示例图片
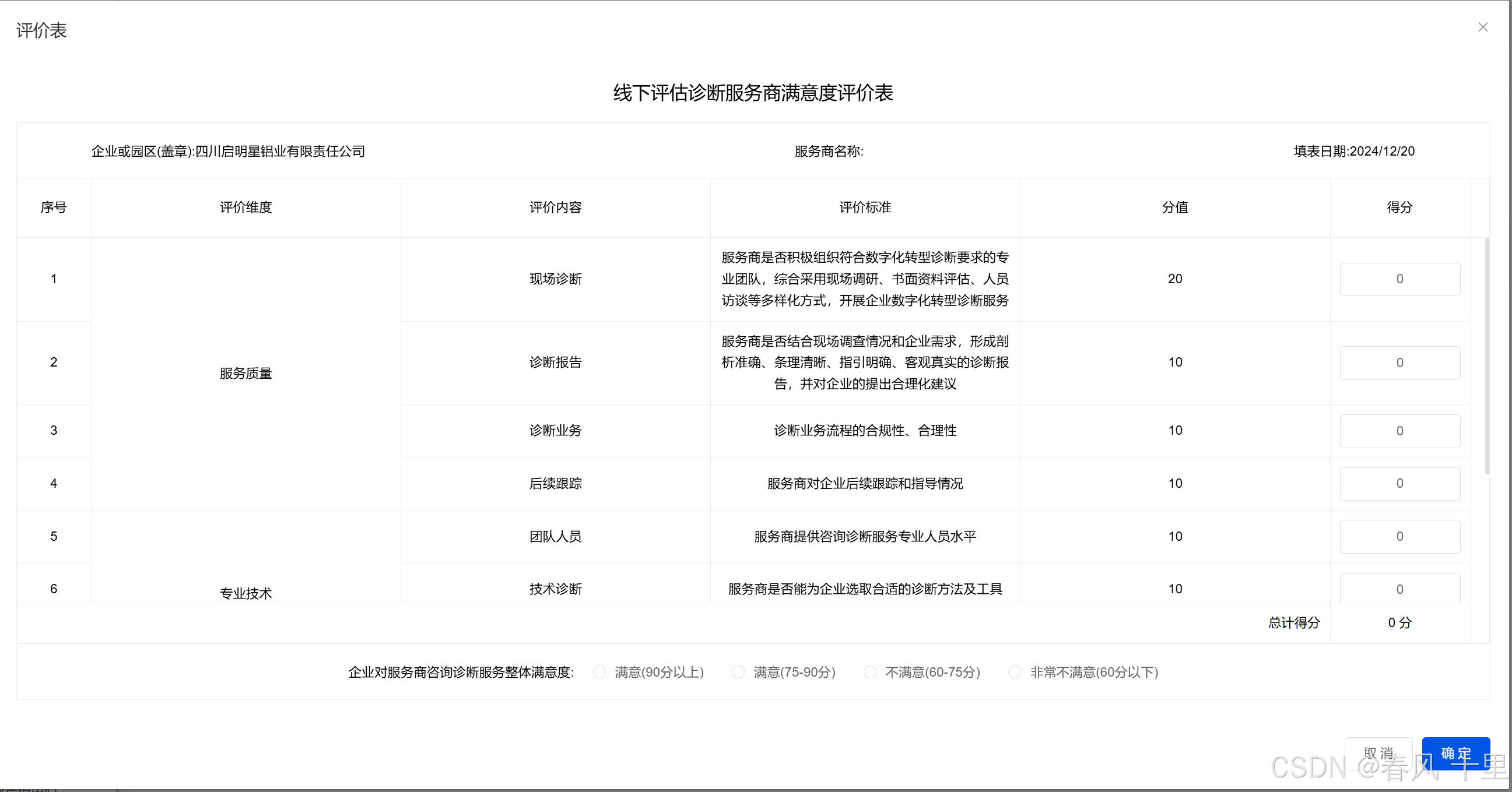
代码示例
<template>
<div class="evaluate_table">
<div class="title-text">线下评估诊断服务商满意度评价表</div>
<div class="name-title">
<span v-for="item in nameTextList" :key="item.name">{{ item.name + item.text }}</span>
</div>
<el-table :data="tableData" border height="500" :span-method="objectSpanMethod" :summary-method="getSummaries"
show-summary style="width: 100%;">
<el-table-column align="center" type="index" label="序号" width="80" />
<el-table-column align="center" prop="dimensionality" label="评价维度">
</el-table-column>
<el-table-column align="center" prop="content" label="评价内容">
</el-table-column>
<el-table-column align="center" prop="standard" label="评价标准">
</el-table-column>
<el-table-column align="center" prop="scoreValue" label="分值">
</el-table-column>
<el-table-column prop="score" align="center" width="150" label="得分">
<template slot-scope="scope">
<el-input-number :controls="false" :min="0" :max="scope.row.scoreValue" v-model="scope.row.score"
style="width: 100%;" />
</template>
</el-table-column>
</el-table>
<div class="satisfied-text">
<span>企业对服务商咨询诊断服务整体满意度:</span>
<el-radio-group v-model="form.radio" style="margin-left: 20px;">
<el-radio v-for="item in satisfiedList" :key="item.value" :label="item.label" :value="item.value">{{ item.label
}}</el-radio>
</el-radio-group>
</div>
</div>
</template>
<script>
export default {
data() {
return {
loading: false,
tableData: [
{
dimensionality: '服务质量',
content: '现场诊断',
standard: '服务商是否积极组织符合数字化转型诊断要求的专业团队,综合采用现场调研、书面资料评估、人员访谈等多样化方式,开展企业数字化转型诊断服务',
scoreValue: 20,
score: null
},
{
dimensionality: '服务质量',
content: '诊断报告',
standard: '服务商是否结合现场调查情况和企业需求,形成剖析准确、条理清晰、指引明确、客观真实的诊断报告,并对企业的提出合理化建议',
scoreValue: 10,
score: null
},
{
dimensionality: '服务质量',
content: '诊断业务',
standard: '诊断业务流程的合规性、合理性',
scoreValue: 10,
score: null
},
{
dimensionality: '服务质量',
content: '后续跟踪',
standard: '服务商对企业后续跟踪和指导情况',
scoreValue: 10,
score: null
},
{
dimensionality: '专业技术',
content: '团队人员',
standard: '服务商提供咨询诊断服务专业人员水平',
scoreValue: 10,
score: null
},
{
dimensionality: '专业技术',
content: '技术诊断',
standard: '服务商是否能为企业选取合适的诊断方法及工具',
scoreValue: 10,
score: null
},
{
dimensionality: '专业技术',
content: '综合分析',
standard: '服务商是否能对企业数字化转型的整体和局部水平开展综合诊断及精准的痛点分析',
scoreValue: 10,
score: null
},
{
dimensionality: '项目成效',
content: '企业适配度',
standard: '服务商出具咨询诊断报告对帮助企业智改数转产生的效果',
scoreValue: 10,
score: null
},
{
dimensionality: '项目成效',
content: '企业转型意愿',
standard: '在服务商完成咨询诊断服务后,企业对数字化转型是否形成有效认知,并具有开展数字化转型的意愿',
scoreValue: 10,
score: null
},
],
nameTextList: [{
name: '企业或园区(盖章):',
text: ''
}, {
name: '服务商名称:',
text: ''
}, {
name: '填表日期:',
text: ''
},],
satisfiedList: [{
label: '满意(90分以上)',
value: 1
}, {
