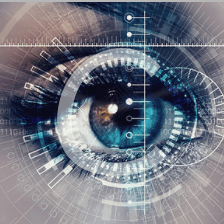
LeetCode
文章平均质量分 64
BoilerHouseKing
Stay hungry,stay foolish.
展开
-
[LeetCode] Single Number
Given an array of integers, every element appears twice except for one. Find that single one.Note:Your algorithm should have a linear runtime complexity. Could you implement it without using extra memory?原创 2014-08-01 21:56:45 · 592 阅读 · 0 评论 -
[LeetCode]Maximum Depth of Binary Tree
Given a binary tree, find its maximum depth.The maximum depth is the number of nodes along the longest path from the root node down to the farthest leaf node./** * Definition for binary tree原创 2014-08-02 21:29:49 · 684 阅读 · 0 评论 -
[LeetCode]Reverse Words in a String
href: https://oj.leetcode.com/problems/reverse-words-in-a-string/Given an input string, reverse the string word by word.For example,Given s = "the sky is blue",return "blue is sky the".原创 2014-08-02 20:55:54 · 595 阅读 · 0 评论 -
[LeetCode]Reverse Integer
Reverse digits of an integer.Example1: x = 123, return 321Example2: x = -123, return -321原创 2014-08-03 21:03:14 · 491 阅读 · 0 评论 -
[LeetCode]Same Tree
Given two binary trees, write a function to check if they are equal or not.Two binary trees are considered equal if they are structurally identical and the nodes have the same value./**原创 2014-08-03 09:52:56 · 566 阅读 · 0 评论 -
Swap Nodes in Pairs
Given a linked list, swap every two adjacent nodes and return its head.For example,Given 1->2->3->4, you should return the list as 2->1->4->3.Your algorithm should use only constant space. Y原创 2014-09-04 14:22:57 · 556 阅读 · 0 评论 -
[LeetCOde]Reverse Nodes in k-Group
Given a linked list, reverse the nodes of a linked list k at a time and return its modified list.If the number of nodes is not a multiple of k then left-out nodes in the end should remain as it is原创 2014-09-04 22:09:53 · 653 阅读 · 0 评论 -
[LeetCode]Remove Element
Given an array and a value, remove all instances of that value in place and return the new length.The order of elements can be changed. It doesn't matter what you leave beyond the new length.p原创 2014-09-05 22:11:36 · 482 阅读 · 0 评论 -
[LeetCode]经典 Combination Sum II
Given a collection of candidate numbers (C) and a target number (T), find all unique combinations in C where the candidate numbers sums to T.Each number in C may only be used once in the combina原创 2014-09-18 20:49:35 · 668 阅读 · 0 评论 -
[LeetCode]Longest Common Prefix
Write a function to find the longest common prefix string amongst an array of strings.public class Solution { public String longestCommonPrefix(String[] strs) { if(strs.length==0){原创 2014-08-22 21:13:56 · 509 阅读 · 0 评论 -
[LeetCode]Combination Sum
Given a set of candidate numbers (C) and a target number (T), find all unique combinations in C where the candidate numbers sums to T.The same repeated number may be chosen from C unlimited numb原创 2014-09-18 20:39:54 · 594 阅读 · 0 评论 -
Container With Most Water
Given n non-negative integers a1, a2, ..., an, where each represents a point at coordinate (i, ai). n vertical lines are drawn such that the two endpoints of line i is at (i, ai) and (i, 0). Fin原创 2014-08-21 21:19:35 · 491 阅读 · 0 评论 -
[LeetCode]Integer to Roman AND ROman to Integer
Given an integer, convert it to a roman numeral.Input is guaranteed to be within the range from 1 to 3999.public class Solution { public String intToRoman(int num) { int[] number =原创 2014-08-21 21:46:19 · 687 阅读 · 0 评论 -
[LeetCode]First Missing Positive
Given an unsorted integer array, find the first missing positive integer.For example,Given [1,2,0] return 3,and [3,4,-1,1] return 2.Your algorithm should run in O(n) time and uses constant原创 2014-09-20 19:31:06 · 524 阅读 · 0 评论 -
[LeetCode]Median of Two Sorted Arrays
There are two sorted arrays A and B of size m and n respectively. Find the median of the two sorted arrays. The overall run time complexity should be O(log (m+n)).public class Solution {原创 2014-08-11 11:40:18 · 648 阅读 · 0 评论 -
[LeetCode]Longest Substring Without Repeating Characters
Given a string, find the length of the longest substring without repeating characters. For example, the longest substring without repeating letters for "abcabcbb" is "abc", which the length is 3. Fo原创 2014-08-11 22:14:40 · 518 阅读 · 0 评论 -
Remove Duplicates from Sorted Array.java
public class Solution { public int removeDuplicates(int[] A) { final int max = (int) Math.pow(2, 31); int length = A.length; int i = 0,comp = max,res = 0; while(i<length){原创 2014-09-09 21:51:18 · 476 阅读 · 0 评论 -
Implement strStr()
public class Solution { public String strStr(String haystack, String needle) { if(haystack==null||haystack.length()<needle.length()){ return null; } int hlen = haystack.length原创 2014-09-09 21:53:21 · 534 阅读 · 0 评论 -
Letter Combinations of a Phone Number
Given a digit string, return all possible letter combinations that the number could represent.A mapping of digit to letters (just like on the telephone buttons) is given below.Input:Digit原创 2014-09-10 21:22:31 · 708 阅读 · 0 评论 -
Search in Rotated Sorted Array
Suppose a sorted array is rotated at some pivot unknown to you beforehand.(i.e., 0 1 2 4 5 6 7 might become 4 5 6 7 0 1 2).You are given a target value to search. If found in the array retur原创 2014-09-11 22:23:43 · 847 阅读 · 0 评论 -
