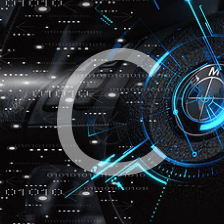
树
小·幸·运
你的所有努力最后都会回赠予你。
展开
-
python二叉树的层序遍历模版
使用两个队列存储二叉树,主队列循环遍历各层,临时队列对每一层元素遍历,代码如下,res存放层序遍历结果:class Solution: def levelOrder(self, root: TreeNode) -> List[List[int]]: if not root: return [] queue = [root] #主队列 res = [] level = 0 #标记层号原创 2021-07-24 19:18:12 · 243 阅读 · 1 评论 -
python二叉树的非递归遍历
在树的深度优先遍历中(包括前序、中序、后序遍历),递归方法最为直观易懂,但考虑到效率,我们通常不推荐使用递归。栈迭代方法虽然提高了效率,但其嵌套循环却非常烧脑,不易理解,容易造成“一看就懂,一写就废”的窘况。而且对于不同的遍历顺序(前序、中序、后序),循环结构差异很大,更增加了记忆负担。因此,我在这里介绍一种“灰白标记法”,兼具栈迭代方法的高效,又像递归方法一样简洁易懂,更重要的是,这种方法对于前序、中序、后序遍历,能够写出完全一致的代码。其核心思想如下:使用颜色标记节点的状态,新节点为白色,已原创 2021-07-24 19:11:12 · 1068 阅读 · 0 评论 -
LeetCode:117. 填充每个节点的下一个右侧节点指针 II
填充每个节点的下一个右侧节点指针 II难度中等给定一个二叉树struct Node { int val; Node *left; Node *right; Node *next;}填充它的每个 next 指针,让这个指针指向其下一个右侧节点。如果找不到下一个右侧节点,则将 next 指针设置为 NULL。初始状态下,所有 next 指针都被设置为 NULL。进阶:你只能使用常量级额外空间。使用递归解题也符合要求,本题中递归程序占用的栈空间不算做额外的空间复杂度。示.原创 2021-03-26 11:35:13 · 164 阅读 · 1 评论 -
PAT甲级A1115 Counting Nodes in a BST (30 分)
A Binary Search Tree (BST) is recursively defined as a binary tree which has the following properties:The left subtree of a node contains only nodes with keys less than or equal to the node's key. ...原创 2019-03-01 16:04:35 · 133 阅读 · 0 评论 -
PAT甲级A1102 Invert a Binary Tree (25 分)
The following is from Max Howell @twitter:Google: 90% of our engineers use the software you wrote (Homebrew), but you can't invert a binary tree on a whiteboard so fuck off.Now it's your turn to...原创 2019-02-25 20:26:30 · 153 阅读 · 0 评论 -
PAT甲级A1119 Pre- and Post-order Traversals (30 分)
Suppose that all the keys in a binary tree are distinct positive integers. A unique binary tree can be determined by a given pair of postorder and inorder traversal sequences, or preorder and inorder ...原创 2019-03-01 21:42:24 · 370 阅读 · 0 评论 -
PAT甲级A1106 Lowest Price in Supply Chain (25 分)
A supply chain is a network of retailers(零售商), distributors(经销商), and suppliers(供应商)-- everyone involved in moving a product from supplier to customer.Starting from one root supplier, everyone on th...原创 2019-02-26 19:35:57 · 170 阅读 · 0 评论 -
PAT甲级A1123 Is It a Complete AVL Tree (30 分)
An AVL tree is a self-balancing binary search tree. In an AVL tree, the heights of the two child subtrees of any node differ by at most one; if at any time they differ by more than one, rebalancing is...原创 2019-03-08 15:43:01 · 159 阅读 · 0 评论 -
PAT甲级A1127 ZigZagging on a Tree (30 分)
Suppose that all the keys in a binary tree are distinct positive integers. A unique binary tree can be determined by a given pair of postorder and inorder traversal sequences. And it is a simple stand...原创 2019-03-08 19:44:16 · 258 阅读 · 0 评论 -
PAT甲级A1130 Infix Expression (25 分)
Given a syntax tree (binary), you are supposed to output the corresponding infix expression, with parentheses reflecting the precedences of the operators.Input Specification:Each input file contai...原创 2019-03-09 17:28:43 · 227 阅读 · 0 评论 -
PAT甲级A1143 Lowest Common Ancestor (30 分)
The lowest common ancestor (LCA) of two nodes U and V in a tree is the deepest node that has both U and V as descendants.A binary search tree (BST) is recursively defined as a binary tree which has ...原创 2019-03-15 14:10:44 · 389 阅读 · 0 评论 -
PAT甲级A1135 Is It A Red-Black Tree (30 分)
There is a kind of balanced binary search tree named red-black tree in the data structure. It has the following 5 properties:(1) Every node is either red or black. (2) The root is black. (3) Every...原创 2019-03-11 17:20:03 · 382 阅读 · 0 评论 -
PAT甲级A1138 Postorder Traversal (25 分)
Suppose that all the keys in a binary tree are distinct positive integers. Given the preorder and inorder traversal sequences, you are supposed to output the first number of the postorder traversal se...原创 2019-03-12 20:12:19 · 231 阅读 · 0 评论 -
PAT甲级A1099 Build A Binary Search Tree (30 分)
A Binary Search Tree (BST) is recursively defined as a binary tree which has the following properties:The left subtree of a node contains only nodes with keys less than the node's key. The right su...原创 2019-02-25 15:00:00 · 222 阅读 · 0 评论 -
PAT甲级A1079 Total Sales of Supply Chain (25 分)
