如何安装svelte
This tutorial is for anyone who creates single-page-apps and wants to know how to configure routing properly on the server side. It will use Svelte as the framework, but the techniques here will work for any app using client side routing.
本教程适用于创建单页应用程序并且想知道如何在服务器端正确配置路由的任何人。 它将使用Svelte作为框架,但是此处的技术将适用于所有使用客户端路由的应用程序。
The Problem
问题
Client-side routing solutions like svelte-routing
work great, but they will break if you try to refresh your page when using them, and this means that it’s impossible to use hot reloading. This is because all routing logic is performed on the client, and when you initially load a page, say localhost:5000/home
, the client code isn’t yet loaded. This means that your browser will attempt to find the file home.html
at localhost:5000
but it won’t be there, because it’s a single page app. It only has index.html
.
像svelte-routing
这样的客户端路由解决方案效果很好,但是如果您在使用它们时尝试刷新页面,它们将会中断,这意味着不可能使用热重装。 这是因为所有路由逻辑都是在客户端上执行的,并且当您最初加载页面(例如localhost:5000/home
,尚未加载客户端代码。 这意味着您的浏览器将尝试在localhost:5000
处找到文件home.html
,但由于它是一个单页应用程序,因此不会在其中。 它只有index.html
。
Here’s an example.
这是一个例子。
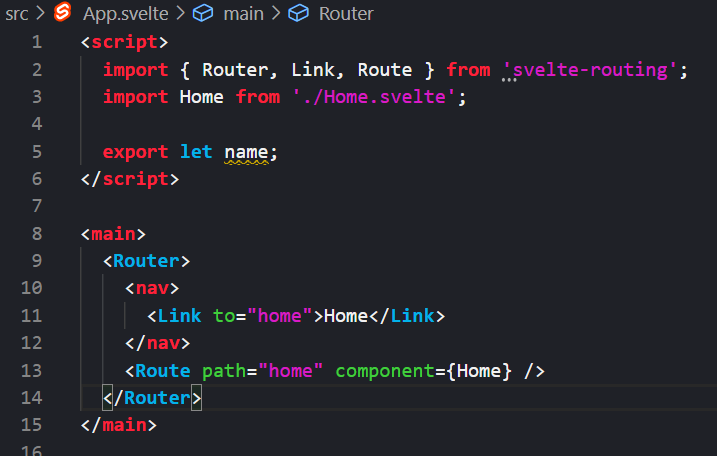
The code above creates a simple Svelte app and uses svelte-routing
as the routing solution. It then creates a Route
for the Home
component and a link to navigate for it. The Home
component only returns a p
tag that says ‘Hello, this is the homepage’.
上面的代码创建了一个简单的Svelte应用程序,并使用svelte-routing
作为路由解决方案。 然后,它为Home
组件创建一个Route
并为其导航。 Home
组件仅返回一个p
标记,上面写着“您好,这是首页”。
When I load up this app, I see the root page.
加载此应用程序时,我会看到根页面。
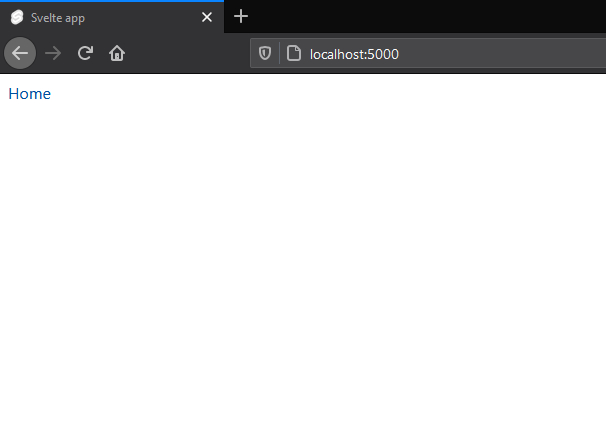
And when I click Home
, I am routed to the Home page.
当我单击Home
,我将转到首页。
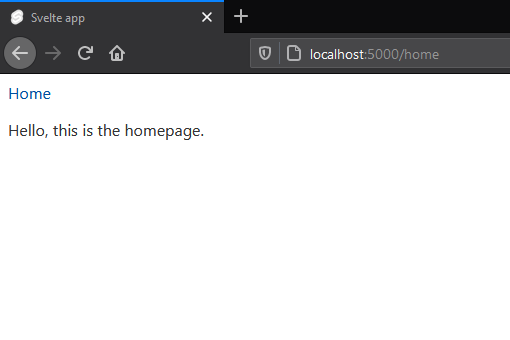
The problem arises when I attempt to navigate to localhost:5000/home
directly, or when I refresh the page. If I do that, I’ll see a blank page, or a Not Found error.
