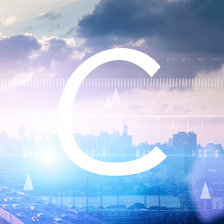
LeetCode
文章平均质量分 50
益达915
一名在读大学生,渣渣一枚,进取中...
展开
-
去除数组中重复元素
类型总结:双指针问题 说明:遍历时利用2个下标,边遍历边更新。1、26.Remove Duplicates from Sorted Array 从一个包含重复元素的数组里面去除重复的元素,要求不能另外开辟空间思路:由于是一个经过排序的数组,可以设置一个临时变量,从第1个元素(起始是0个)开始遍历,如果遇到不同的,就将其赋值。并且当前index++,存入新的点。遇到相同的这些都不会发生。一开始我想原创 2016-07-29 10:01:36 · 1319 阅读 · 0 评论 -
从有序数组中寻找元素
类型总结:二分 说明:利用数组有序的性质,使用二分实现快速查找3、33. Search in Rotated Sorted Array 题目的大意是一个顺序的数组,但是在某个地方被反转了,例如 (i.e., 0 1 2 4 5 6 7 might become 4 5 6 7 0 1 2). 那么给出一个数,问找到这个数的下标。 实际上是一个变相的二分,只是在进行二分之前,不能仅仅按照ta原创 2016-07-29 10:15:02 · 473 阅读 · 0 评论 -
求和
主要思想:哈希 双指针 内容:用unordered_map来改善哈希表的性能5、128. Longest Consecutive Sequence Given an unsorted array of integers, find the length of the longest consecutive elements sequence.For example, Given [100, 4原创 2016-07-29 19:35:22 · 363 阅读 · 0 评论 -
求数组中多个数相加等于某一值
主要思想:排序+两端逼近 内容:排序使得整个数组有序从而可以使用双指针从数组的两端向中间逼近所需要的值7、3Sum 顾名思义,求数组中3个数相加等于某一特定的数自己写了一个似乎是O(n^2) 汗颜 在Two Sum的引导下,我成功使用了unordered_map 然而在run code的时候就发现了一个无法解决的问题: Your input [-1,0,1,2,-1,-4] Your a原创 2016-07-29 19:41:36 · 19398 阅读 · 0 评论 -
数学和模拟
思想:利用数学规律或者模拟 内容:注意next_permutation的求取方式 Trapping Rain Water和Candy的单调方式 Single Num涉及到的位运算 11、Next Permutation 纯数学题,/(ㄒoㄒ)/~~class Solution {public: void nextPermutation(vector<int>& nums) {原创 2016-07-30 18:04:23 · 490 阅读 · 0 评论 -
LeetCode | Valid Palindrome
Given a string, determine if it is a palindrome, considering only alphanumeric characters and ignoring cases. For example, “A man, a plan, a canal: Panama” is a palindrome. “race a car” is n原创 2016-08-03 20:44:49 · 281 阅读 · 0 评论 -
LeetCode | Implement strStr()
求子串的,暴力O(n*m) 等我看完了KMP算法那些O(m+n),再来考虑这个优化比较有趣的是空串是任意串的子串,并且对于子串长度已经超过父串可以容纳的时候,pass(这个很重要,不然会TLE)class Solution {public: int strStr(string haystack, string needle) { int len=haystack.size(原创 2016-08-03 21:00:28 · 225 阅读 · 0 评论 -
LeetCode | String to Integer
讲道理这道题挺无聊的…各种特判 不过这个代码风格还是可以学习一下的。。。 Implement atoi to convert a string to an integer. Hint: Carefully consider all possible input cases. If you want a challenge, please do not see below and原创 2016-08-03 21:52:50 · 196 阅读 · 0 评论 -
LeetCode | Add Binary
Given two binary strings, return their sum (also a binary string). For example, a = “11” b = “1” Return “100”.简单的二进制加法模拟,为了让代码更优雅,模仿了之前高精度和链表加法里面的做法 即 i>=0 || j>=0 再对i、j进行特判给的提示也是这样做的,不过使用了好多函数其原创 2016-08-03 22:11:48 · 207 阅读 · 0 评论 -
LeetCode | Add Two Numbers
之前都是好些题目为一个小章节放在一起在csdn上面的,还是分开吧这样可以更加清楚一点这道题卡了我好久, 一来是好久没有写指针操作 二来是初次使用leetcode,它对于指针的操作和之前自己的习惯不一样。还是mark一下吧。 虽然代码跟翔一样,但是这是自己一步步推导的过程/** * Definition for singly-linked list. * struct ListNode {原创 2016-07-31 16:43:43 · 266 阅读 · 0 评论 -
LeetCode | Reverse Linked List II
这道链表反转题也是搞的我焦头烂额,好久没有写链表了,注意记忆这些 Reverse Linked List II QuestionEditorial Solution My Submissions Total Accepted: 78774 Total Submissions: 274380 Difficulty: Medium Reverse a linked list from原创 2016-07-31 16:48:43 · 230 阅读 · 0 评论 -
LeetCode | Partition List
Given a linked list and a value x, partition it such that all nodes less than x come before nodes greater than or equal to x. You should preserve the original relative order of the nodes in eac原创 2016-07-31 17:29:33 · 274 阅读 · 0 评论 -
LeetCode | Rotate List
一开始想错了,以为要在k的位置旋转 其实是要循环滚动地推k次,比如 [1,2] 2 结果应当为[1,2],以为推一圈又回来了 而 [1,2] 3 则应当是[2,1]效果和1是一样的于是加一个mod判断就好了class Solution {public: ListNode* rotateRight(ListNode* head, int k) { //空或左原创 2016-07-31 20:57:03 · 248 阅读 · 0 评论 -
LeetCode | Palindrome Partitioning I,II
