Drap Object by Container border checking
<head>
<base href="">
<meta charset="utf-8">
<meta name="author" content="unix2linux">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<meta name="description" content="A powerful and conceptual apps base dashboard template that especially build for developers and programmers.">
<!-- Fav Icon -->
<link rel="shortcut icon" href="./images/favicon.png">
<!-- Page Title -->
<title>Designer</title>
<!-- StyleSheets -->
<link rel="stylesheet" href="./assets/css/dashlite.css?ver=2.2.0">
<link id="skin-default" rel="stylesheet" href="./assets/css/theme.css?ver=2.2.0">
<style>
.outer-container {
width: 800px;
height: 600px;
border: 1px dashed red;
margin: auto;
position: relative;
}
.inner-object {
width: 160px;
height: 120px;
background-color:forestgreen;
cursor: move;
position: absolute;
top: 20px;
}
.inner-object:hover {
box-shadow: 1px 1px 5px 0px rgba(0, 0, 0, 0.618);
-webkit-box-shadow: 1px 1px 5px 0px rgba(0, 0, 0, 0.618);
-moz-box-shadow: 1px 1px 5px 0px rgba(0, 0, 0, 0.618);
}
</style>
</head>
<body>
<div class="outer-container">
<div class="inner-object" style="left: 40px;"></div>
<div class="inner-object" style="right: 40px;background-color: blue"></div>
</div>
<script src="./assets/js/bundle.js?ver=2.2.0"></script>
<script src="./assets/js/scripts.js?ver=2.2.0"></script>
</body>
<script>
function dragCheckBorder(dragObj, containerObj) {
$(dragObj).mousedown(function (e) {
var _this = $(this)
var containerHeight = $(containerObj)[0].offsetHeight
containerWidth = $(containerObj)[0].offsetWidth
var dragHeight = $(this)[0].offsetHeight
dragWidth = $(this)[0].offsetWidth
var dragX = e.clientX - $(this)[0].offsetLeft
dragY = e.clientY - $(this)[0].offsetTop
$(this).css('z-index','999').siblings().css('z-index','1')
$(document).mousemove(function (e) {
var l = e.clientX - dragX
var t = e.clientY - dragY
if (l < 0) {
l = 0
} else if (l > containerWidth - dragWidth) {
l = containerWidth - dragWidth
}
if (t < 0) {
t = 0
} else if (t > containerHeight - dragHeight) {
t = containerHeight - dragHeight
}
_this.css({
left: l + 'px',
top: t + 'px',
})
})
});
$(document).mouseup(function () {
$(this).off('mousemove')
});
}
</script>
<script>
dragCheckBorder('.inner-object', '.outer-container');
</script>
https://github.com/givanz/VvvebJs
https://github.com/kevinchappell/formBuilder
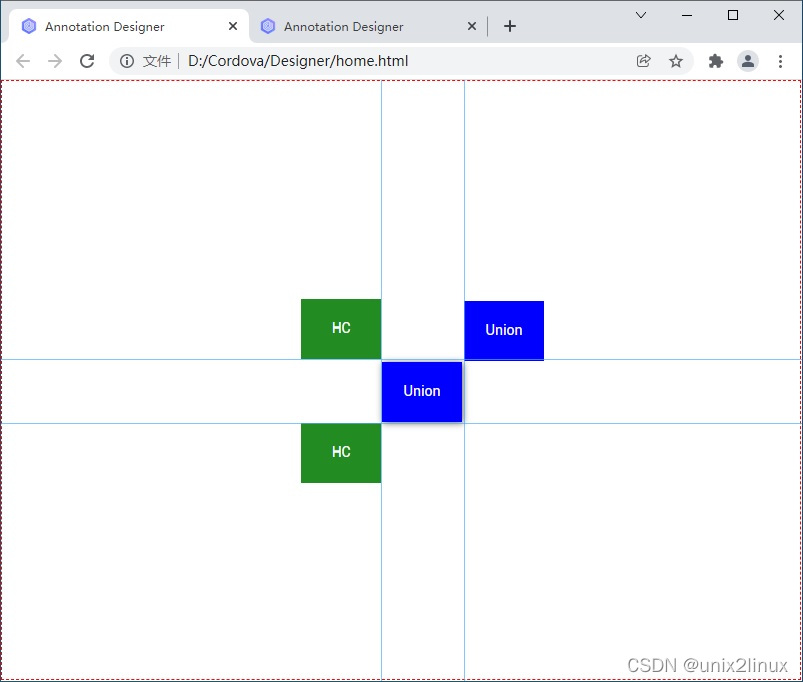