[LeetCode]Permutations
Given a collection of numbers, return all possible permutations.For example,[1,2,3] have the following permutations:[1,2,3], [1,3,2], [2,1,3], [2,3,1], [3,1,2], and [3,2,1].public class So原创 2014-09-22 20:15:19 · 551 阅读 · 0 评论 -
[LeetCode]Longest Palindromic Substring最长回文字符串
Given a string S, find the longest palindromic substring in S. You may assume that the maximum length of S is 1000, and there exists one unique longest palindromic substring.动态规划 时间:O(n^2)原创 2014-08-15 22:05:06 · 574 阅读 · 0 评论 -
[LeetCode]Trapping Rain Water
Given n non-negative integers representing an elevation map where the width of each bar is 1, compute how much water it is able to trap after raining.For example, Given [0,1,0,2,1,0,1,3,2,1,2,1]原创 2014-09-23 14:33:22 · 742 阅读 · 0 评论 -
[LeetCode]Substring with Concatenation of All Words
You are given a string, S, and a list of words, L, that are all of the same length. Find all starting indices of substring(s) in S that is a concatenation of each word in L exactly once and without an原创 2014-09-12 20:59:09 · 983 阅读 · 0 评论 -
[LeetCode]ZigZag Conversion
The string "PAYPALISHIRING" is written in a zigzag pattern on a given number of rows like this: (you may want to display this pattern in a fixed font for better legibility)P A H NA P L S I原创 2014-08-17 11:17:57 · 501 阅读 · 0 评论 -
[LeetCode]Longest Valid Parentheses
Given a string containing just the characters '(' and ')', find the length of the longest valid (well-formed) parentheses substring.For "(()", the longest valid parentheses substring is "()",原创 2014-09-13 20:01:24 · 621 阅读 · 0 评论 -
[LeetCode]3Sum Closest
Given an array S of n integers, find three integers in S such that the sum is closest to a given number, target. Return the sum of the three integers. You may assume that each input would have exact原创 2014-08-28 21:12:42 · 751 阅读 · 1 评论 -
[LeetCode]Jump Game II
Given an array of non-negative integers, you are initially positioned at the first index of the array.Each element in the array represents your maximum jump length at that position.Your goal i原创 2014-09-23 16:21:15 · 673 阅读 · 0 评论 -
[LeetCode][I]Permutations II
Given a collection of numbers that might contain duplicates, return all possible unique permutations.For example,[1,1,2] have the following unique permutations:[1,1,2], [1,2,1], and [2,1,1].原创 2014-09-23 13:11:58 · 825 阅读 · 0 评论 -
[LeetCode]Palindrome Number
Determine whether an integer is a palindrome. Do this without extra space.click to show spoilers.Some hints:Could negative integers be palindromes? (ie, -1)If you are thinking of converting th原创 2014-08-17 21:11:41 · 535 阅读 · 0 评论 -
[LeetCode]3Sum
Given an array S of n integers, are there elements a, b, c in S such that a + b + c = 0? Find all unique triplets in the array which gives the sum of zero.Note:Elements in a triplet (a,b,c原创 2014-08-28 11:53:27 · 712 阅读 · 0 评论 -
Valid Parentheses
Given a string containing just the characters '(', ')', '{', '}', '[' and ']', determine if the input string is valid.The brackets must close in the correct order, "()" and "()[]{}" are all va原创 2014-08-30 21:28:08 · 543 阅读 · 0 评论 -
[LeetCode]Next Permutation
Implement next permutation, which rearranges numbers into the lexicographically next greater permutation of numbers.If such arrangement is not possible, it must rearrange it as the lowest possible原创 2014-09-12 21:55:57 · 729 阅读 · 0 评论 -
Valid Sudoku
Determine if a Sudoku is valid, according to: Sudoku Puzzles - The Rules.The Sudoku board could be partially filled, where empty cells are filled with the character '.'原创 2014-09-14 22:19:35 · 530 阅读 · 0 评论 -
[LeetCode]Search Insert Position
Given a sorted array and a target value, return the index if the target is found. If not, return the index where it would be if it were inserted in order.You may assume no duplicates in the array.原创 2014-09-14 21:39:12 · 811 阅读 · 0 评论 -
[LeetCode]Merge k Sorted Lists
Merge k sorted linked lists and return it as one sorted list. Analyze and describe its complexity.分治法/** * Definition for singly-linked list. * public class ListNode { * int val; * L原创 2014-09-02 20:36:03 · 464 阅读 · 0 评论 -
[LeetCode]Pow(x, n)
Implement pow(x, n).public class Solution { public double pow(double x, int n) { if(n>0) return powInt(x,n); else return 1/powInt(x,-n); } private double powInt(double x, int n){ if原创 2014-09-26 16:06:30 · 927 阅读 · 0 评论 -
[LeetCode] Rotate Image
You are given an n x n 2D matrix representing an image.Rotate the image by 90 degrees (clockwise).Follow up:Could you do this in-place?思路:先沿着主对角线交换矩阵内的数字,然后沿中间列交换。public class Soluti原创 2014-09-26 14:39:01 · 723 阅读 · 0 评论 -
Sudoku Solver
Write a program to solve a Sudoku puzzle by filling the empty cells.Empty cells are indicated by the character '.'.You may assume that there will be only one unique solution.A sudoku puzzl原创 2014-09-16 20:54:17 · 664 阅读 · 0 评论 -
[LeetCode]Count and Say
The count-and-say sequence is the sequence of integers beginning as follows:1, 11, 21, 1211, 111221, ...1 is read off as "one 1" or 11.11 is read off as "two 1s" or 21.21 is read off as原创 2014-09-17 20:03:32 · 535 阅读 · 0 评论