Given a string s, partition s such that every substring of the partition is a palindrome.Return all possible palindrome partitioning of s.For example, given s = “aab”, Return[ [“aa”,”b”], [“a”,”原创 2016-08-15 18:50:44 · 256 阅读 · 0 评论 -
LeetCode | Unique Paths I,II
A robot is located at the top-left corner of a m x n grid (marked ‘Start’ in the diagram below). The robot can only move either down or right at any point in time. The robot is trying to reach the b原创 2016-08-15 22:25:42 · 293 阅读 · 0 评论 -
LeetCode | Regular Expression Matching
Implement regular expression matching with support for ‘.’ and ‘*’.‘.’ Matches any single character. ‘*’ Matches zero or more of the preceding element.The matching should cover the entire input string原创 2016-08-16 10:07:17 · 243 阅读 · 0 评论 -
LeetCode | Remove Nth Node From End of List
Given a linked list, remove the nth node from the end of list and return its head. For example, Given linked list: 1->2->3->4->5, and n = 2. After removing the second node from the en原创 2016-07-31 21:56:18 · 235 阅读 · 0 评论 -
LeetCode | Swap Nodes in Pairs
Given a linked list, swap every two adjacent nodes and return its head. For example, Given 1->2->3->4, you should return the list as 2->1->4->3. Your algorithm should use only constant原创 2016-07-31 23:07:41 · 264 阅读 · 0 评论 -
LeetCode | Remove Duplicates from Sorted List II
这道题一开始以为是双指针问题… Given a sorted linked list, delete all nodes that have duplicate numbers, leaving only distinct numbers from the original list. For example, Given 1->2->3->3->4->4->5, return原创 2016-07-31 19:29:15 · 285 阅读 · 0 评论 -
LeetCode | Longest Palindromic Substring
题目 Given a string S, find the longest palindromic substring in S. You may assume that the maximum length of S is 1000, and there exists one unique longest palindromic substring.最长回文串问题,网上也有超多的原创 2016-08-04 17:01:24 · 241 阅读 · 0 评论 -
LeetCode | N-Queens I,II
The n-queens puzzle is the problem of placing n queens on an n×n chessboard such that no two queens attack each other. Given an integer n, return all distinct solutions to the n-queens puzzle.Each s原创 2016-08-16 17:05:15 · 260 阅读 · 0 评论 -
LeetCode | Restore IP Addresses
Given a string containing only digits, restore it by returning all possible valid IP address combinations.For example: Given “25525511135”,return [“255.255.11.135”, “255.255.111.35”]. (Order does not原创 2016-08-16 17:56:39 · 259 阅读 · 0 评论 -
LeetCode | Longest Common Prefix
Write a function to find the longest common prefix string amongst an array of strings.求字符串最长公共前缀,这题也没啥好说的,就代码可读性上说一说好了。我写的虽然也是4ms虽然也没错,但是可读性上来说相对差了一点class Solution {public: string longestCommonPr原创 2016-08-05 00:01:17 · 210 阅读 · 0 评论 -
LeetCode | Valid Number
写到一半不想写了,今天暂且写到这,下次继续。 按照DFA进行判断。无符号整数 无符号小数 class Solution {public: bool isNumber(string s) { return work(s.c_str()); } bool work(const char* s){ //1、忽略前置空格 wh原创 2016-08-05 00:29:48 · 234 阅读 · 0 评论 -
LeetCode | Reverse Nodes in k-Group
链表的反转 写的是生无可恋啊… 我的思路….其实和正确答案都差不多,但是一直ce调不出来… Given a linked list, reverse the nodes of a linked list k at a time and return its modified list. If the number of nodes is not a multiple of k原创 2016-08-01 23:49:06 · 228 阅读 · 0 评论 -
LeetCode | Combination Sum I,II
Given a set of candidate numbers (C) and a target number (T), find all unique combinations in C where the candidate numbers sums to T.The same repeated number may be chosen from C unlimited number of t原创 2016-08-16 21:08:03 · 303 阅读 · 0 评论 -
LeetCode | Length of Last Word
