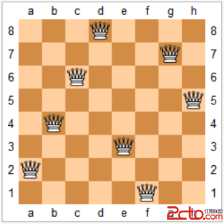
leetcode 题解
详细的 leetcode 题解
子辰曦
这个作者很懒,什么都没留下…
展开
-
[leetcode Q58] Length of Last Word
1. 题目 Given a string s consists of upper/lower-case alphabets and empty space characters ’ ‘, return the length of last word in the string. If the last word does not exist, return 0. Note:原创 2016-03-06 21:34:14 · 653 阅读 · 0 评论 -
[leetcode Q50] Pow(x, n)
1. 问题实现幂运算。标签为二分搜索。2. 思路二分搜索的简单应用,需要注意的是当 n 为负数,或是 INT_MIN 时的情况。INT_MIN 的负数是 INT_MAX + 1(无法用整数表示)3. 实现class Solution {public: double myPow(double x, int n) { if (n == 0) return原创 2016-03-06 15:21:36 · 1299 阅读 · 0 评论 -
[leetcode Q43] Multiply String
1. 问题 Given two numbers represented as strings, return multiplication of the numbers as a string. Note: The numbers can be arbitrarily large and are non-negative.2. 思路大整数乘法思路,实现竖式乘法运算即可。3. 实现cl原创 2016-03-06 14:01:35 · 568 阅读 · 0 评论 -
[leetcode Q41] First Missing Positive
1. 题目 Given an unsorted integer array, find the first missing positive integer. For example, Given [1,2,0] return 3, and [3,4,-1,1] return 2. Your algorithm should run in O(n) time an原创 2016-03-04 23:06:25 · 534 阅读 · 0 评论 -
[leetcode Q36] valid sudoku
1. 题目检查 9x9 宫格中的行列,子宫格(3x3)是否有重复的数字出现。标签为 hash table2. 思路简单的思路是使用三次循环,第一次检查每行是否有效,第二次检查每列是否有效,第三次检查每个子宫格是否有效。检查过程另外使用一个数组来保存当前状态,标签虽然是 hash table ,但没有用到常见的哈希算法,更多的是借用数组的下标来实现。3. 实现class Solution {publ原创 2016-03-04 21:29:14 · 545 阅读 · 0 评论 -
[leetcode Q34&Q35] Search for a Range&Search Insert Position
Q34 和 Q35都是使用二分查找的题目,所以放在一起。1. 问题 Given a sorted array of integers, find the starting and ending position of a given target value. Your algorithm’s runtime complexity must be in the order of O(l原创 2016-02-29 13:19:50 · 509 阅读 · 0 评论 -
[leetcode Q33] Search in Rotated Sorted Array
1. 问题 Suppose a sorted array is rotated at some pivot unknown to you beforehand. (i.e., 0 1 2 4 5 6 7 might become 4 5 6 7 0 1 2). You are given a target value to search. If found in the ar原创 2016-02-29 13:14:04 · 505 阅读 · 0 评论 -
[leetcode Q29] Divide Two Integers
1. 题目 Divide two integers without using multiplication, division and mod operator. If it is overflow, return MAX_INT. 要求不使用乘除法实现两个整数相除运算。2. 思路不使用乘法除法,要想快数得到结果就只能考虑位运算。本题主要考察位运算。被除数减去一次除数,结果 +原创 2016-02-29 13:08:35 · 589 阅读 · 0 评论 -
[leetcode Q28] Implement strStr()
实现 c 函数 strstr() Implement strStr(). Returns the index of the first occurrence of needle in haystack, or -1 if needle is not part of haystack. 返回子字符串在原字符串中第一次匹配的位置,否则放回 -1实现还是比较简单的就是需要注意一些细节思路原创 2016-02-27 15:14:53 · 515 阅读 · 0 评论 -
[leetcode Q26] Remove Duplicates from Sorted Array
1. 题目要求 Given a sorted array, remove the duplicates in place such that each element appear only once and return the new length. Do not allocate extra space for another array, you must do this in原创 2016-02-27 14:14:17 · 437 阅读 · 0 评论 -
[leetcode Q20] Valid Parentheses
题目要求检查括号字符串序列是否匹配简单的算法是使用一个栈:做一个空栈,顺序扫描字符串直到末尾如果读到开放符号 “([{” 则入栈否则读到 “)]}”,检查栈是否为空,空则报错栈不空则检查栈顶元素是否与字符匹配扫描结束,检查栈是否为空,不空则报错cpp 实现代码如下: bool isValid(string s) { stack<char> helpStack;原创 2016-02-26 17:39:43 · 602 阅读 · 0 评论 -
[leetcode Q3] —— Longest Substring Without Repeating Characters
Given a string, find the length of the longest substring without repeating characters. For example, the longest substring without repeating letters for “abcabcbb” is “abc”, which the length is 3. For “原创 2016-02-25 22:15:39 · 637 阅读 · 0 评论 -
[leetcode Q9] Container With Most Water
题意是有个高度数组,就相当于隔板的高度,求数组中任意两隔板间盛水的最大量。隔板间的距离与较低隔板的高度乘积即为盛水的容量。类似于2Sum的思想,两边设一个指针,然后计算area,如果height[i] <= height[j],那么i++,因为在这里height[i]是瓶颈,j往里移只会减少面积,不会再增加area。这是一个贪心的策略,每次取两边围栏最矮的一个推进,希望获取更多的水。int maxA原创 2016-02-23 22:33:36 · 508 阅读 · 0 评论 -
[leetcode-Q5] Longest Palindromic Substring
要求一个字符串的最长回文子字符串。暴力解法的时间复杂度为 O3下面提供一个时间复杂度为 O2 的解法动态规划法动态规划是在暴力解法上进行优化,通过激励一些我们需要的东西,来避免暴力解法中很多重复的判断。假设 flag[i][j] 表示子串 s[i ... j] 是否时回文,那么对于动态规划表 flag 的打表方式如下:初始化:{flag[i][j]=trueothers=false(i>=j)原创 2016-02-21 22:45:59 · 554 阅读 · 0 评论 -
leetcode——String to Integer
问题实现自己的 atoi 字符转换函数。一直以来都有听说过在面试过程中会问到实现 atoi 函数,现在碰到这题,正好拿来练练手。思路核心思路就一条语句:(m 为要返回的整数)m = m * 10 + str[i] - ‘0’;需要注意比较多的细节删掉起始空格正符号判断删掉有效字符(0-9数字字符)后面的所有无效字符整数是否溢出围绕着那条核心语句,一点点将上述四点条件考虑进去,可以写出如下原创 2016-02-20 22:02:06 · 657 阅读 · 0 评论 -
leetcode——Reverse Integer
问题描述题目如下:Reverse digits of an integer.Example1: x = 123, return 321Example2: x = -123, return -321 完全有自己思考完成的第一道题,一道简单题。很容易就写出了如下代码:int reverse(int x) { int m = x/10; int n = x%10;原创 2016-02-20 19:38:03 · 447 阅读 · 0 评论