当我尝试直接导航到localhost:5000/home
或刷新页面时,就会出现问题。 如果这样做,我会看到空白页或未找到错误。
The Solution
解决方案
To solve this problem, we need a way of intercepting the HTTP calls to localhost:5000
and then redirecting them all back to index.html
. When we do this, the server will return the root page, which will load all of our code, and then the routing will kick into action and display the home page.
要解决此问题,我们需要一种方法来拦截对localhost:5000
的HTTP调用,然后将它们全部重定向回index.html
。 当我们这样做时,服务器将返回根页面,该页面将加载我们的所有代码,然后路由将开始执行并显示主页。
We can do this by creating an express server to serve our app, so let’s do that.
我们可以通过创建一个快速服务器来为我们的应用程序提供服务来做到这一点,让我们开始吧。
First, install express, by running npm i express
and then create a file, server.js
at the root of your project. Add the following boilerplate to create the server.
首先,通过运行npm i express
安装express,然后在项目的根目录下创建一个文件server.js
。 添加以下样板来创建服务器。
var express = require('express')
var app = express()app.listen(3000, () => {
console.log("App listening on port 3000")
})
Now, we need to do two things. We need to configure a static directory which will tell express where it can find our html & js files. Then we need to intercept all http calls and route them back to index.html.
现在,我们需要做两件事。 我们需要配置一个静态目录,该目录将告诉express在哪里可以找到我们的html&js文件。 然后,我们需要拦截所有http调用,并将它们路由回到index.html。
The static directory for Svelte will be the public
directory because that’s where all of the built files are placed. This is standard so will probably be the same for other frameworks, but obviously make sure you check.
Svelte的静态目录将是public
目录,因为这是所有构建文件所在的位置。 这是标准的,因此对于其他框架可能是相同的,但是显然请确保您进行了检查。
To define a static folder in express, we do this:
要在express中定义一个静态文件夹,我们可以这样做:
app.use(express.static('public'))
app.use(express.static('public'))
So add that to server.js
因此,将其添加到server.js
var express = require('express')
var app = express()app.use(express.static('public'))app.listen(3000, () => {
console.log("App listening on port 3000")
})
Now, if you run your server with node server.js
, you should actually see your application loaded in the browser. However, we still haven’t solved the problem.
现在,如果使用node server.js
运行服务器,则实际上应该看到应用程序已加载到浏览器中。 但是,我们仍然没有解决问题。
Now we need to re-write all http calls to index.html
. This is straightforward, and looks like the following.
现在,我们需要将所有http调用重写为index.html
。 这很简单,如下所示。
app.get('*', (req, res) => {
res.sendFile(__dirname + "/public/index.html")
})
The asterisk character intercepts all calls, and then we tell the server to return the index.html
file from the public folder. We use __dirname
to get the fully qualified path.
星号字符会拦截所有调用,然后我们告诉服务器从公用文件夹返回index.html
文件。 我们使用__dirname
获取完全限定的路径。
If you run your server now, you should be able to navigate directly to localhost:5000/home
. There is still one problem, though.
如果现在运行服务器,则应该能够直接导航到localhost:5000/home
。 但是,仍然存在一个问题。
Hot Reloading
热装
One of the benefits of this was that it would enable hot reloading. However, now that we aren’t using Svelte’s npm run dev
to run our app, we aren’t getting the benefit of that. Currently, we’d need to stop the server, compile the app and restart it again to make hot-reloading work. This is a pain to do manually, but we can automate it relatively easily.
这样做的好处之一是可以进行热重装。 但是,既然我们不使用Svelte的npm run dev
来运行我们的应用程序,我们就无法从中受益。 当前,我们需要停止服务器,编译应用程序,然后再次重新启动它以使热重装工作正常。 手动操作很麻烦,但是我们可以相对轻松地实现自动化。
There’s a nice little npm package called concurrently
. The last step of our process is to install this with npm i concurrently
and then create a script in our package.json
to run both our server and our hot-reloading. Here’s how that looks for svelte (it will be almost identical for other frameworks).
有一个不错的concurrently
调用的小npm包。 我们过程的最后一步是与npm i concurrently
安装,然后在package.json
创建一个脚本以运行我们的服务器和热重载。 这就是寻找苗条的样子(其他框架几乎一样)。
"scripts": {
"build": "rollup -c",
"dev": "rollup -c -w",
"start": "sirv public",
"serve": "concurrently \"node server.js\" \"npm run dev\" "
},
Our serve
command will run both the server and the dev
command. Because the dev command recreates the public
folder on every save, and our server reads from it on every request, we always get the most up-to-date code for the app.
我们的serve
命令将同时运行服务器和dev
命令。 由于开发命令再现了public
每次保存文件夹,我们的服务器从它在每次请求读取,我们始终得到了应用最先进的日期代码。
That’s it. Simple process, but quite annoying if you don’t know what you’re looking for. Thanks for reading.
而已。 简单的过程,但是如果您不知道要查找的内容,则很烦人。 谢谢阅读。
如何安装svelte