js魔术字符串
Recently I’ve been working with Next.js to create a teaching tool for elementary school music classrooms. This is the first time I’ve used Next’s parent company, Vercel (formerly Zeit.co), for deployments. If you aren’t familiar, here’s how Vercel describes themselves:
最近,我一直在与Next.js合作,为小学音乐教室创建教学工具。 这是我第一次使用Next的母公司Vercel(以前称为Zeit.co)进行部署。 如果您不熟悉,以下是Vercel描述自己的方式:
Vercel is a cloud platform for static sites and Serverless Functions that fits perfectly with your workflow. It enables developers to host Jamstack websites and web services that deploy instantly, scale automatically, and requires no supervision, all with no configuration.
Vercel是一个用于静态站点和无服务器功能的云平台,非常适合您的工作流程。 它使开发人员可以托管Jamstack网站和Web服务,这些网站和Web服务可以立即部署,自动扩展,并且无需监管,而且都无需配置。
So far I’ve been super pleased; I don’t think I’ve ever had an easier CI/CD experience. I just push my changes, and boom, they’re in prod. Setup was super easy.
到目前为止,我一直感到非常高兴。 我认为我从未有过更轻松的CI / CD体验。 我只是推动我的变化,繁荣发展,它们在生产中。 安装非常简单。
However, once I started passing JSON Web Tokens (JWTs) from the client side to the backend hosted on Firebase / Google Cloud Platform (GCP), I started running into some issues. This was because I was using a CORS policy to interface from client to server, which wasn’t passing the tokens properly.
但是,一旦我开始将JSON Web令牌(JWT)从客户端传递到Firebase / Google Cloud Platform(GCP)上托管的后端,便开始遇到一些问题。 这是因为我正在使用CORS策略从客户端到服务器进行接口,但未正确传递令牌。
It made more sense at this point to set up a proxy instead, so that all routes starting with something like /api
or /ol
would get routed to my Firebase backend. In this way, the tokens would be passed easily without setting up an OPTIONS method for every endpoint.
在这一点上,更有意义的是设置代理,这样所有以/ api
或/ol
路由都将路由到我的Firebase后端。 这样,无需为每个端点设置OPTIONS方法即可轻松传递令牌。
I created this server.js file below, and changed my dev script to run this server file with"dev": "node server.js"
. Key points are explicitly initializing the next.js app on line 20, and adding a proxy with HTTP Proxy Middleware (HPM) on line 27.
我在下面创建了这个server.js文件,并更改了我的dev脚本以使用"dev": "node server.js"
运行该服务器文件。 关键是在第20行显式初始化next.js应用程序,并在第27行添加具有HTTP代理中间件(HPM)的代理。
This actually works great for local development. I’ve even used a very similar setup for sites that had a depoloyed server.js file in the past. But, the point of using something like Vercel is that it removes the hassle of setting up servers like this in production!
这实际上对本地发展非常有用。 对于过去曾经部署过server.js文件的网站,我什至使用了非常相似的设置。 但是,使用Vercel之类的东西的目的在于,它消除了在生产环境中设置此类服务器的麻烦!
I tried messing around with the config to have my start command be "start": "NODE_ENV=production node server.js"
, but only found errors when deploying. I also messed around with creating a vercel.json
config file. No luck. It was time to take a step back.
我尝试弄乱配置,以使启动命令为"start": "NODE_ENV=production node server.js"
,但仅在部署时发现错误。 我还弄乱了创建一个vercel.json
配置文件。 没运气。 现在该退后一步了。
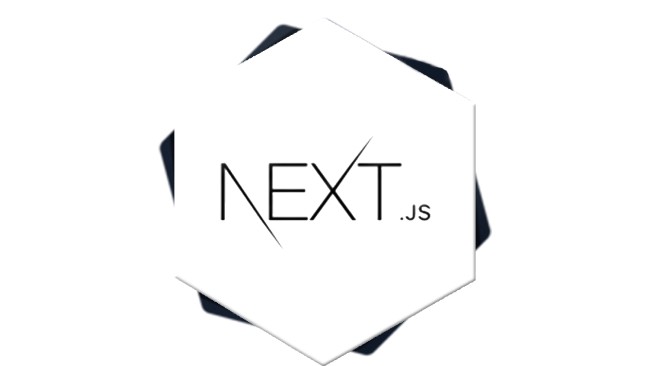
The beauty of Next.js is that creating new routes for site pages is incredibly straightforward. If you want a new page for your site on /info, you simply create a file that exports a React component in the /pages directory called “info”, like pages/info.jsx
.
Next.js的优点在于,为网站页面创建新路由非常简单。 如果您想在/ info上为您的站点创建一个新页面,只需在/ pages目录中创建一个导出React组件的文件,该文件称为“ info”,例如pages/info.jsx
。
If you want to build an API route, it’s similar, except that you create a file in the /api directory, for the route you want to create. For example, a file called api/quizzes.js
could be a GET endpoint which retrieves all quizzes.
如果要构建API路由,除了在/ api目录中为要创建的路由创建文件之外,其他方法都相似。 例如,一个名为api/quizzes.js
的文件可以是一个GET端点,用于检索所有测验。
This would be fine, but my backend was already hosted through Firebase and written in TS instead of JS, and I honestly don’t like the idea of keeping the orchestration and ui layer so close in the code.
这样做很好,但是我的后端已经通过Firebase托管,并且是用TS而不是JS编写的,老实说,我不喜欢在代码中保持业务流程和ui层如此紧密的想法。
The solution I stumbled upon was a combination of using the /api feature of Next.js with HPM:
我偶然发现的解决方案是结合使用Next.js的/ api功能和HPM:
Note that the file name is […args].js in the /api directory, which will accept anything that starts with /api and apply this proxy middleware.
请注意,/ api目录中的文件名为[…args] .js,它将接受以/ api开头的任何内容并应用此代理中间件。
This code is more concise, and and removes the manual setup of Next. Best of all, deploying it with Vercel was seamless.
此代码更加简洁,并且删除了Next的手动设置。 最重要的是,与Vercel一起部署是无缝的。
翻译自: https://medium.com/@patrick.krisko/proxy-magic-with-next-js-vercel-360a42350702
js魔术字符串