getIntegerByField(String field);
/**
* 获取具有特定名称的Long字段。
*
* @throws ClassCastException If that field is not a Long
* @throws IllegalArgumentException - if field does not exist
*/
Long getLongByField(String field);
/**
* 获取具有特定名称的布尔字段。
*
* @throws ClassCastException If that field is not a Boolean
* @throws IllegalArgumentException - if field does not exist
*/
Boolean getBooleanByField(String field);
Short getShortByField(String field);
Byte getByteByField(String field);
Double getDoubleByField(String field);
Float getFloatByField(String field);
/**
*获取具有特定名称的字节数组字段。
*
* @throws ClassCastException If that field is not a byte array
* @throws IllegalArgumentException - if field does not exist
*/
byte[] getBinaryByField(String field);
/**
* 获取此元组中的所有值。
*/
List<Object> getValues();
}
参考文件:
storm知识点学习:http://www.tianshouzhi.com/api/tutorials/storm/52
tuple详解:https://www.cnblogs.com/zlslch/p/5989281.html