A supply chain is a network of retailers(零售商), distributors(经销商), and suppliers(供应商)-- everyone involved in moving a product from supplier to customer.Starting from one root supplier, everyone on th...原创 2019-02-19 14:58:47 · 240 阅读 · 0 评论 -
LeetCode--606. Construct String from Binary Tree
You need to construct a string consists of parenthesis and integers from a binary tree with the preorder traversing way.The null node needs to be represented by empty parenthesis pair "()". And you ne...原创 2018-07-07 22:51:24 · 117 阅读 · 0 评论 -
PAT甲级A1004 Counting Leaves (30)
A family hierarchy is usually presented by a pedigree tree. Your job is to count those family members who have no child.InputEach input file contains one test case. Each case starts with a line co...原创 2018-07-19 21:35:21 · 179 阅读 · 0 评论 -
PAT甲级A1020 Tree Traversals (25)
Suppose that all the keys in a binary tree are distinct positive integers. Given the postorder and inorder traversal sequences, you are supposed to output the level order traversal sequence of the cor...原创 2018-08-07 22:28:58 · 158 阅读 · 0 评论 -
PAT甲级A1043 Is It a Binary Search Tree(25 分)
1043 Is It a Binary Search Tree(25 分)A Binary Search Tree (BST) is recursively defined as a binary tree which has the following properties:The left subtree of a node contains only nodes with keys ...原创 2018-09-01 22:17:36 · 505 阅读 · 0 评论 -
PAT甲级A1147 Heaps(30 分)
1147 Heaps(30 分)In computer science, a heap is a specialized tree-based data structure that satisfies the heap property: if P is a parent node of C, then the key (the value) of P is either greater t...原创 2018-09-06 22:03:09 · 278 阅读 · 0 评论 -
PAT甲级A1151 LCA in a Binary Tree(30 分)
1151 LCA in a Binary Tree(30 分)The lowest common ancestor (LCA) of two nodes U and V in a tree is the deepest node that has both U and V as descendants.Given any two nodes in a binary tree, you ar...原创 2018-09-10 23:46:45 · 304 阅读 · 0 评论 -
PAT甲级A1064 Complete Binary Search Tree (30 分)
A Binary Search Tree (BST) is recursively defined as a binary tree which has the following properties:The left subtree of a node contains only nodes with keys less than the node's key. The right su...原创 2019-01-24 22:16:41 · 193 阅读 · 0 评论 -
PAT甲级A1053 Path of Equal Weight (30 分)
Given a non-empty tree with root RRR, and with weight WiW_iWi assigned to each tree node TiT_iTi. The weight of a path from RRR to LLL is defined to be the sum of the weights of all the nodes al...原创 2019-01-20 17:03:07 · 277 阅读 · 0 评论 -
PAT甲级A1066 Root of AVL Tree (25 分)
An AVL tree is a self-balancing binary search tree. In an AVL tree, the heights of the two child subtrees of any node differ by at most one; if at any time they differ by more than one, rebalancing is...原创 2019-01-26 22:14:33 · 180 阅读 · 0 评论 -
PAT甲级A1086 Tree Traversals Again (25 分)
An inorder binary tree traversal can be implemented in a non-recursive way with a stack. For example, suppose that when a 6-node binary tree (with the keys numbered from 1 to 6) is traversed, the stac...原创 2019-02-21 16:04:36 · 162 阅读 · 0 评论 -
PAT甲级A1110 Complete Binary Tree (25 分)
Given a tree, you are supposed to tell if it is a complete binary tree.Input Specification:Each input file contains one test case. For each case, the first line gives a positive integer N (≤20) wh...原创 2019-02-27 20:19:08 · 261 阅读 · 0 评论 -
PAT甲级A1090 Highest Price in Supply Chain (25 分)
A supply chain is a network of retailers(零售商), distributors(经销商), and suppliers(供应商)-- everyone involved in moving a product from supplier to customer.Starting from one root supplier, everyone on th...原创 2019-02-23 17:27:53 · 212 阅读 · 0 评论 -
PAT甲级A1094 The Largest Generation (25 分)
A family hierarchy is usually presented by a pedigree tree where all the nodes on the same level belong to the same generation. Your task is to find the generation with the largest population.Input ...原创 2019-02-23 20:14:22 · 185 阅读 · 0 评论 -
LeetCode-617-Merge Two Binary Trees
Given two binary trees and imagine that when you put one of them to cover the other, some nodes of the two trees are overlapped while the others are not. You need to merge them into a new binary tree....原创 2018-05-25 17:17:17 · 133 阅读 · 0 评论