没啥好说 ,字符串模拟, 注意从后面去除空格就好 另外,要注意这个平台string越界(-1 或者 大于 s.size())是不会报错的,如果不加pos>=0 会出现输出奇葩的长度,故注意边界控制class Solution {public: int lengthOfLastWord(string s) { int n=s.size(),len=0; i原创 2016-08-05 12:00:42 · 228 阅读 · 0 评论 -
LeetCode | Copy List with Random Pointer
拷贝一个带随机指针的链表 A linked list is given such that each node contains an additional random pointer which could point to any node in the list or null. Return a deep copy of the list.这道题的思路很重要… 首先原创 2016-08-02 09:31:34 · 431 阅读 · 0 评论 -
LeetCode | Linked List Cycle I,II
Given a linked list, determine if it has a cycle in it. Follow up: Can you solve it without using extra space?这道题想做出来不难,关键是快慢指针这种一般想不到。。 一般想到的是拿哈希存储已经经过的pointer 这种空间复杂度O(n) 快慢指针这个很巧妙,设置两个指针fas原创 2016-08-02 11:27:30 · 264 阅读 · 0 评论 -
LeetCode | Binary Tree Preorder Traversal
先序遍历 Given a binary tree, return the preorder traversal of its nodes’ values. For example: Given binary tree {1,#,2,3}, 1 \ 2 3 return [1,2,3]. Note: Recursive sol原创 2016-08-05 17:24:44 · 283 阅读 · 0 评论 -
LeetCode | Binary Tree Inorder Traversal
Given a binary tree, return the inorder traversal of its nodes’ values. For example: Given binary tree [1,null,2,3], 1 \ 2 / 3 return [1,3,2].Note: Rec原创 2016-08-05 19:02:25 · 223 阅读 · 0 评论 -
LeetCode | Binary Tree Postorder Traversal
后序遍历,当然,,,用栈实现后序遍历的关键在于从左孩子回到根节点和从右孩子回到跟节点。 如果是从左回的,要继续向右。如果是从右边回来的(表明左右孩子已经遍历结束)这时候就需要将自身输出。具体到代码上,就是使用一个q指针,用于保存上一个走过的节点(确切的说应该是输出的节点)。 然后从栈顶取出元素的时候,判断p->right==q? 来决定它是从哪个方向回来的~ 完成使命~~注释里面也说的比较清楚原创 2016-08-05 19:54:42 · 259 阅读 · 0 评论 -
LeetCode | Reorder List
Given a singly linked list L: L0→L1→…→Ln-1→Ln, reorder it to: L0→Ln→L1→Ln-1→L2→Ln-2→… You must do this in-place without altering the nodes’ values. For example, Given {1,2,3,4}, reorder it原创 2016-08-02 20:00:18 · 235 阅读 · 0 评论 -
LeetCode | Word Break II
Given a string s and a dictionary of words dict, add spaces in s to construct a sentence where each word is a valid dictionary word.Return all such possible sentences.For example, givens = "原创 2016-08-27 11:51:48 · 545 阅读 · 0 评论 -
LeetCode | Binary Tree Level Order Traversal I,II
Given a binary tree, return the level order traversal of its nodes’ values. (ie, from left to right, level by level). For example: Given binary tree [3,9,20,null,null,15,7], 3 /原创 2016-08-05 20:45:59 · 265 阅读 · 0 评论 -
LeetCode | Binary Tree Zigzag Level Order Traversal
二叉树Z型输出Given a binary tree, return the zigzag level order traversal of its nodes’ values. (ie, from left to right, then right to left for the next level and alternate between).For example: Given binar原创 2016-08-06 12:04:22 · 241 阅读 · 0 评论 -
LeetCode | Recover Binary Search Tree
参考文章:http://www.cnblogs.com/TenosDoIt/p/3445682.htmlTwo elements of a binary search tree (BST) are swapped by mistake.Recover the tree without changing its structure.Note: A solution using O(n) space原创 2016-08-06 15:51:32 · 270 阅读 · 0 评论 -
LeetCode | Same Tree
判断两棵树是否相同~在下写的是递归版本~~class Solution {public: bool isSameTree(TreeNode* p, TreeNode* q) { if((p && !q )|| (!p && q)) return false; //只有一个为空,必然是false //其实上面一句可以改成 if(!p || !q)原创 2016-08-06 16:05:15 · 204 阅读 · 0 评论 -
LeetCode | Symmetric Tree
Given a binary tree, check whether it is a mirror of itself (ie, symmetric around its center).For example, this binary tree [1,2,2,3,4,4,3] is symmetric:1/ \ 2 2 / \ / \ 3 4 4 3 But the fol原创 2016-08-06 17:27:05 · 238 阅读 · 0 评论 -
LeetCode | Invert Binary Tree
调转二叉树的左右孩子Invert a binary tree. 4/ \ 2 7 / \ / \ 1 3 6 9 to 4 / \ 7 2 / \ / \ 9 6 3 1如果用递归的思路将会非常之简单和简洁 每次遇到非空节点的时候,使用temp指针交换左右节点。然后递归调用函数,将左右孩子的子树进行调换原创 2016-08-06 17:29:58 · 228 阅读 · 0 评论