label: '满意(75-90分)',
value: 2
}, {
label: '不满意(60-75分)',
value: 3
}, {
label: '非常不满意(60分以下)',
value: 4
}],
form: {
radio: null
}
}
},
created() {
this.tableArr({ key: 'dimensionality' })
},
methods: {
tableArr(config) {
this.spanArr = [];
let pos = 0;
const { key } = config;
this.tableData.forEach((item, index) => {
if (index === 0) {
this.spanArr.push(1);
pos = 0;
} else {
if (this.tableData[index][key] === this.tableData[index - 1][key]) {
this.spanArr[pos] += 1;
this.spanArr.push(0);
} else {
this.spanArr.push(1);
pos = index;
}
}
});
},
objectSpanMethod({ row, column, rowIndex, columnIndex }) {
if (columnIndex === 1) {
const _row = this.spanArr[rowIndex];
const _col = _row > 0 ? 1 : 0;
return [_row, _col];
}
},
getSummaries(param) {
const { columns, data } = param;
const sums = [];
columns.forEach((column, index) => {
if (index === 4) {
sums[index] = '总计得分';
return;
}
const values = data.map(item => Number(item[column.property]));
if (!values.every(value => isNaN(value)) && index === 5) {
sums[index] = values.reduce((prev, curr) => {
const value = Number(curr);
if (!isNaN(value)) {
return prev + curr;
} else {
return prev;
}
}, 0);
sums[index] += ' 分';
} else {
sums[index] = '';
}
this.$nextTick(() => {
const tds = document.querySelectorAll(
".el-table__footer-wrapper .has-gutter tr>td"
);
tds[0].style.display = "none";
tds[1].style.display = "none";
tds[2].style.display = "none";
tds[3].style.display = "none";
tds[4].style.textAlign = "right";
tds[4].colSpan = 5;
});
});
return sums;
},
reset(row) {
if (row) {
this.nameTextList[0].text = row.deptName || ''
this.nameTextList[1].text = row.serviceProviderName || ''
this.nameTextList[2].text = new Date().toLocaleDateString()
}
this.form.radio = null
this.tableData.forEach(item => {
item.score = null
})
}
}
}
</script>
<style lang="scss" scoped>
.evaluate_table {
font-size: 14px;
color: #000;
.title-text {
text-align: center;
font-size: 20px;
padding: 0 20px 20px;
}
.name-title {
width: 100%;
display: flex;
justify-content: space-between;
padding: 20px 80px;
border: 1px solid #EBEEF5;
border-bottom: none;
}
::v-deep .el-table__cell {
background: #fff !important;
.cell {
color: #000 !important;
font-weight: 400 !important;
}
}
::v-deep .el-table__body-wrapper::-webkit-scrollbar {
width: 6px;
height: 6px;
}
::v-deep .el-table__body-wrapper::-webkit-scrollbar-thumb {
background-color: #ddd;
border-radius: 3px;
}
.satisfied-text {
text-align: center;
padding: 20px;
border: 1px solid #EBEEF5;
border-top: none;
}
}
</style>
获取总分
submitForm() {
const { form, tableData } = this.$refs.evaluateTable
const sumNum = tableData.reduce((prev, curr) => {
return prev + curr.score
}, 0)
console.log(form,sumNum);
}